Effective Modern C++ emphasizes the use of C++11 and C++14 features to write cleaner, safer, and more efficient code while leveraging best practices.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> numbers = {4, 2, 7, 1, 3};
std::sort(numbers.begin(), numbers.end());
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is Modern C++?
Modern C++ refers to the evolution of the C++ programming language from its earlier versions (C++98, C++03) to the more recent standards, starting with C++11 and progressing through C++14, C++17, and the most current C++20. Each new iteration introduced features aimed at improving the language's efficiency, safety, and expressiveness.
Key principles of effective modern C++ include:
- Efficiency: Enabling faster and smarter resource management.
- Abstraction: Allowing developers to write higher-level code that is cleaner and easier to understand.
- Safety: Offering tools and mechanisms to reduce the risk of bugs and memory leaks.
The importance of utilizing Modern C++ cannot be overstated as it brings a host of advantages over legacy approaches that are still prevalent in many industries, with enhanced performance and better type safety being foremost.
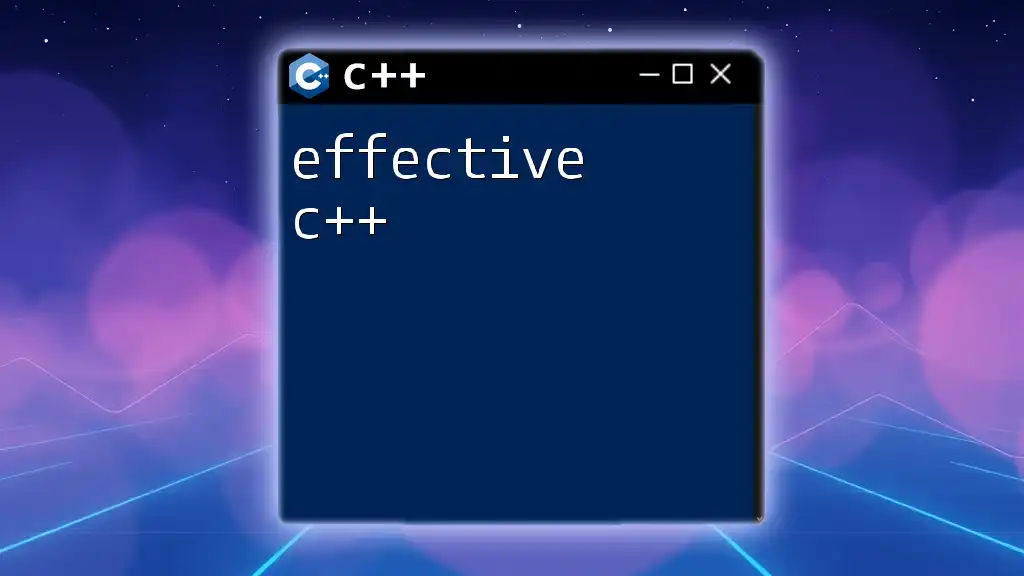
Key Features of Modern C++
Smart Pointers
Understanding Smart Pointers
Smart pointers are a foundation of modern C++ resource management, implementing RAII (Resource Acquisition Is Initialization). They automatically manage memory allocation and deallocation, reducing the likelihood of memory leaks.
The main types of smart pointers include:
- `std::unique_ptr`: Represents exclusive ownership. Only one `unique_ptr` can own a particular resource at a time.
- `std::shared_ptr`: Allows multiple pointers to share ownership of a resource.
- `std::weak_ptr`: Works in conjunction with `std::shared_ptr` to prevent circular references.
Code Example: Using Smart Pointers
#include <iostream>
#include <memory>
void smartPointerExample() {
std::unique_ptr<int> ptr = std::make_unique<int>(5);
std::cout << *ptr << std::endl; // Outputs: 5
}
In this example, `std::unique_ptr` takes ownership of the dynamically allocated integer, ensuring the memory is automatically freed when it goes out of scope.
Auto Type Deduction
Benefits of Automatic Type Inference
The `auto` keyword enhances code readability by allowing the compiler to deduce the type of a variable automatically. This reduces the verbosity of declarations and improves code maintenance.
