A 2D dynamic array in C++ allows you to create a matrix where both the number of rows and columns can be defined at runtime using pointers.
Here’s an example of how to create, initialize, and free a 2D dynamic array in C++:
#include <iostream>
int main() {
int rows = 3, cols = 4;
int** array = new int*[rows]; // Allocate memory for rows
for (int i = 0; i < rows; ++i) {
array[i] = new int[cols]; // Allocate memory for columns
}
// Initializing the array
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
array[i][j] = i * cols + j; // Example value
}
}
// Displaying the array
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
std::cout << array[i][j] << " ";
}
std::cout << std::endl;
}
// Freeing the allocated memory
for (int i = 0; i < rows; ++i) {
delete[] array[i]; // Delete columns
}
delete[] array; // Delete rows
return 0;
}
Understanding Dynamic 2D Arrays
A 2D array is essentially an array of arrays, allowing you to create a table-like structure where you can store data in rows and columns. When you declare a static 2D array in C++, the size of the array has to be fixed at compile time. However, with dynamic 2D arrays, you can allocate memory at runtime, offering flexibility to accommodate variable sizes based on the needs of your application.
Importance of Dynamic Memory Allocation
Dynamic memory allocation is crucial in scenarios where the exact size of the data isn't known beforehand or varies during execution. Using dynamic memory has several advantages:
- Flexibility: You can work with varying sizes, with memory being allocated only when needed.
- Efficient Memory Usage: You can free memory that is no longer required, minimizing waste.
- Avoiding Stack Overflow: Large data sets can exceed stack limits, but dynamic memory is allocated on the heap, which has a significantly larger limit.
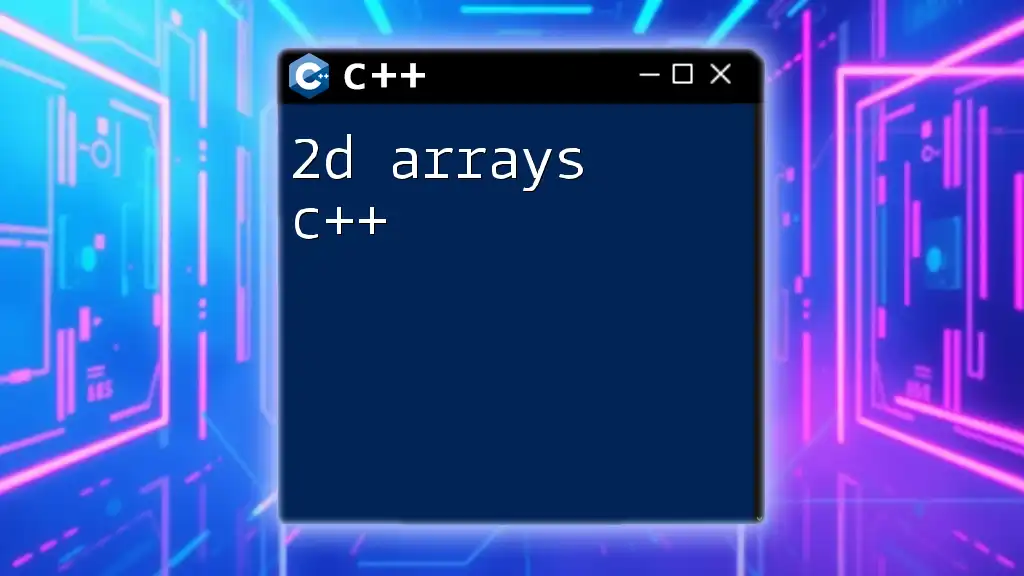
Understanding Dynamic Memory Allocation in C++
In C++, dynamic memory management is primarily handled through the `new` and `delete` operators.
- The `new` operator allocates memory on the heap and returns a pointer to that memory.
- The `delete` operator frees memory previously allocated with `new`, preventing memory leaks.
When working with arrays, there are key concepts you should understand:
- Pointers: A pointer is a variable that stores the address of another variable, including arrays.
- References: A reference is an alias for another variable, typically used for easier access.
