Object C is an object-oriented programming language primarily used for macOS and iOS development, while C++ is a general-purpose programming language that extends C with object-oriented features, allowing for more extensive and complex application development.
Here’s a simple code snippet showcasing a class definition in both languages:
// C++ example
class MyClass {
public:
void display() {
std::cout << "Hello from C++!" << std::endl;
}
};
// Objective-C example
@interface MyClass : NSObject
- (void)display;
@end
@implementation MyClass
- (void)display {
NSLog(@"Hello from Objective-C!");
}
@end
What is Objective-C?
Definition and History
Objective-C is a powerful programming language that extends the C language by adding Smalltalk-like messaging features. Developed in the early 1980s, it was the primary language used by Apple for iOS and macOS applications until Swift's arrival. Its influence can be seen in Objective-C's dynamic typing and message-passing capabilities, which enable robust runtime interactions.
Key Features
-
Dynamic Typing: In Objective-C, types are determined at runtime. This flexibility allows for rapid development but can introduce runtime errors if not handled properly.
Example:
id myObject = [[MyClass alloc] init]; [myObject greet]; // No need to specify the object's type
-
Message Sending: Instead of function calls, Objective-C uses a unique syntax for sending messages to objects. This is akin to sending commands, rather than calling functions.
Example:
[myObject performSelector:@selector(greet)];
-
Object-Oriented Programming: Objective-C supports classes, inheritance, and encapsulation, allowing developers to model complex systems effectively.
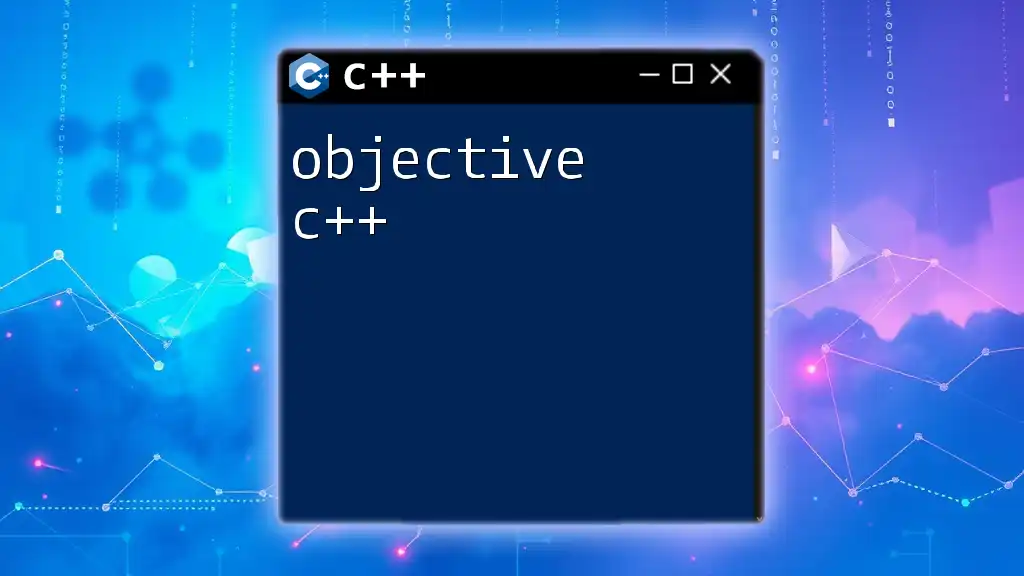
What is C++?
Definition and History
C++ is a multi-paradigm programming language that enhances the C programming language with standard libraries to support object-oriented programming (OOP) principles. Developed by Bjarne Stroustrup in the early 1980s, C++ has evolved significantly, now being widely used for system-level programming, game development, and performance-intensive applications.
Key Features
-
Static Typing: In C++, data types are determined at compile time, promoting type safety and generating errors at compile time rather than runtime.
Example:
MyClass obj; obj.greet(); // Type is known at compile time
-
Compilation: C++ code is compiled into machine language, which generally leads to better performance when executing the program compared to interpreted languages.
-
Object-Oriented Programming: C++ brings in classes, inheritance, polymorphism, and encapsulation, allowing for the design of extensible software systems.
