In C++, an array of objects allows you to create multiple instances of a class, enabling you to manage collections of related data more efficiently.
Here’s an example:
#include <iostream>
using namespace std;
class Student {
public:
string name;
int age;
};
int main() {
Student students[3]; // Array of 3 Student objects
// Assigning values to each object
students[0].name = "Alice";
students[0].age = 20;
students[1].name = "Bob";
students[1].age = 21;
students[2].name = "Charlie";
students[2].age = 22;
// Displaying the student information
for (int i = 0; i < 3; i++) {
cout << "Name: " << students[i].name << ", Age: " << students[i].age << endl;
}
return 0;
}
Understanding C++ Arrays
What is an Array in C++?
An array in C++ is a collection of elements of the same type. Arrays are indexed and are stored in contiguous memory locations. This allows for efficient access and manipulation of data. Here's a simple example of a one-dimensional array:
int numbers[5] = {1, 2, 3, 4, 5};
In this case, `numbers` is an array of integers with five elements. You can access an individual element using its index, such as `numbers[0]` for the first element.
Types of Arrays in C++
- 1-D Arrays: These are the most common types of arrays, which consist of a single row of elements. They are generally used for storing lists of items.
- Multi-Dimensional Arrays: These arrays have more than one row and column, allowing for the storage of data in matrix-like formats. For example:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
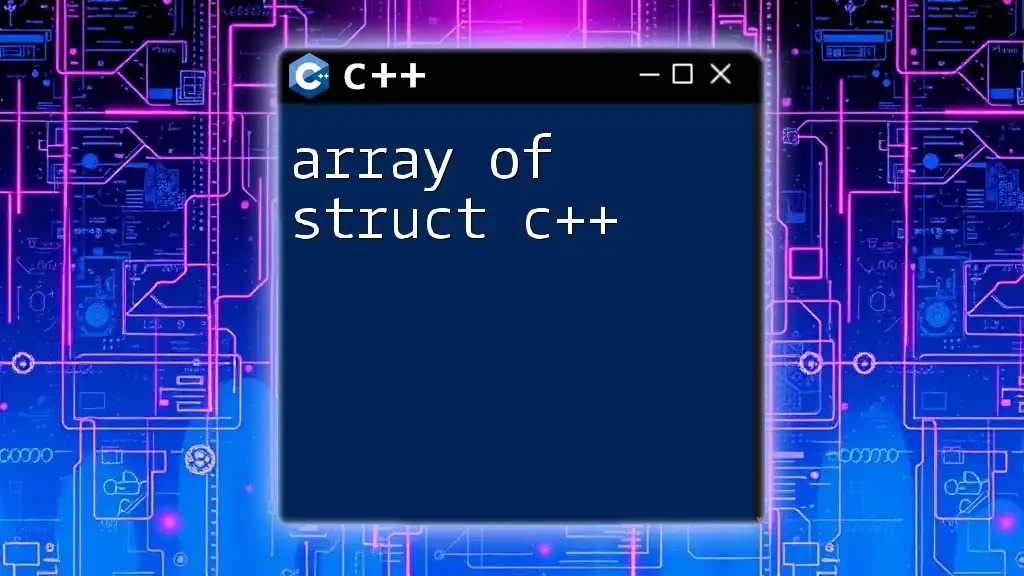
Creating an Array of Objects in C++
Defining a Class
Before creating an array of objects, you need to define a class. A class in C++ serves as a blueprint for creating objects. It can contain properties (attributes) and methods (functions). Here’s an example of a simple class:
class Student {
public:
std::string name;
int age;
// Constructor
Student(std::string studentName, int studentAge) {
name = studentName;
age = studentAge;
}
void displayInfo() {
std::cout << "Name: " << name << ", Age: " << age << std::endl;
}
};
Declaring an Array of Objects
Once the class is defined, you can declare an array of objects from that class. The syntax is straightforward:
Student students[3]; // Declares an array of 3 Student objects
This creates an array named `students` that can hold three `Student` objects.
