In C++, building a map involves using the `std::map` container from the Standard Template Library (STL) to create a collection of key-value pairs that can be efficiently accessed.
Here’s a simple example:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> ageMap;
ageMap["Alice"] = 30;
ageMap["Bob"] = 25;
for (const auto& pair : ageMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
return 0;
}
What is a Map?
In C++, a map is an associative container that stores elements in key-value pairs. Each key is unique within the map, allowing for efficient data retrieval and management. Maps are a powerful tool in data structure design, as they enable the organization and access of data through distinct identifiers, or keys. This concept is fundamental to many programming tasks, where finding specific values efficiently can make a significant difference.
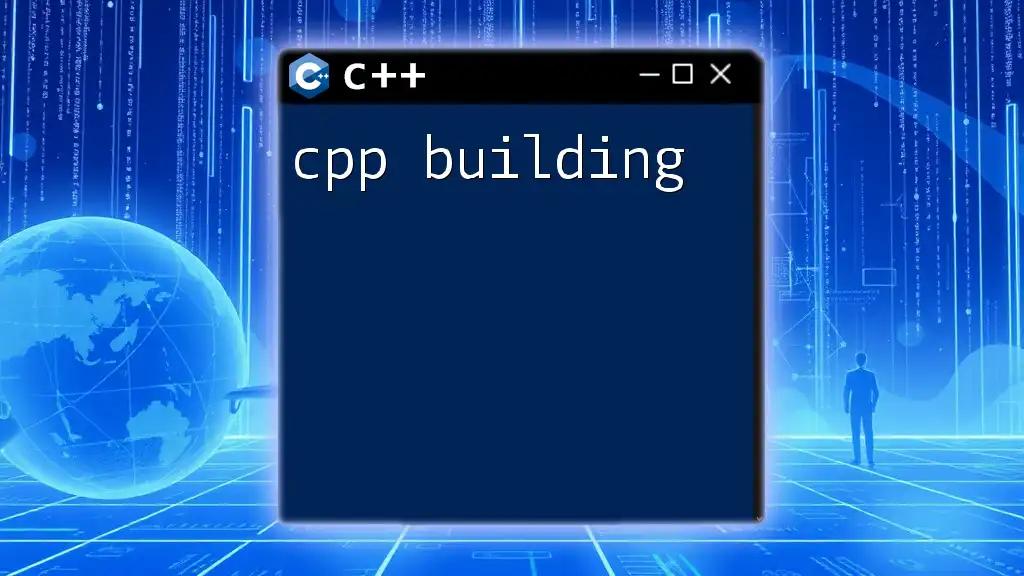
Key Features of Maps
Maps boast several important characteristics:
- Key-Value Pair Storage: Each entry in a map consists of a key and a value. The key is used to identify the location of the value in the map, allowing fast access.
- Ordered vs Unordered Maps: Ordered maps (like `std::map`) maintain their elements in a specific order based on the keys. In contrast, unordered maps (like `std::unordered_map`) do not maintain order but provide faster access to elements based on hashing.
- Use Cases in Real-World Applications: Maps are extensively used across various applications, including database management, caching mechanisms, and even certain algorithm designs that require frequent access to associated data.
