In C++, boolean values represent true or false states, which are fundamental for controlling program flow and logic.
Here’s a simple example demonstrating the use of boolean values:
#include <iostream>
int main() {
bool isRaining = false;
if (isRaining) {
std::cout << "Don't forget your umbrella!" << std::endl;
} else {
std::cout << "Enjoy your day!" << std::endl;
}
return 0;
}
What are Boolean Values?
Boolean values are fundamental to programming, representing truth values in the context of logical operations. In C++, the Boolean data type is denoted as `bool`, which can hold one of two possible values: true or false. These values are crucial for decision-making processes within a program, enabling the execution of specific code based on conditions.

Understanding the Basics of Boolean Values
What is a Boolean?
In C++, a Boolean is a data type that exclusively represents one of two states: true or false. This binary nature makes Boolean values ideal for controlling program flow and logical operations. Understanding how to leverage Boolean values is essential for effective programming in C++.
The Syntax of Boolean Variables
Declaring Boolean variables is straightforward. You can define a Boolean variable by simply stating its type and assigning it a value:
bool isActive = true;
bool isComplete = false;
In this example, `isActive` is set to true, indicating that it is in an active state, while `isComplete` is set to false, signifying that a task is not finished.

The Role of Boolean Operators
Logical Operators
Logical operators play a vital role in evaluating Boolean expressions. The three primary logical operators in C++ are:
- AND (`&&`): This operator evaluates to true only if both operands are true.
- OR (`||`): This operator evaluates to true if at least one of the operands is true.
- NOT (`!`): This operator negates the truth value, converting true to false and vice versa.
Examples of Logical Operators
Here’s how you can use these logical operators in conditional statements:
if (isActive && isComplete) {
// Execute if both isActive and isComplete are true
}
In this example, the code block executes only when both `isActive` and `isComplete` are true.
Comparison Operators
Comparison operators are also integral to working with Boolean values, as they return a Boolean result based on the relation of two values. Key comparison operators include:
- `==`: Equal to
- `!=`: Not equal to
- `>`: Greater than
- `<`: Less than
- `>=`: Greater than or equal to
- `<=`: Less than or equal to
Example Code Snippets
You can illustrate comparisons with the following snippets:
bool isEqual = (5 == 5); // Evaluates to true
bool isGreater = (10 > 5); // Evaluates to true
In these examples, `isEqual` is true because both operands match, while `isGreater` confirms that 10 surpasses 5.
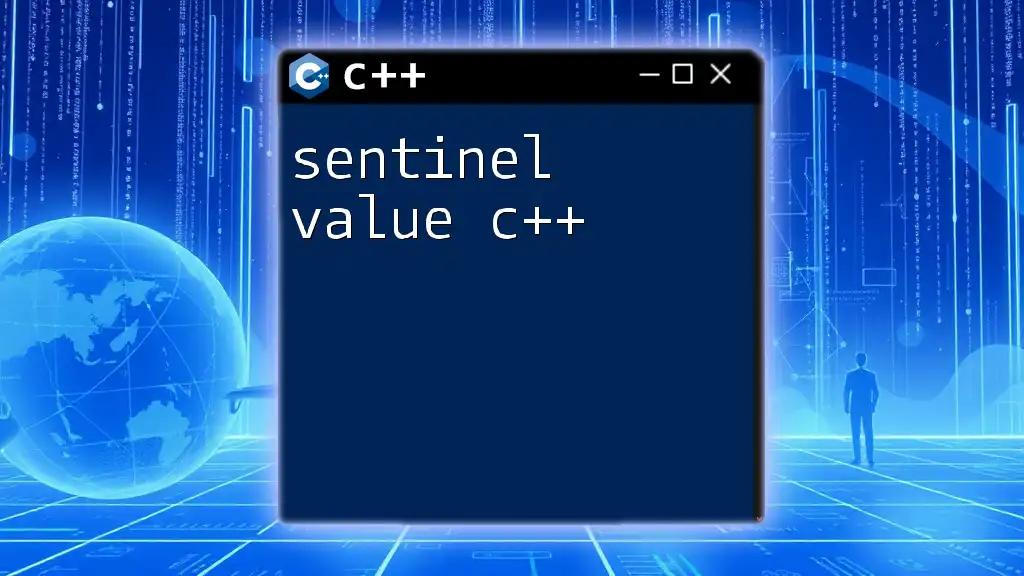
Conditional Statements Using Booleans
If Statements
One of the most common uses of Boolean values is in `if` statements, where conditions are evaluated to determine which block of code to execute. For instance:
if (isActive) {
// Code to execute if isActive is true
}
In the above example, if `isActive` is true, the code within the `if` block will run.
Switch Statement
While Boolean values don’t directly work with switch-case structures, you can use them in a broader logical context. For instance, based on Boolean conditions, you can control flow using nested cases or flags.
Ternary Operator
The ternary operator provides a concise way to assign values based on a Boolean condition. It follows the syntax `condition ? value_if_true : value_if_false`. Here's an example:
bool isWeekend = true;
string activity = isWeekend ? "Relax" : "Work";
In this example, if `isWeekend` is true, the variable `activity` will be set to "Relax"; otherwise, it will be "Work".
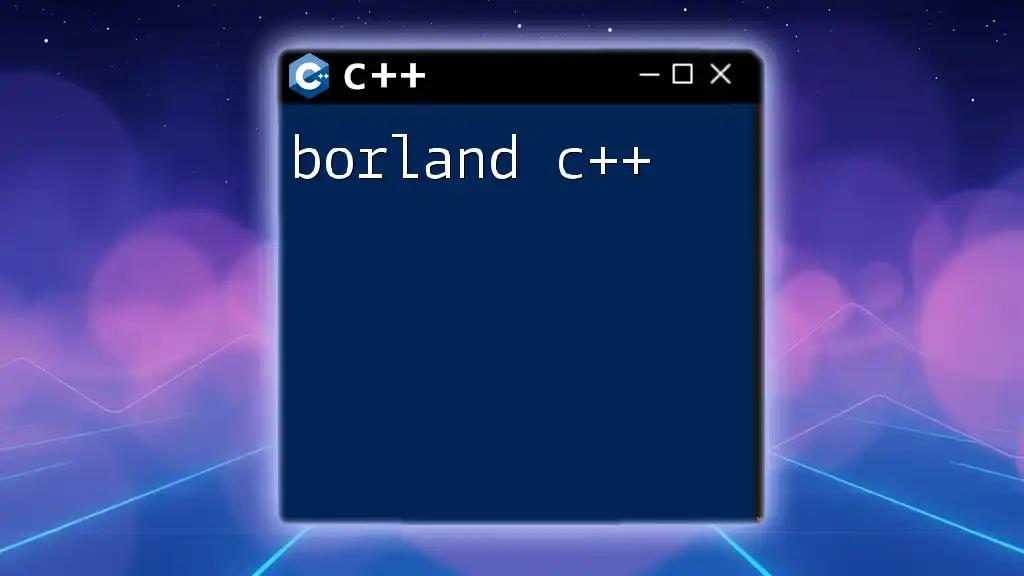
The Importance of Boolean Logic in Algorithms
Control Flow
Boolean values are instrumental in controlling program execution flow. They dictate logical pathways in your code, determining which blocks are executed based on specific conditions, ultimately enhancing decision-making capabilities.
Boolean Algebra in Programming
Boolean algebra forms the backbone of logical operations within programming. It allows developers to implement complex logical expressions and conditions succinctly, contributing to cleaner and more efficient code.

