`valarray` in C++ is a class designed for handling arrays of values with built-in support for element-wise operations, allowing for more efficient numerical computations.
Here’s a simple example of using `valarray`:
#include <iostream>
#include <valarray>
int main() {
std::valarray<int> arr = {1, 2, 3, 4, 5};
std::valarray<int> result = arr * 2; // Element-wise multiplication by 2
std::cout << "Result: ";
for (auto v : result) std::cout << v << " "; // Output: 2 4 6 8 10
return 0;
}
Understanding `valarray`
In C++, a `valarray` is a specialized container defined in the `<valarray>` header. It is primarily designed for applications involving numerical computations. While it resembles a traditional array or a `std::vector`, a `valarray` is optimized for element-wise operations and offers significant performance benefits, particularly for large datasets.
Key Features of `valarray` include automatic memory management, the ability to perform mathematical operations directly, and optimized performance for bulk data processing. These characteristics make `valarray` a powerful tool for mathematical computations in scientific and engineering applications.
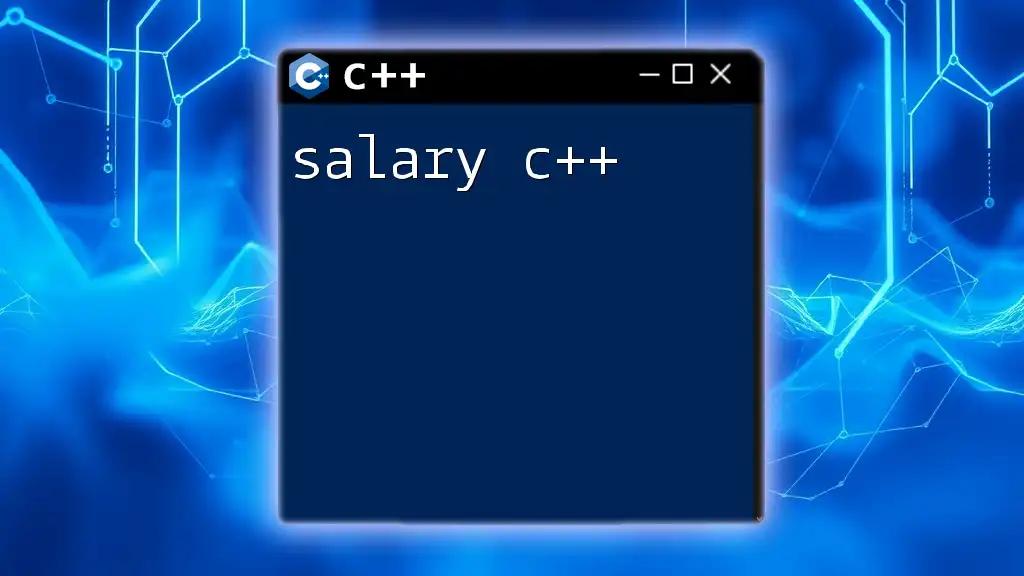
Getting Started with `valarray`
Including the Necessary Header
To use `valarray`, you must include the appropriate header:
#include <valarray>
Creating and Initializing `valarray`
Declaring a `valarray` is straightforward. You can create an empty `valarray`, initialize it with a specific size, or even define it with preset values. For example:
std::valarray<int> arr1(5); // Initializes a valarray of size 5 with default values
std::valarray<double> arr2 = {1.0, 2.0, 3.0}; // Initializes with specific values
The first example creates a `valarray` of integers containing five elements, all initialized to zero. The second example initializes a `valarray` of doubles with values 1.0, 2.0, and 3.0.
Resizing a `valarray`
You can dynamically resize a `valarray` using the `resize` method. This flexibility allows your `valarray` to grow or shrink as needed:
arr1.resize(10); // Changes the size of arr1 to 10
When resizing, be aware that increasing the size may introduce new elements initialized to zero, while reducing the size truncates the data.
