In C++, you can obtain the ASCII value of a character by simply casting it to an integer type, which yields its corresponding integer representation.
#include <iostream>
int main() {
char character = 'A';
int asciiValue = static_cast<int>(character);
std::cout << "The ASCII value of '" << character << "' is " << asciiValue << std::endl;
return 0;
}
Understanding ASCII Values
What are ASCII Values?
ASCII, or the American Standard Code for Information Interchange, is a character encoding standard that assigns unique numerical values to characters, allowing computers to represent text. Initially developed in the early 1960s, ASCII has become a cornerstone of computing, enabling consistent text representation across various systems. The standard ASCII set ranges from 0 to 127, with a corresponding character for each value, while extended ASCII includes values from 128 to 255, accommodating additional symbols and characters.
Common ASCII Values
In ASCII, each character has a corresponding value. The notable characters in the standard range include:
- Control characters (0-31): Non-printable characters, such as newline (`\n`), tab (`\t`), and carriage return (`\r`).
- Printable characters (32-126): These include digits (0-9), uppercase letters (A-Z), lowercase letters (a-z), punctuation marks, and space.
Understanding these values is fundamental when manipulating characters in C++ programming.
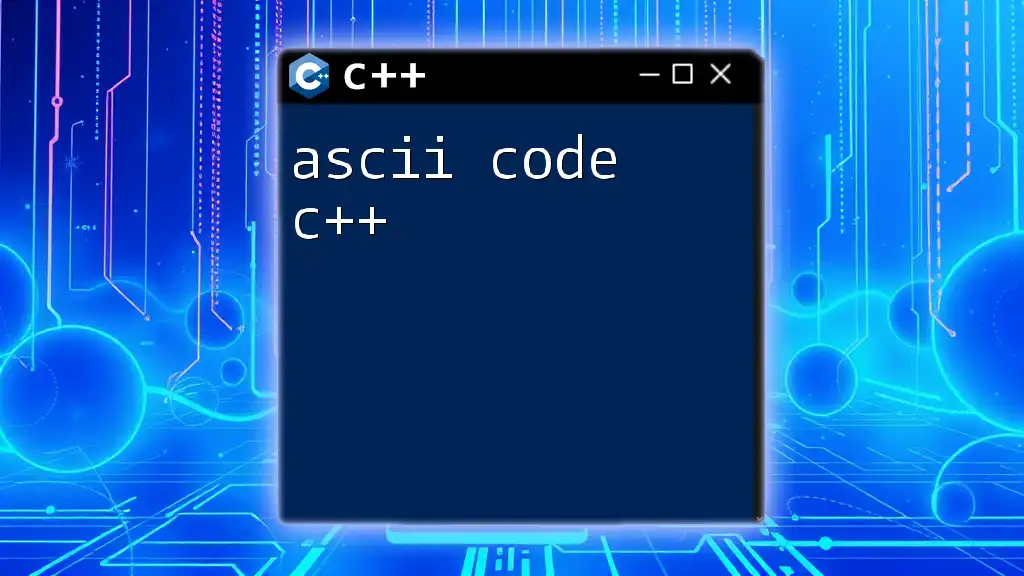
How to Use ASCII Values in C++
Accessing ASCII Values
In C++, the `char` type allows you to store a single character. You can retrieve its ASCII value by using the `static_cast` operator. This is a convenient way to convert the `char` to an `int`, which gives you its ASCII representation.
char character = 'A';
int asciiValue = static_cast<int>(character);
std::cout << "ASCII value of " << character << " is " << asciiValue << std::endl;
Example Code: Displaying ASCII Values of Characters
You can create simple programs to iterate through characters and display their ASCII values. Here's a foundational example that displays ASCII values for uppercase letters:
#include <iostream>
int main() {
for (char c = 'A'; c <= 'Z'; c++) {
std::cout << "ASCII value of " << c << " is " << static_cast<int>(c) << std::endl;
}
return 0;
}
This program iterates from `A` to `Z`, showcasing how you can utilize loops in C++ to explore ASCII values dynamically.
Inputting Characters and Displaying ASCII Values
You can also allow user input to analyze ASCII values interactively:
#include <iostream>
int main() {
char inputChar;
std::cout << "Enter a character: ";
std::cin >> inputChar;
std::cout << "ASCII value of " << inputChar << " is " << static_cast<int>(inputChar) << std::endl;
return 0;
}
In this snippet, the program prompts the user for input, enabling them to discover the ASCII value for any character they choose.