Common Mistakes and Best Practices
Common Mistakes
Ineffective use of Boolean expressions can lead to bugs and logical errors. Common pitfalls include misunderstanding negations or misplacing logical operators.
Best Practices for Using Boolean Values
To ensure best practices, consider using clear and descriptive naming conventions for your Boolean variables. This enhances code readability and assists in understanding the program's logic at a glance. For instance, instead of naming a variable `flag`, you might name it `isUserLoggedIn` to convey its purpose clearly.

Debugging and Testing with Boolean Values
Techniques for Troubleshooting
When debugging Boolean values, ensure that you examine the logic used in conditions carefully. Adding print statements can help clarify which paths your program is taking.
Using Assertions
Assertions are a powerful way to validate assumptions in your code. By using assertions, you can automatically check that your Boolean conditions hold true during execution:
assert(isActive == true);
This line of code will trigger an error if `isActive` is not true during runtime, assisting you in maintaining logical integrity throughout your program.
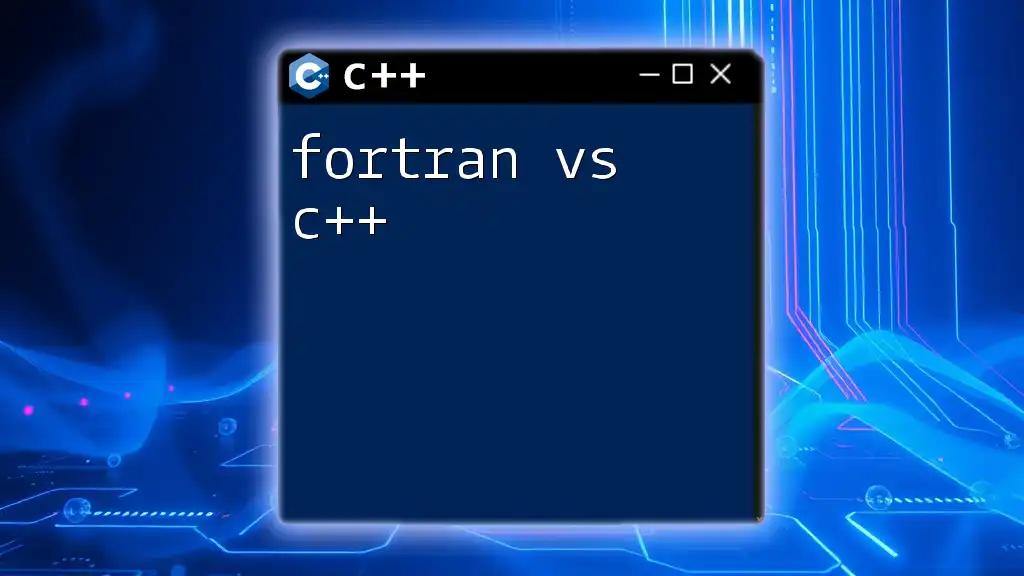
Conclusion
In summary, mastering boolean values in C++ is crucial for effective programming. By understanding their basic syntax, logical and comparison operators, and how to use them in conditional statements, developers can create more robust and efficient code. Practice using these concepts with various examples to reinforce your understanding and elevate your programming skills.