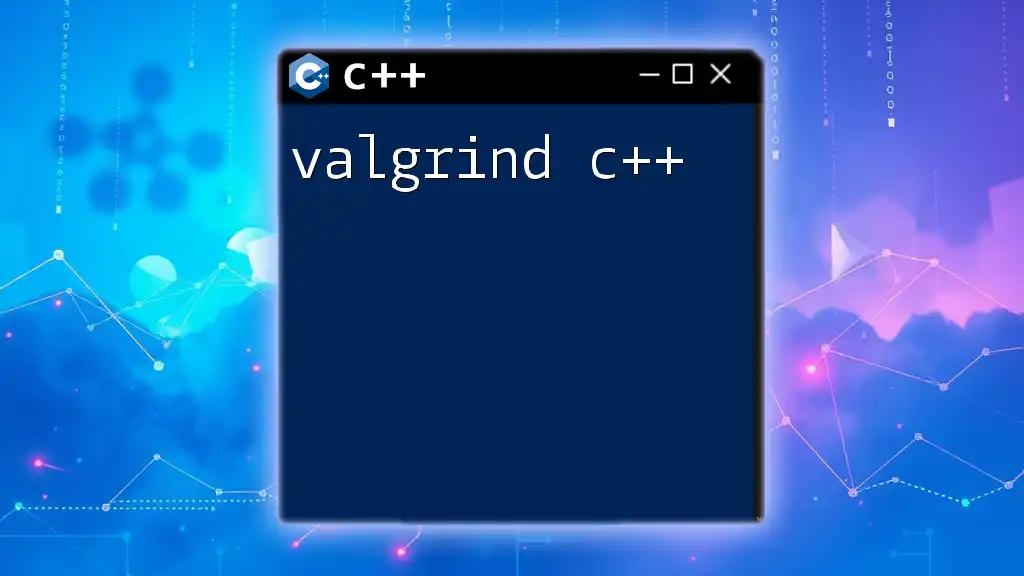
Common Operations with `valarray`
Mathematical Operations
`valarray` supports a wide range of mathematical operations that can be performed directly on the entire array. For example:
std::valarray<int> arr1 = {1, 2, 3};
std::valarray<int> arr2 = arr1 + 2; // Each element adds 2
In this case, every element of `arr1` is incremented by 2, producing a new `valarray` containing {3, 4, 5}.
Element-wise Operations
The `apply` function allows for more complex operations on each element. This member function takes a function and applies it to every item in the `valarray`. Here is an example:
std::valarray<double> values = {1.0, 2.0, 3.5};
auto squared = values.apply([](double x) { return x * x; }); // Squares every element
The result, `squared`, will contain {1.0, 4.0, 12.25}, as each value in `values` is squared.
Slicing and Indexing
Accessing elements in a `valarray` can be done using standard indexing. For example:
int x = arr1[2]; // Accessing the 3rd element of arr1
Slicing is another powerful feature that enables you to extract subarrays from a `valarray`. Here’s how it works:
std::valarray<int> sliced = arr1[std::slice(1, 3, 1)]; // Extracts elements starting from index 1 to 3
In this example, `sliced` will contain elements from index 1 to index 3, effectively allowing you to create subarrays easily.
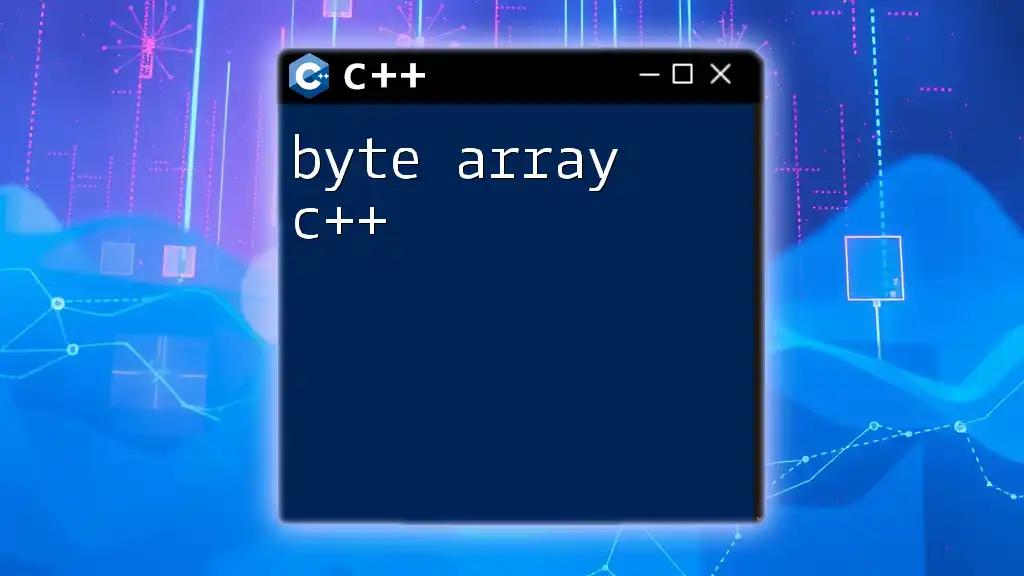
Advanced Concepts
Combining `valarray` with STL Algorithms
`valarray` can be seamlessly integrated with the Standard Template Library (STL) algorithms. For instance, you can apply sorting directly to a `valarray`:
std::sort(std::begin(arr2), std::end(arr2)); // Sorts arr2
`valarray` and Generic Programming
You can design template functions that work flexibly with `valarray`. Below is a simple example of a function that prints the contents of a `valarray`:
template<typename T>
void printValarray(const std::valarray<T>& arr) {
for (const auto& element : arr) {
std::cout << element << ' ';
}
std::cout << std::endl;
}
This function iterates through each element of the given `valarray` and outputs its content.
