The "cpp build" process involves using the C++ compiler to compile source code files into executable programs.
g++ -o my_program main.cpp utils.cpp
Understanding the C++ Build Cycle
What Happens During a Build?
The build process in C++ involves several crucial steps that convert human-readable source code into executable machine code. Primarily, this includes compilation — the process of translating source code into object code. Following compilation, additional steps involve linking and generating the final executable.
For example, consider the following simple C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In the build process, this source code must undergo compilation to convert it into an object file and then link it to produce an executable.
Components of a Build
A typical C++ build comprises several key components:
-
Source Files: These files carry the actual C++ code that you write. They usually have a `.cpp` extension.
-
Header Files: These files define classes, functions, and variables that can be used across multiple source files. Their extensions generally end in `.h` or `.hpp`.
-
Object Files: After compilation, the code is transformed into object files (with a `.o` extension), which contain machine code but are not yet linked.
-
Executable Files: Finally, after linking the object files, the result is an executable file ready to be run on the target system.
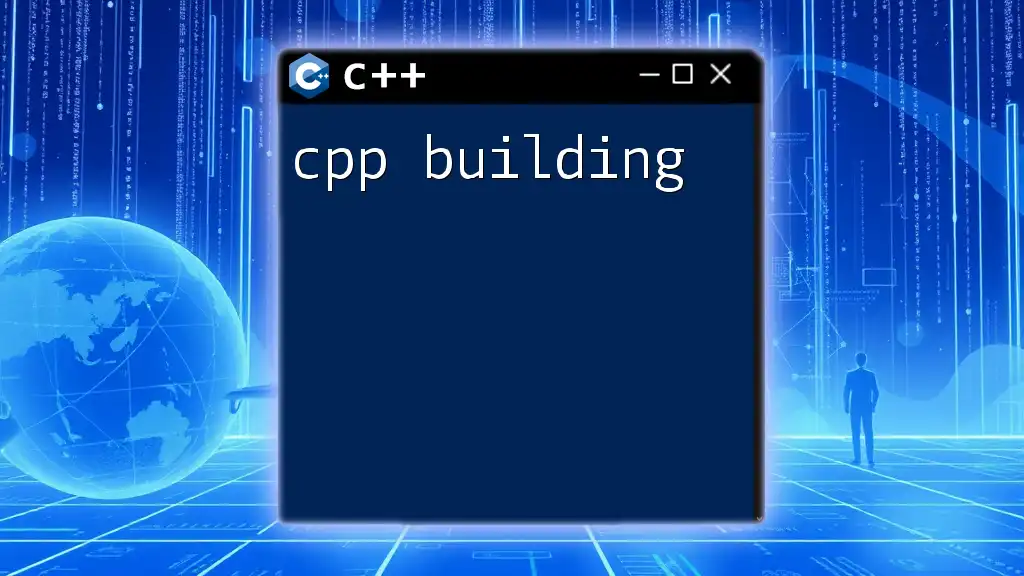
Essential C++ Build Tools
Compilers
Compiler selection is crucial for successful builds. Two of the most popular compilers are:
-
GCC (GNU Compiler Collection): This is a widely used compiler suite that supports C++ among other languages. It is known for its performance and optimization capabilities.
To compile a simple program using GCC, you might use the following command:
g++ -o hello hello.cpp
-
Clang: Another powerful compiler known for its user-friendly error messages. Clang often performs better with newer C++ standards.
A Clang compilation command looks similar:
clang++ -o hello hello.cpp
Build Systems
A build system automates the compilation process. Two common build systems in the C++ ecosystem are:
-
Make: Make utilizes Makefiles to describe how the program should be built.
Here’s a basic example of a Makefile:
all: hello hello: hello.o g++ -o hello hello.o hello.o: hello.cpp g++ -c hello.cpp clean: rm -f hello hello.o
In this Makefile, the target `hello` depends on the object file `hello.o`, which is built from `hello.cpp`.
-
CMake: CMake is a more modern tool that allows for greater cross-platform support and is preferred in larger projects.