Example of class inheritance:
class Base { public: void show() { std::cout << "Base Class"; } }; class Derived : public Base { void show() { std::cout << "Derived Class"; } };
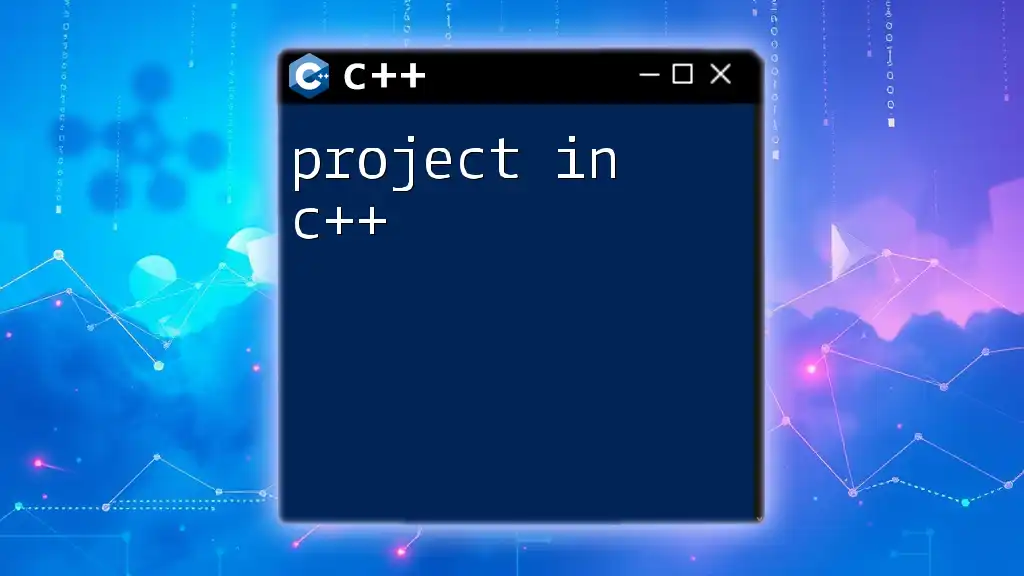
Objective-C vs C++
Syntax Comparison
Basic Syntax
The syntax of Objective-C and C++ differs notably in defining classes and methods.
In Objective-C, classes are defined with @interface and @implementation directives. For instance:
@interface MyClass : NSObject
@property (nonatomic, strong) NSString *name;
- (void)greet;
@end
@implementation MyClass
- (void)greet {
NSLog(@"Hello, %@", self.name);
}
@end
In contrast, C++ uses a more traditional C-like approach:
class MyClass {
public:
std::string name;
void greet() {
std::cout << "Hello, " << name << std::endl;
}
};
Memory Management
Objective-C employs Automatic Reference Counting (ARC) to handle memory management, making it simpler to manage object lifecycles.
Example:
self.name = @"Hello, World!"; // ARC automatically manages memory
C++ offers more manual control over memory management with pointers and smart pointers. Using smart pointers can help reduce memory leaks:
std::unique_ptr<MyClass> obj = std::make_unique<MyClass>();
Performance
C++ often outperforms Objective-C due to its compiled nature and optimized execution of code. For developers needing maximum efficiency, C++ provides fine control over system resources, which can lead to performance gains, particularly in system-level programming and gaming applications.
Multithreading and Concurrency
Objective-C provides multi-threading capabilities through Grand Central Dispatch (GCD), which facilitates managing concurrent operations without needing to thread explicitly.
Example using GCD:
dispatch_async(dispatch_get_global_queue(DISPATCH_QUEUE_PRIORITY_HIGH, 0), ^{
// Perform time-consuming task here
});
C++ offers threading support via the `<thread>` library, allowing fine control of threads, mutexes, and locks.
