"CPP building 17 refers to the process of compiling and running C++17 code, which introduces several modern features and improvements to the language."
#include <iostream>
#include <string>
int main() {
std::string name{"World"}; // Using curly braces for initialization (C++17 feature)
std::cout << "Hello, " << name << "!" << std::endl;
return 0;
}
Understanding C++17
Key Features of C++17
C++17 introduced several exciting features that enhance both the language and the standard library. Among these features are new library components, improvements in syntax, and enhanced support for programming paradigms.
-
New Standard Library Features: C++17 brought in modules, parallel algorithms, and improvements to existing libraries, enabling developers to write cleaner and more efficient code.
-
Improved Language Expressions: With the introduction of structured bindings, if constexpr, and more, coding becomes both more intuitive and safer.
-
New Keywords and Syntax Changes: Keywords like `if constexpr` allow for conditional compilation, enabling better optimization and code clarity.
Comparisons with Previous C++ Standards
When considering migrating your code to C++17, it's essential to understand the major differences from C++11 and C++14. C++17 focuses more on usability enhancement, which can significantly contribute to improved development workflows. Features like structured bindings, guaranteed copy elision, and improved lambda expressions lead to less boilerplate code and make the language more expressive.
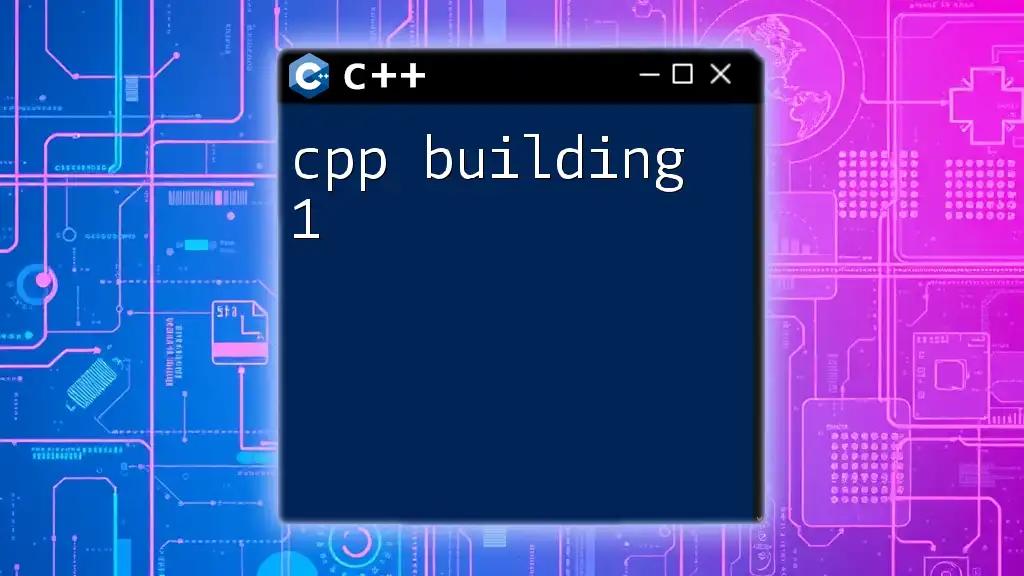
Setting Up Your Environment for C++17
Choosing the Right Compiler
To effectively work with C++17, you must choose a compatible compiler. Popular options include:
-
GCC: The GNU Compiler Collection regularly updates its support, and you can usually find the latest versions on their official website.
-
Clang: Known for its performance and diagnostics, Clang is an excellent choice for modern C++ development.
-
MSVC: Microsoft's Visual Studio Compiler offers robust support for C++17 features and is widely used for Windows development.
It’s crucial to install and update to versions that support C++17 features. Consider using package managers like `vcpkg` or `Conan` for easier management of C++ libraries.
Configuring Your Development Environment
You should configure your chosen Integrated Development Environment (IDE) to ensure it targets C++17. Commonly used IDEs include:
-
Visual Studio: Set the project properties to target C++17 under Configuration Properties > C/C++ > Language > C++ Language Standard.
-
Code::Blocks: Ensure you have the latest version and set the compiler settings to support C++17.