Code Example: Auto Keyword
auto number = 42; // inferred as int
auto message = "Hello"; // inferred as const char*
Effective use of `auto` makes the code clearer. Nonetheless, it’s essential to avoid overusing it as it can sometimes decrease code readability and make type determination unclear for other developers.
Range-Based For Loops
Simplifying Iteration
Range-based for loops simplify the way we iterate over collections, making the code cleaner and more expressive compared to traditional loops.
Code Example: Range-Based For Loop
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (const auto& num : numbers) {
std::cout << num << " ";
}
This code demonstrates how to iterate through a vector seamlessly, prioritizing clarity and reducing the likelihood of off-by-one errors that are common in traditional loops.
Lambda Expressions
What Are Lambdas?
Lambdas are a powerful feature of Modern C++ that allows you to define anonymous functions at the point where they are used. They are versatile and can be stored, passed, or executed immediately.
Code Example: Using Lambdas
auto add = [](int a, int b) { return a + b; };
std::cout << add(3, 4); // Outputs: 7
Lambdas can profoundly streamline programming workflows, particularly in operations requiring callbacks, such as in `std::sort` or event handling systems.
Move Semantics
Understanding Move Semantics
Move semantics is a game-changing feature in modern C++ that provides optimizations by moving resources rather than copying them. This avoids unnecessary overhead and enhances performance, especially for large objects.
Code Example: Implementing Move Semantics
#include <iostream>
#include <string>
class MyClass {
public:
MyClass(std::string str) : data(std::move(str)) {}
private:
std::string data;
};
By employing move constructors, your code not only runs faster but also benefits from a cleaner approach to memory management.
The Standard Library and Containers
Importance of Using STL
The Standard Template Library (STL) provides incredibly powerful generic types and algorithms that enhance productivity and maintainability. By using STL containers, you benefit from tested and optimized implementations rather than managing raw arrays, which can lead to errors.
Code Example: Using STL Containers
#include <vector>
std::vector<int> squares;
for (int i = 0; i < 10; ++i) {
squares.push_back(i * i);
}
The `std::vector` container automatically handles dynamic resizing and memory management, allowing developers to focus on application logic instead.
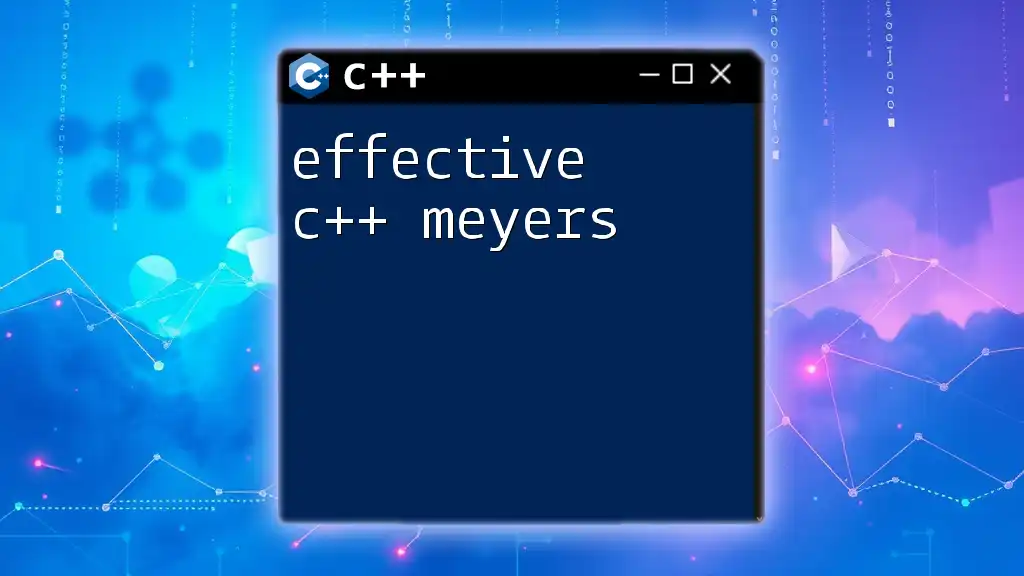
Best Practices for Effective Modern C++
Writing Clear and Concise Code
Writing clear and concise code is paramount. Consider using meaningful variable names and structuring your code intuitively. Comments should provide clarity and context, especially for complex algorithms or business logic, while remaining succinct.
