In C++, an array of char arrays (often referred to as a 2D character array) is used to store multiple strings, where each row represents a separate character array.
Here’s a code snippet that demonstrates how to declare and initialize an array of char arrays:
#include <iostream>
int main() {
const char* cities[3] = {"New York", "Los Angeles", "Chicago"};
for (int i = 0; i < 3; i++) {
std::cout << cities[i] << std::endl;
}
return 0;
}
Understanding Char Arrays
What is a Char Array?
A char array is a collection of elements that are of the type `char`. This collection is stored in contiguous memory locations, making it useful for storing strings or individual characters together. Understanding how to properly declare and manipulate char arrays is crucial in C++ programming.
Here’s a basic example of declaring a char array:
char hello[6] = "Hello";
This declaration creates a character array capable of holding five characters plus an additional null terminator (`\0`), which indicates the end of the string.
Use Cases for Char Arrays
Char arrays are primarily used in C++ for storing strings, but they can be applied in various situations that require manipulation of character data. They are particularly useful when interfacing with low-level APIs or performing string operations that need direct memory handling.

Introduction to C++ Array of Char Arrays
What is an Array of Char Arrays?
An array of char arrays, often referred to as a 2D array of characters, is a data structure that can hold multiple strings. This is particularly useful for managing collections of strings efficiently. The distinction between a single char array and an array of char arrays is crucial; the former can hold only a singular string, while the latter can manage many strings.
Here’s how you can declare an array of char arrays:
char names[3][20]; // Array of 3 strings, each capable of holding up to 19 characters + null terminator
Visualizing the Structure
When you declare an array of char arrays, memory is allocated for each string, allowing for direct access via indexing. For instance, `names[0]` accesses the first string, `names[1]` the second, and so forth. Each string itself is treated as a separate char array within the larger array.
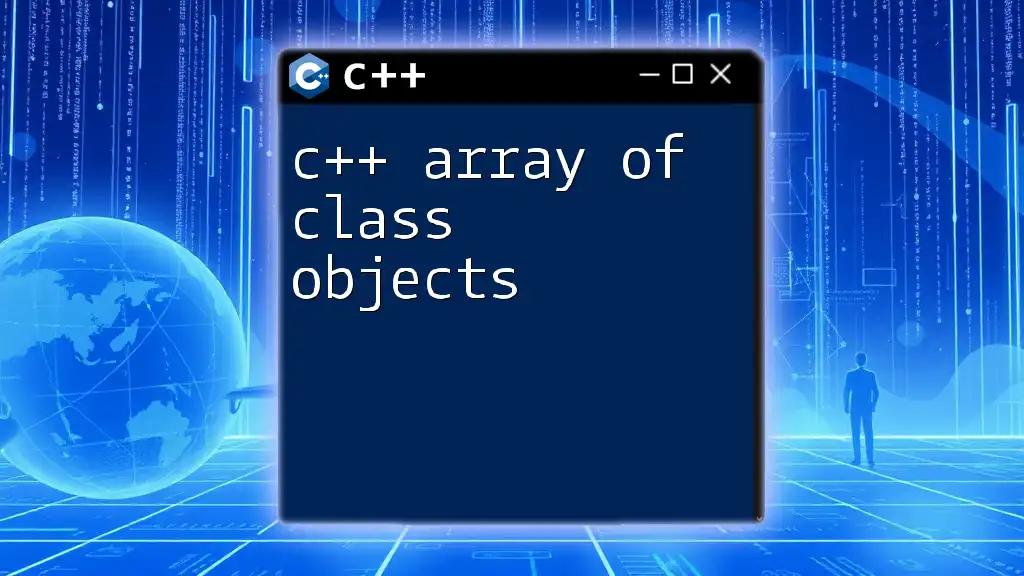
Declaring and Initializing an Array of Char Arrays
Syntax for Declaration
The syntax for declaring an array of char arrays can vary, but the core concept remains the same. Here are a few examples:
char fruits[3][10]; // Three char arrays, each can hold up to 9 characters
Initialization Techniques
When it comes to initializing an array of char arrays, two common methods are used.
