In C++, you can fill an array with a specific value using a simple loop or the `std::fill` function from the `<algorithm>` header.
#include <iostream>
#include <algorithm>
int main() {
const int size = 5;
int array[size];
// Fill the array with the value 42
std::fill(array, array + size, 42);
// Print the array
for (int i = 0; i < size; ++i) {
std::cout << array[i] << " ";
}
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a data structure that allows you to store a fixed-size sequential collection of elements of the same type. Arrays are essential in C++ for managing collections of data efficiently. There are two primary types of arrays:
- Static Arrays: These have a fixed size and their size must be known at compile time.
- Dynamic Arrays: These can change in size during program execution, often created using pointers and dynamically allocated memory.
Here’s a simple example of declaring a static array in C++:
int arr[5]; // declaration of an array with a size of 5
Array Properties
Arrays in C++ are indexed, meaning that each element can be accessed using its corresponding index. The indices start at 0 and range to size-1. Each element in an array must be of the same type, ensuring consistency in how data is processed. Here’s an example of accessing elements in an array:
int arr[5] = {10, 20, 30, 40, 50};
cout << arr[0]; // Outputs: 10
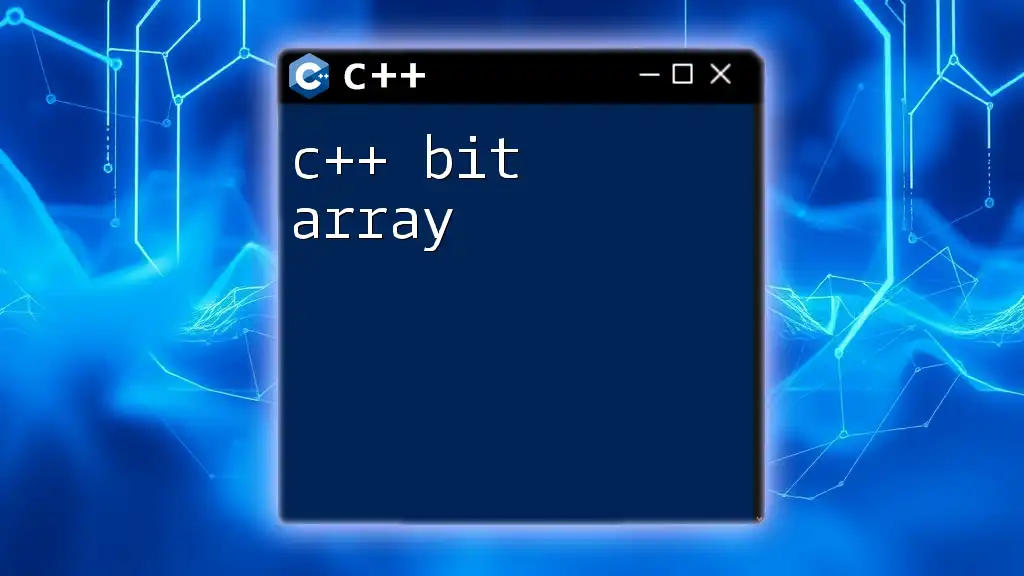
Filling Arrays in C++
Using Loops
Filling an array can be efficiently achieved using loops.
For Loop
The `for` loop is the most commonly used loop for filling arrays. It allows you to iterate through each index of the array seamlessly. Here’s an example of using a `for` loop to fill an array with even numbers:
int arr[5];
for (int i = 0; i < 5; i++) {
arr[i] = i * 2; // Filling array with even numbers
}
While Loop
The `while` loop is another option that can be used to fill arrays, although it's less common. It continues to execute as long as a specified condition is true. Here’s how you could use a `while` loop to fill an array with multiples of 3:
int arr[5];
int i = 0;
while (i < 5) {
arr[i] = i * 3; // Filling array with multiples of 3
i++;
}
Using Standard Library Functions
The C++ Standard Library provides efficient functions for working with arrays.
std::fill
The `std::fill` function is a convenient way to set all elements of an array to a specific value. It requires the `<algorithm>` header and can be implemented as follows:
#include <algorithm> // Required for std::fill
#include <iostream>
using namespace std;
int main() {
int arr[5];
std::fill(arr, arr + 5, 7); // Fill with the value 7
for (int i : arr) {
cout << i << " "; // Output: 7 7 7 7 7
}
return 0;
}
In this example, `std::fill` assigns the value 7 to each element in the array efficiently.
std::generate
`std::generate` allows you to fill an array with values produced by a generator function or a lambda expression. This is particularly useful for filling an array with complex values. Here’s an example that demonstrates this:
#include <algorithm>
#include <iostream>
using namespace std;
int main() {
int arr[5];
int value = 0;
std::generate(arr, arr + 5, [&value]() { return value += 5; }); // Fill with increasing multiples of 5
for (int i : arr) {
cout << i << " "; // Output: 5 10 15 20 25
}
return 0;
}
In this case, `[]() { return value += 5; }` is a lambda function that increases the value of value by 5 for each element in the array.
