In C++, you can copy an array using a loop or the `std::copy` function from the `<algorithm>` header for better readability and performance.
Here's a code snippet demonstrating both methods:
#include <iostream>
#include <algorithm>
int main() {
int source[] = {1, 2, 3, 4, 5};
int destination[5];
// Method 1: Using a loop
for (int i = 0; i < 5; ++i) {
destination[i] = source[i];
}
// Method 2: Using std::copy
std::copy(std::begin(source), std::end(source), destination);
// Output the destination array
for (int i : destination) {
std::cout << i << " ";
}
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a collection of elements, all of the same type, stored at contiguous memory locations. This allows for efficient data processing and manipulation. In C++, arrays can be declared using the following syntax:
type arrayName[arraySize];
For example, to declare an integer array with five elements:
int numbers[5];
Types of Arrays
Arrays come in various forms:
-
One-Dimensional Arrays: These are the simplest form of arrays, designed to hold a linear sequence of elements.
-
Multi-Dimensional Arrays: These arrays, such as two-dimensional arrays (matrix), allow for the storage of data in more than one dimension, enabling complex data structures.
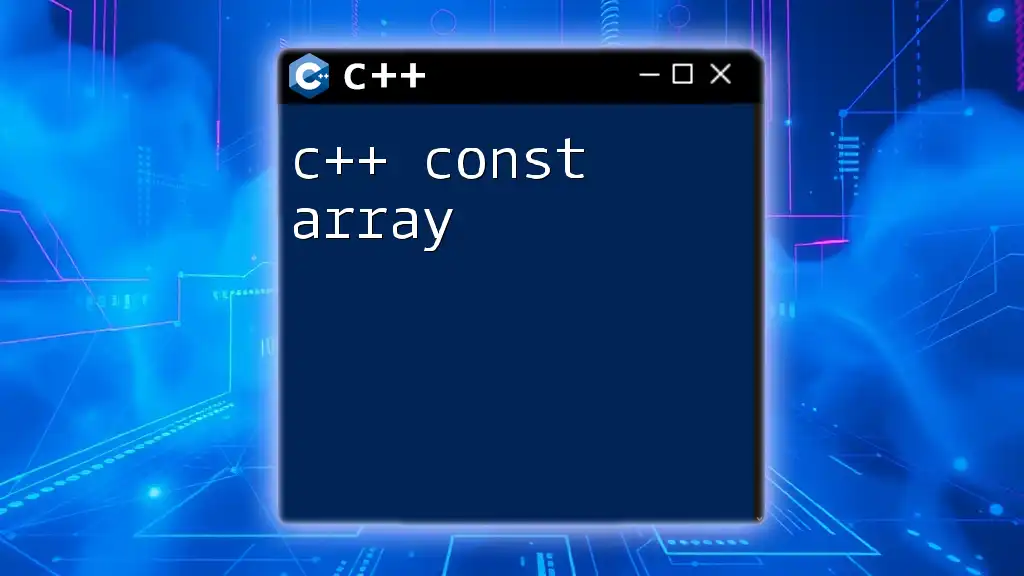
Why Copy Arrays?
When You Need to Copy an Array
Copying arrays is crucial in scenarios where data needs to be replicated without altering the original source. Some common situations that require duplication include:
- Storing a backup of an array.
- Modifying a copy of the array without impacting the original data.
- Passing array data to functions where changes should not reflect in the source array.
Understanding the performance considerations is also essential. Copying large arrays can be resource-intensive, so selecting the appropriate method is vital for maintaining efficiency.