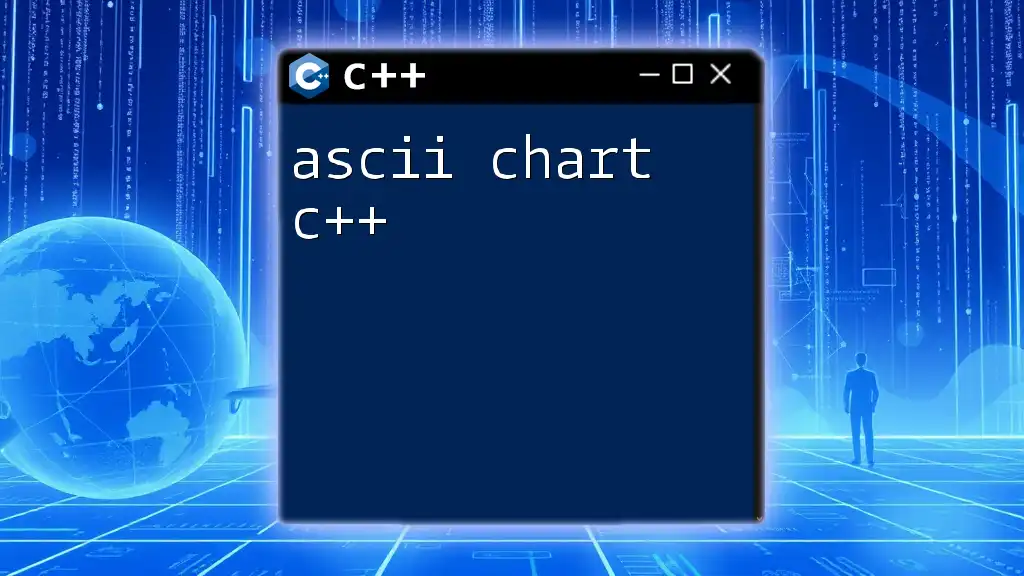
Manipulating ASCII Values
Character Arithmetic
Characters in C++ can be manipulated just like integers. This means you can use arithmetic operations such as addition and subtraction. For example, you can increment a character to get the next character in the ASCII table:
char ch = 'A';
ch++;
std::cout << "Next character after A is " << ch << std::endl; // Output: B
This feature allows you to perform operations like encoding and decoding messages by shifting characters based on their ASCII values.
Converting ASCII Values to Characters
If you have an ASCII value and wish to convert it back to its corresponding character, you can do so using `static_cast` as well. This is often helpful when you're working with numerical data that represents characters:
int asciiValue = 65;
char character = static_cast<char>(asciiValue);
std::cout << "Character for ASCII value " << asciiValue << " is " << character << std::endl;
This code demonstrates the reverse conversion, illustrating how easily you can navigate between characters and their ASCII equivalents in C++.
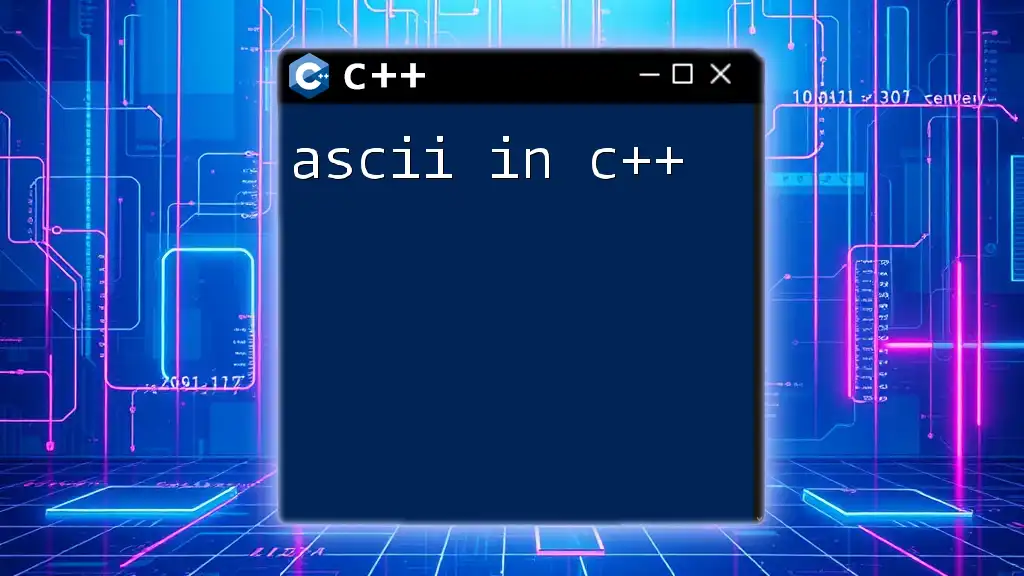
ASCII Values in Strings
Getting ASCII Values for Each Character in a String
When dealing with strings, you can iterate through each character to fetch their ASCII values. This is particularly useful when analyzing or manipulating text data.