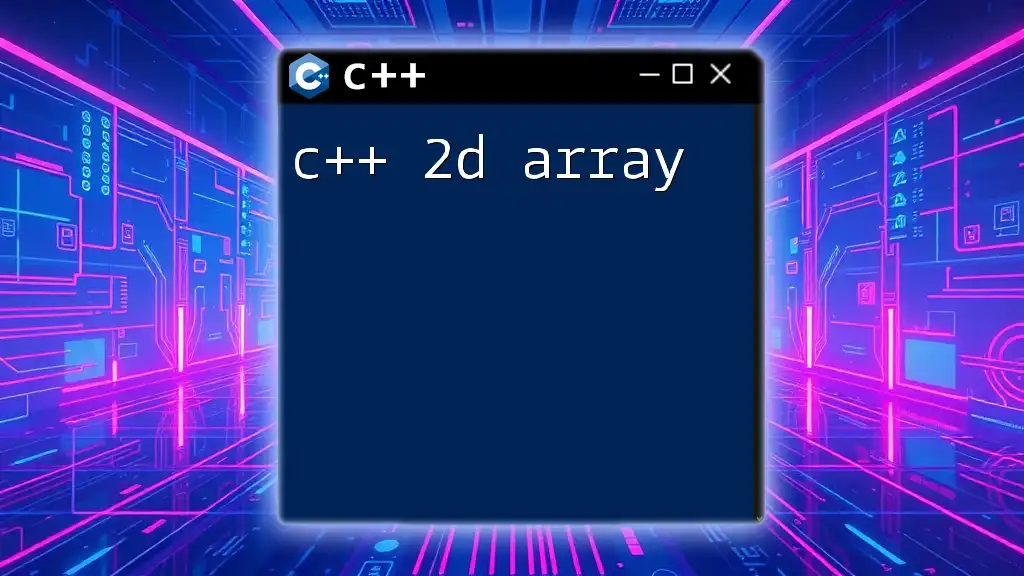
Filling Multi-Dimensional Arrays
What are Multi-Dimensional Arrays?
Multi-dimensional arrays are arrays of arrays, allowing the storage of data in a tabular form. The most common type is the 2D array, which is often used for representing matrices.
Here’s a way to declare a 2D array in C++:
int arr[3][3]; // Declaration of a 3x3 2D array
Filling Multi-Dimensional Arrays
Filling a multi-dimensional array can be done using nested loops.
Using Nested Loops
When filling a 2D array, nested loops are crucial, as you must iterate over both rows and columns. Here’s an example:
int arr[3][3];
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
arr[i][j] = i * j; // Filling 2D array with products of indices
}
}
Each element `arr[i][j]` is filled with the products of its indices, thus creating a multiplication table.
Using std::fill for 2D Arrays
You can also use `std::fill` to fill each row of a 2D array. This is effective when you want to initialize all elements in a specific row to the same value. Here’s how you can do it:
int arr[3][3];
for (int i = 0; i < 3; i++) {
std::fill(arr[i], arr[i] + 3, 1); // Fill each row with 1s
}
This example fills each row of the 2D array with the value 1.
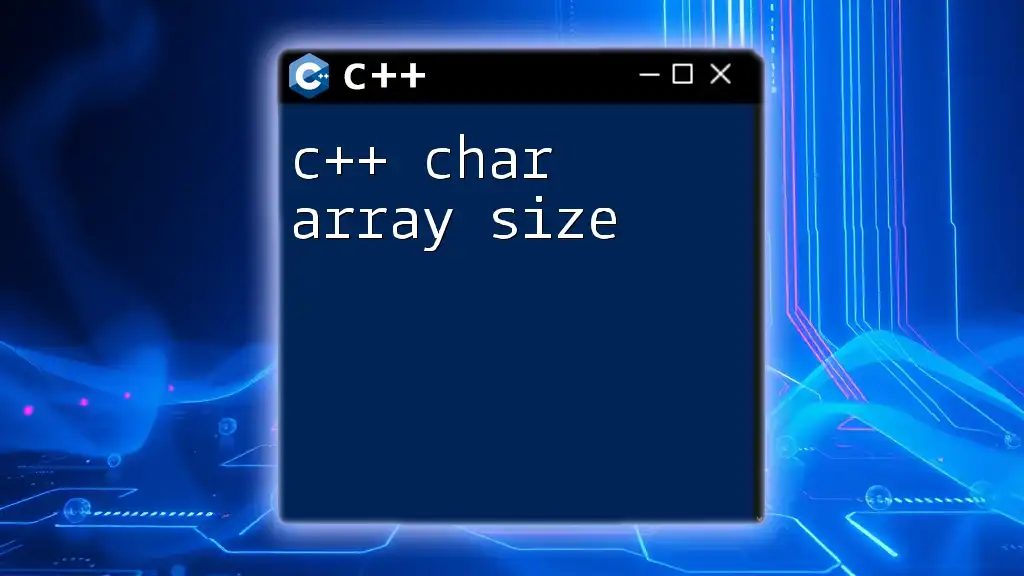
Best Practices for Filling Arrays
Initial Value Assignment
To avoid garbage values, ensure that arrays are properly initialized upon declaration. Setting a default value helps maintain consistent and predictable behavior within your applications.
