Choosing between C++ and C# depends on your goals; C++ is ideal for systems-level programming and performance-critical applications, while C# is better suited for Windows development and rapid application development.
// Simple C++ Hello World program
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
C++: An Overview
History and Evolution
C++ was developed by Bjarne Stroustrup in the late 1970s as an enhancement over the C programming language. It introduced object-oriented programming features to improve the management of large software projects. Over the years, C++ has evolved significantly through several standardized versions, each adding new functionalities and optimizations.
Key Features of C++
Performance and Efficiency
C++ is renowned for its high performance. By allowing direct manipulation of hardware and memory, C++ provides developers with the ability to write performance-critical applications. For example, C++ often outperforms interpreted languages like Python in execution speed. A simple benchmark could illustrate this, where computational tasks in C++ run several times faster than their Python counterparts.
Object-Oriented Programming
C++ supports key OOP principles such as encapsulation, inheritance, and polymorphism. This enables developers to create modular, maintainable, and reusable code. Here's a basic class definition in C++:
class Car {
public:
string model;
int year;
Car(string m, int y) {
model = m;
year = y;
}
void display() {
cout << "Model: " << model << ", Year: " << year << endl;
}
};
Use Cases for C++
System Programming
C++ is frequently used for system-level programming, creating operating systems and drivers. Its capability to perform low-level operations makes it indispensable in this domain.
Game Development
Due to its performance and control over hardware, C++ is widely used in game development. Major game engines like Unreal Engine utilize C++ for scripting and development.
Embedded Systems
In embedded systems, C++ plays a crucial role due to its efficiency and ability to work close to the hardware, making it a popular choice for IoT devices.
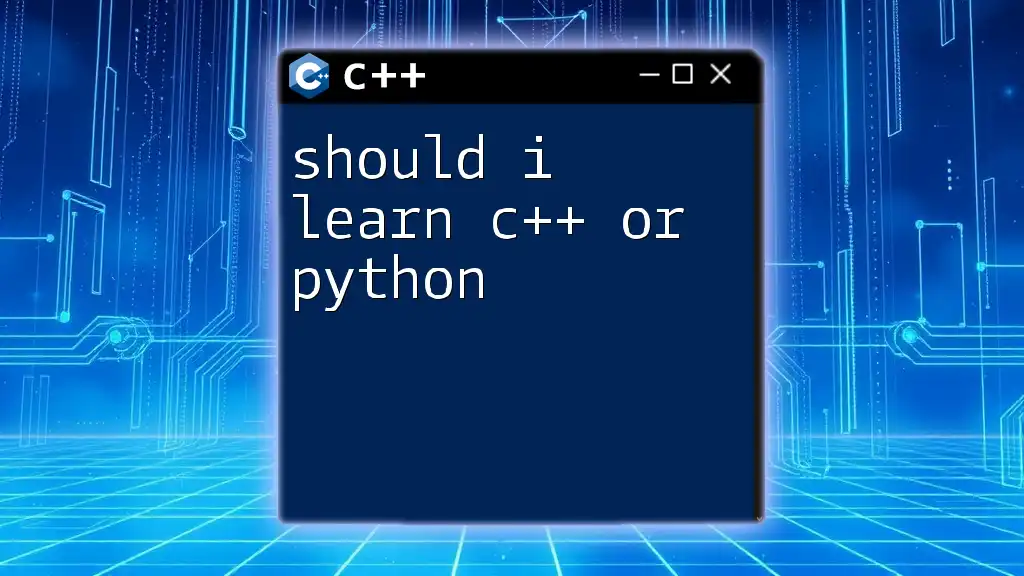
C#: An Overview
History and Evolution
C# was introduced by Microsoft in 2000 as part of the .NET initiative. The language was designed to support a wide range of applications while being easy to use, featuring a syntax similar to Java.
Key Features of C#
Ease of Use and Readability
C# is known for its clean and straightforward syntax, which allows developers to write readable code easily. Here’s a simple "Hello, World!" example in C#:
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
}
Rich Libraries and Frameworks
C# benefits from the extensive .NET framework, providing numerous libraries and tools that expedite development. This makes it ideal for desktop and web applications.