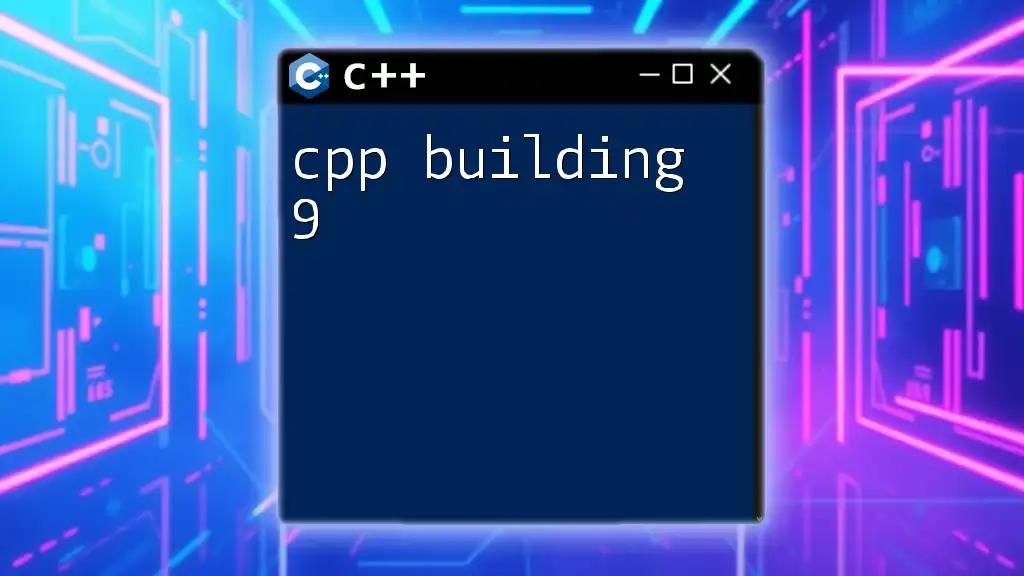
Declaring and Initializing a Map
To work with maps in C++, the first step is to declare and initialize them. The syntax for declaring a map is straightforward. Below is an example of how to declare a map, where the keys are of type `std::string` and the values are of type `int`:
#include <iostream>
#include <map>
int main() {
std::map<std::string, int> myMap; // Declare a map
myMap["Apple"] = 1; // Initialize the map with a key-value pair
myMap["Banana"] = 2; // Another key-value pair
return 0;
}
Initially, we have defined a map called `myMap`, which associates fruit names with their respective quantities. This syntax is foundational for creating a map and serves as the groundwork for performing various operations on it.
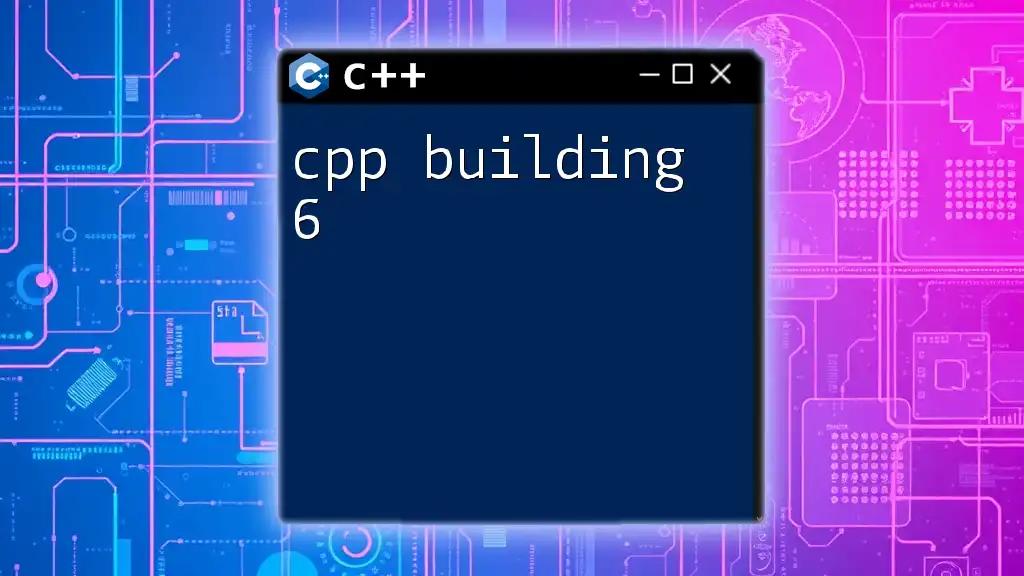
Different Types of Maps
C++ offers different types of maps, primarily ordered maps (`std::map`) and unordered maps (`std::unordered_map`).