- Memory Allocation: Dynamically allocated memory can be resized and released, providing more control over resource management.
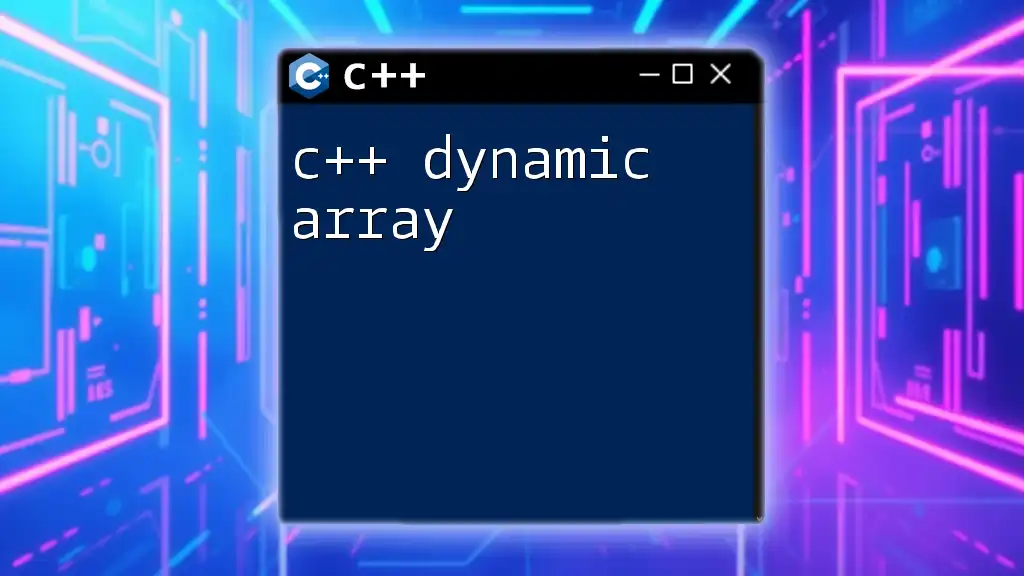
Creating a Dynamic 2D Array in C++
To create a dynamic 2D array in C++, you typically use pointers. Below is the basic syntax for creating a dynamic 2D array:
int** array = new int*[rows];
for (int i = 0; i < rows; i++) {
array[i] = new int[cols];
}
In this snippet, we declare a pointer to a pointer (`int** array`). The first allocation takes place for the array of row pointers (each pointing to a column of integers). In the second step, we allocate memory for each row to hold the column values.
Example of Dynamic 2D Array Initialization
Let’s initialize a dynamic 2D array with 3 rows and 4 columns:
int rows = 3, cols = 4;
int** array = new int*[rows];
for (int i = 0; i < rows; i++) {
array[i] = new int[cols];
}
In this example, we allocate memory for 3 pointers that represent the rows, and for each row, we allocate space for 4 integers. Initially, the values inside the array are undetermined until explicitly set.