Use Cases for C#
Web Development with ASP.NET
C# is a staple in the realm of web development, particularly with ASP.NET, enabling the creation of dynamic, high-performance websites.
Windows Application Development
The language excels in creating native Windows applications. WPF (Windows Presentation Foundation) allows for rich user interface design. An example would be creating a simple WPF application where developers can leverage XAML for UI and C# for business logic.
Game Development with Unity
Unity is one of the most popular game development platforms, extensively using C#. Developers can easily script game behaviors in C#, making it accessible for both budding and experienced game developers.
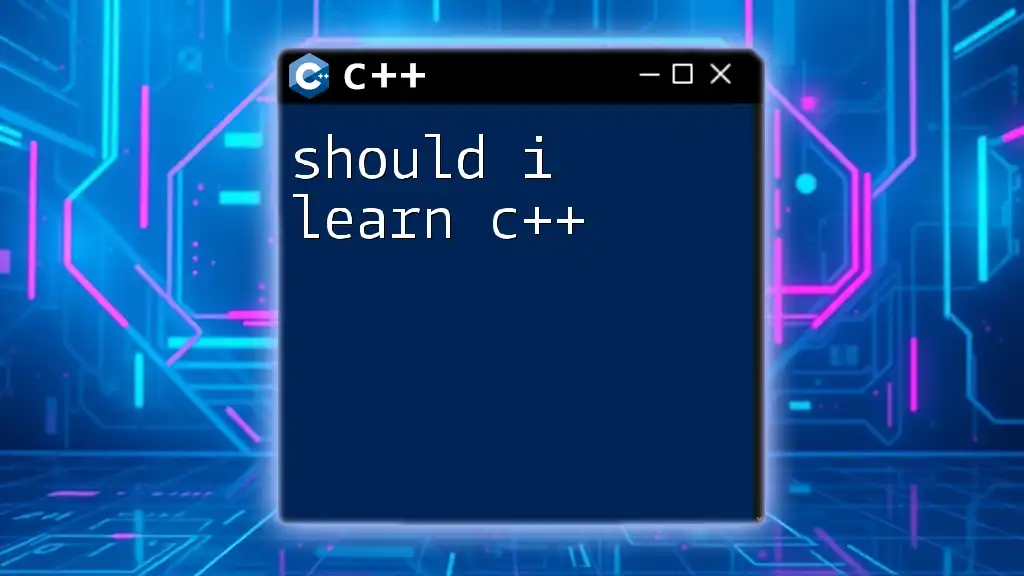
C++ vs C#: Key Differences
Performance and Control
When discussing whether to learn C++ or C#, the performance aspect must be highlighted. C++ allows for low-level memory management, enabling developers to optimize their applications meticulously. Conversely, C# manages memory through garbage collection, which simplifies development but may lead to performance overhead in critical applications.
Complexity vs Simplicity
C++ often presents a steeper learning curve due to its intricate syntax and features like pointers and manual memory management. C#, however, is designed to be user-friendly, making it easier to pick up for beginners.
Community and Ecosystem
Both languages have vibrant communities and robust ecosystems. C++ boasts long-standing prowess in performance-related domains, while C# showcases a modern approach centered around application development, particularly with cutting-edge technologies like cloud computing.