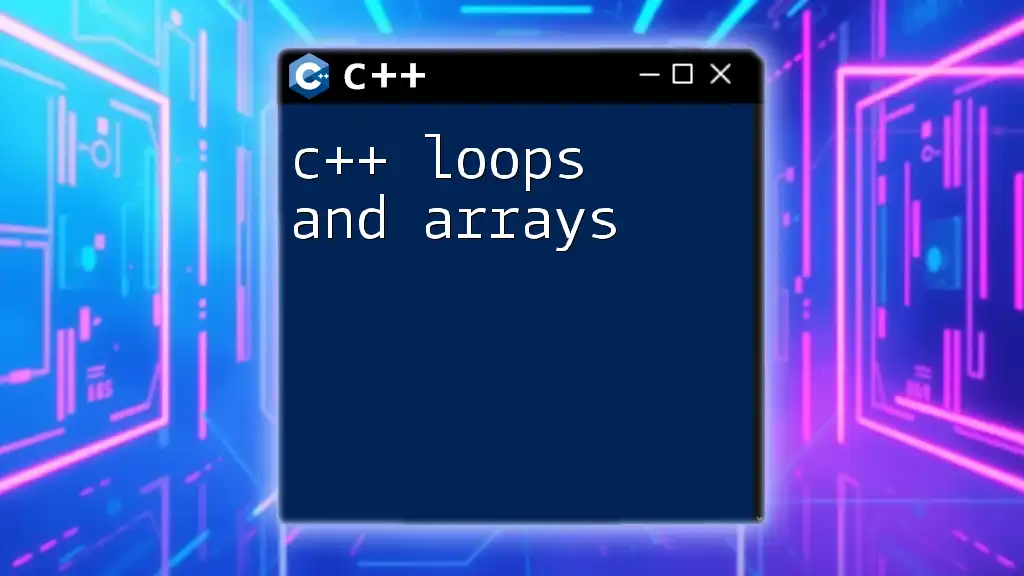
Methods to Copy Arrays in C++
Using a Loop to Copy an Array
One of the most fundamental methods for copying an array is through loops. This approach provides clear semantics on how each element is transferred.
- For Loop Method: The `for` loop is a straightforward way to copy each element.
int source[] = {1, 2, 3, 4, 5};
int destination[5];
for (int i = 0; i < 5; i++) {
destination[i] = source[i];
}
In this example, each element in the `source` array is copied into the `destination` array one by one. This method's simplicity makes it easy to understand and debug.
- While Loop Method: Alternatively, a `while` loop can also be employed for this task:
int source[] = {1, 2, 3, 4, 5};
int destination[5];
int i = 0;
while (i < 5) {
destination[i] = source[i];
i++;
}
Using a `while` loop may be less conventional but can be useful in certain scenarios where the termination condition may be dynamic.
Using the `std::copy` Function from the Standard Library
The C++ Standard Library provides a powerful utility for copying arrays—`std::copy`. This function simplifies the copying process and optimizes performance.
#include <algorithm>
int main() {
int source[] = {1, 2, 3, 4, 5};
int destination[5];
std::copy(source, source + 5, destination);
}
This method fosters cleaner code and leverages the optimizations within the standard library implementation, making it a preferred choice in modern C++.
Copying Arrays Using `memcpy`
If performance is your primary concern, especially for large arrays, `memcpy` from the `<cstring>` header is an incredibly efficient option.
#include <cstring>
int main() {
int source[] = {1, 2, 3, 4, 5};
int destination[5];
std::memcpy(destination, source, sizeof(source));
}
Note: While `memcpy` is fast, it performs a shallow copy and does not account for dynamic data structures, which may lead to unexpected behaviors if used improperly.
Using C++11 Range-Based For Loops
With the advent of C++11, a more modern syntax emerged that simplifies array copying through range-based for loops:
int source[] = {1, 2, 3, 4, 5};
int destination[5];
int i = 0;
for (const auto& value : source) {
destination[i++] = value; // Assume 'i' starts from 0
}
This approach enhances code readability and promotes a more intuitive understanding of the copying process.