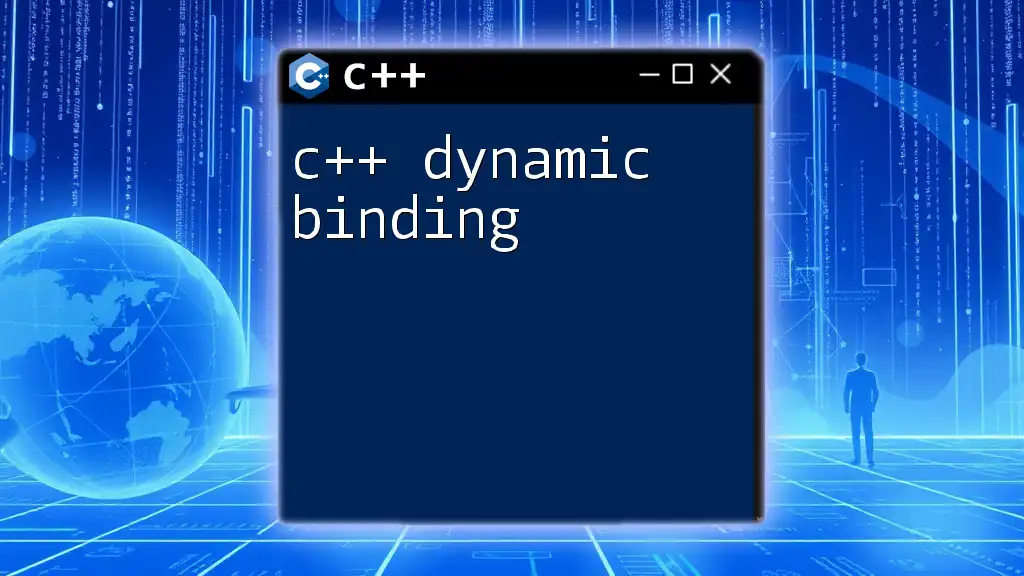
Accessing and Modifying Elements in a Dynamic 2D Array
Accessing elements in a dynamic 2D array is straightforward. You can do this by referencing the row and column indices directly:
array[i][j] = value; // Assign value to the element at row i, column j
Modifying Elements
To modify an element, you assign a new value to a specified position. For instance:
array[0][1] = 5; // Assigning value 5 to the element at row 0, column 1
This operation allows for efficient access and modification of elements, key features in many algorithms, from data processing to game development.
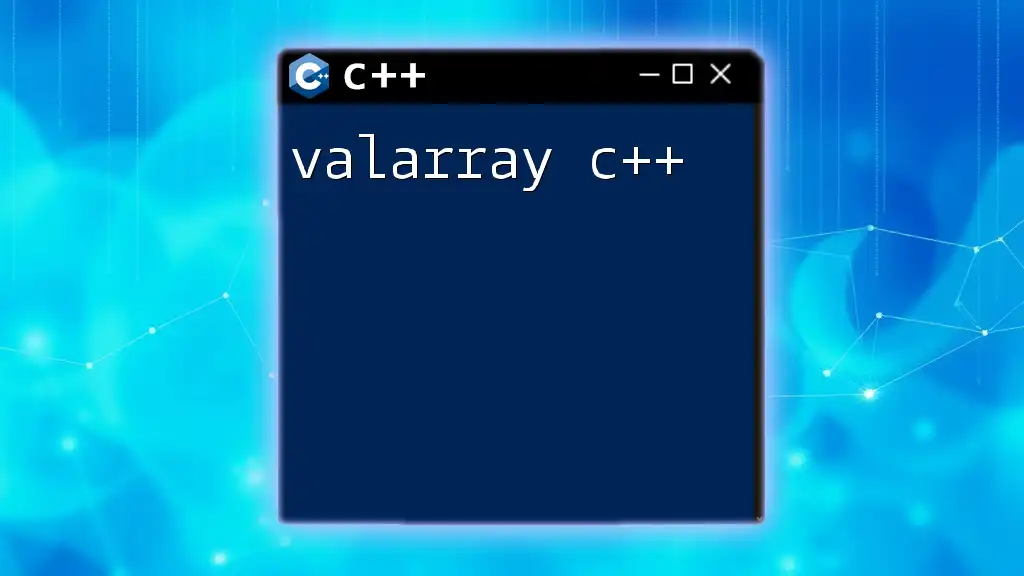
Deleting a Dynamic 2D Array
Memory management is crucial to prevent memory leaks and ensure efficient operation. To delete a dynamic 2D array, you must free memory in a specific order:
for (int i = 0; i < rows; i++) {
delete[] array[i]; // Freeing each row
}
delete[] array; // Freeing the array of pointers
Complete Example
Here is a complete example that covers both creation and deletion of a dynamic 2D array:
int rows = 3, cols = 4;
int** array = new int*[rows];
// Initializing the dynamic 2D array
for (int i = 0; i < rows; i++) {
array[i] = new int[cols];
}
// Example of assigning values
array[0][0] = 1;
array[0][1] = 2;
// Deleting the dynamic 2D array
for (int i = 0; i < rows; i++) {
delete[] array[i];
}
delete[] array;
In this example, we create a 3x4 dynamic array, assign values, and properly delete the memory at the end to prevent any leaks.
