In C++, you can terminate a thread using the `std::thread::detach()` method to allow the thread to run independently, or the `std::terminate()` function, but it's important to note that threads should generally be stopped gracefully if possible.
Here’s a code snippet demonstrating how to use `std::thread::detach()`:
#include <iostream>
#include <thread>
#include <chrono>
void threadFunction() {
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Thread finished executing." << std::endl;
}
int main() {
std::thread myThread(threadFunction);
myThread.detach(); // Detach the thread to allow it to run independently
std::cout << "Main thread is continuing..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(1));
return 0;
}
Keep in mind that detaching a thread makes it difficult to manage and join its state later, so design your threading model carefully.
Understanding Threads in C++
What is a Thread?
In programming, a thread is the smallest unit of processing that can be scheduled by an operating system. It's a sequence of instructions that can be executed independently from the rest of the program. In C++, threads allow multiple operations to run in parallel, significantly improving performance in applications that are designed for concurrent execution.
Benefits of Using Threads
Using threads in your C++ applications has several advantages:
-
Improved Application Performance: Threads enable tasks to be performed simultaneously, which can significantly reduce overall execution time, especially on multi-core processors.
-
Concurrent Execution: By allowing multiple threads to execute concurrently, you can manage large tasks more efficiently. For instance, you can run background operations while keeping your application responsive to user input.
-
Efficient Resource Utilization: Threads share the same memory space, making them lighter than processes. This leads to better resource management, especially in applications that require frequent context switching.
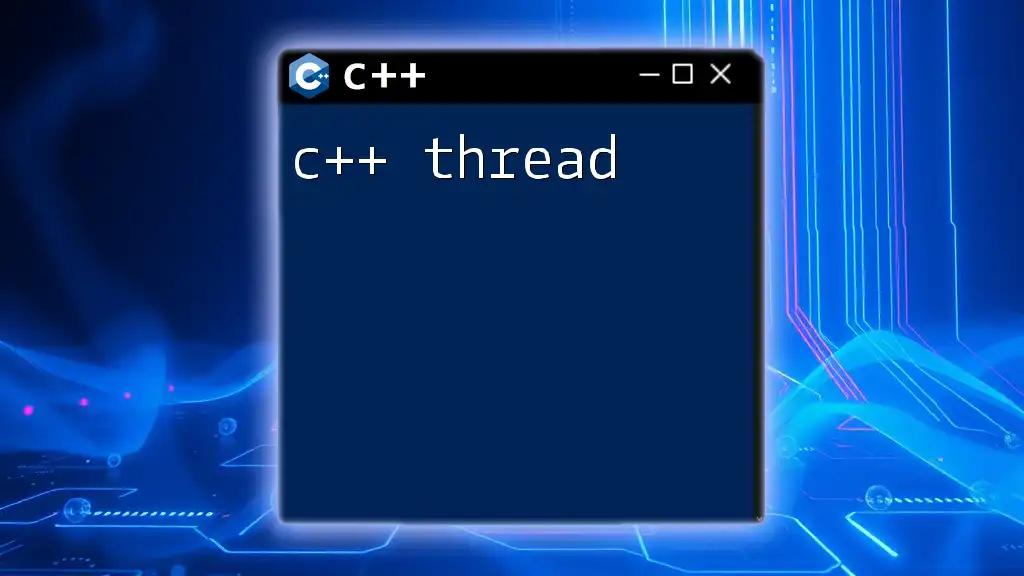
Thread Lifecycle
Creation
In C++, threads can be created using the `std::thread` class, which is part of the C++ Standard Library. This class provides a simple and flexible interface for managing threads.
Here’s an example of creating a thread:
#include <iostream>
#include <thread>
void threadFunction() {
std::cout << "Thread is running" << std::endl;
}
int main() {
std::thread myThread(threadFunction); // Create a new thread
myThread.join(); // Wait for the thread to finish
return 0;
}
Running
Once created, a thread runs independently and executes the function it was assigned. Threads can perform various tasks concurrently, and in the above example, the message "Thread is running" will be printed once the function executes.