Example:
#include <thread>
void performTask() {
// Perform task in another thread
}
std::thread t(performTask);
t.join();
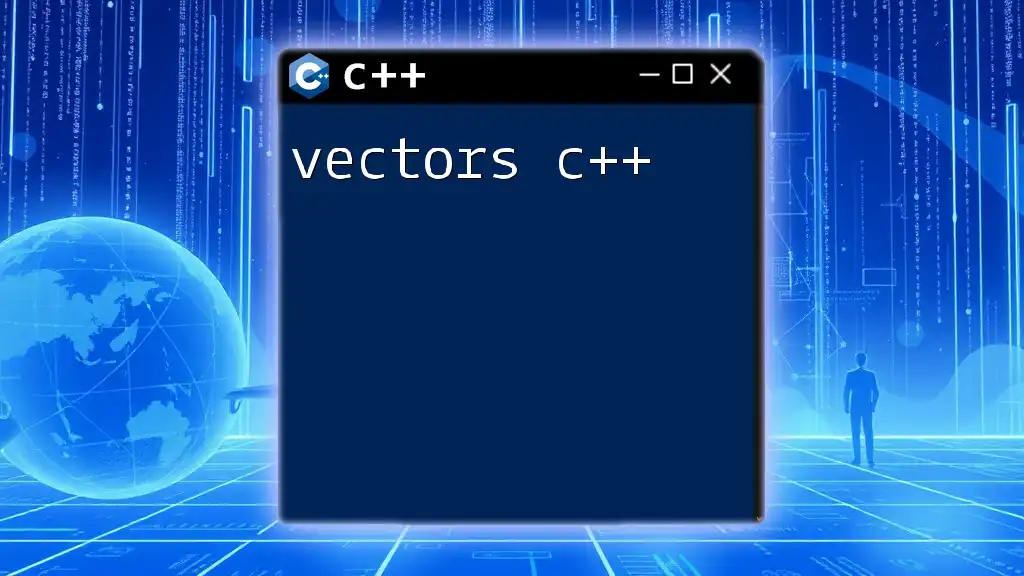
Use Cases for Objective-C and C++
When to Use Objective-C
Objective-C is particularly well-suited for iOS and macOS app development, where its integration with Cocoa and Cocoa Touch frameworks provides robust tools for creating user interfaces and application logic.
When to Use C++
C++ shines in applications where performance is critical. Fields such as game development, real-time simulation, and system programming leverage C++'s efficiency, access to low-level system resources, and fine-grained control over memory management.
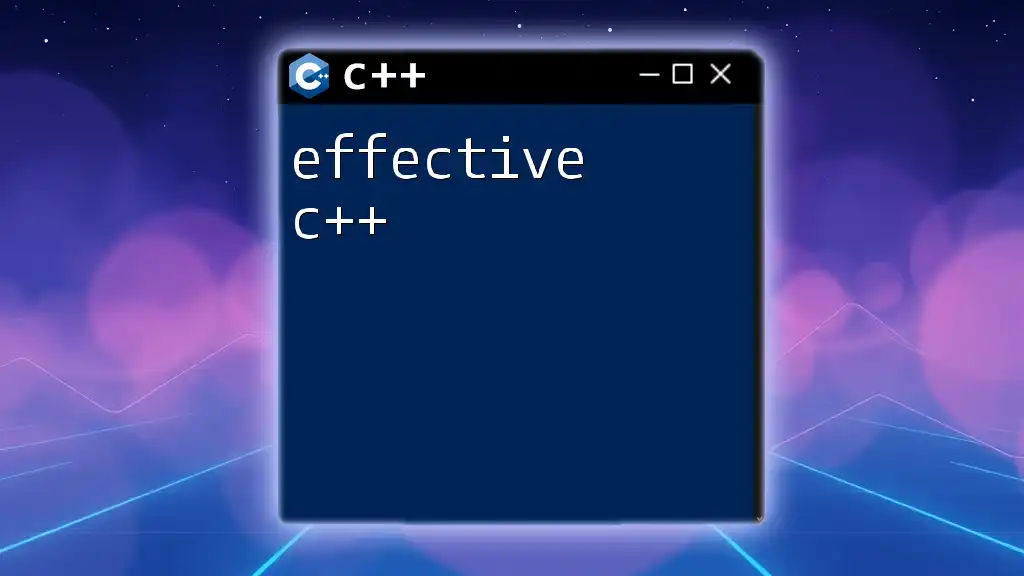
Community and Ecosystem
Tools and Libraries
For developing with Objective-C, Xcode is the primary Integrated Development Environment (IDE), equipped with tools tailored for Apple development, including Interface Builder for UI design. C++ developers often use IDEs like Visual Studio, which offers powerful debugging and coding assistance.
Community Support
Both Objective-C and C++ boast strong community support and extensive resources. Websites like Stack Overflow, GitHub, and dedicated forums enrich the user experience, enabling developers to solve issues and share knowledge easily.