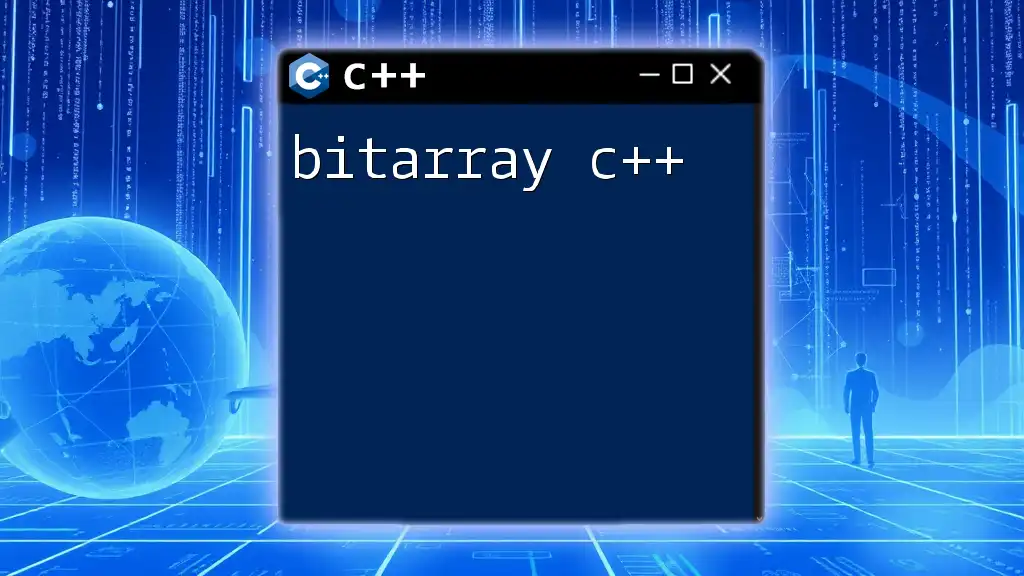
Resizing a Dynamic 2D Array
One of the challenges when working with dynamic arrays is resizing them. If the size needs to change during runtime, you'll need to create a new 2D array, copy existing data, and delete the old array.
Techniques for Resizing a Dynamic 2D Array
- Create a new array with the desired dimensions.
- Copy data from the old array to the new one.
- Delete the old array to free memory.
Here’s a code snippet demonstrating this procedure:
int newRows = 5, newCols = 6;
int** newArray = new int*[newRows];
for (int i = 0; i < newRows; i++) {
newArray[i] = new int[newCols];
}
// Copying existing data (Example only, adjust according to your own logic)
for(int i = 0; i < rows; i++) {
for(int j = 0; j < cols; j++) {
if (i < newRows && j < newCols) {
newArray[i][j] = array[i][j]; // Copying data
}
}
}
// Clean up old array
for (int i = 0; i < rows; i++) {
delete[] array[i];
}
delete[] array;
// Now use newArray for further operations
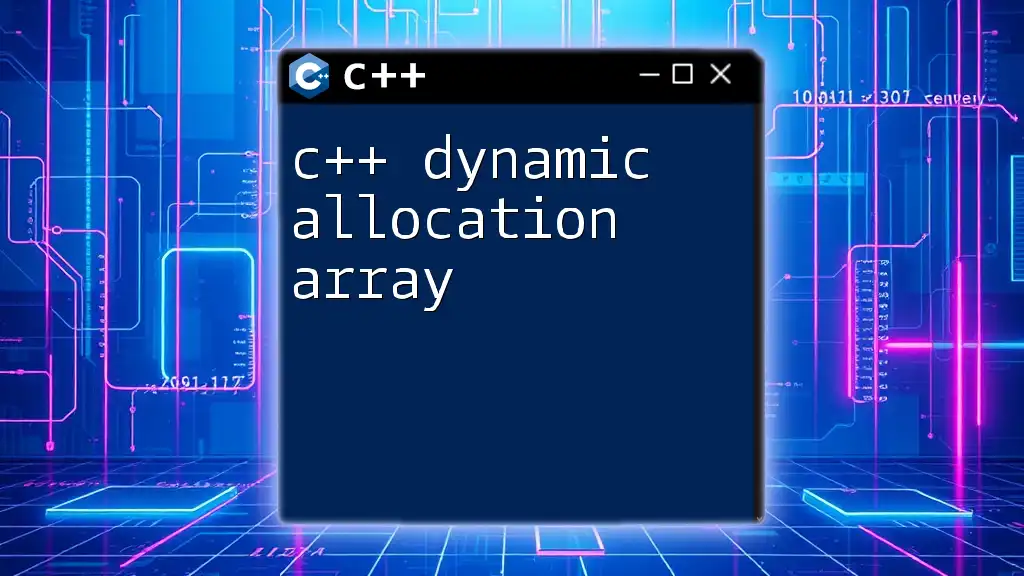
Common Mistakes When Using Dynamic 2D Arrays
Even experienced programmers can make mistakes when handling dynamic memory. Here are some common issues to be aware of:
Memory Leaks
A memory leak occurs when you allocate memory without freeing it. This can lead to a lack of available memory over time, deteriorating performance.