Leveraging Compile-Time Mechanisms
Modern C++ offers powerful compile-time tools such as `constexpr`, enabling the execution of functions at compile time. This not only improves performance but also ensures certain properties of your program are checked during compilation, minimizing runtime errors.
Code Example: Compile-Time Calculations
constexpr int square(int x) { return x * x; }
Using `constexpr` effectively ensures that certain values are computed ahead of time, which can lead to performance optimizations in your applications.
Exception Handling
Modernized Error Handling
C++ has refined how exceptions are handled, allowing more specific and intentional error management. This improvement means developers can focus on anticipating and catching errors without cluttering their core logic.
Code Example: Safe Exception Handling
try {
// Some code that can throw
} catch (const std::exception& e) {
std::cerr << "Error occurred: " << e.what() << std::endl;
}
Employing catch blocks effectively empowers developers to create more robust applications by ensuring graceful handling of unforeseen circumstances.
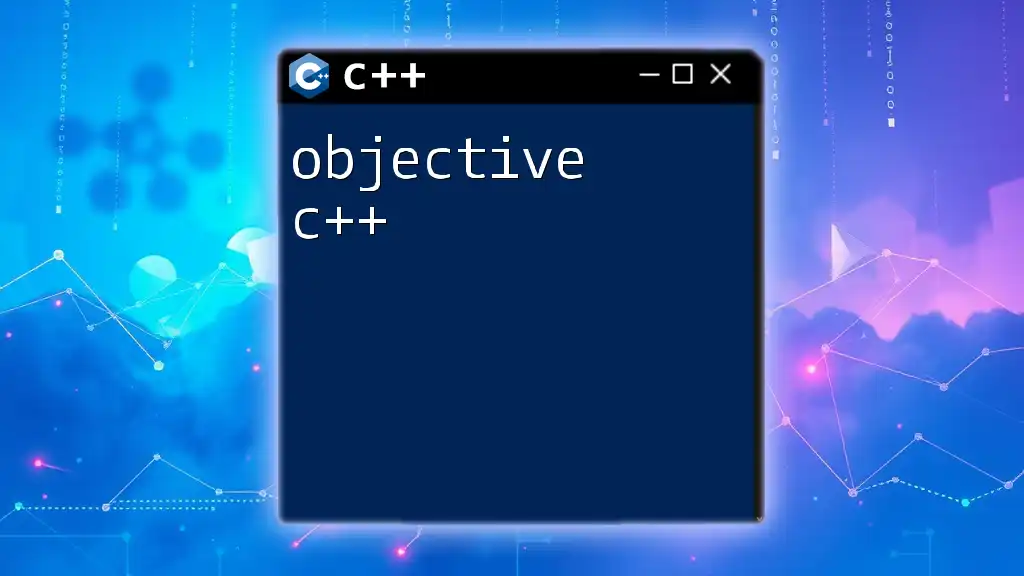
Conclusion
In summary, embracing effective modern C++ enables developers to write cleaner, more efficient, and maintainable code. The highlighted features—smart pointers, `auto` type deduction, range-based for loops, lambda expressions, move semantics, and the STL—each contribute significantly to this goal.
Transitioning to Modern C++ not only enhances your programming capabilities but also can drastically improve productivity and code quality in your projects. So, take the plunge, adopt modern techniques, and witness the transformation in your development experience.
Additional Resources
To further deepen your understanding of Modern C++, consider exploring reputable books, online courses, and community forums dedicated to C++ programming. They offer valuable insights and support for both beginners and seasoned developers.
Call to Action
We’d love to hear your experiences and thoughts about Modern C++. Feel free to share your insights or questions in the comments section and engage with our community for collaborative learning!