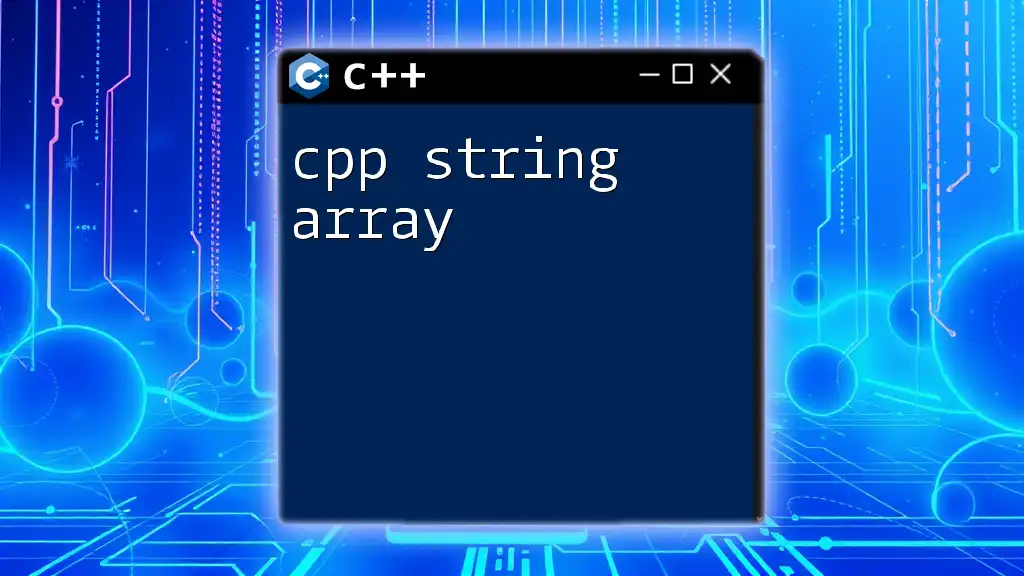
Copying Multi-Dimensional Arrays
Copying 2D Arrays with Loops
For multi-dimensional arrays, copying requires nested loops. Consider the following example for copying a two-dimensional array:
int source[2][3] = {{1, 2, 3}, {4, 5, 6}};
int destination[2][3];
for (int i = 0; i < 2; i++) {
for (int j = 0; j < 3; j++) {
destination[i][j] = source[i][j];
}
}
This double-loop structure allows you to traverse and copy each element from the source matrix to the destination matrix meticulously.
Using Nested Loops with `std::copy`
For added clarity, you can also combine nested loops with `std::copy` for copying multi-dimensional arrays.
#include <algorithm>
int main() {
int source[2][3] = {{1, 2, 3}, {4, 5, 6}};
int destination[2][3];
for (int i = 0; i < 2; i++) {
std::copy(source[i], source[i] + 3, destination[i]);
}
}
This technique maintains clarity while taking advantage of library functions for performance.
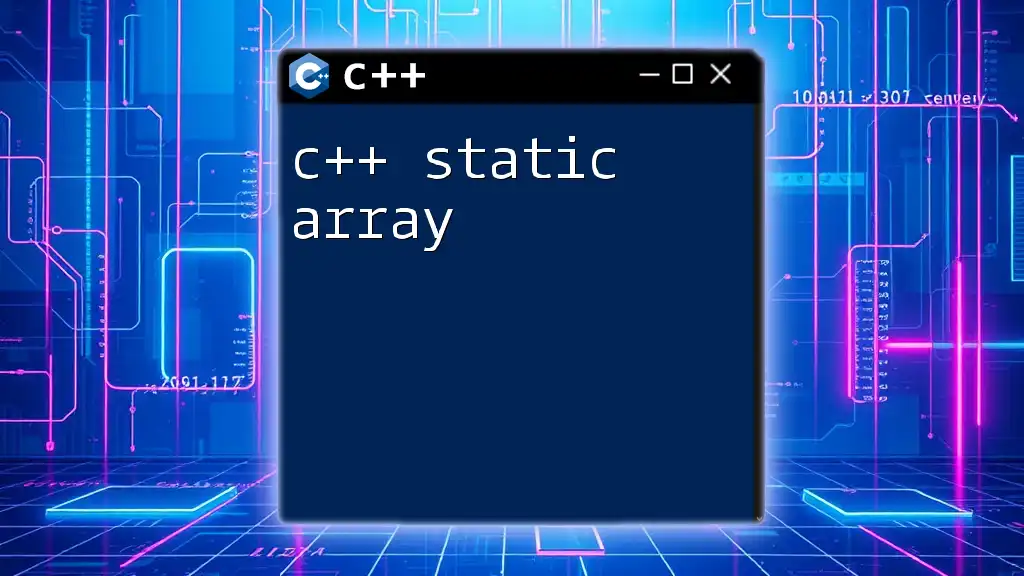
Common Pitfalls When Copying Arrays
Handling Different Sizes
When copying, always ensure that the destination array is of the same size or larger than the source array. Failing to do so can lead to memory corruption and unexpected behavior.