An example `CMakeLists.txt` would look like this:
cmake_minimum_required(VERSION 3.10) project(HelloWorld) add_executable(hello hello.cpp)
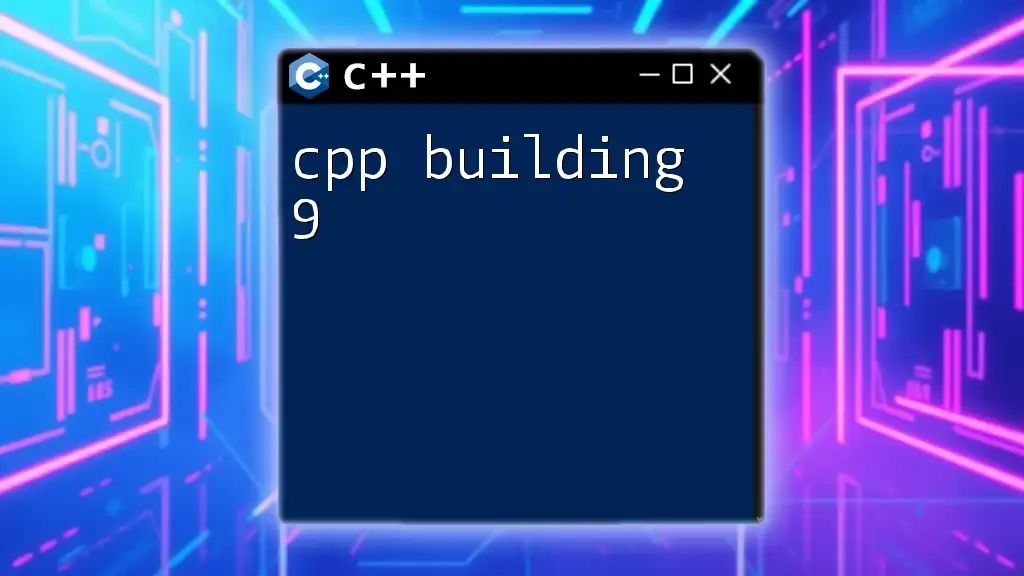
Building Process in Different Environments
Building on Windows
When building on Windows, various tools are available such as Visual Studio and MinGW. Setting up the environment with MinGW involves downloading and adding it to your system path. You can compile code in the command prompt with:
g++ -o hello hello.cpp
Building on Linux
On Linux, building is generally done through the terminal. You may need to install the relevant packages via your package manager (e.g., `apt`, `yum`).
To execute a complete build and run a program, you might execute:
g++ -o hello hello.cpp && ./hello
Building on macOS
Building on macOS follows a similar pattern to Linux. You might use Xcode and its command-line tools. Installing these via:
xcode-select --install
Once installed, you can compile and run as you'd do in a Linux environment.
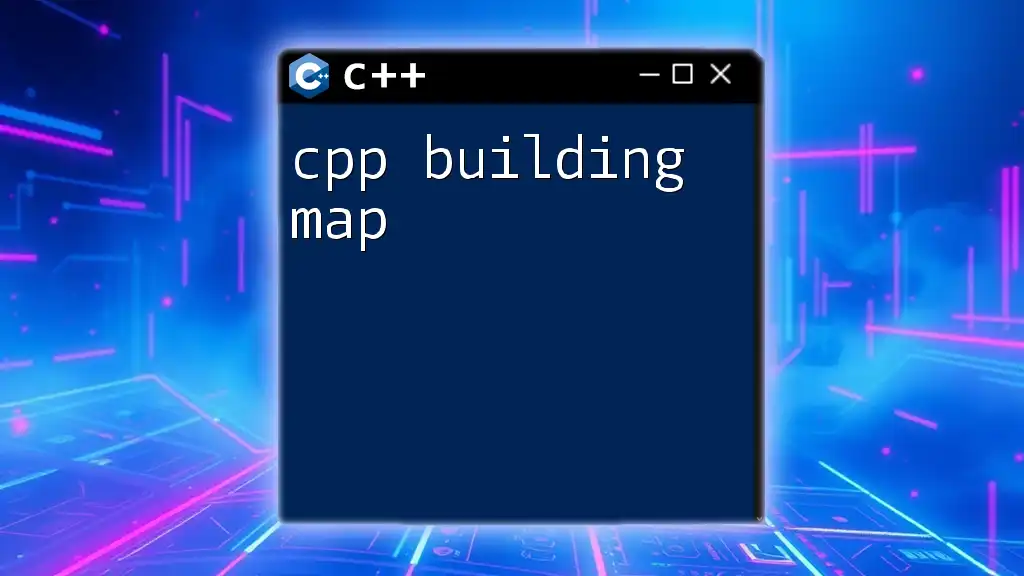
Debugging Builds
Common Build Errors
Not every build goes as planned. Some common issues you might face include:
-
Syntax Errors: These are the easiest to diagnose, usually indicating you’ve made a typographical error in your code.
