Objective-C++ is a programming language that combines Objective-C's object-oriented features with C++'s powerful capabilities, allowing developers to use both languages seamlessly in the same source file.
Here's a simple example demonstrating the integration of Objective-C and C++:
#import <Foundation/Foundation.h>
class CPPClass {
public:
void showMessage() {
std::cout << "Hello from C++!" << std::endl;
}
};
int main() {
@autoreleasepool {
CPPClass cppObj;
cppObj.showMessage();
NSString *greeting = @"Hello from Objective-C!";
NSLog(@"%@", greeting);
}
return 0;
}
What is Objective C++?
Understanding the Basics
Objective C++ is a powerful hybrid programming language that brings together the dynamic and reflective capabilities of Objective-C with the efficiency and performance of C++. This allows developers to utilize the strengths of both languages, enabling more complex and efficient applications, particularly in the context of macOS and iOS. The entwined nature of C++ and Objective-C allows developers to create rich, object-oriented applications while taking advantage of existing C++ libraries and codebases.
Key Features of Objective C++
Objective C++ inherits the essential features of both languages:
- Object-oriented programming: Code can be organized using classes and objects, promoting reuse and modularity.
- Dynamic typing and messaging: Borrowing from Objective-C, it allows runtime flexibility, where methods can be called dynamically.
- C++ features: Makes it possible to leverage C++ templates, STL (Standard Template Library), and strong type checking, thus enhancing performance and functionality.
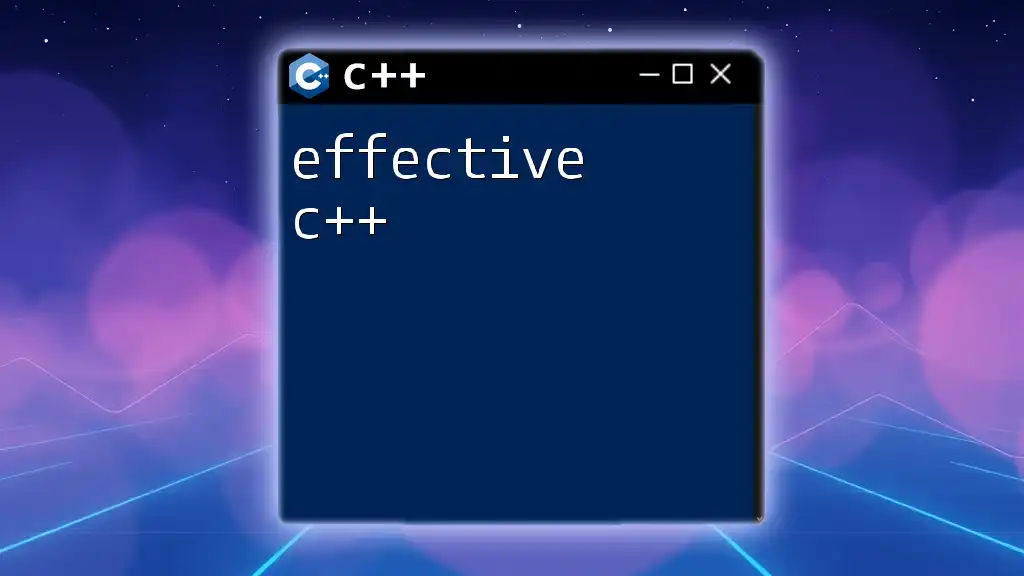
Setting Up an Objective C++ Environment
Tools Required
To begin with Objective C++, you will primarily need a capable Integrated Development Environment (IDE). The go-to choice is Xcode, Apple's official IDE for macOS and iOS development.
Creating a New Objective C++ Project
Getting started with a new Objective C++ project in Xcode is straightforward:
- Open Xcode and select "Create a new Xcode project."
- Choose the appropriate template for your application (e.g., macOS App).
- Set your project name and ensure all project details are filled out.
- When adding new files, use the `.mm` extension for Objective C++ files, signaling that they can contain both C++ and Objective-C code.
