C++ multithreading allows concurrent execution of tasks, enabling efficient use of system resources and improved performance for applications.
Here's a simple example showcasing how to create and run multiple threads in C++:
#include <iostream>
#include <thread>
void greet(int id) {
std::cout << "Hello from thread " << id << std::endl;
}
int main() {
std::thread t1(greet, 1);
std::thread t2(greet, 2);
t1.join();
t2.join();
return 0;
}
What is Multithreading in C++?
Multithreading in C++ refers to the concurrent execution of multiple threads within a single process. A thread is the smallest unit of processing that can be executed independently. Multithreading allows a program to perform multiple operations simultaneously, enhancing performance, responsiveness, and resource utilization, particularly in applications that require parallel processing.
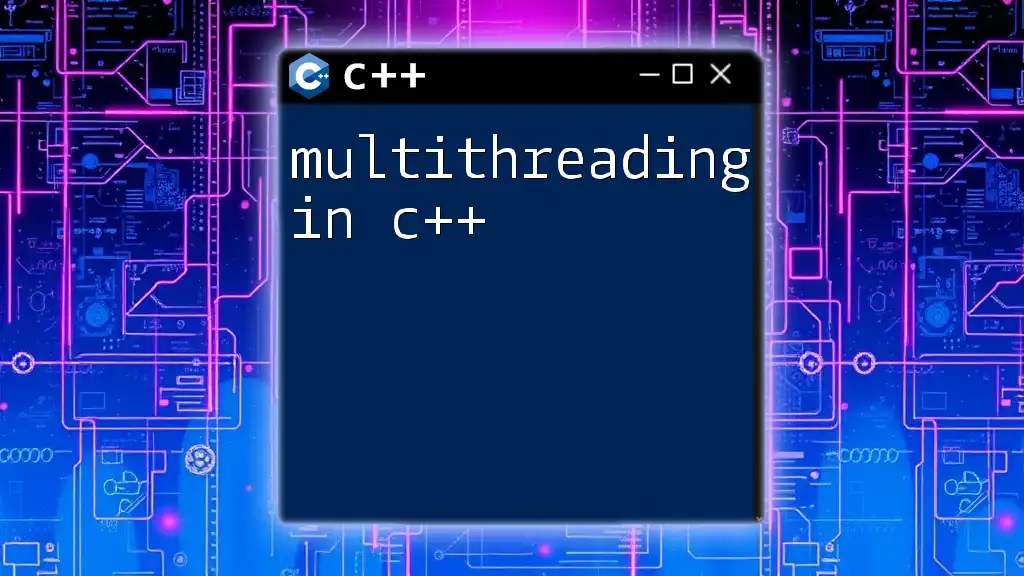
Understanding the Basics of Multithreading in C++
Defining Threads
A thread is often compared to a lightweight process. Unlike processes, which are independent, threads within the same process share resources but can execute independently. This makes communication between threads faster and more efficient.
How Multithreading Works in C++
In C++, multithreading is primarily supported through the Standard Library, which includes the `<thread>` header. This library abstracts the complexities of thread operations, providing a simple and effective API for multi-thread management.
The thread lifecycle includes the following stages:
- Creation: A thread is created, either running a function or executing a lambda expression.
- Execution: The thread runs concurrently with other threads.
- Destruction: Once the task is completed, the thread can be joined or detached from the main execution flow.