Ordered Map (`std::map`)
The `std::map` container stores elements in a specific order based on the keys. This ordering is generally determined by a strict weak ordering criterion, which means that keys will be sorted as they are added, allowing for efficient range queries and sorted operations. Such maps are immensely useful when the order of data matters.
Unordered Map (`std::unordered_map`)
Unlike `std::map`, the `std::unordered_map` uses a hash table to store data, which allows for faster data access times but does not maintain any specific order of keys. This makes unordered maps suitable for scenarios needing quick searches or insertions without regard to the order of elements.
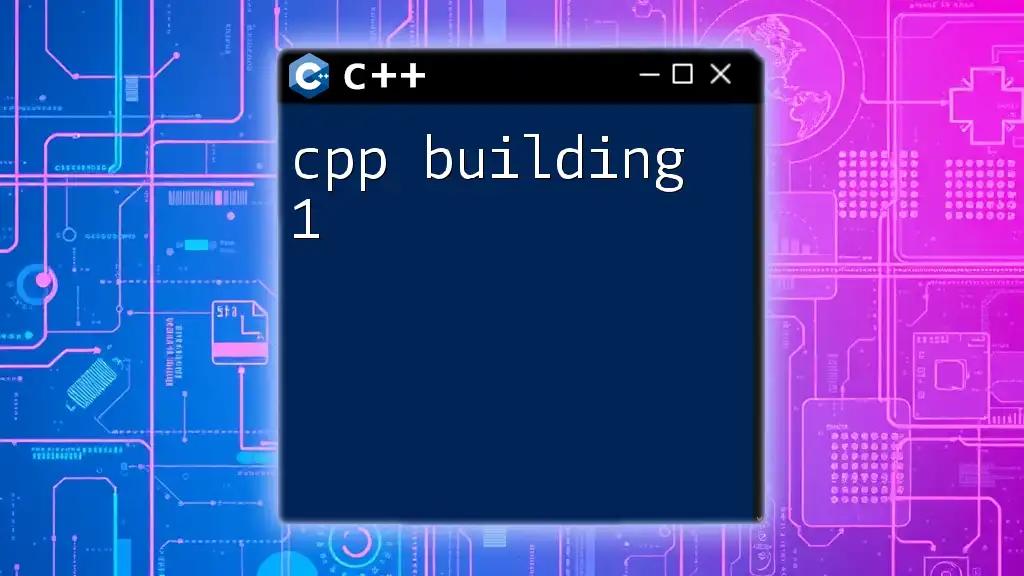
Inserting Elements into a Map
Inserting elements into a map is straightforward. The `insert` method can be employed, as seen in the following example:
myMap.insert({"Cherry", 3}); // Using the insert function
You can also directly assign values to keys using the square bracket notation, as shown earlier. It's important to note that if the key already exists in the map, assigning a new value will simply overwrite the existing value, which is something to be cautious about.
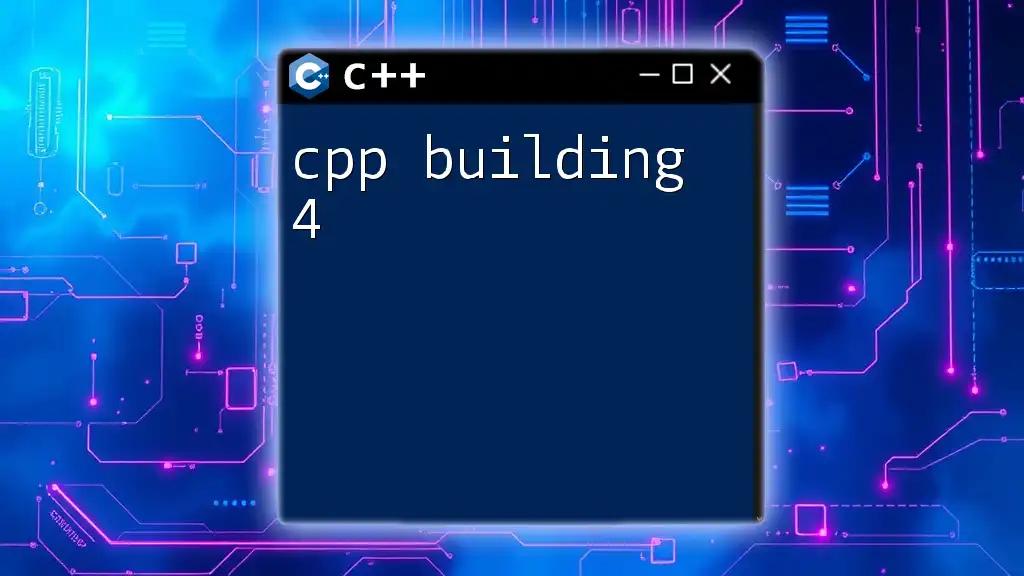