-
CLion: By default, CLion supports various C++ standards; just choose C++17 in your project settings.
Familiarizing yourself with these configuration steps can significantly smooth your learning curve.
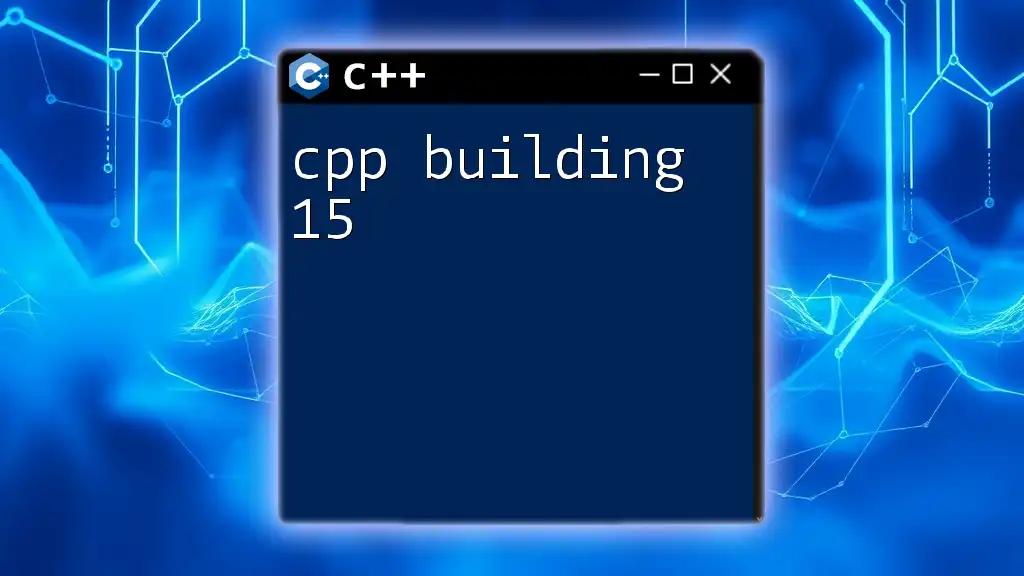
New Features in C++17
std::optional
The `std::optional` type represents an optional value that may or may not be present. It's a safer alternative to using pointers for nullable states.
Use Case: In functions where a return value may not always be present (e.g., division), you can use `std::optional` to signal that directly.
#include <optional>
#include <iostream>
std::optional<int> safe_divide(int a, int b) {
if (b == 0) return std::nullopt; // No division by zero
return a / b;
}
int main() {
auto result = safe_divide(10, 0);
if (result) {
std::cout << "Result: " << *result << '\n';
} else {
std::cout << "Division by zero error.\n";
}
}
In this code, you can see that `safe_divide` safely handles the division, returning `std::nullopt` when the divisor is zero. This approach enhances your code's safety and clarity.
std::variant
`std::variant` is a type-safe union that can hold one of several types. It provides a way to define variables that can represent different types without sacrificing type safety.
Use Case: This feature is valuable when you want to return different types from a function based on conditions.
#include <variant>
#include <iostream>
std::variant<int, std::string> get_value(bool condition) {
if (condition)
return 42;
return "Hello, C++17!";
}
int main() {
auto val = get_value(false);
std::visit([](auto&& arg){ std::cout << arg << '\n'; }, val);
}
Here, `get_value` returns either an `int` or a `std::string` based on the boolean input. The `std::visit` function handles displaying the contained value appropriately.
std::any
The `std::any` type provides a way to store any type of value, providing a flexible data storage mechanism but at the cost of type safety.
Use Case: Use it when the type of data cannot be predetermined.
#include <any>
#include <iostream>
int main() {
std::any my_var = 10; // Holds an integer
std::cout << std::any_cast<int>(my_var) << std::endl;
my_var = std::string("Hello, C++17!");
std::cout << std::any_cast<std::string>(my_var) << std::endl;
}
The ability to switch types in `std::any` is flexible, but you need to handle `std::bad_any_cast` exceptions for type safety.
New Features in the Standard Library
Filesystem Library
C++17 introduced a standardized filesystem library, enabling operations related to file and directory manipulation directly within the standard library.