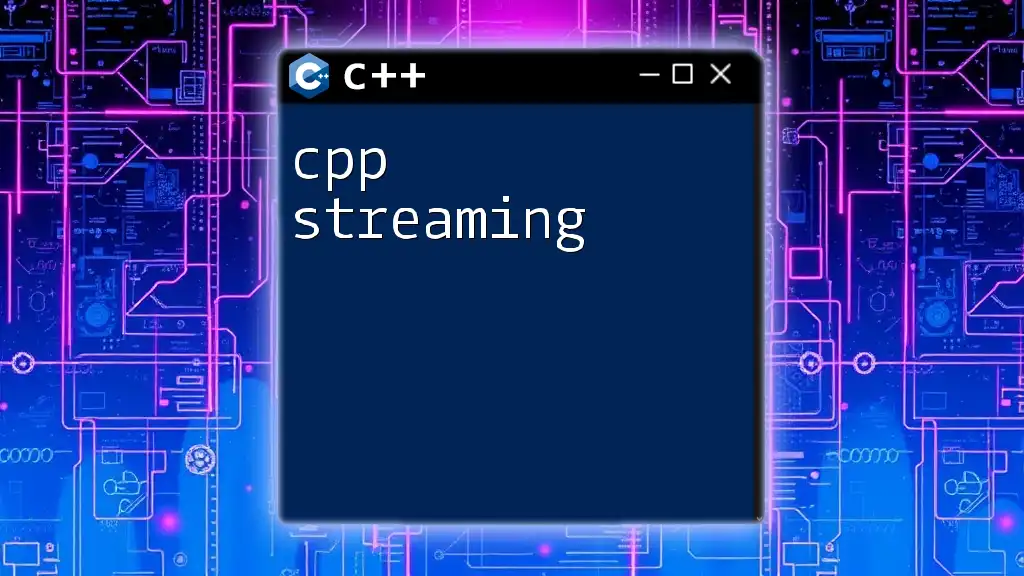
Getting Started with Multithreading in C++
Setting Up Your Environment
Before you dive into coding, ensure that your development environment is set up correctly. You will need:
- A C++ compiler that supports C++11 or later. Examples include GCC and Microsoft Visual Studio.
- Basic libraries that come with your compiler will typically include threading support.
Creating Your First Thread
Creating a thread in C++ is straightforward using the `<thread>` library. Here’s a simple example:
#include <iostream>
#include <thread>
void func() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(func); // Creating a thread
t.join(); // Wait for thread to finish
return 0;
}
In this example, `func` is the function executed by the newly created thread `t`. The `join` method is crucial as it ensures that the main thread waits for `t` to complete its execution before terminating.
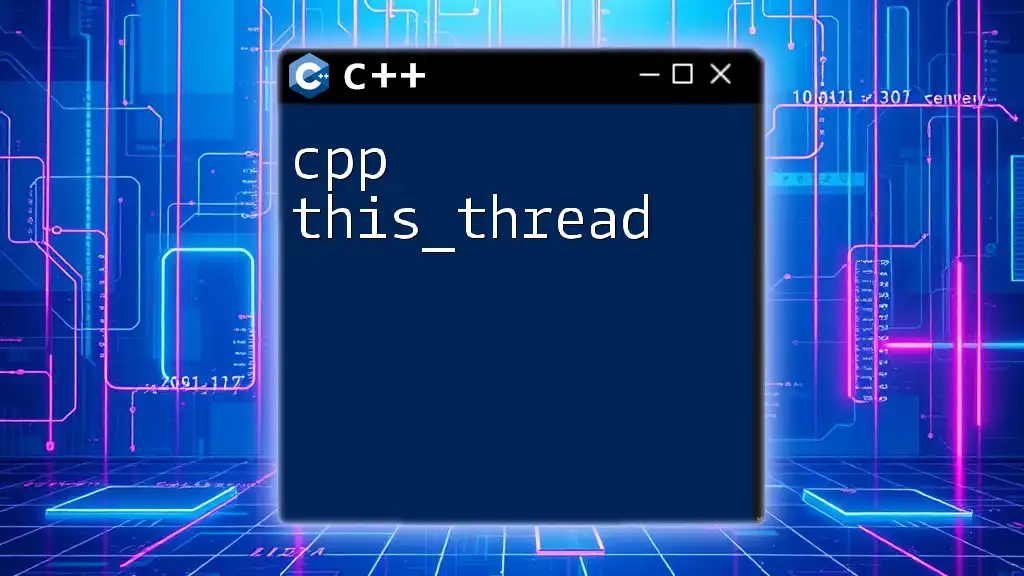
Key Concepts in C++ Multithreading
Thread Management
Managing threads involves creating, joining, and, in some cases, detaching them.
-
Creating and Joining Threads: As shown previously, you can create a thread and wait for it to complete using `join`. Failing to join a thread that has not finished executing can lead to program termination or undefined behavior.
-
Detaching Threads: If you don’t need to wait for a thread to finish, you can detach it. This allows it to run independently.
// Example
std::thread t1(func);
t1.detach(); // Thread runs independently
Detaching can lead to resource management issues if not handled appropriately, especially if the main thread exits before detached threads finish their work.
Thread Synchronization
Synchronizing threads is vital to prevent race conditions, where two threads attempt to modify shared data simultaneously. This can lead to inconsistent or corrupted results.