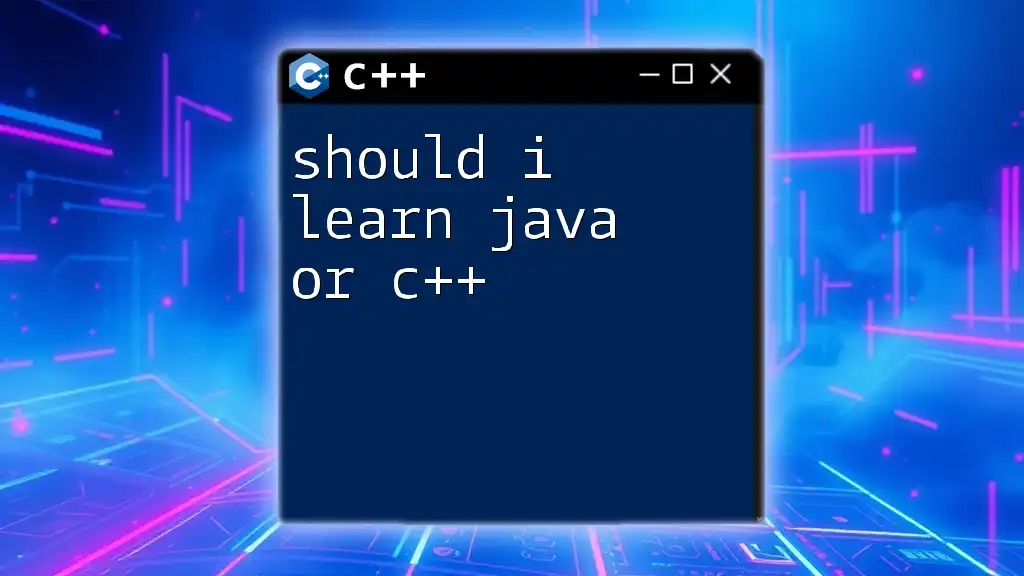
Choosing the Right Language for You
Consider Your Goals
The choice between C++ and C# should align with your career aspirations. If you are interested in system-level programming, game development, or high-performance applications, C++ may be more beneficial. On the other hand, if you're inclined towards web development, application development on Windows, or game development with Unity, C# is likely the better fit.
Learning Curve
Both languages have their unique challenges. C++ may require a more significant investment in understanding concepts related to memory management and system architecture, whereas C# often allows quicker entry into productivity. To ease the learning process, consider starting with C# if you are a beginner, then transition to C++ as you gain confidence.
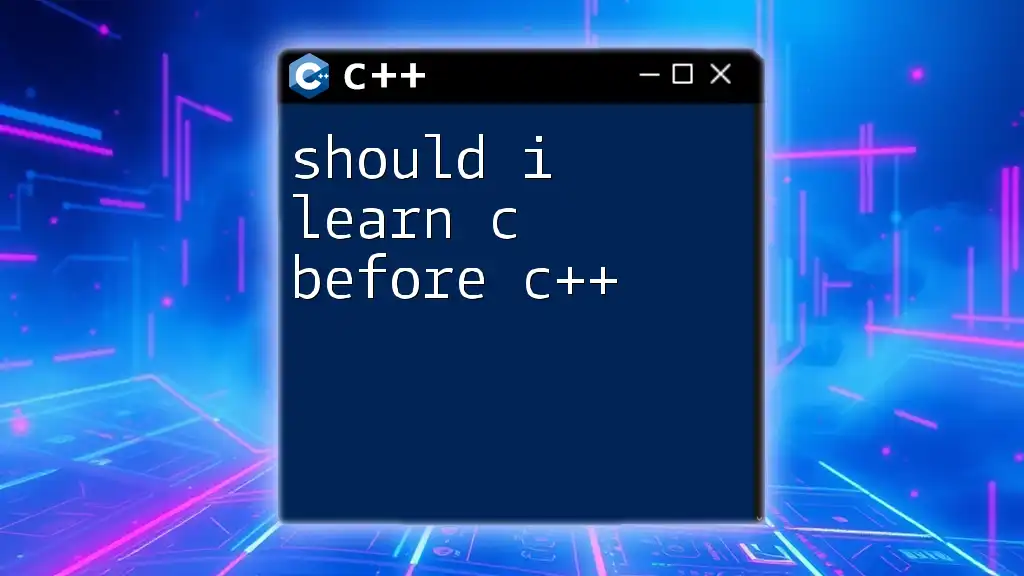
Future Trends: C++ and C# Together
Emerging Technologies
Both languages play significant roles in emerging technologies. C++ remains vital in fields requiring high performance, like gaming and machine learning, while C# continues to thrive in enterprise applications and cloud computing.
Learning Both Languages
There's considerable value in learning both languages, as they can complement each other. Understanding C++ enhances your problem-solving skills, and knowing C# opens doors to modern application development. Transitioning between the two becomes more manageable as you develop your foundation in programming principles.