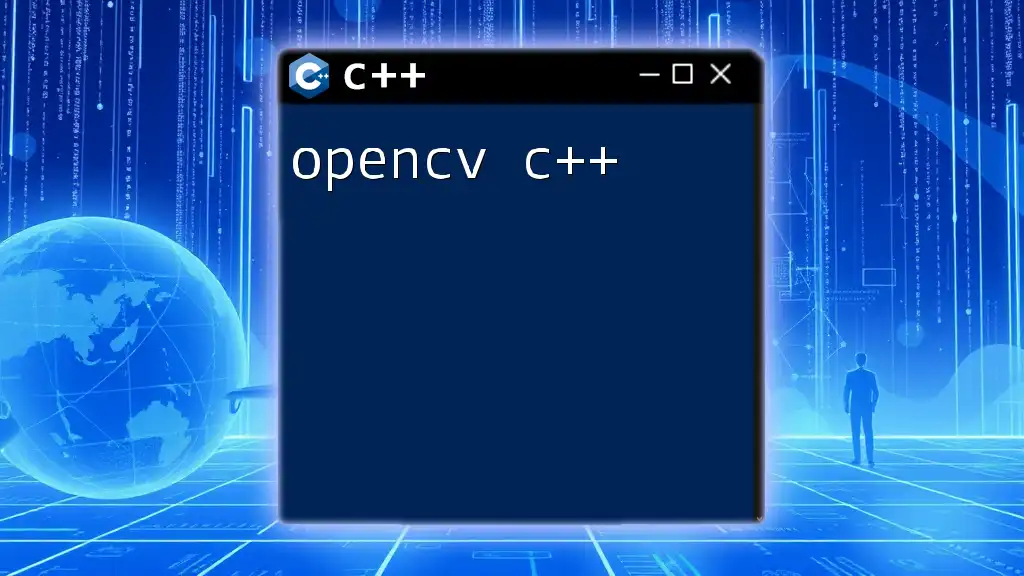
Conclusion
Understanding the differences between Objective-C and C++ is essential for choosing the right tool for your project. Both languages come with unique features and advantages, making them suitable for different tasks. Experimenting with both languages will provide a comprehensive skillset that enhances your programming capabilities, leading to more successful projects in your development journey.
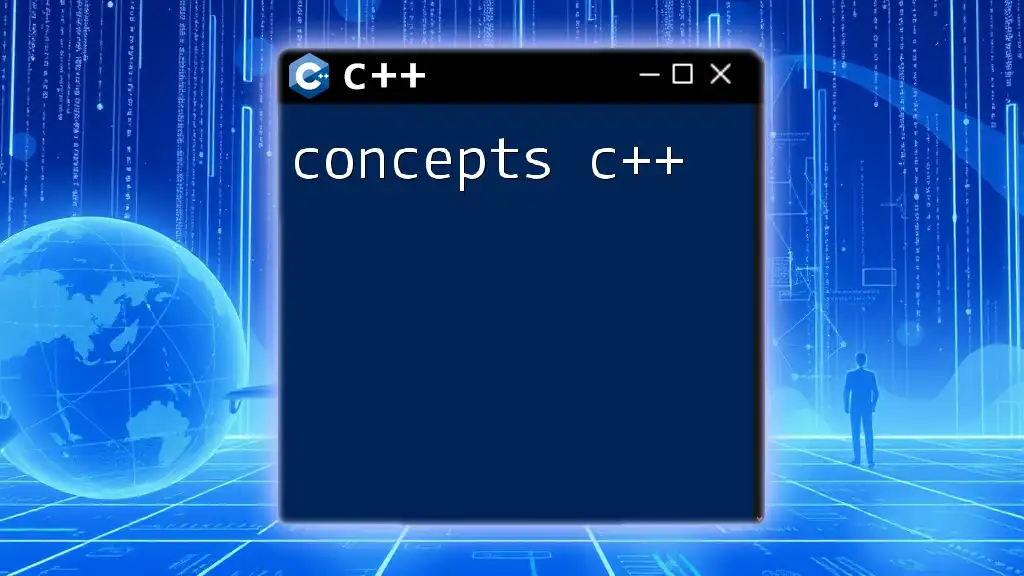
Resources
To deepen your know-how, consider exploring various books, online courses, and tutorials specifically designed for Objective-C and C++. Engaging with example projects and open-source repositories on platforms like GitHub can also solidify your understanding and lead to practical experience in coding with these two languages.