Example: Here's how you can iterate over files in a directory.
#include <filesystem>
#include <iostream>
int main() {
for (const auto& entry : std::filesystem::directory_iterator(".")) {
std::cout << entry.path() << std::endl;
}
}
This example lists all files in the current directory, showcasing how C++17 simplifies filesystem operations.
Parallel Algorithms
C++17 introduced parallel algorithms, greatly improving performance in data processing. By using execution policies, you can easily switch between sequential and parallel processing.
Example: Here is a basic illustration using `std::for_each`:
#include <algorithm>
#include <execution>
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(std::execution::par, numbers.begin(), numbers.end(), [](int& n){ n *= 2; });
for (const auto& n : numbers) {
std::cout << n << " ";
}
}
In this case, `std::for_each` runs in parallel, allowing for faster computations when working with large datasets.
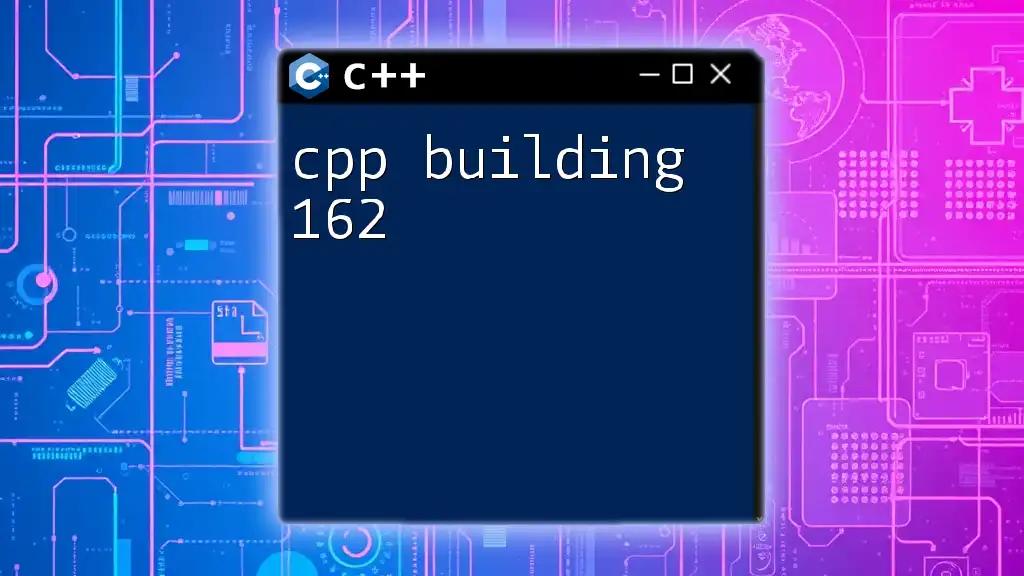
Best Practices for Using C++17
Writing Clean and Efficient Code
Utilizing C++17 features encourages developers to write cleaner, more maintainable code. Focus on minimizing code duplication, using appropriate standard library features like `std::optional` where necessary, and leveraging structured bindings for better variable declarations.
-
Organize Your Code: Proper use of headers and source files keeps your code structured.
-
Readability and Maintainability: Your code should be easy to read and understand, especially for others who may work on it in the future.
Leveraging C++17 Features Effectively
Understanding when to use specific C++17 features is crucial.
-
std::optional is perfect for situations where a function may not return a valid value.
-
std::variant is beneficial for functions that can return different types based on conditions.
-
Deploying parallel algorithms allows for significant performance improvements on large-scale data processing.