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
for (char c : str) {
std::cout << "ASCII value of " << c << " is " << static_cast<int>(c) << std::endl;
}
return 0;
}
In this example, we iteratively check each character in the string "Hello," demonstrating how to loop through and obtain individual character ASCII values.
Example Application: Counting Characters Based on ASCII Ranges
You can extend your understanding of ASCII values to include more complex operations, such as counting specific characters based on their ASCII values. Here’s a simple program that counts uppercase characters in a given string:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello World!";
int uppercaseCount = 0;
for (char c : str) {
if (c >= 'A' && c <= 'Z') {
uppercaseCount++;
}
}
std::cout << "Uppercase character count: " << uppercaseCount << std::endl;
return 0;
}
This code efficiently demonstrates how to analyze strings using ASCII conditions, further showcasing the versatility of character manipulation in C++.
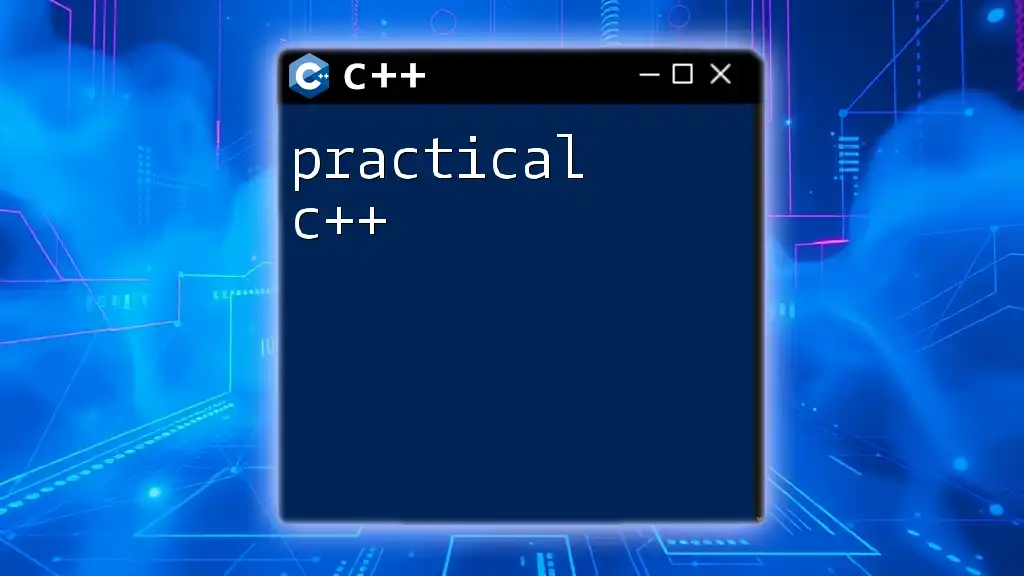
Conclusion
Throughout this article, we explored the fundamental concepts of ASCII value in C++. Understanding how to manipulate and utilize ASCII values is essential for efficient text processing in programming. ASCII values have practical applications in a variety of programming tasks, from user input processing to character encoding and more.
For anyone looking to deepen their C++ programming skills, exploring ASCII values is a fantastic starting point. Experiment with the provided code snippets and methods to familiarize yourself with character manipulation in C++.