Static Initialization
Static initialization occurs when you assign values at the point of declaration:
char colors[3][10] = {"Red", "Green", "Blue"};
Dynamic Initialization
Dynamic initialization requires separate calls to assign values post-declaration. For example:
char vehicles[2][15];
strcpy(vehicles[0], "Car");
strcpy(vehicles[1], "Bus");
Be cautious of common pitfalls, such as not allocating sufficient memory for each string, which can lead to undefined behavior or crashes.
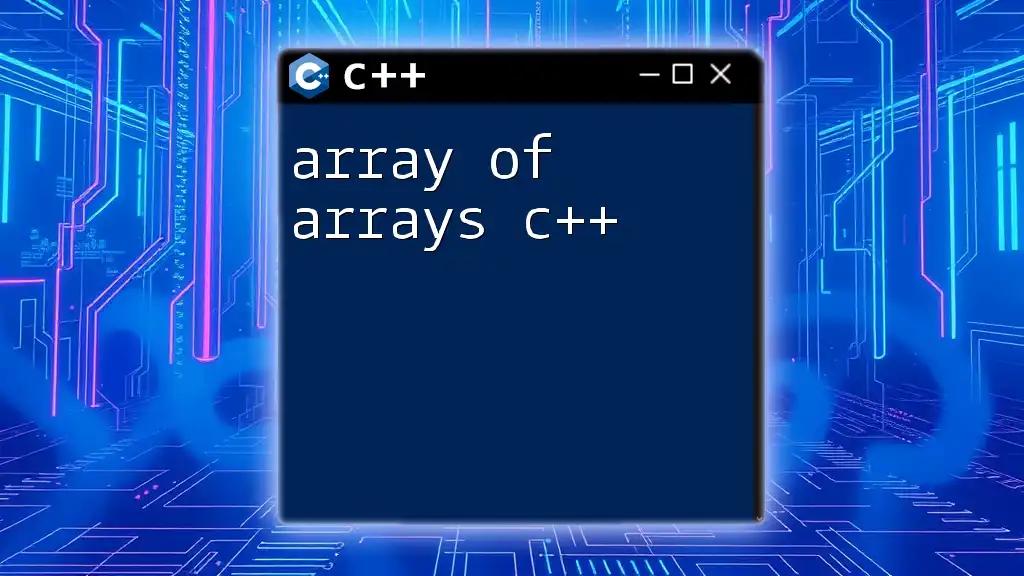
Accessing Elements in an Array of Char Arrays
Indexing Basics
Accessing elements using indexes is straightforward with an array of char arrays. For example:
char words[2][15] = {"Hello", "World"};
cout << words[0]; // Outputs: Hello
Looping Through Array Elements
Utilizing loops allows for effective traversal of an array of char arrays. Here’s an example using a for-loop:
for (int i = 0; i < 2; i++) {
cout << words[i] << endl; // Outputs: Hello World
}
Modifying Elements
You can easily modify specific elements in an array of char arrays. For instance, to change the first string to "Hi":
strcpy(words[0], "Hi");
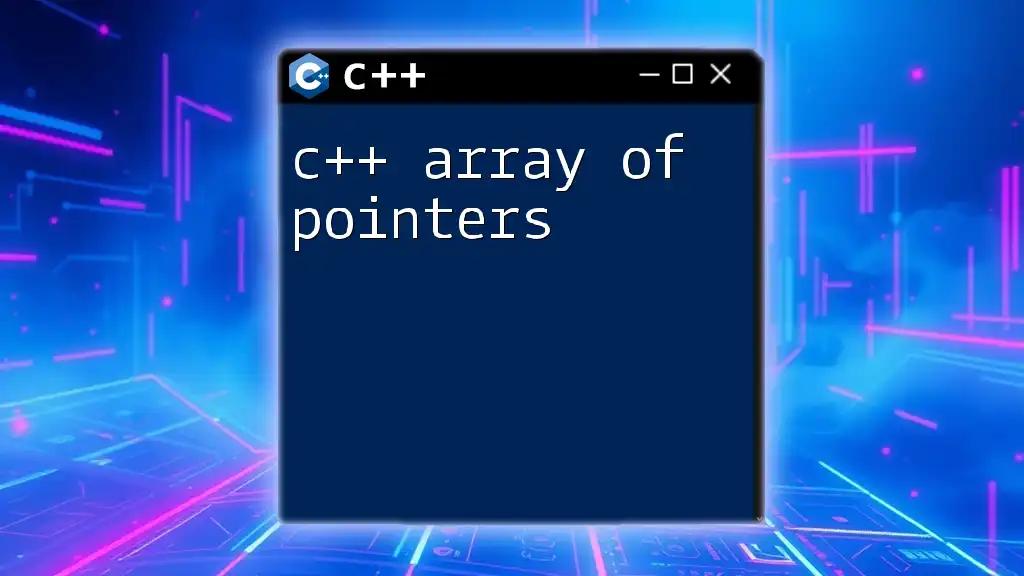
Working with C++ Char Arrays
String Manipulation Techniques
Certain functions are widely used for manipulating char arrays. Some of the most common include:
- `strlen`: Returns the length of a string (excluding the null terminator).
- `strcpy`: Copies one string to another.