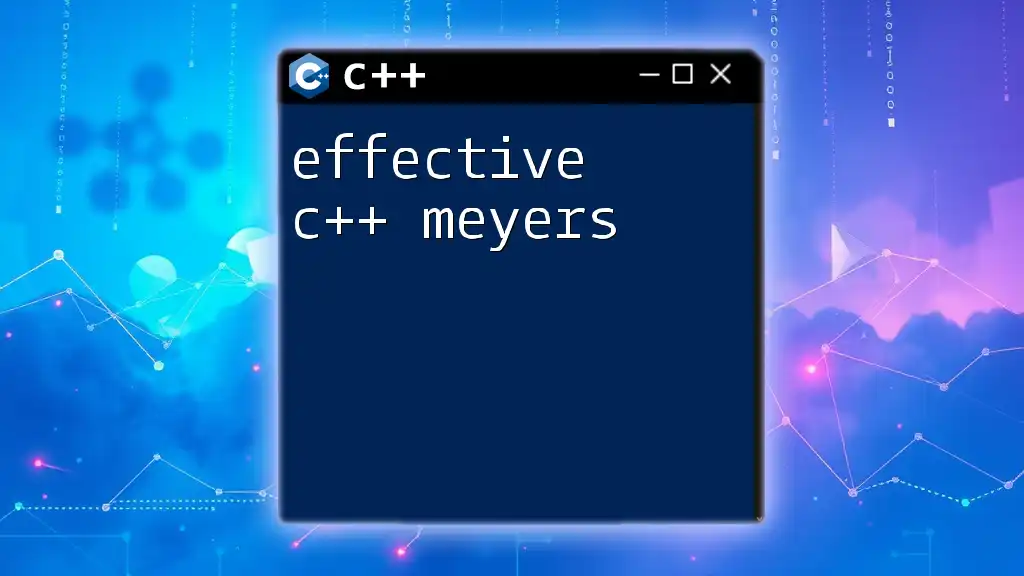
Syntax and Structure of Objective C++
Basic Syntax Rules
When you combine C++ code with Objective-C in the same file, it is critical to follow a few general syntax rules. Use the `.mm` extension to signal the compiler to treat the file as Objective C++. This allows you to seamlessly intersperse C++ code within Objective-C code without modification.
Key Differences from Standard C++
While Objective C++ maintains heritage from both languages, there are a few key differences to note:
- Message passing: In Objective-C, method calls use a unique messaging syntax which cannot be directly translated to standard C++. Instead of calling methods directly, Objective-C uses `sendMessage:` syntax, which facilitates dynamic method resolution.
- Memory management: Objective-C employs Automatic Reference Counting (ARC) to manage memory, while C++ requires explicit memory management (e.g., using `new` and `delete`). Understanding the distinctions between these two paradigms is vital to leverage the capabilities of Objective C++.