Mutex and Lock
A mutex (short for mutual exclusion) is a synchronization primitive that prevents multiple threads from accessing shared data at the same time. Here's how to use a mutex:
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx;
void safePrint() {
mtx.lock();
std::cout << "Thread-safe output." << std::endl;
mtx.unlock();
}
In this example, `safePrint` locks the mutex before accessing shared resources, ensuring no other thread can modify them simultaneously. It's essential to unlock the mutex after use to avoid deadlocks.
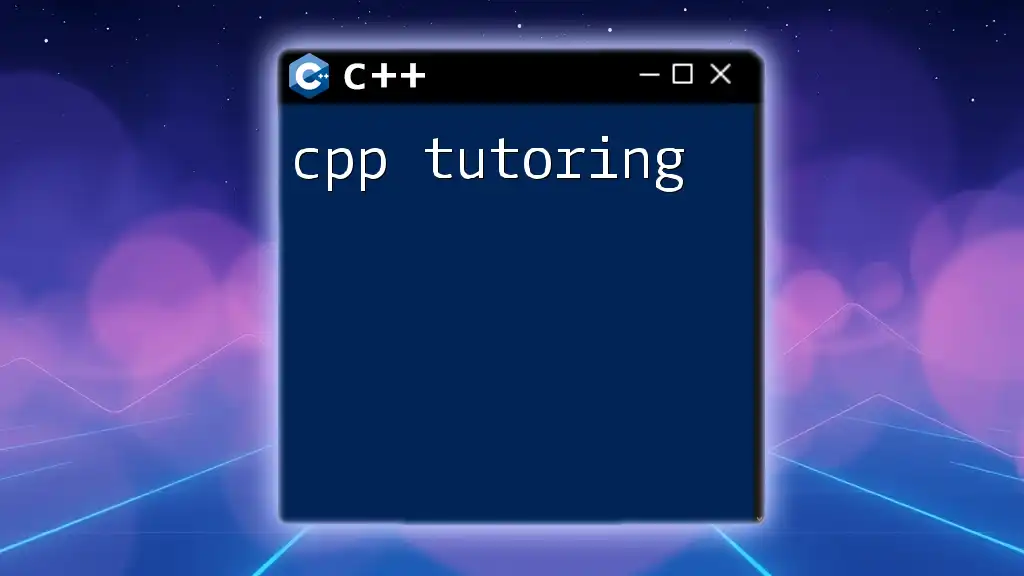
Advanced Multithreading Techniques
Using `std::future` and `std::async`
Futures are another powerful feature provided by C++ for managing asynchronous operations, allowing you to retrieve results from threads. The `std::async` function is an easy way to launch tasks asynchronously:
#include <iostream>
#include <future>
int compute() {
return 42; // Simulated complex computation
}
int main() {
std::future<int> result = std::async(std::launch::async, compute);
std::cout << "Result: " << result.get() << std::endl; // Blocks until the result is ready
return 0;
}
In this code, `compute` runs in a separate thread, and `result.get()` will block until the computation is finished, allowing you to safely retrieve the value.
Thread Pools
Thread pools manage a collection of worker threads and can significantly enhance performance by reusing threads instead of creating new ones for every task. While implementing a thread pool can be complex, the general idea is to have a pool of threads that take tasks from a queue. This optimizes resource allocation and reduces the overhead of thread creation.