- `strcat`: Concatenates two strings.
Here's how these can be implemented:
char first[10] = "Hello";
char second[10] = "World";
char result[20];
// Using strcpy
strcpy(result, first);
strcat(result, second); // Result now contains "HelloWorld"
Comparing Strings
To compare strings, C++ provides the `strcmp` function. This checks the lexicographical ordering of two strings:
if (strcmp(fruits[0], fruits[1]) == 0) {
cout << "Fruits are the same." << endl;
} else {
cout << "Fruits are different." << endl;
}

Use Cases for Array of Char Arrays
Real-World Applications
A common application for arrays of char arrays is managing command-line arguments in C++. Here’s how you can access them:
int main(int argc, char* argv[]) {
for (int i = 0; i < argc; i++) {
cout << argv[i] << endl; // Outputs each command-line argument
}
}
Implementing a Simple Text-Based Menu
Arrays of char arrays are also effective for creating text-based menus. Here’s an example of a simple menu implementation:
char menuOptions[3][20] = {"Start Game", "Settings", "Quit"};
for (int i = 0; i < 3; i++) {
cout << menuOptions[i] << endl;
}
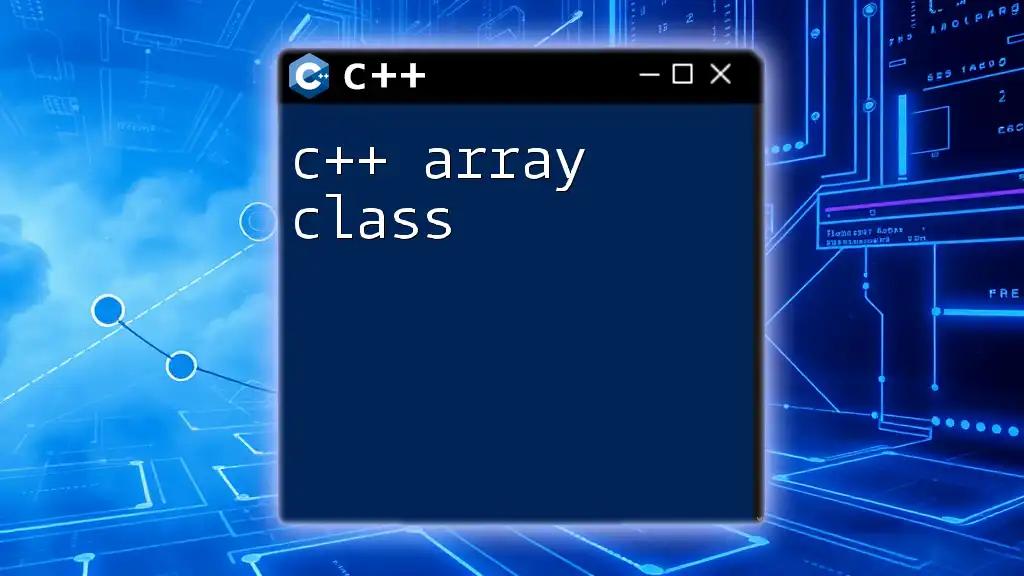
Common Mistakes and Best Practices
Avoiding Dangling Pointers
One common issue with char arrays is creating dangling pointers, which occur when a pointer references a memory location that has already been freed. Always ensure that memory remains valid while it is being accessed.