Accessing Elements in a Map
Retrieving values from a map is done using the key associated with the value. The following code snippet demonstrates how to access a specific value:
std::cout << "Value for Banana: " << myMap["Banana"] << std::endl;
This command will output the value associated with the key "Banana". If the specified key does not exist, the map will automatically insert a default value for that key, which can be an implicit result you may not want, leading us to use the `find` function instead:
if (myMap.find("Orange") != myMap.end()) {
std::cout << "Found Orange!" << std::endl;
} else {
std::cout << "Orange not found." << std::endl;
}
This usage helps in avoiding unintended insertions when retrieving values.
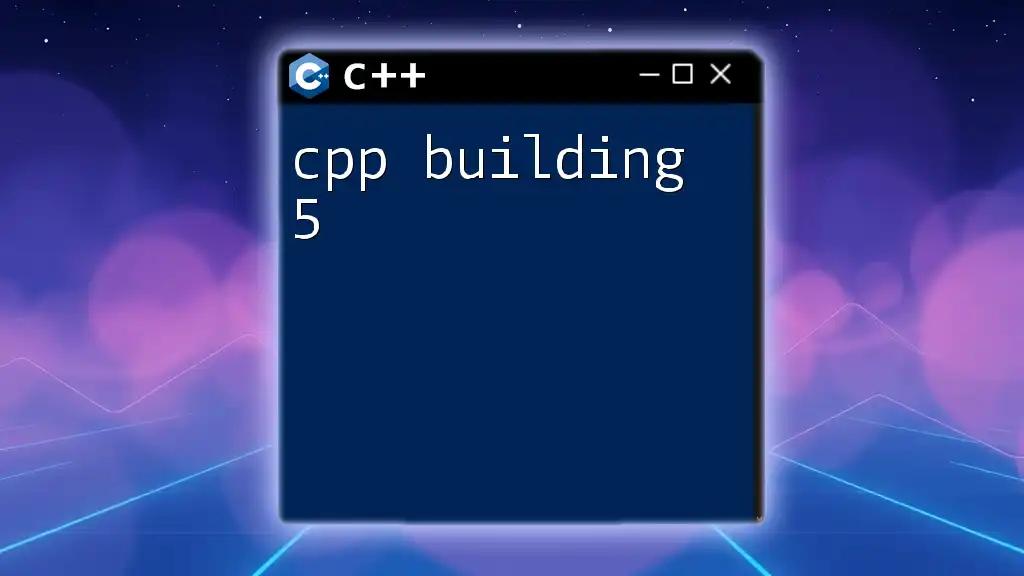
Deleting Elements from a Map
Removing an element from a map can be efficiently done using the `erase` method. The following snippet shows how to delete an entry:
myMap.erase("Apple"); // Removes the key-value pair for Apple
When an entry is erased, it is essential to remember that the memory allocated for that entry is freed. This can prevent memory leaks, especially when dealing with larger datasets or complex data structures housed in maps.