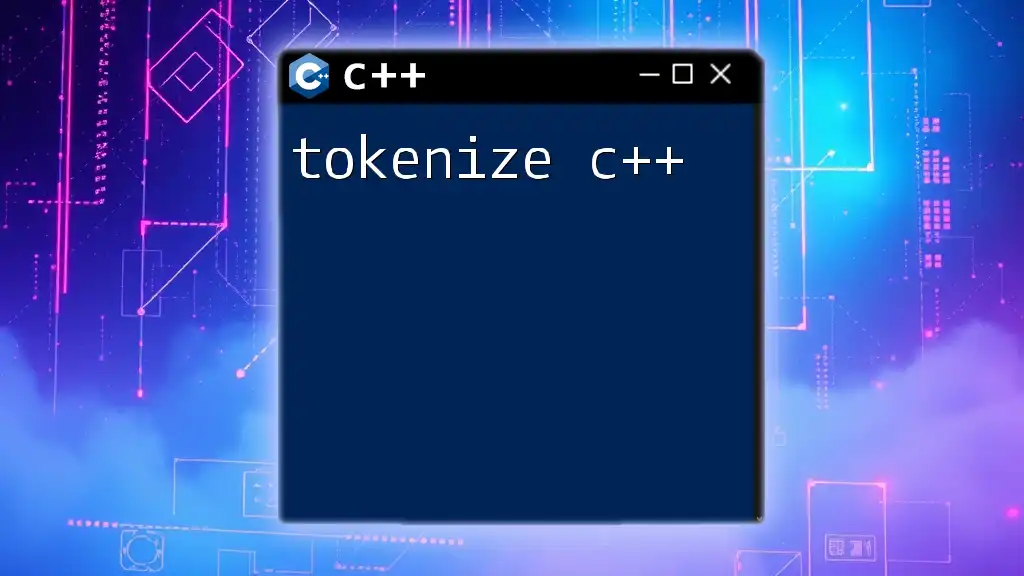
Working with Classes and Objects
Defining Objective C++ Classes
Creating a simple Objective C++ class can be achieved through the following structure:
class MyClass : public NSObject {
@property (nonatomic, strong) NSString *name;
- (void) printName;
};
// Implementation
@implementation MyClass
- (void) printName {
NSLog(@"%@", self.name);
}
@end
In this example, `MyClass` inherits from `NSObject`, allowing it to function within the Cocoa framework. The `printName` method logs `name` to the console. The use of properties facilitates memory management through ARC.
Interacting with C++ Objects
To create and utilize C++ classes within an Objective C++ context, here's an example of a simple class:
class Example {
public:
void greet() {
std::cout << "Hello from C++!" << std::endl;
}
};
To utilize the `Example` class within Objective C++ code, you can initialize an object and call its method:
Example example;
example.greet();
This allows you to take full advantage of C++ functionalities while still leveraging Objective-C's features.
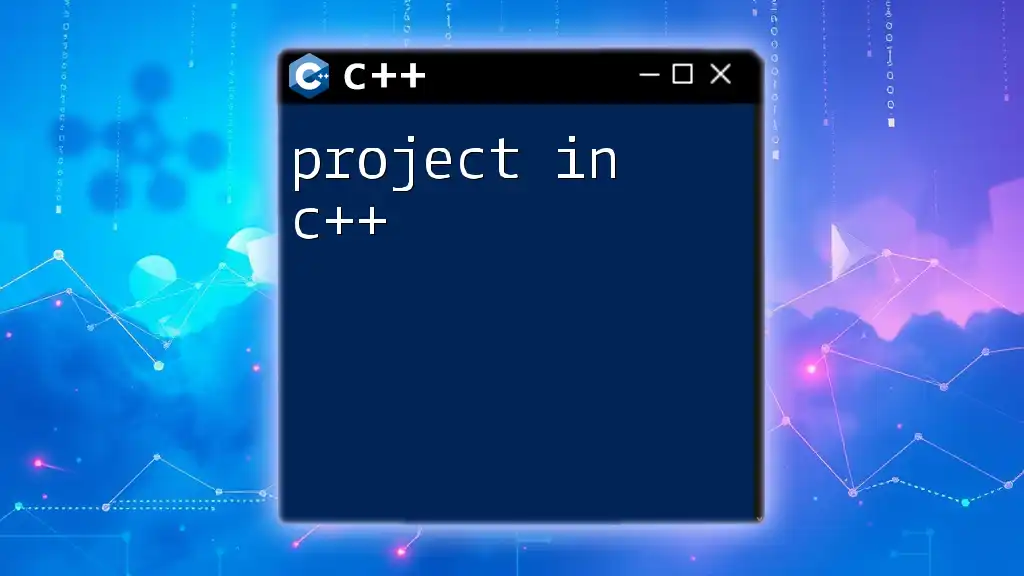
Bridging C++ and Objective-C
Using C++ Libraries in Objective-C
Bridging C++ libraries into your Objective C++ project is seamless. Begin by including the desired C++ headers in your Objective C++ files. This can be achieved with the `#include` directive, enabling you to call C++ functions and methods directly from your Objective-C code.