For example:
#include <iostream> int main() std::cout << "Hello, World!" << std::endl; return 0; }
The lack of braces will lead to a syntax error.
-
Linker Errors: These occur when the compiler can’t find the definitions for declared functions or variables.
In a case where you may have forgotten to include a source file during linkage, you might see an error indicating that a function is undefined.
Using Debugging Tools
GDB (GNU Debugger) is a powerful command-line tool available for debugging C++ programs.
To use it:
-
Compile your program with debug symbols:
g++ -g -o hello hello.cpp
-
Start a debugging session with:
gdb hello
Within GDB, you can set breakpoints, inspect variables, and step through your code.
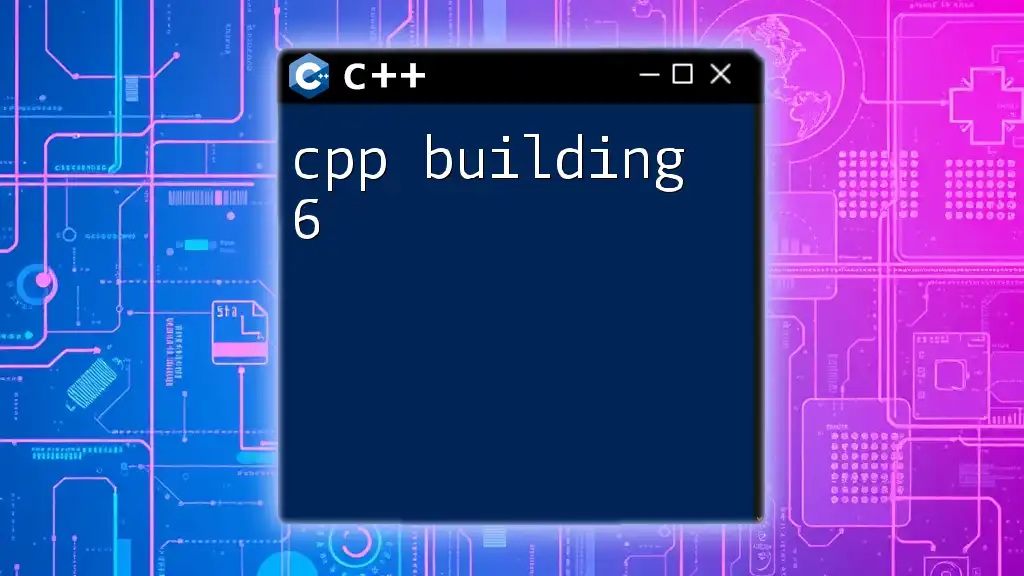
Best Practices for Effective Builds
Code Organization
Effective code organization is crucial. Ensure that your header files are separate from your source files, and logically group related classes and functions. This enhances readability and maintainability.
Incremental Builds
Understanding incremental builds can save you time during development. An incremental build recompiles only files that have changed, rather than recompiling everything. This speeds up the build process significantly.
Continuous Integration (CI)
As projects grow, employing Continuous Integration (CI) becomes vital. CI automates the testing and building of your C++ applications, notifying you of errors early.
Popular tools for CI include GitHub Actions and Travis CI, allowing you to set up workflows to test and build your code automatically upon every commit, enhancing code quality.