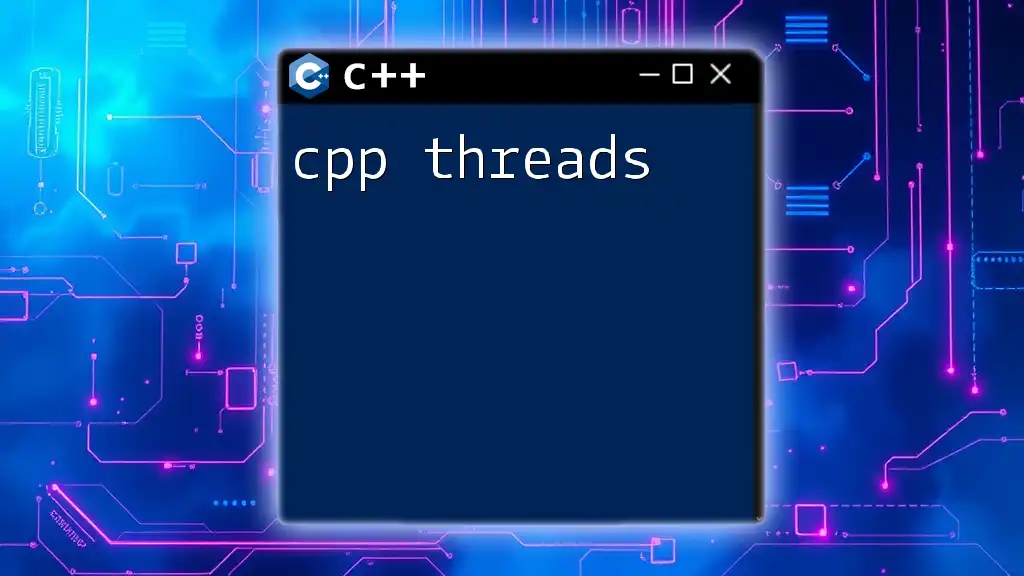
Common Challenges in C++ Multithreading
Race Conditions
Race conditions occur when multiple threads try to access shared data simultaneously, leading to unpredictable results. Consider this scenario:
#include <iostream>
#include <thread>
int counter = 0;
void increment() {
for (int i = 0; i < 1000; ++i) {
++counter; // Race condition here
}
}
int main() {
std::thread t1(increment);
std::thread t2(increment);
t1.join();
t2.join();
std::cout << "Final counter: " << counter << std::endl; // Output may vary
return 0;
}
To avoid race conditions, use mutexes or other synchronization mechanisms to protect shared resources.
Deadlock
Deadlocks occur when two or more threads are waiting for each other to release resources, leading to a standstill. To prevent deadlocks, adopt these strategies:
- Avoid holding multiple mutexes at once.
- Use timed locks, which allow threads to back out if they cannot acquire a lock within a certain timeframe.
- Always lock mutexes in a consistent order.
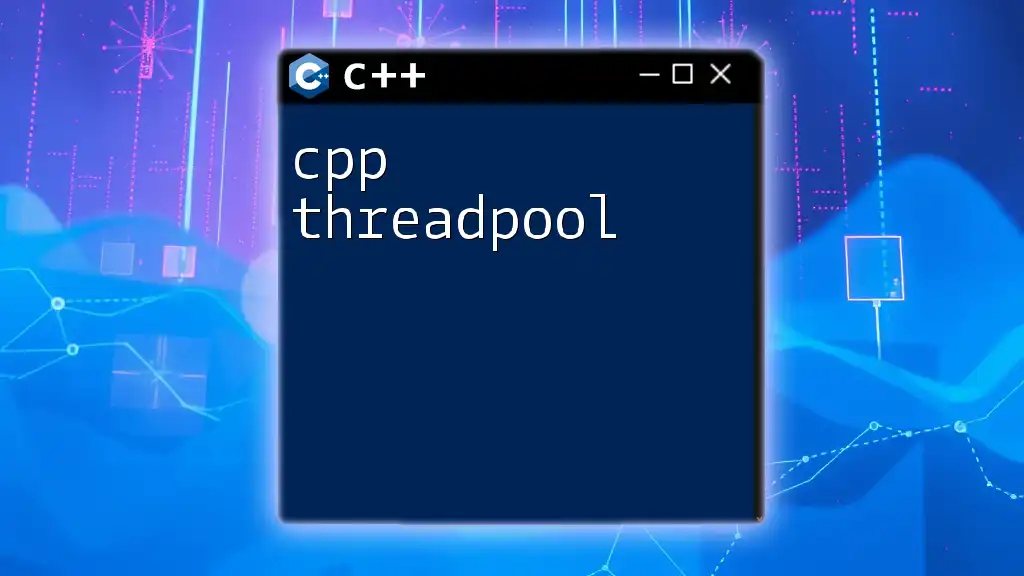
Best Practices for Multithreading in C++
When to Use Multithreading
Multithreading is beneficial in scenarios that involve:
- Independent tasks that can be executed in parallel.
- Long-running, blocking operations that would otherwise impede responsiveness.
- Resource-intensive computations that can be broken down into smaller tasks.
Performance Considerations
While multithreading can boost performance, it’s not always the go-to solution. Analyze whether the overhead of thread management would outweigh the expected performance gain, particularly for lightweight operations.
Testing Multithreaded Applications
Debugging and testing multithreaded applications can be tricky due to non-deterministic behavior. Utilize specialized tools such as Valgrind and ThreadSanitizer for detecting race conditions and other concurrency issues.