Challenges and Solutions
When mixing these two powerful languages, various challenges may arise. For example, managing objects' memory across C++ and Objective-C can lead to confusion. A solid strategy is to encapsulate C++ code in classes that handle their own memory management, thus clarifying ownership and cleanup.
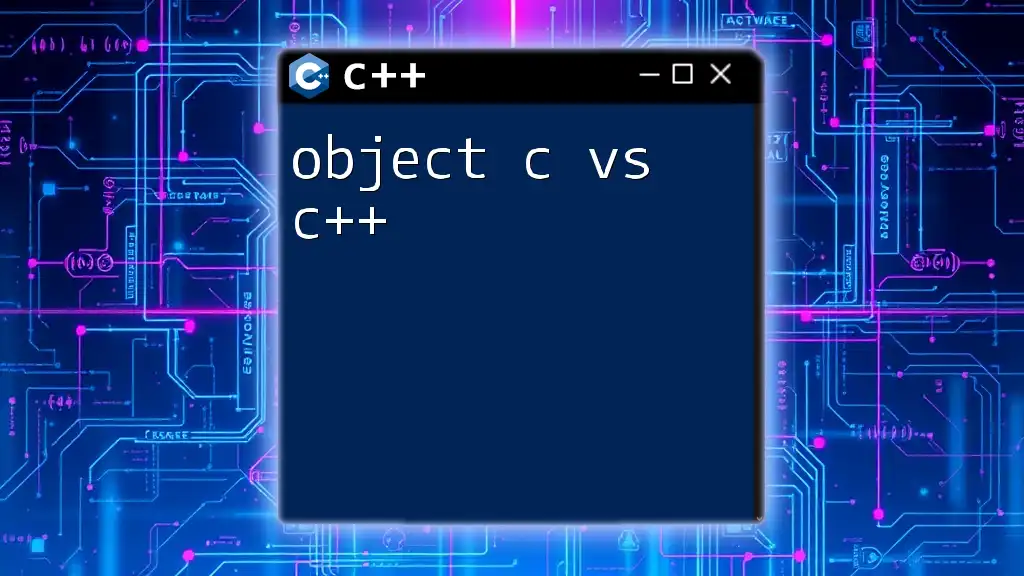
Memory Management in Objective C++
ARC vs. Manual Reference Counting
Understanding how memory is managed in Objective C++ is critical. ARC simplifies memory management for Objective-C objects, while traditional C++ requires more explicit control:
- ARC: Automatic Reference Counting efficiently tracks object references and frees memory when an object is no longer in use, promoting safety and reducing memory leaks.
- Manual reference counting: It is pivotal in C++ to manage memory around `new` and `delete`. Ensure to balance allocations with proper deallocations to avoid leaks.
Memory Management Example
Here’s a brief code snippet demonstrating mixing ARC with manual C++ management:
class ManualMemory {
public:
ManualMemory() { /* allocate resources */ }
~ManualMemory() { /* cleanup */ }
};
@implementation SomeClass
- (void)doSomething {
ManualMemory *mem = new ManualMemory();
// Use mem...
delete mem; // Manual cleanup required.
}
@end
In this example, memory management practices must be strictly observed to prevent leaks.