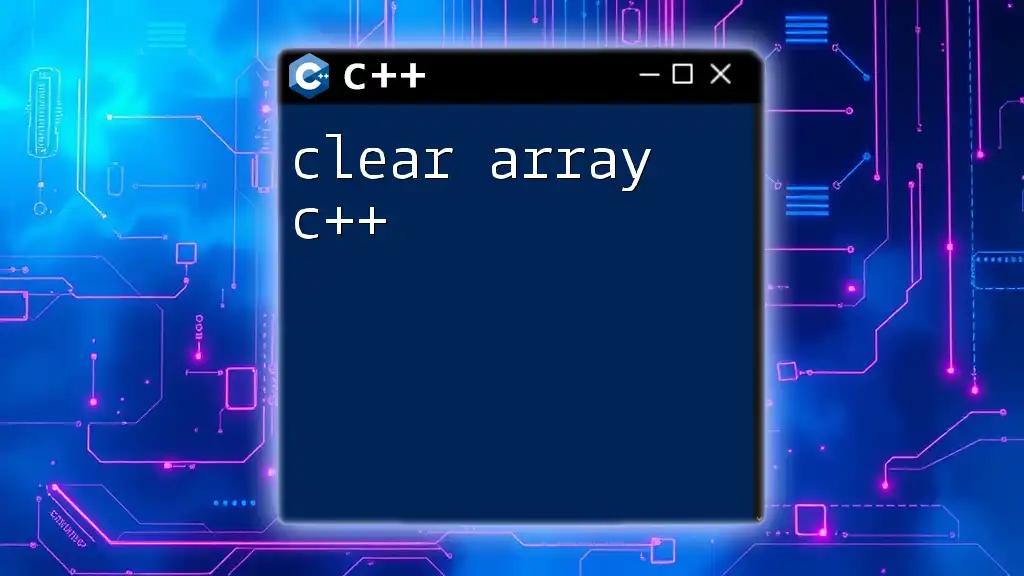
Performance Considerations
When to Use `valarray`
Utilizing `valarray` proves highly advantageous in numerical computations, where efficiency and performance are paramount. It has been specifically designed to handle bulk data operations effectively, making it ideal for applications involving large datasets.
Benchmarking `valarray`
To illustrate its performance benefits, consider doing a benchmark comparing `valarray` to its counterpart, `std::vector`. Conduct tests that measure execution time for various operations, such as addition across large datasets, to highlight the efficiency of `valarray`.