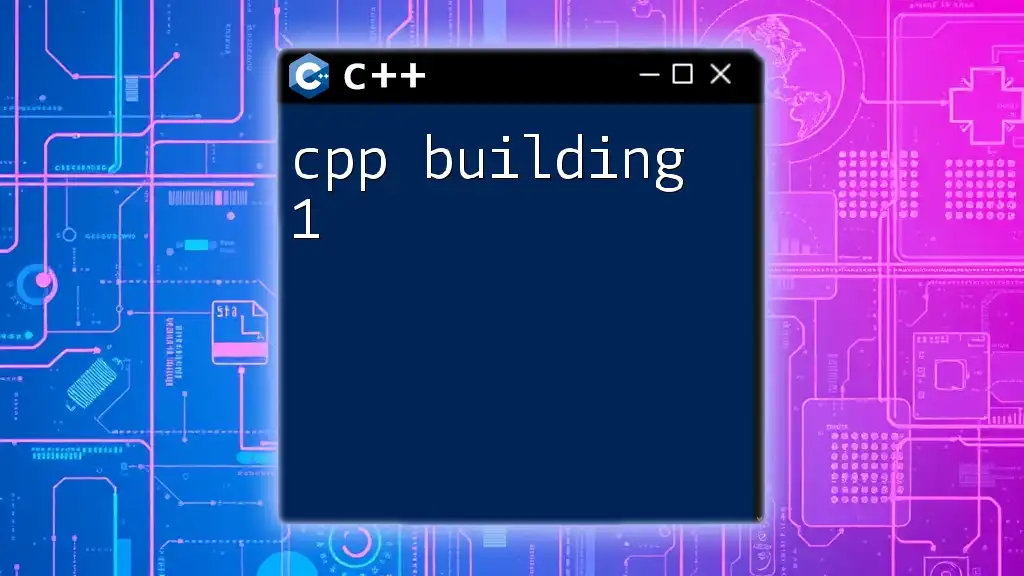
Conclusion
Mastering the C++ build process is fundamental for any C++ developer. Whether you are using GCC, Clang, Make, or CMake, each tool offers unique advantages that cater to different project requirements. By adhering to best practices, minimizing errors, and utilizing effective debugging tools, you can streamline your development process and enhance productivity in your C++ projects.
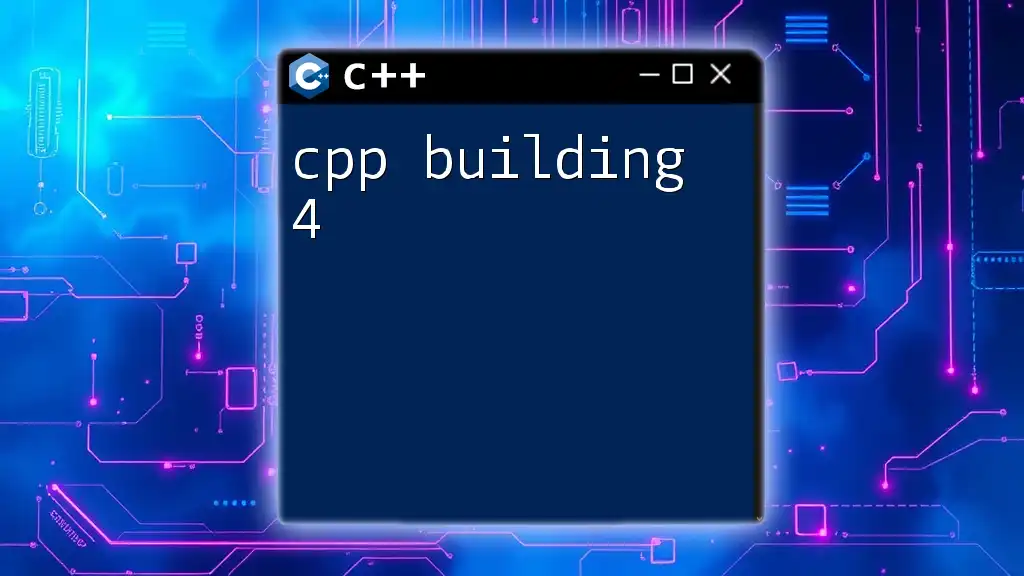
Additional Resources
To deepen your understanding, consult official documentation for tools like GCC, CMake, and GDB, and consider exploring recommended books and online courses designed for aspiring C++ developers.