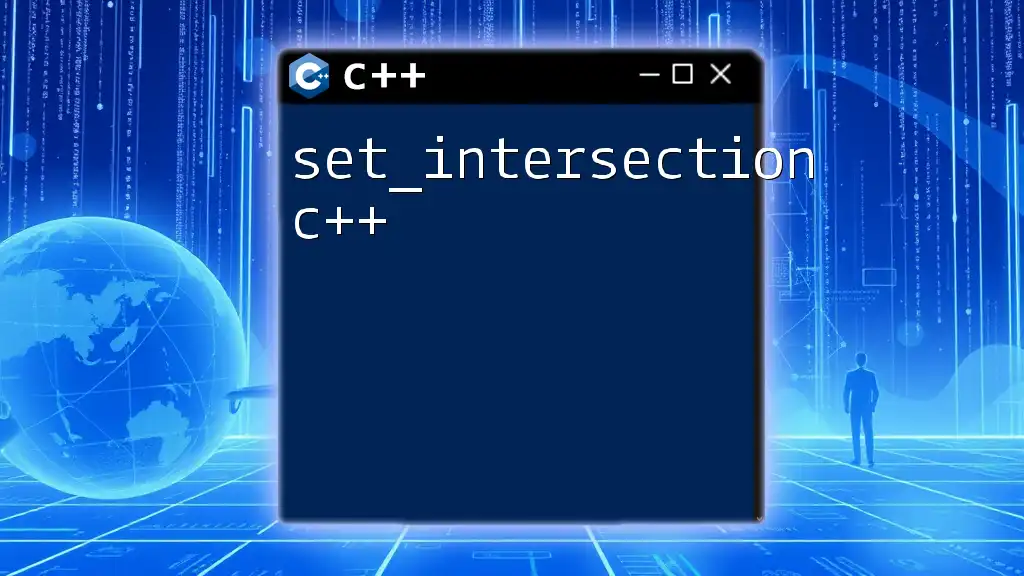
Error Handling in Objective C++
Exception Handling
Handling exceptions can vary between C++ and Objective-C. Objective C provides built-in exception handling via `@try` and `@catch`. The code below illustrates a mix of Objective-C exception handling with C++:
@try {
// Potentially dangerous C++ code
throw std::runtime_error("Example exception");
} @catch (NSException *exception) {
std::cerr << "Caught Objective-C exception: " << exception << std::endl;
}
This structure ensures that both Objective-C and C++ exceptions get handled appropriately.
Using NSError with C++
Integrating NSError into C++ operations can add robustness to error handling. When a method encounters an error in C++, you may convert it into a format that Objective-C understands, like so:
void exampleMethod(NSError **error) {
// Open a file operation
if (/* failure condition */) {
*error = [NSError errorWithDomain:@"CustomErrorDomain"
code:1001
userInfo:nil];
}
}
This allows clear communication of errors back to the Objective-C environment.
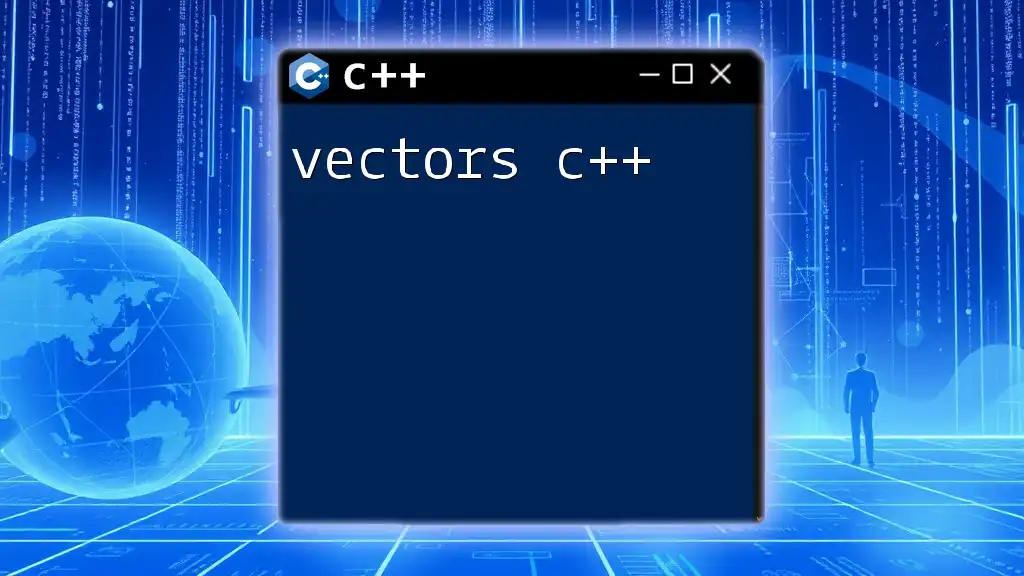
Best Practices for Objective C++
Maintainable and Readable Code
To write maintainable code in Objective C++, adhere to best practices that enhance clarity:
- Utilize clear naming conventions.