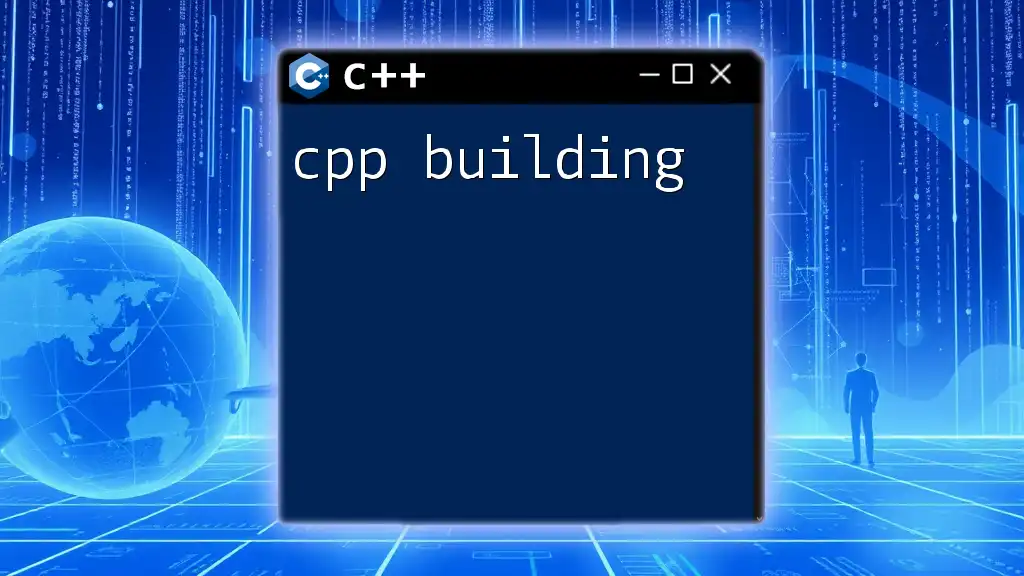
Conclusion
C++ multithreading leverages the powerful capabilities of concurrent programming to enhance application performance and user experience. By understanding the basics, leveraging advanced techniques, recognizing common challenges, and following best practices, you can harness the full potential of multithreading in your C++ applications.
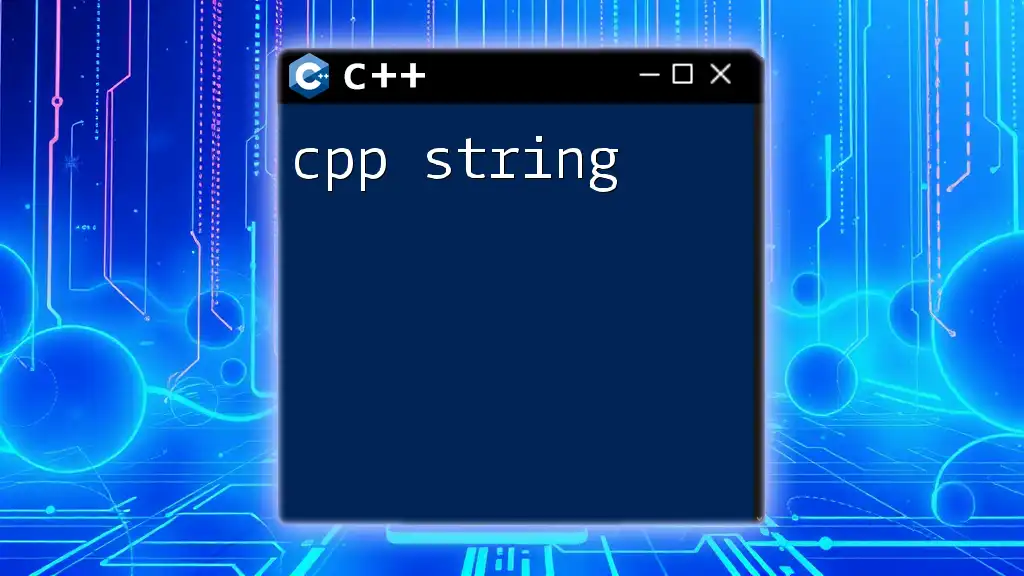
Call to Action
Engage with our community for more resources and tutorials on mastering C++ commands, including comprehensive guides on multithreading and beyond. Expand your programming skills and stay updated with the latest in the C++ world!