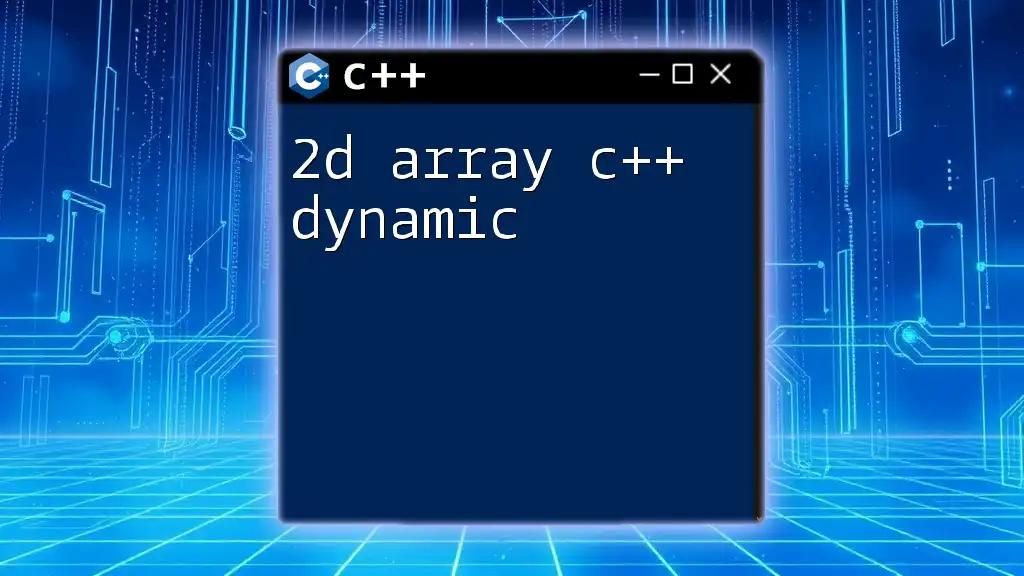
Best Practices for Using `valarray`
Memory Management
To ensure optimal performance, it's vital to manage memory effectively when working with `valarray`. Avoid frequent resizing; instead, preallocate memory if you can estimate the size required. This reduces overhead and improves performance by minimizing memory allocation costs.
Avoiding Common Pitfalls
While `valarray` is powerful, developers should steer clear of common mistakes, such as using non-integer types or failing to apply element-wise operations properly. Debugging should also consider checking the boundaries of slices to avoid accessing invalid indices.
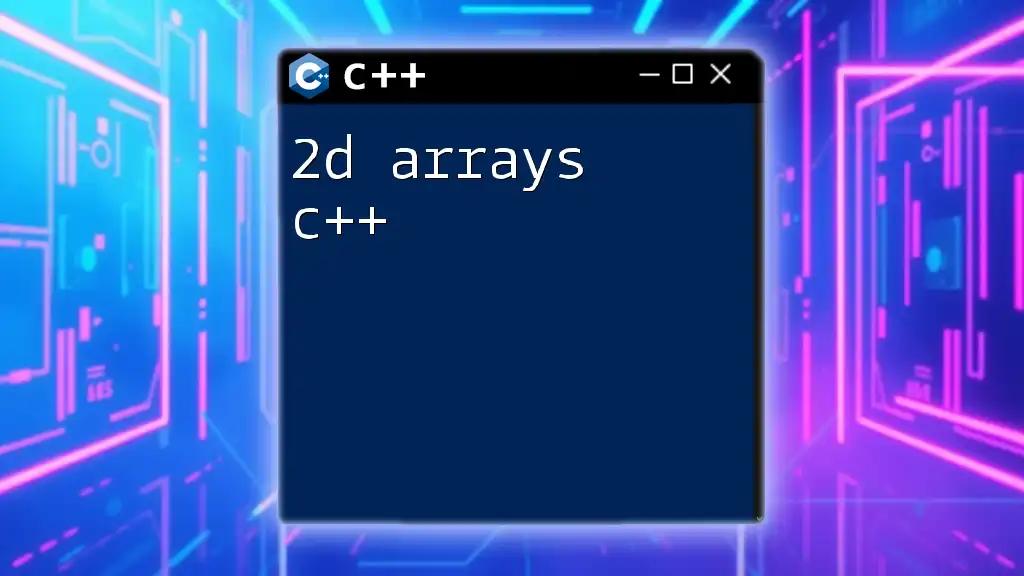
Conclusion
In summary, `valarray` in C++ is an essential tool for efficient numerical computations. It offers distinct advantages in terms of performance and ease of use, making it an excellent choice for developers working with mathematical applications. With a solid understanding and proper implementation, `valarray` can significantly enhance your programming toolkit in C++. For those eager to dive deeper into this topic, there are numerous resources available that provide further insight and advanced training on valarrays and numerical computing in C++.