Joining
Joining a thread is a crucial step in its lifecycle. When you join a thread, you make the main thread wait for it to complete. This ensures that all resources are cleaned up and properly returned, preventing memory leaks.
Here’s an example demonstrating `join()`:
std::thread myThread(threadFunction);
myThread.join(); // Main thread waits for myThread to finish executing
Detaching
Detaching a thread allows it to run independently of the main thread. After detachment, you cannot join that thread; it continues its execution and cleans up its resources when done.
std::thread myThread(threadFunction);
myThread.detach(); // Now myThread runs independently

Killing Threads in C++
Why and When to Kill Threads
Sometimes, it becomes necessary to kill a thread, or terminate its execution, especially in scenarios like:
- Unresponsive threads that lead to application hangs.
- Long-running processes that need to be abruptly stopped based on certain conditions.
However, forcibly killing threads can lead to risks like resource leaks and data corruption, so it should be approached with caution.
C++ Standard Library and Thread Termination
The Need for Thread Safety
When terminating threads, it's vital to ensure thread safety to prevent issues like deadlocks or unintended behavior. Therefore, safe mechanisms for halting thread execution must be considered.
Approaches to Killing Threads
Using Flags for Safe Termination
A common and safe approach to terminate a thread is by using a shared boolean flag. The thread periodically checks the flag to see if it should continue executing. When set to `false`, the thread will exit gracefully.
volatile bool stopThread = false;
void threadFunction() {
while (!stopThread) {
// Execute thread work
}
// Perform cleanup
}
Here, setting `stopThread = true` in the main thread will prompt `threadFunction` to complete its current iteration and exit safely.
Using `std::terminate()`
In extreme cases where you need to abort a thread immediately, you can use `std::terminate()`. This should be used with care, as it does not guarantee resource cleanup.
#include <iostream>
#include <thread>
void dangerFunction() {
// Perform some operation
std::terminate(); // Abruptly kill the thread
}
While effective, this method should be a last resort due to its potential to leave resources in an indeterminate state.
C++20 Features for Thread Management
Introduction to `std::jthread`
C++20 introduced `std::jthread`, which simplifies thread management by automatically joining upon destruction. This new thread type helps prevent common mistakes associated with forgetting to join threads.
Here's a concise example:
#include <iostream>
#include <thread>
void func() {
std::cout << "Thread is processing..." << std::endl;
}
int main() {
std::jthread t(func); // Automatically joins upon destruction
return 0;
}
By using `std::jthread`, you reduce the risk of leaking resources or encountering dangling thread issues.
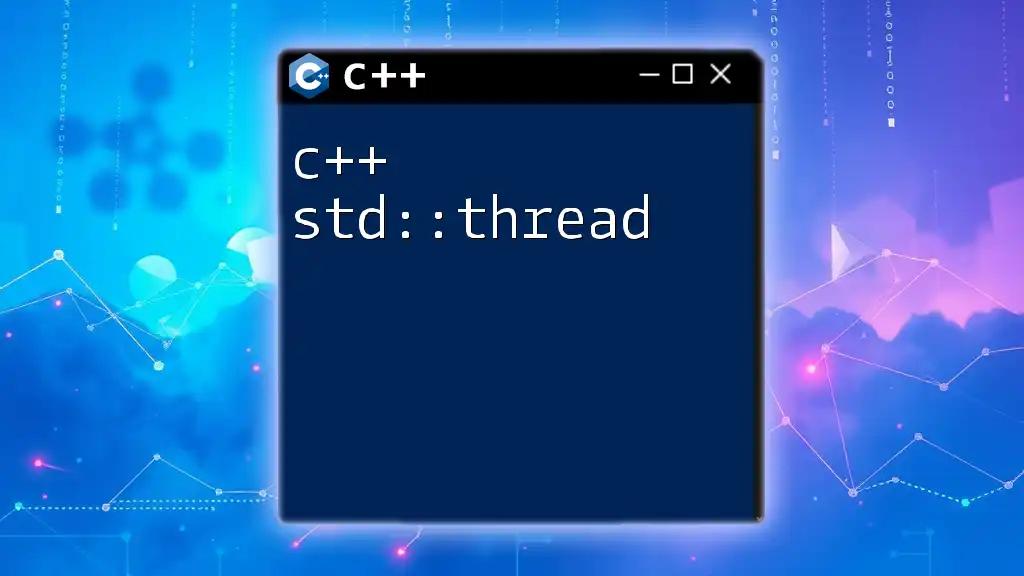
Best Practices for Thread Management
Handling Exceptions
Exception handling in multi-threaded applications is crucial. If a thread encounters an exception and it's not caught, the program can crash. Therefore, you should implement try-catch blocks within your thread functions.