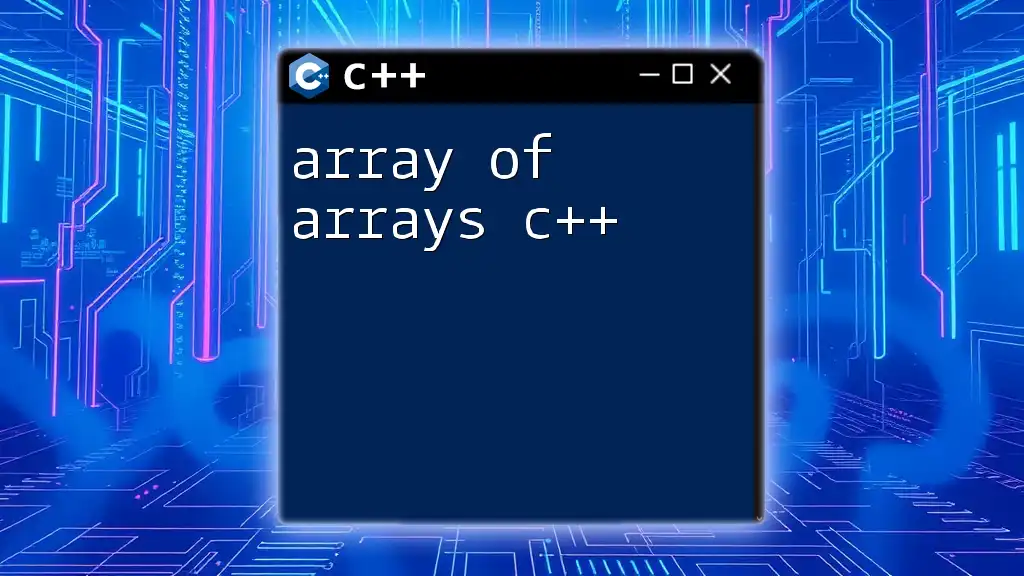
Accessing Array of Objects
Using Indexing to Access Objects
You can access each object in the array using indexing. For example, to access the first object:
students[0].name = "Alice";
students[0].age = 20;
Accessing Object Properties and Methods
To access properties and methods of the objects in the array, you simply use the dot operator. Continuing from the previous example:
students[0].displayInfo(); // Displays: Name: Alice, Age: 20
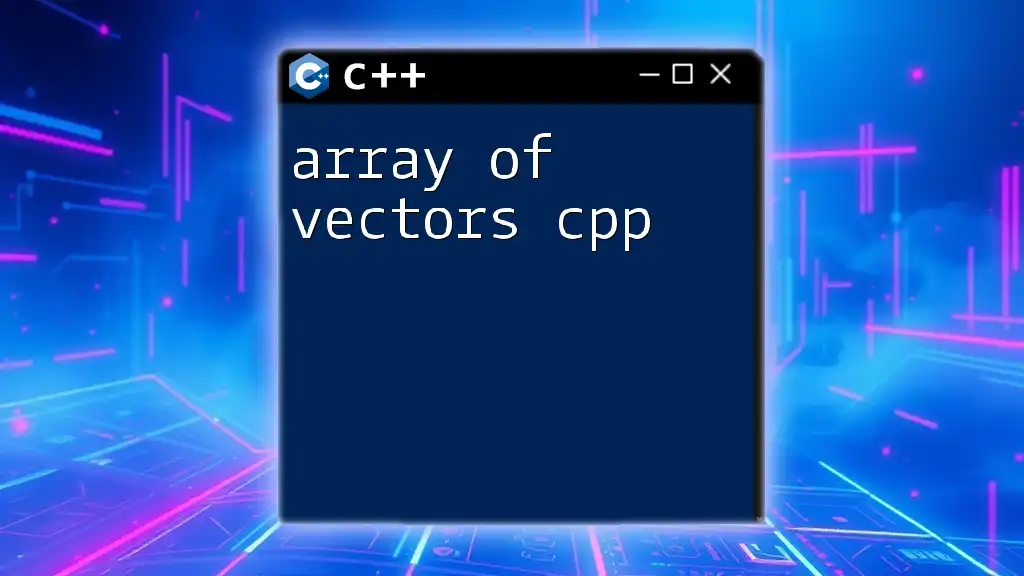
Initializing an Array of Objects
Default Initialization
When an array of objects is declared, the default constructor for the class is invoked. If your class does not have a constructor, the objects will be default-initialized. Here’s how that might look with the `Student` class defined earlier:
Student students[3]; // Default constructor will initialize students to default values
Parameterized Initialization
To initialize objects in an array using parameterized constructors, you can do it at the time of declaration:
Student students[3] = {Student("Alice", 20), Student("Bob", 21), Student("Charlie", 19)};
This will create three objects with the names and ages provided.