Improper Deallocation
Failing to properly deallocate memory can lead to errors. Always follow the same order of deallocation as allocation to avoid undefined behavior.
Using Uninitialized Pointers
Accessing uninitialized memory leads to unpredictable results. Always initialize pointers to `nullptr` and check them before usage.
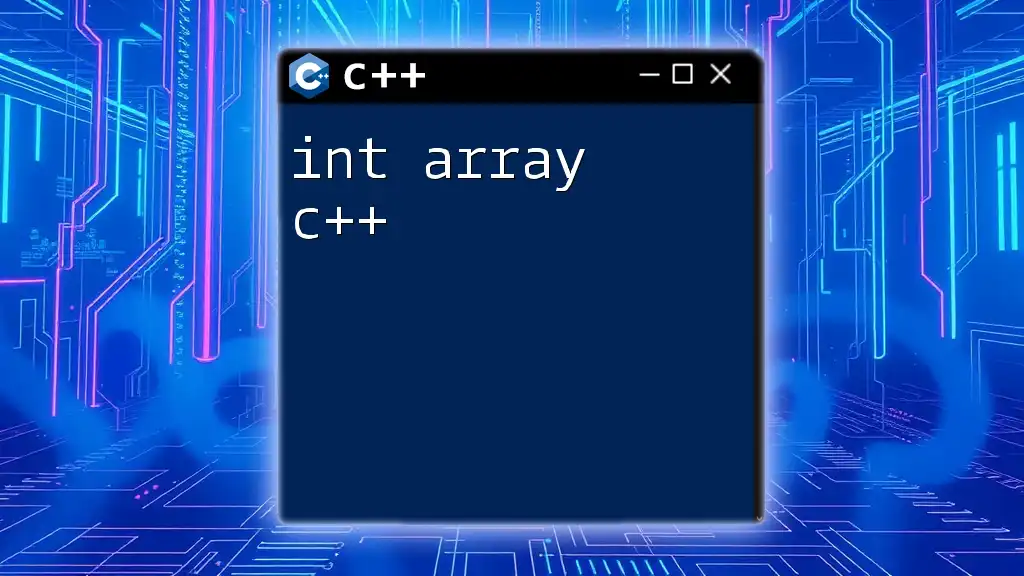
Real-World Applications of Dynamic 2D Arrays in C++
Dynamic 2D arrays find applications in various fields:
Use Cases in Game Development
In game development, you might use dynamic 2D arrays to manage game maps or grids. The ability to resize based on player actions or generated content makes dynamic arrays particularly useful.
Use Cases in Scientific Computing
Dynamic 2D arrays are valuable in scientific computing for tasks such as matrix operations, simulations, and handling large data sets where dimensions are not predetermined.