Shallow vs Deep Copy
Understanding the difference between shallow copies and deep copies is critical:
- A shallow copy duplicates the array's pointers; if data is modified in one array, the changes reflect in the other.
- A deep copy duplicates the actual data, ensuring both arrays can be modified without affecting each other.
Paying careful attention to these differences can enhance data safety and integrity when manipulating arrays.
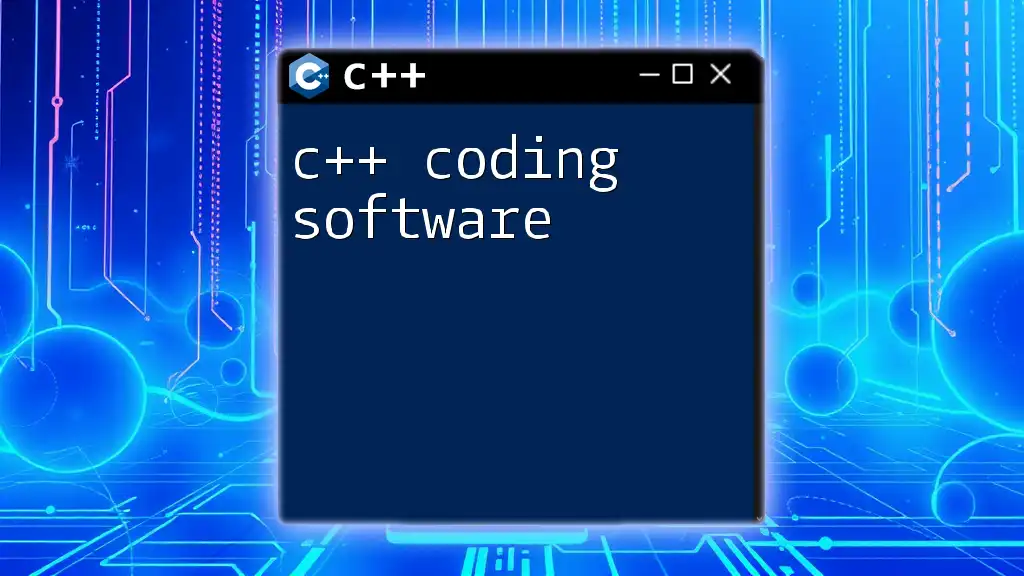
Conclusion
In conclusion, c++ copying arrays is a fundamental skill that every C++ programmer should master. From using loops to employing STL functions like `std::copy` and `memcpy`, various techniques cater to different scenarios and performance requirements. Whether you are handling simple one-dimensional arrays or complex multi-dimensional matrices, understanding these methods will empower you to write efficient and clean code.