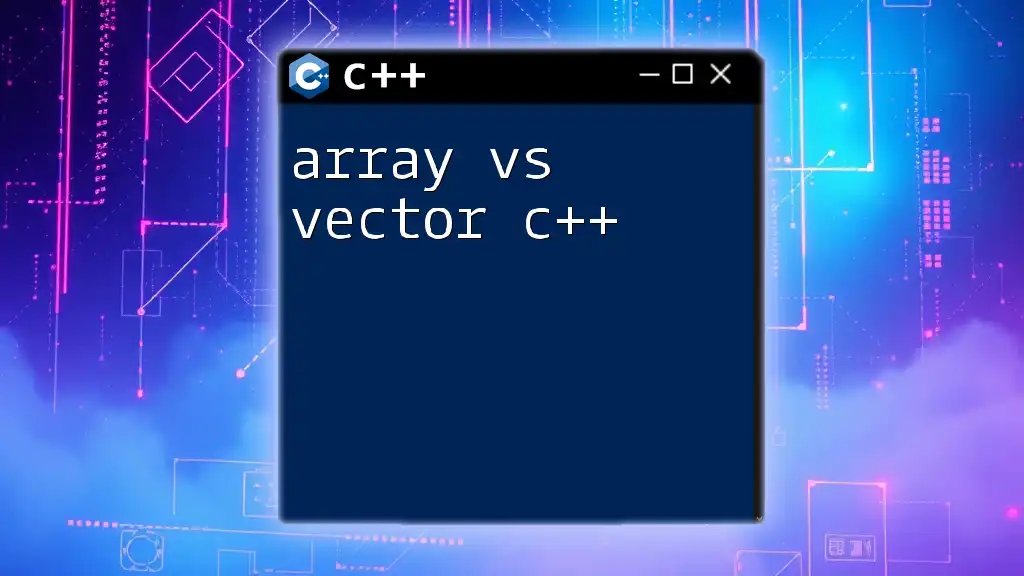
Array of Objects in C++: Use Cases
Managing Collections of Data
Arrays of objects are incredibly useful for managing collections of similar data. For instance, if you're developing an application to manage student records, an array of `Student` objects could help you store each student's information efficiently.
Iterating Through an Array of Objects
When you have an array of objects, it’s essential to know how to iterate through it. You can use a traditional `for` loop:
for (int i = 0; i < 3; i++) {
students[i].displayInfo();
}
Alternatively, you can use a range-based `for` loop introduced in C++11:
for (const auto& student : students) {
student.displayInfo();
}
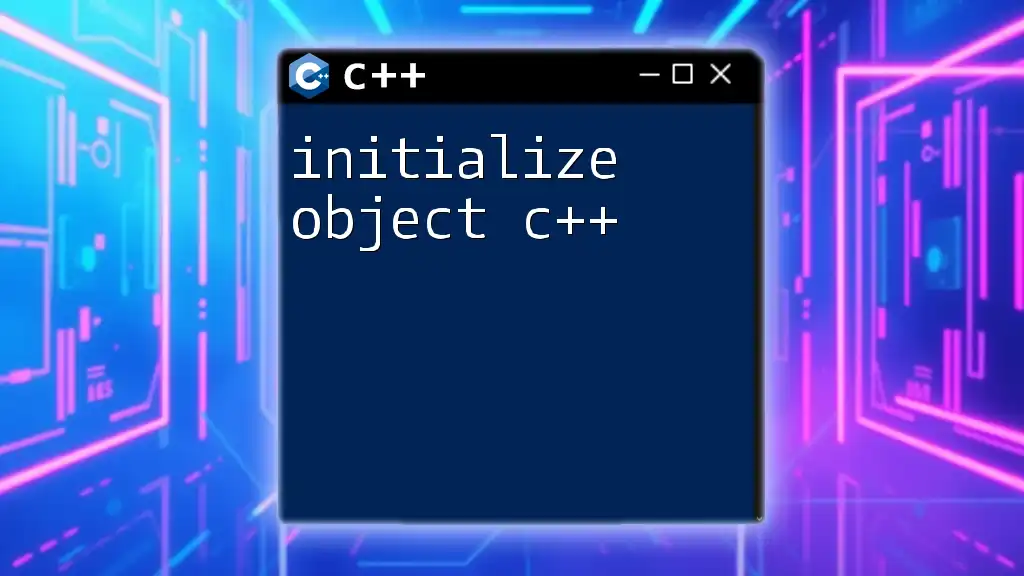
Best Practices
Memory Management Considerations
When working with an array of objects, consider the differences between static and dynamic arrays. Static arrays have a fixed size, which may lead to wasted memory or overflow errors. Dynamic arrays can grow or shrink as needed by using pointers and `new`:
Student* students = new Student[size];
// Don't forget to free the memory afterward
delete[] students;
Using Standard Template Library (STL)
The Standard Template Library (STL) offers powerful data structures, like `std::vector`, which can be used as dynamic arrays. A vector can automatically resize itself, making it a better choice in many situations:
#include <vector>
std::vector<Student> students;
students.push_back(Student("Alice", 20));
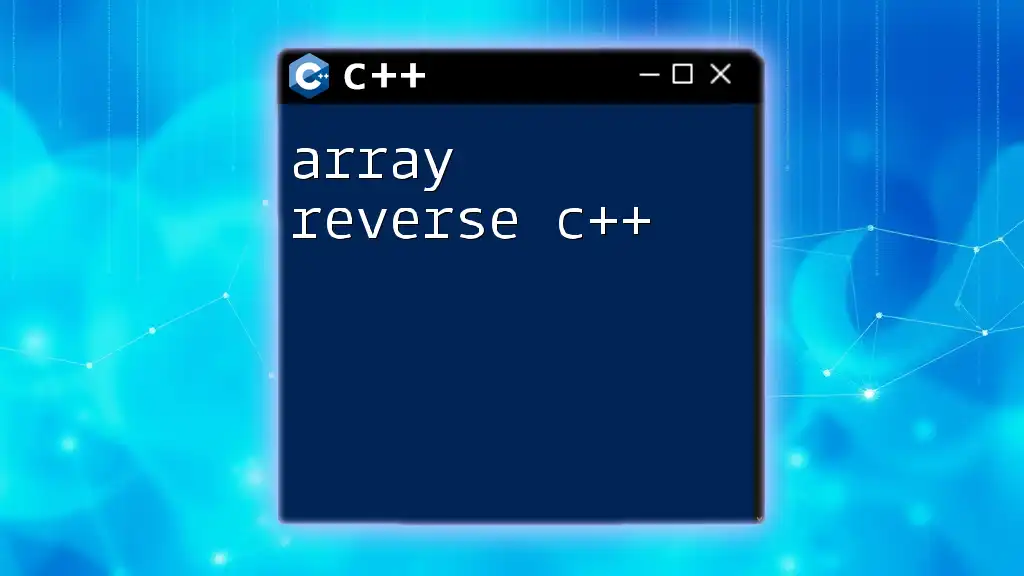
Common Mistakes and How to Avoid Them
Off-by-One Errors
One common pitfall is the off-by-one error, which happens when you forget that array indexing starts at zero. Always remember that the last index of an array with `n` elements is `n-1`.
Improper Object Construction
Improper initialization of objects can lead to undefined behavior. Always ensure that constructors are called correctly when creating objects in an array, and remain aware of the type of constructors your class possesses.