- Implement thorough inline documentation to elucidate complex sections of your code.
- Ensure consistent code formatting to improve readability.
Performance Considerations
Performance is paramount in any application development. When combining C++ and Objective-C, it's essential to be aware of the following:
- Reduce Overhead: Avoid excessive messaging in Objective-C where possible; favor direct method calls in C++.
- Use In-line functions: They often yield better performance in C++ than traditional function calls.
Be vigilant of how data is passed between languages; prefer pointers or references where necessary to minimize copying costs.
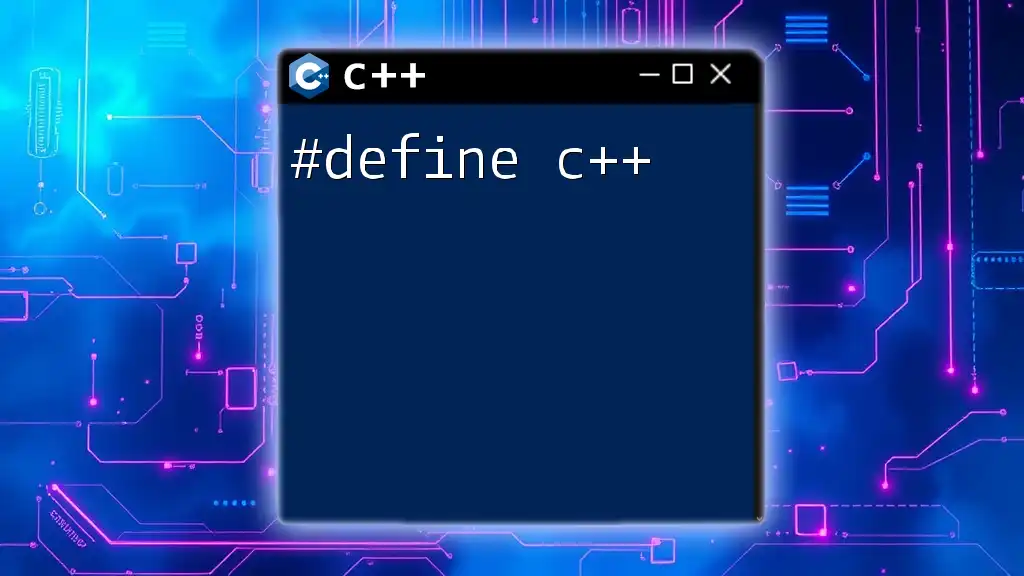
Real-World Applications of Objective C++
Popular Use Cases
Objective C++ shines in various domains, particularly in:
- Game Development: Combining the efficiency of C++ with the flexibility of Objective-C can lead to high-performance games.
- Performance-intensive Applications: Applications requiring heavy processing, such as image processing software or data analytics tools, benefit from Objective C++.
Case studies of well-implemented Objective C++ projects highlight its strengths in real-world applications.