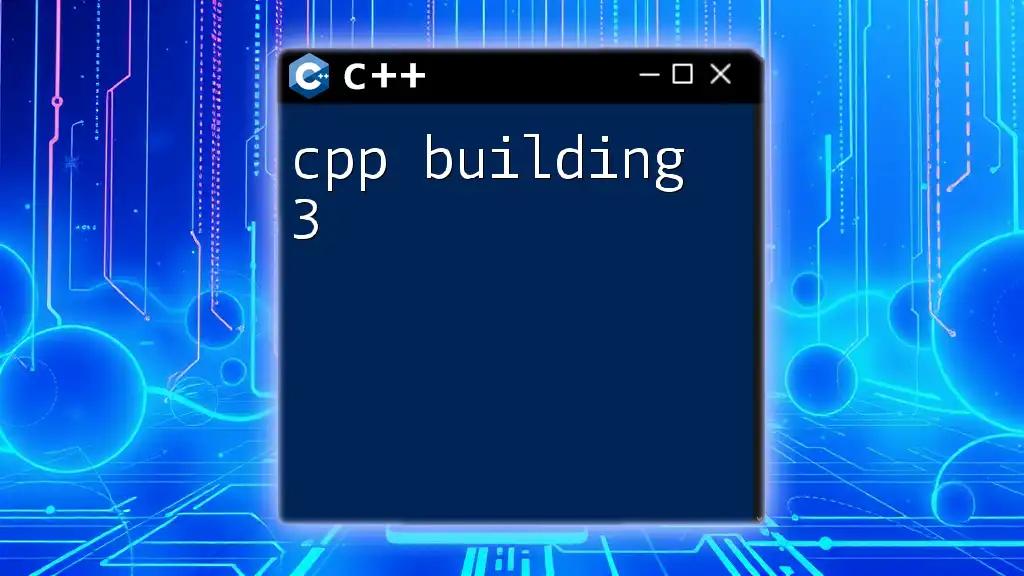
Iterating Through Maps
Iteration over a map allows you to access every key-value pair directly. You can use ** range-based for loops** to achieve this as follows:
for (const auto& pair : myMap) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
This loop will print each fruit and its associated quantity, showcasing how simple it is to traverse a collection of data within a map.
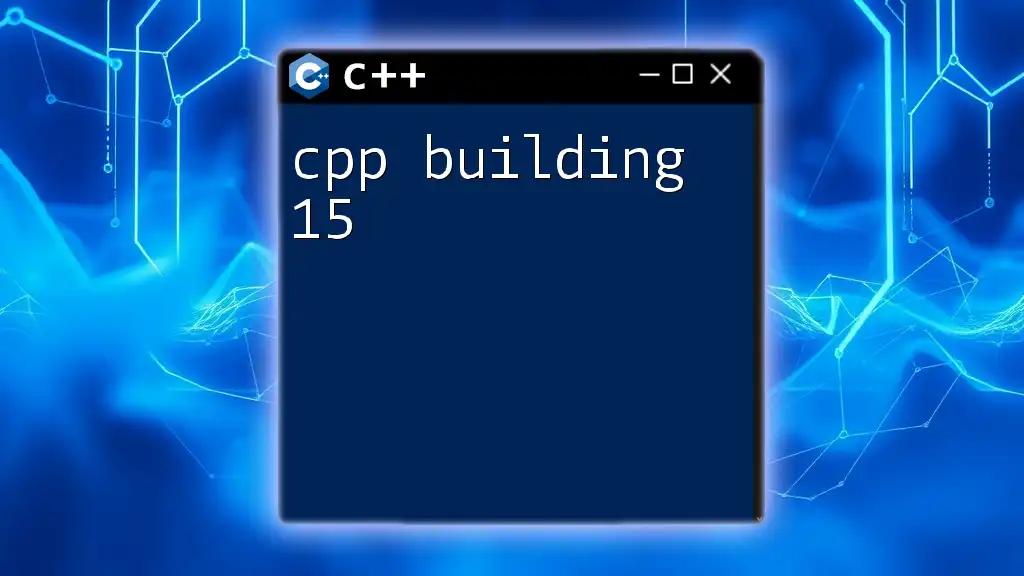
Searching for Elements
Searching for elements in a map is a common operation, and the efficiency of this task varies between `std::map` and `std::unordered_map`. In a `std::map`, the search time complexity is O(log n) due to the underlying tree structure, whereas in `std::unordered_map`, it is O(1) on average due to hashing.