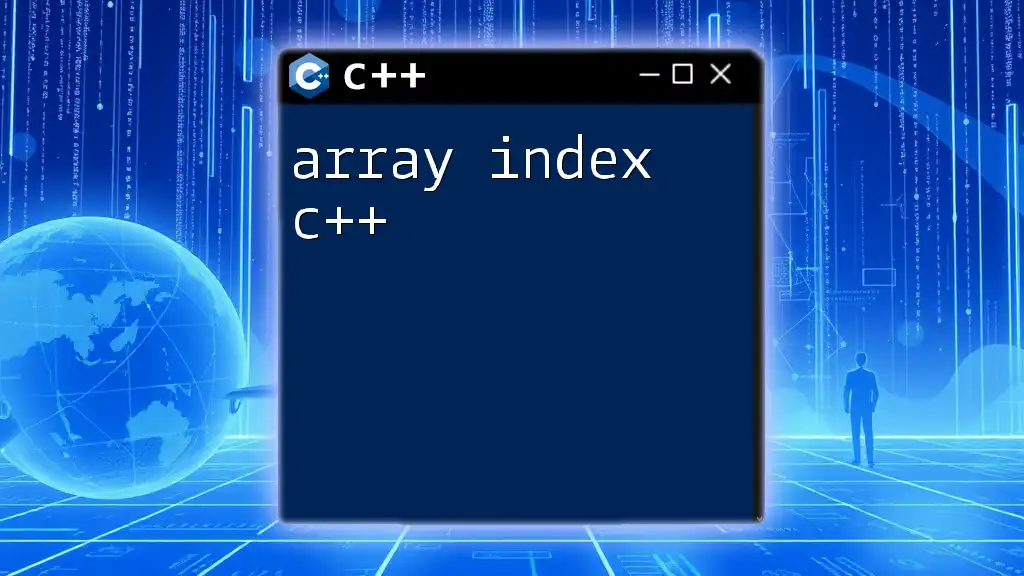
Conclusion
The array of objects in C++ is a powerful construct that allows you to manage and manipulate collections of similar data efficiently. By understanding how to create and use arrays of objects, you can leverage the power of object-oriented programming to write cleaner and more efficient code. Don’t forget to practice these concepts in your own projects to solidify your understanding!
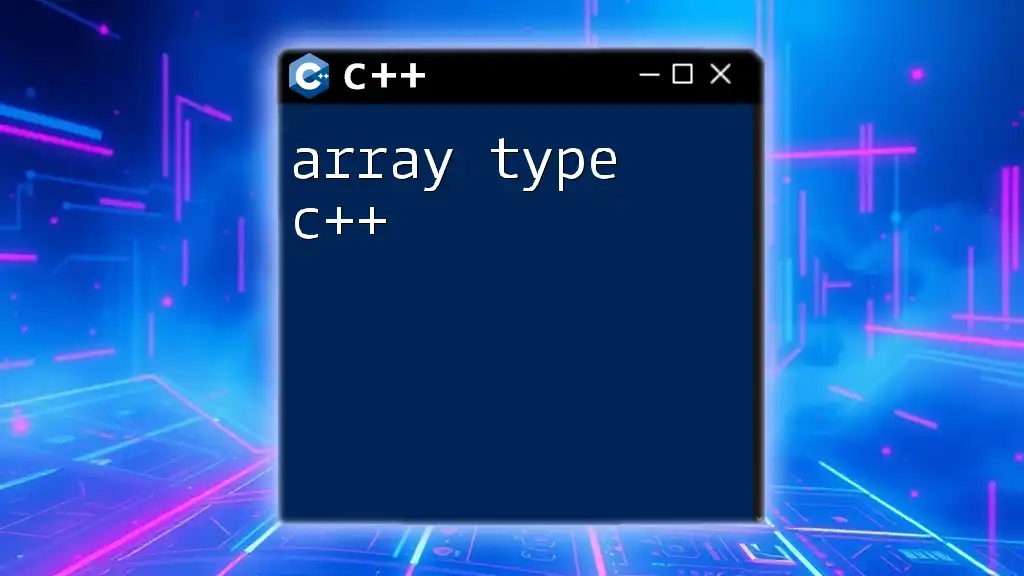
Additional Resources
To further your knowledge on arrays and object-oriented programming in C++, consider exploring textbooks, online courses, and platforms that specialize in programming tutorials. Taking the time to learn these additional details will help enhance your programming skills in C++.