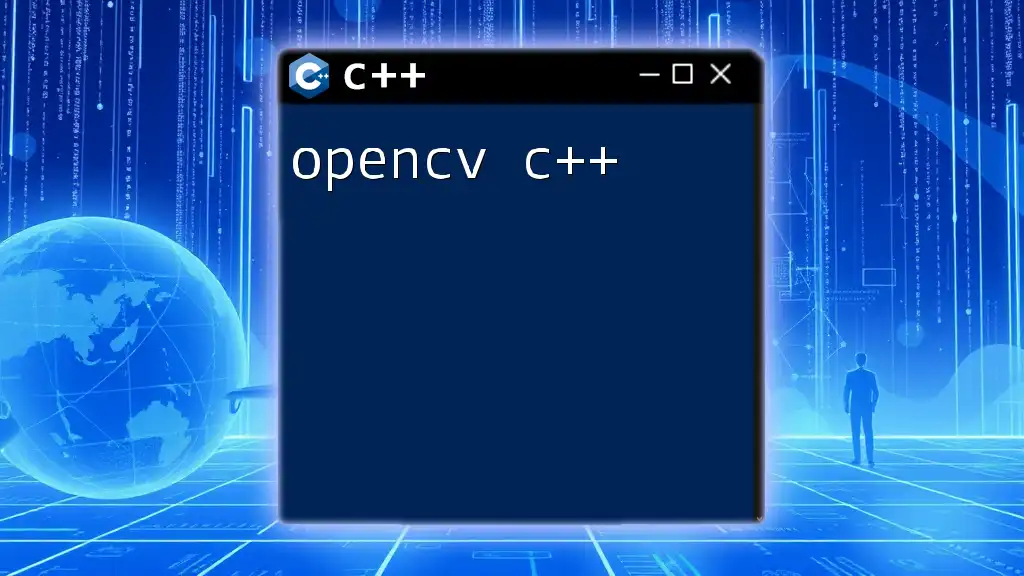
Conclusion
In conclusion, Objective C++ serves as a compelling tool for developers looking to integrate the features of both Objective-C and C++. By understanding its syntax, structure, and best practices, programmers can create powerful, efficient applications for macOS and iOS. The future of programming continues to evolve; mastering Objective C++ opens numerous opportunities for developers leveraging cutting-edge technology.
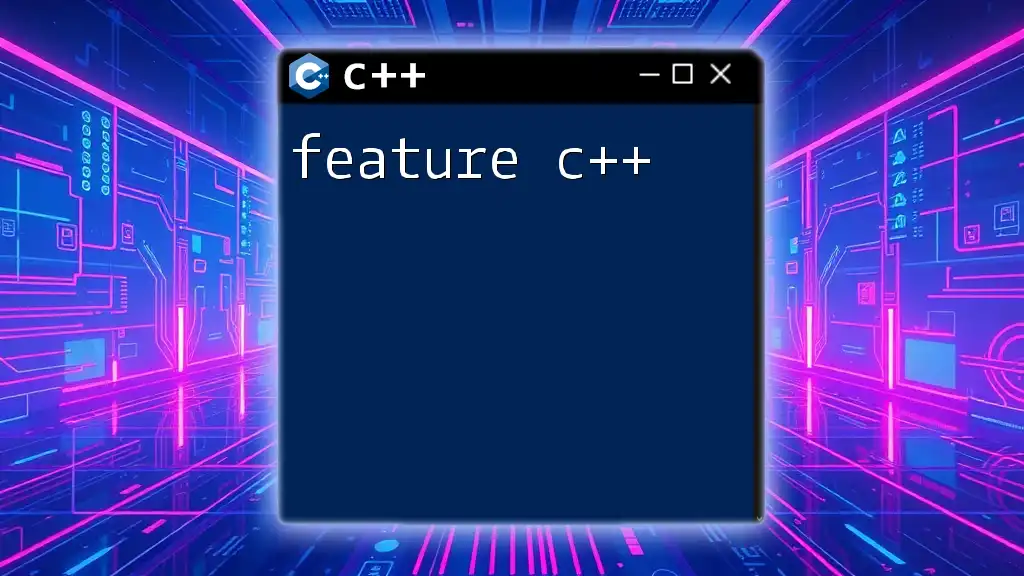
Additional Resources
For readers interested in furthering their knowledge, consider exploring online courses specializing in Objective C++ and reviewing documentation on modern C++ practices. Community forums can also provide valuable insights and solutions to specific challenges encountered during development.