Buffer Overflows
Buffer overflows happen when writing data outside the allocated size for a char array, which can lead to crashes or security vulnerabilities. To mitigate this, always validate the size of strings being copied or concatenated, and consider using safer alternatives like `strncpy`.
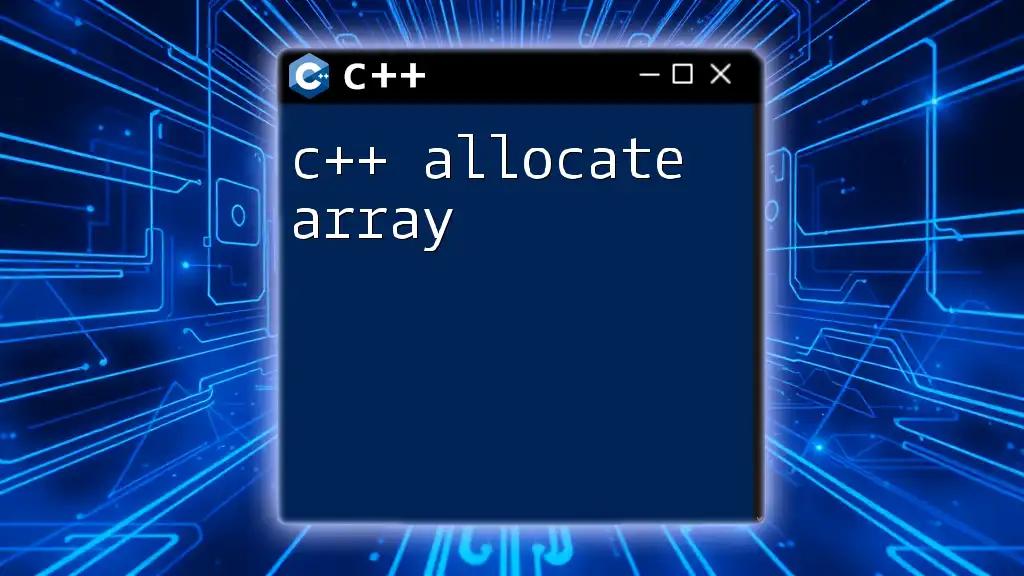
Conclusion
In summary, understanding a C++ array of char arrays empowers you to store and manage a collection of strings effectively. By utilizing proper declaration, initialization, and manipulation techniques, you can streamline your string handling in C++ applications. Embrace these concepts for robust programming practices and enhance your ability to work with character data seamlessly.
Call to Action
Continue your journey in mastering C++ by exploring additional resources and tutorials focusing on char arrays and other related topics. Engage in practical exercises to solidify these concepts and become proficient in C++.

Additional Resources
Further Reading
Dive deeper into character manipulation and arrays with comprehensive documentation and tutorials available online.
Example Projects
Consider implementing projects that leverage arrays of char arrays, such as text-based games or data entry applications, to enhance your understanding and skills.