Sorting Maps
While `std::map` maintains order automatically, you may find it useful to sort elements on demand based on certain criteria. For an unordered map, you can extract elements into a vector and then sort it:
std::vector<std::pair<std::string, int>> sortedMap(myMap.begin(), myMap.end());
std::sort(sortedMap.begin(), sortedMap.end(), [](const auto& a, const auto& b) {
return a.second < b.second; // Sort by value
});
This code demonstrates how to sort the entries of an unordered map based on the values.
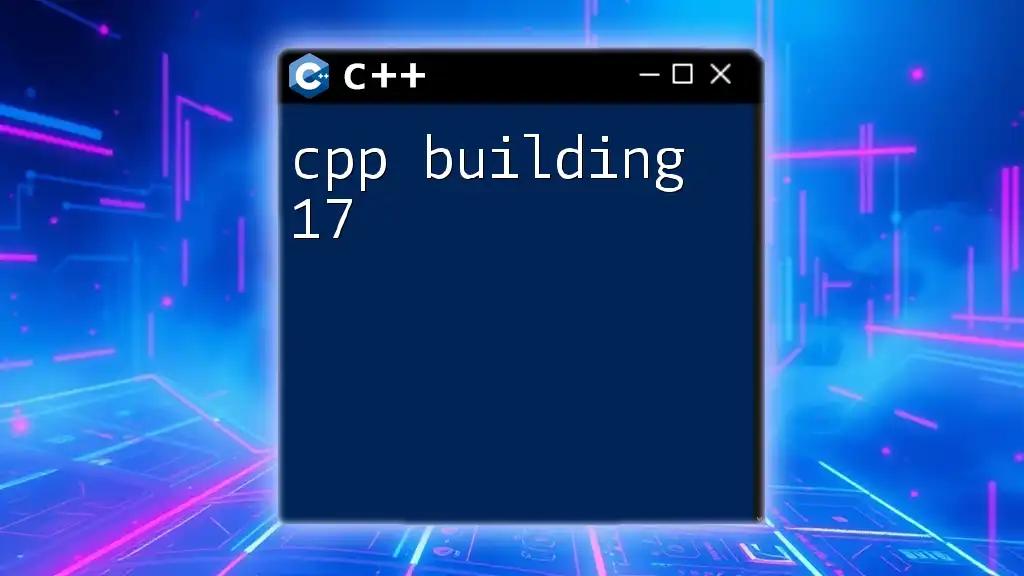
Applications in Algorithm Design
Maps play a crucial role in algorithm design, especially in cases where data needs to be categorized and accessed efficiently. For instance, when implementing algorithms like Dijkstra’s or Kruskal’s, maps can be used to maintain vertex relationships or to count occurrences of certain values.
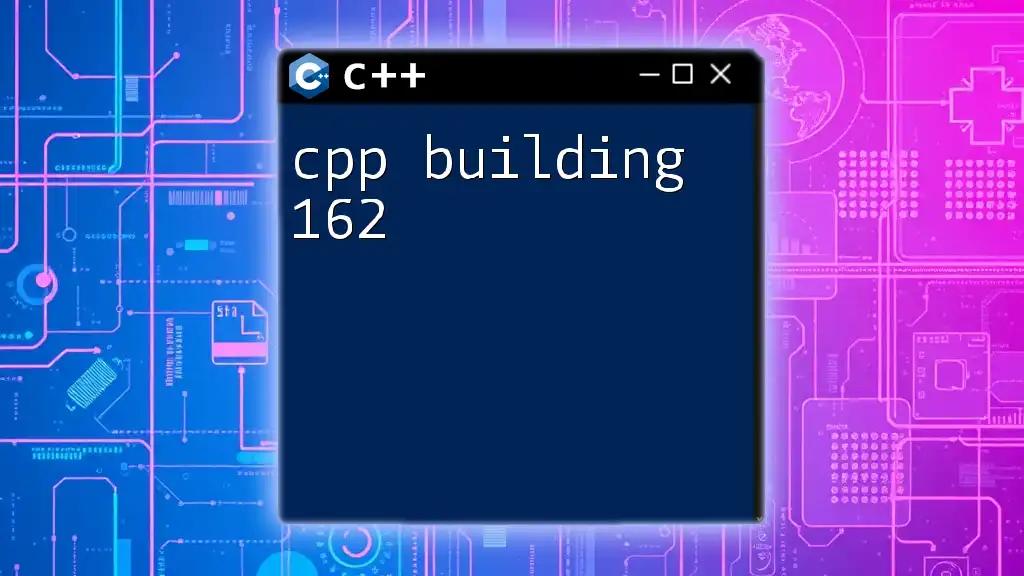
Common Challenges and Best Practices
Handling Collisions in Unordered Maps
While using `std::unordered_map`, understanding collision management is critical. The map relies on a hash function to transform keys into indices in a hash table. If two keys hash to the same index, it creates a collision. Common strategies to address collisions include chaining, where collisions are managed using linked lists, or open addressing, where alternative indices are used.