Resource Management
Properly managing resources within your threads is imperative. Always ensure that any allocated resources are released upon thread completion. This can often be managed in the thread’s destructor or in a cleanup phase before exiting.
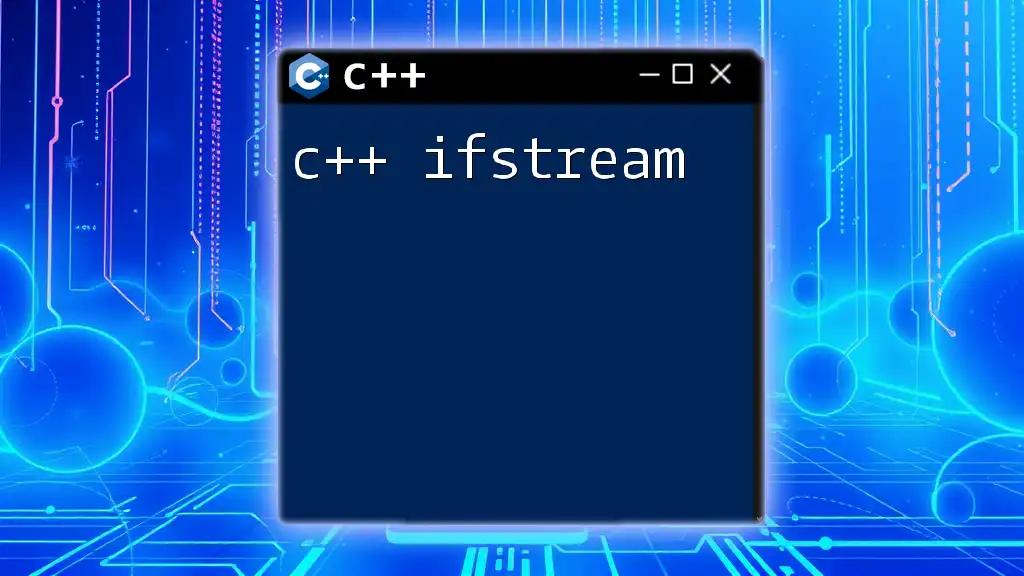
Conclusion
Understanding how to manage threads in C++ effectively is essential for developing robust applications. The ability to kill a thread safely involves recognizing when it is necessary and implementing proper strategies to do so without risking data integrity or resource leaks. As you delve deeper into threading in C++, consider exploring more advanced topics such as thread pools and synchronization mechanisms to further enhance your applications.

Additional Resources
For further insights into thread management in C++, consider exploring the following:
- Recommended books: C++ Concurrency in Action by Anthony Williams
- Official C++ documentation related to threading for up-to-date standards
- Comprehensive online courses focused on multi-threading in C++

FAQs
Can I forcibly kill a thread in C++?
It is generally discouraged to forcibly kill threads as it can lead to serious problems like resource leaks and inconsistent states.
What happens if I leave a thread running?
Leaving a thread running without termination could lead to wasted resources and potentially prevent your application from shutting down cleanly.
Are there alternatives to using threads?
Yes, alternatives such as thread pools and async functions can provide better resource management and flexibility, especially for tasks that do not require continuous execution.
By applying the principles discussed in this guide, you can effectively manage thread execution, ensuring a more efficient and reliable application experience.