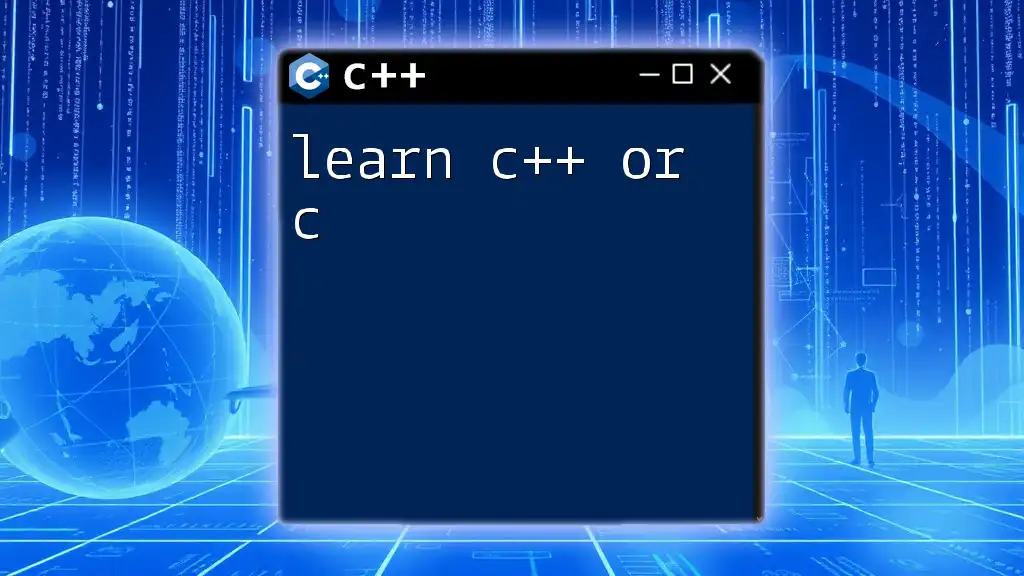
Conclusion
In making the decision about whether to learn C++ or C#, take into account your goals, the areas that you find most exciting, and the types of projects you wish to engage in. Ultimately, both languages are powerful tools that can lead you to a variety of career paths in software development. Your choice should reflect not only current trends but also your personal interests and aspirations in the tech industry.
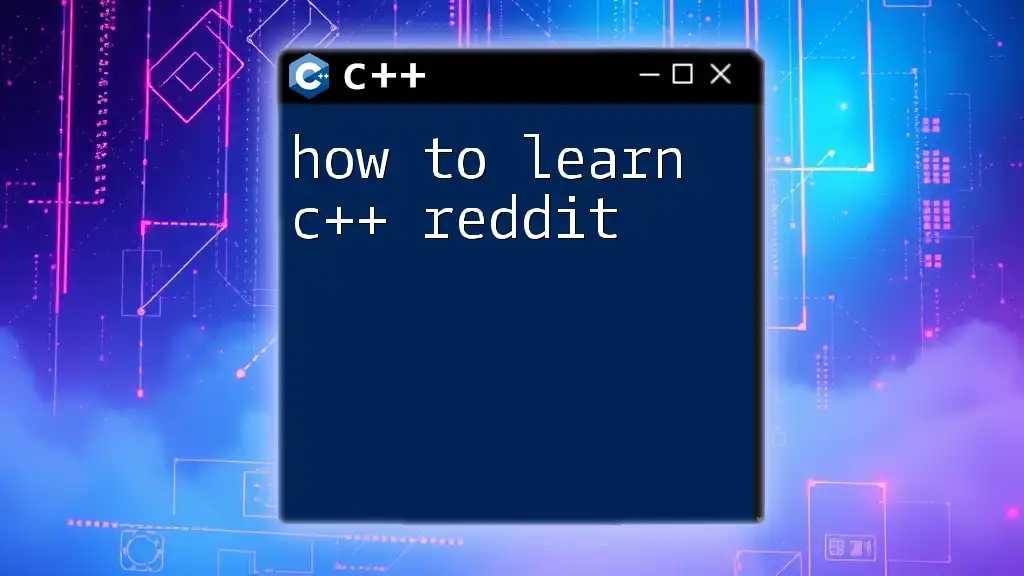
References and Resources
For further exploration into C++ and C#, consider the following resources:
- Online courses on platforms like Coursera or Udemy
- Books such as "Effective C++" by Scott Meyers and "C# 9.0 in a Nutshell" by Joseph Albahari
- The official documentation of C++ and C# for in-depth understanding of language features and libraries.