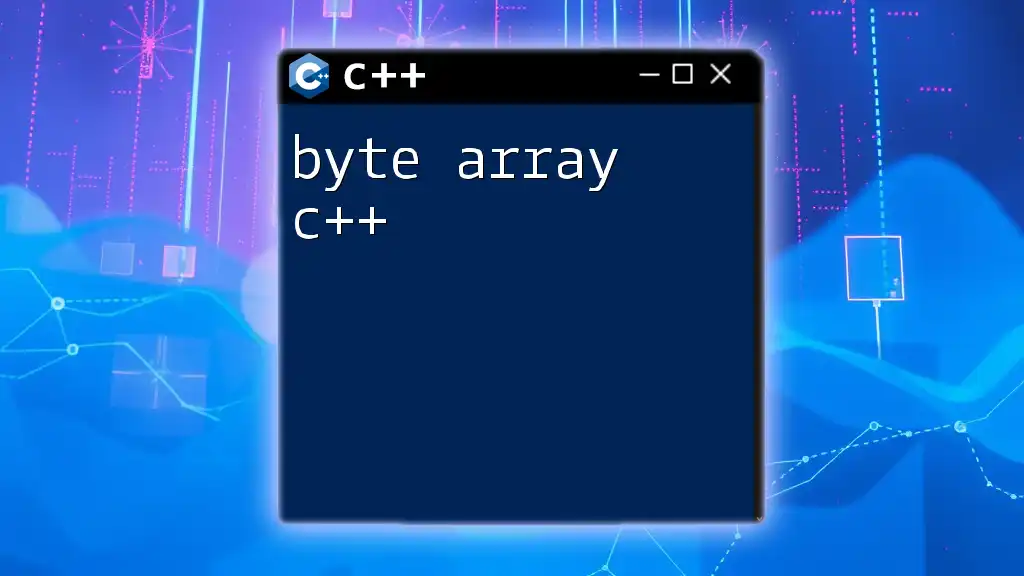
Conclusion
Dynamic 2D arrays are an essential part of C++ programming, providing flexibility and efficient memory management. By mastering their creation, manipulation, resizing, and proper cleanup, you can enhance the quality and performance of your applications. Practice implementing these concepts to become proficient in using dynamic 2D arrays in your projects.
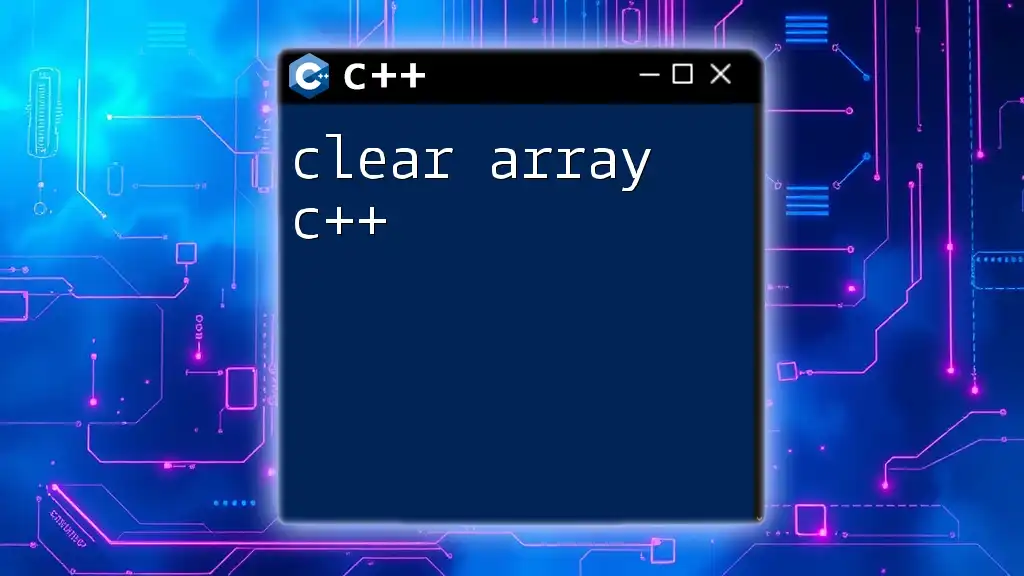
Additional Resources
To deepen your understanding, consider referencing books on C++ programming, online forums, and community resources where you can engage with experts and peers for further learning.