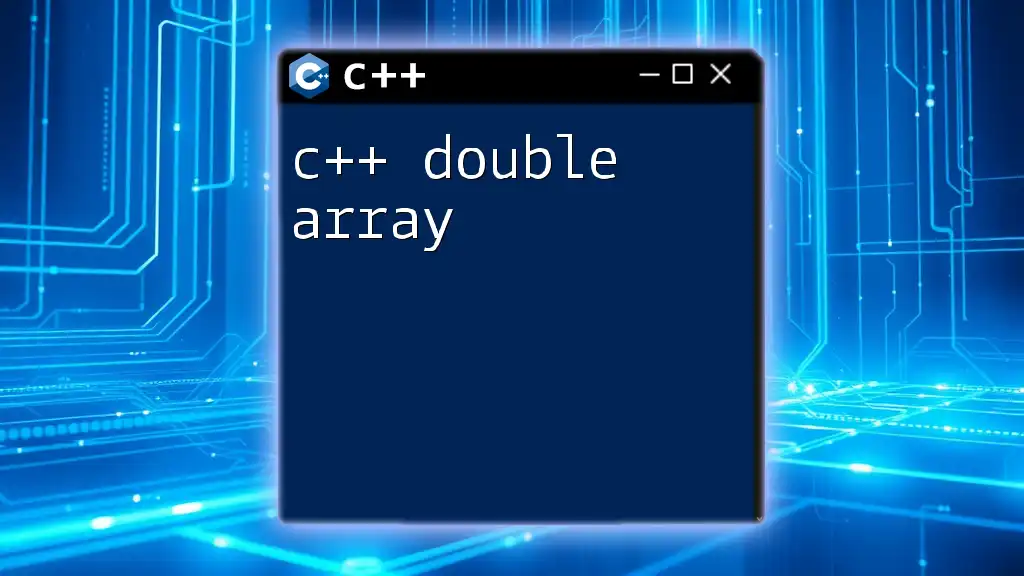
Further Reading and Resources
To expand your knowledge, consider looking into C++ documentation for Standard Library functions and exploring practical projects that involve complex data structures.
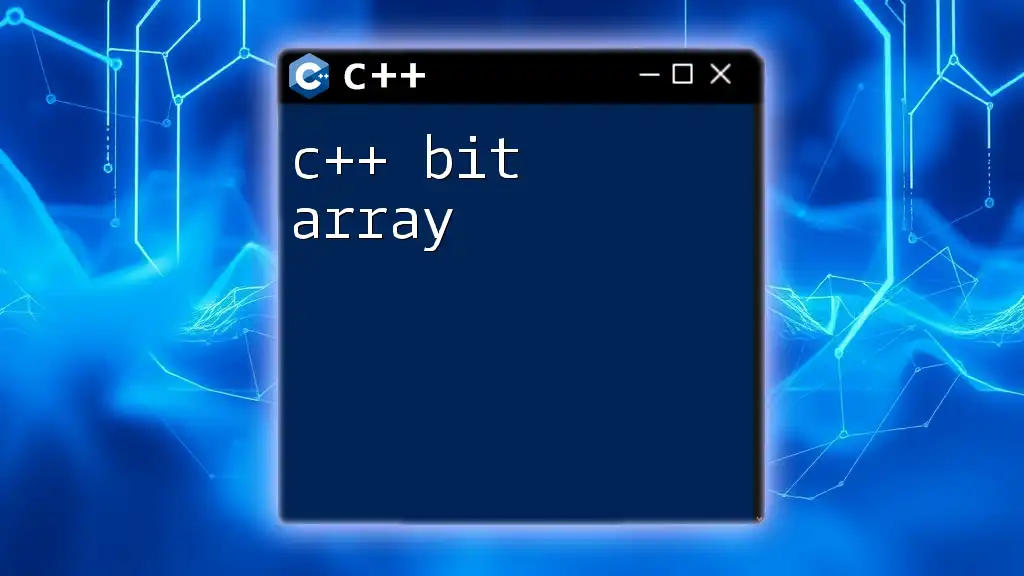
Call to Action
Feel free to share your experiences with copying arrays in C++, or ask questions if you encounter any challenges or have insights to provide!