Best Practices for Using Maps
When using maps, it's essential to:
- Choose the right type of map based on your needs (ordered vs. unordered).
- Limit the size of keys and values to enhance performance.
- Minimize unnecessary re-allocations by reserving space when possible in unordered maps.
- Avoid using complex data types for keys unless you define a custom hash function and equality comparator.

Conclusion
In this comprehensive guide, we have covered the fundamentals and advanced features of C++ maps. You now possess a robust understanding of how to declare, initialize, and manipulate maps, the different types available, and their use cases in programming.
For practical use, it’s beneficial to try out the examples and code snippets provided. Experimenting with map operations will solidify your understanding and enhance your ability to leverage maps effectively in your coding endeavors.
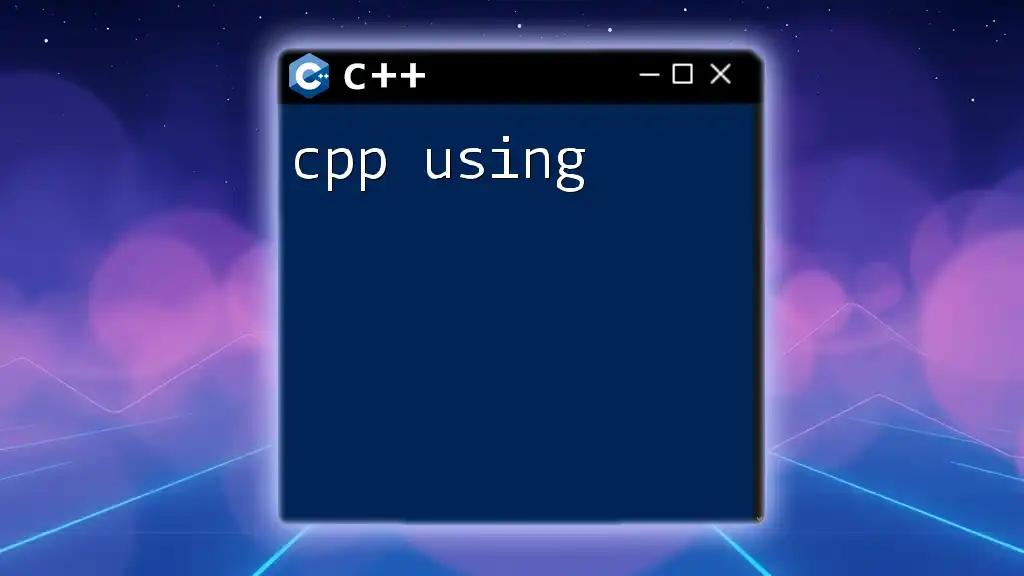
Additional Resources
For further exploration, consider looking into advanced C++ tutorials, documentation on `std::map` and `std::unordered_map`, and practical applications of maps in real-world software development scenarios.