Avoiding Out-of-Bounds Access
Out-of-bounds access can lead to undefined behavior or runtime errors. Always ensure that your loop indices are within the bounds of the array size. Implement validation checks where necessary.
Performance Optimization
The choice between using loops and standard library functions can significantly affect performance, especially with larger arrays. In general, `std::fill` and `std::generate` are highly optimized and should be preferred for better performance compared to manual iteration techniques.
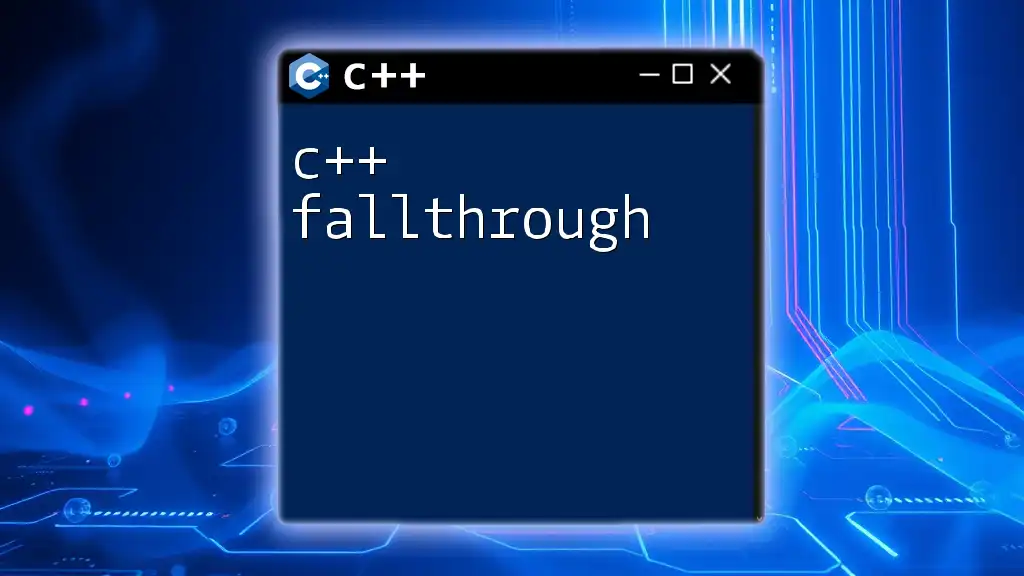
Conclusion
This comprehensive approach to the topic of c++ fill array provides a clear direction on how to efficiently fill arrays using various methods, from conventional loops to modern C++ standard algorithms. Practice with these techniques will not only enhance your proficiency in C++ programming but also help you write cleaner, more maintainable code. Experiment with filling arrays using different methods to find the most efficient solutions for your specific needs.
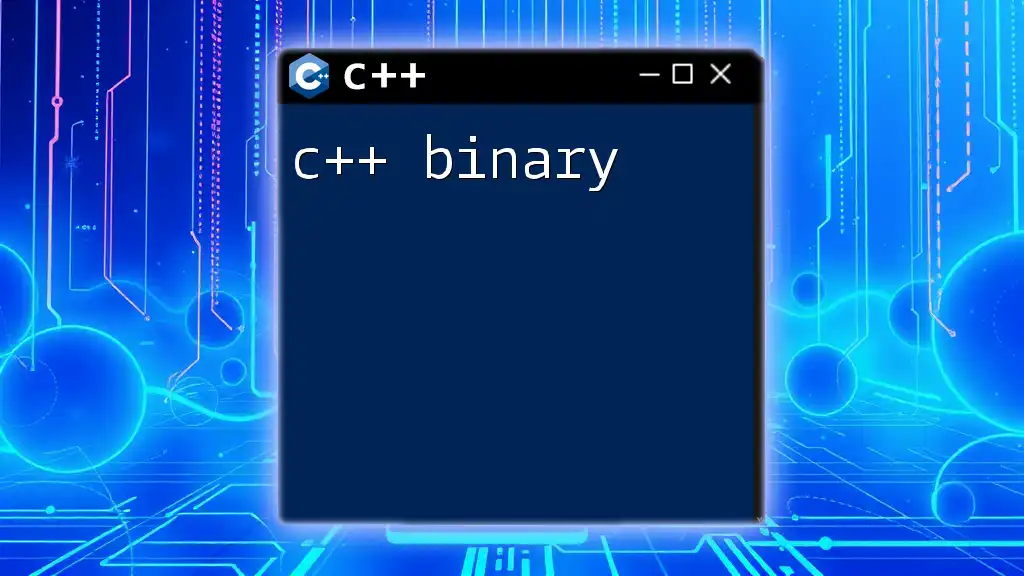
Additional Resources
For further exploration of C++ array manipulation, consider reading the official C++ documentation and online tutorials that focus on advanced techniques in array handling and optimization strategies.