When deciding whether to learn Java or C++, consider that C++ offers more control over system resources and performance, while Java provides a simpler syntax and built-in memory management, making it ideal for beginners.
Here's a simple C++ code snippet demonstrating a basic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a powerful, high-performance programming language that was developed as an extension of the C programming language. Created by Bjarne Stroustrup in the early 1980s, C++ combines the efficiency of C with features that support object-oriented programming (OOP). This makes it suitable for systems programming, software development, and applications that demand high performance.
Key features of C++ include:
- Object-Oriented Programming: Facilitates code reuse and organization through classes and inheritance.
- Low-Level Manipulation: Allows access to hardware resources, making it flexible for tasks requiring precise control.
- Standard Template Library (STL): A collection of generic classes and functions, providing algorithms and data structures.
Advantages of Learning C++
Performance and Efficiency
One of the standout qualities of C++ is its unmatched performance. Because C++ is close to the hardware, it is often the language of choice for performance-critical applications like game engines, real-time systems, and high-frequency trading platforms. With C++, developers can write code that executes quickly and efficiently.
For example, popular game engines like Unreal Engine are built with C++, allowing developers to harness the full capability of system resources.
Control Over System Resources
C++ provides developers with explicit control over system memory and hardware resources. The language offers pointers, references, and manual memory management features, enabling developers to allocate and deallocate memory as needed. This capability, while powerful, requires a solid understanding of memory management to avoid leaks and segmentation faults.
Here is a simple example of dynamic memory allocation in C++:
int* ptr = new int(5); // Dynamically allocate memory
delete ptr; // Free the memory
Object-Oriented Programming
With its robust support for object-oriented principles, C++ allows developers to create modular and reusable code. Developers can define classes and objects, giving them flexibility in building both simple and complex applications.
For instance, consider this class structure:
class Base {
public:
virtual void show() { cout << "Base class"; }
};
class Derived : public Base {
public:
void show() override { cout << "Derived class"; }
};
In this example, the `Derived` class inherits from `Base` and overrides its `show()` method; this allows for polymorphism, a core principle of OOP.
Disadvantages of Learning C++
Complex Syntax
While C++ is powerful, its syntax can be overwhelming for beginners. The intricate details of pointers, templates, and multiple inheritance can introduce a steep learning curve. Beginners may initially struggle to grasp these concepts, which could frustrate them.
Manual Memory Management
The responsibility of managing memory can lead to potential errors if not handled correctly. Developers must be vigilant about memory allocation and deallocation to prevent leaks and buffer overflows. Such errors can be particularly tricky to debug, especially for those new to programming.

Understanding Java
What is Java?
Java is a widely-used, high-level programming language that was developed by Sun Microsystems (now owned by Oracle) in the mid-1990s. Java emphasizes portability, readability, and simplicity. It was designed with the mantra “Write Once, Run Anywhere,” thanks to the Java Virtual Machine (JVM), which enables Java applications to run on any platform that has a JVM installed.
Key features of Java include:
- Object-Oriented: Promotes principles of encapsulation, inheritance, and polymorphism.
- Automatic Memory Management: Contains a built-in garbage collector that automatically manages memory allocation and deallocation.
- Rich API: Offers extensive libraries and frameworks for various applications, including web and mobile development.
Advantages of Learning Java
Platform Independence
One of Java's most significant advantages is its platform independence. When Java code is compiled, it is translated into bytecode that can run on any device equipped with the JVM. This "write once, run anywhere" approach eliminates compatibility issues across different operating systems and hardware.
Rich Standard Library
Java boasts a comprehensive set of built-in libraries that simplify tasks like networking, data manipulation, and user interface design. This functionality allows developers to leverage existing resources without reinventing the wheel.
Here’s a simple Java example that demonstrates the use of an ArrayList, part of Java's Collections Framework:
import java.util.ArrayList;
ArrayList<String> list = new ArrayList<>();
list.add("Hello, Java!"); // Adding an element to the list
Robustness and Security
Java's design emphasizes robustness and security. The language's strong type-checking at both compile-time and runtime minimizes errors. Java also has a robust garbage collection mechanism that helps manage memory by automatically reclaiming unused memory.
Disadvantages of Learning Java
Performance Overhead
While Java is user-friendly, its performance can lag compared to C++. This is mainly due to the overhead of the JVM and garbage collection. For high-performance applications where every millisecond matters, C++ may still be the better choice.
Less Control Over System Resources
Java's automatic memory management is a double-edged sword. While it simplifies memory handling, it deprives developers of fine-grained control over system resources. This abstraction can hinder performance optimization in critical areas.