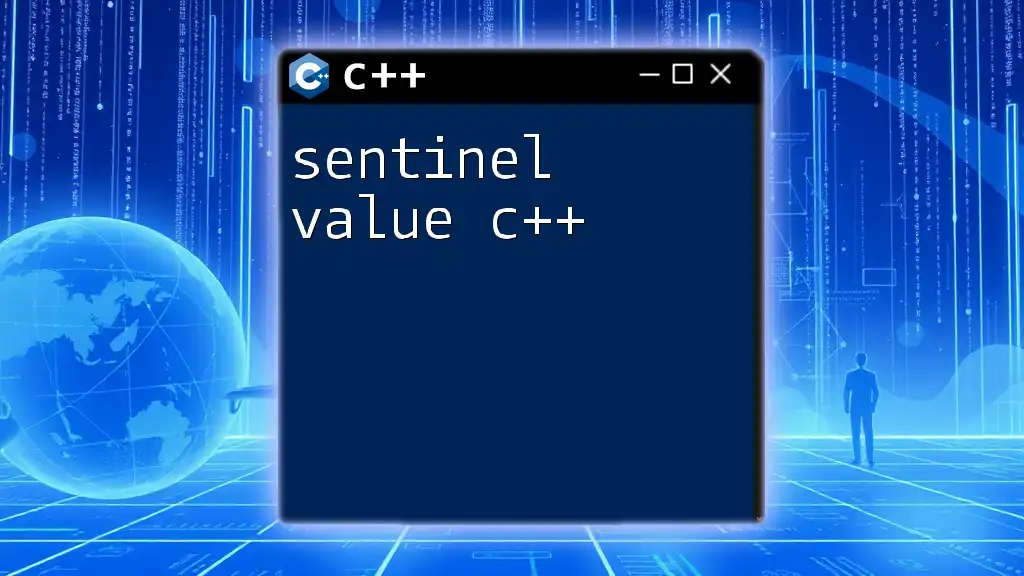
Additional Resources
For more comprehensive learning, consider exploring textbooks dedicated to C++ programming and online tutorials that elaborate on character encoding and manipulation techniques. Several reputable platforms offer valuable insights and exercises that will strengthen your grasp of the subject.
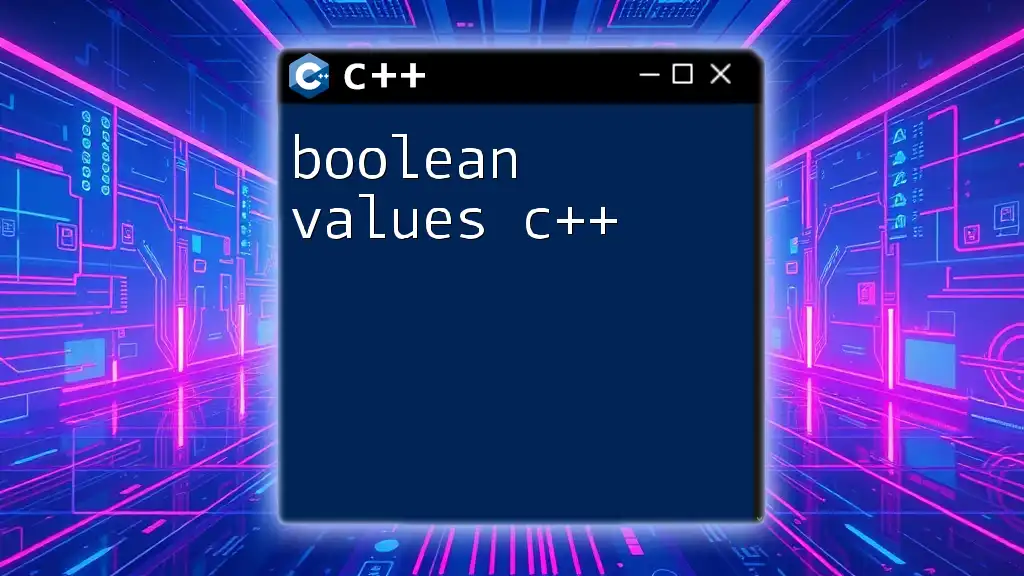
FAQs
If you have any lingering questions about ASCII values and their utilization in C++, do not hesitate to explore online forums, programming communities, and additional educational materials. They can provide further clarification and insight into the nuances of working with ASCII in your programming journey.