Comparison: C++ vs. Java
Learning Curve and Ease of Use
When it comes to ease of use, Java typically has a gentler learning curve than C++. While C++ has intricate syntax and requires a solid understanding of pointers and memory management, Java's syntax is more straightforward, making it easier for beginners to learn.
Application Domains
Where C++ Excels
C++ shines in areas requiring rigorous performance and control, such as:
- Game Development: Many gaming engines and AAA titles are written in C++ due to its performance capabilities.
- System Software: Operating systems and drivers often rely on C++ for their efficiency.
- Embedded Systems: Real-time applications and embedded systems often necessitate the direct control provided by C++.
Where Java Excels
Java is preferred in areas such as:
- Web Applications: Java’s robustness and extensive libraries make it suitable for building scalable web applications.
- Enterprise Solutions: Java is widely used in large-scale enterprise applications due to its security and stability.
- Android Development: Java is one of the primary languages for developing Android applications.
Community and Ecosystem
The programming community for both languages is vast. C++ has a strong community focused on systems programming and game development, while Java has a larger ecosystem around web development, cloud solutions, and enterprise software.
There are abundant resources available for learning both languages, including:
- Books: "The C++ Programming Language" for C++ and "Effective Java" for Java.
- Online Courses: Platforms like Coursera, edX, and Udemy offer extensive courses for both languages.
- Forums and Community Support: Online communities such as Stack Overflow, Reddit, and specialized forums are excellent places for learning and troubleshooting.
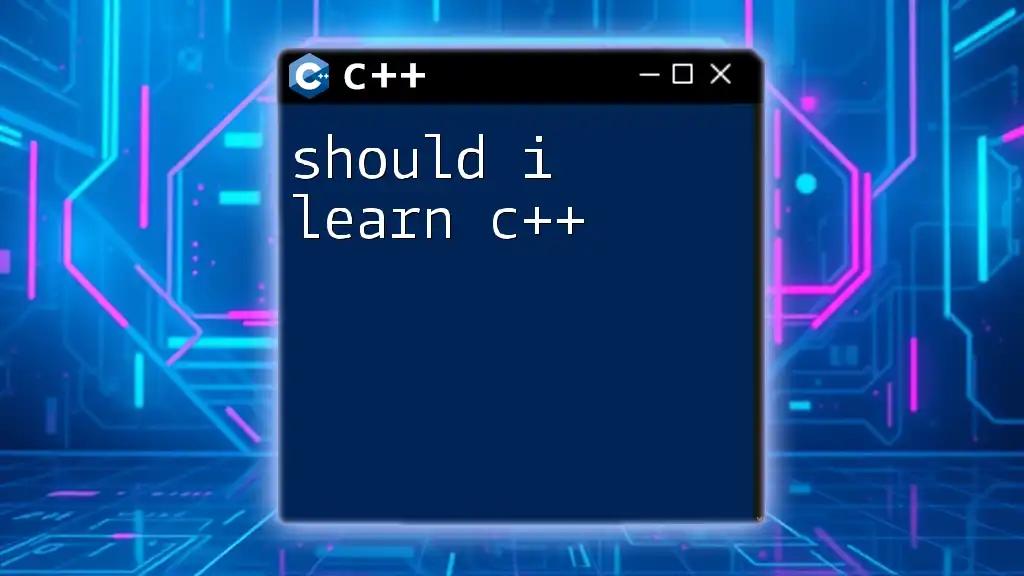
Conclusion
Should I Learn C++ or Java?
Deciding whether to learn C++ or Java hinges on your career goals and interests. If you're passionate about game development, system programming, or performance-critical applications, then C++ is likely the better choice. Conversely, if you are looking to enter fields like web development, enterprise solutions, or mobile applications, Java is a strong candidate.
Both languages have their merits, and there’s value in learning one over the other based on the trajectory you envision for your programming career. Exploring both languages can provide a comprehensive view of programming paradigms and enhance your skill set.
Additional Resources
To further support your learning journey, consider these resources:
- Tutorials and Documentation: Refer to official documentation like cppreference.com for C++ and Oracle's Java documentation.
- Online Learning Platforms: Engage in platforms like Codecademy or LeetCode to practice coding challenges in both languages.
- Community Engagement: Join forums and discussion groups to connect with peers and experienced programmers.
Final Thoughts
Ultimately, the journey into programming can be enriching and fulfilling. Stay curious, experiment with both languages, and enjoy the learning process!