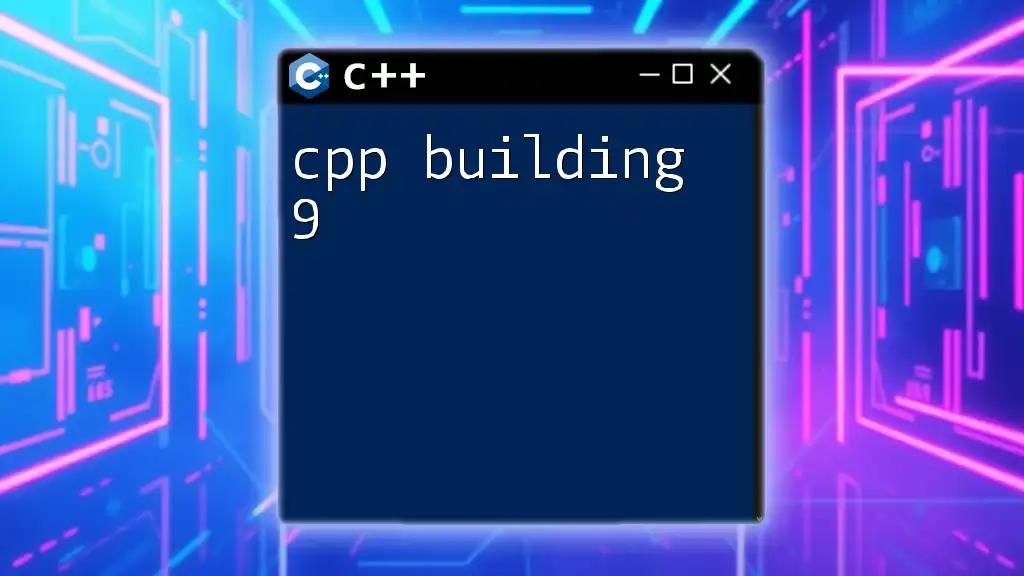
Common Pitfalls to Avoid
Mistakes with New Syntax
Transitioning to C++17 may lead to confusion with new syntax. Familiarize yourself with:
-
Structured Bindings: Ensure variable declarations using this syntax are correctly done to avoid compilation errors.
-
std::visit: Using `std::visit` incorrectly can lead to compile-time errors if the visitor does not handle all types within the `std::variant`.
Understanding Compiler Support
Before diving into C++17, it's crucial to verify your chosen compiler supports all the features you plan to use. Each compiler's implementation may vary, so check compatibility and feature availability through official documentation.
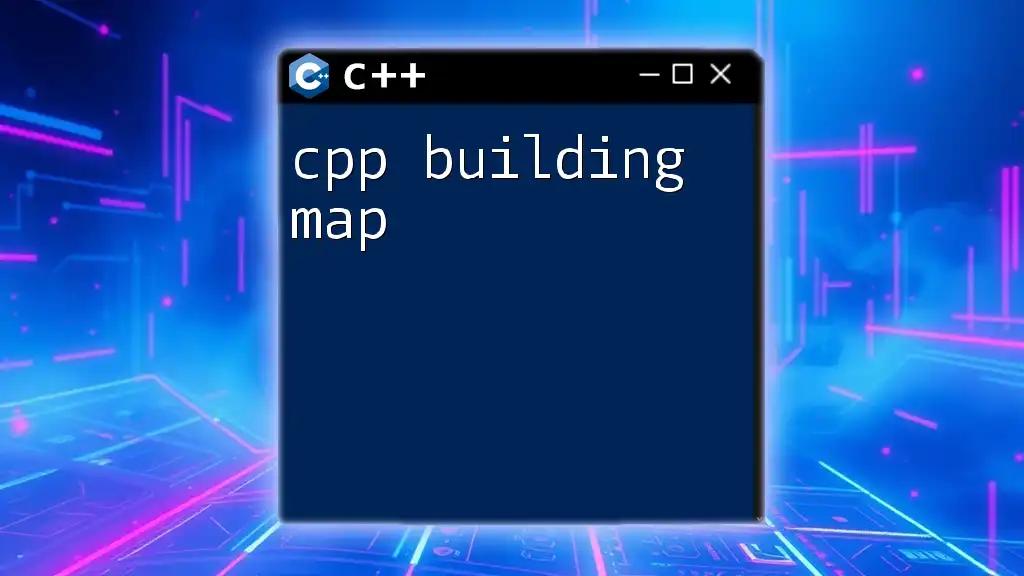
Conclusion
Understanding and effectively utilizing C++17 features through cpp building 17 enhances your coding practices, improving both efficiency and clarity in your programs. As you delve deeper, remember to practice consistently and explore advanced concepts to further enrich your C++ toolkit.
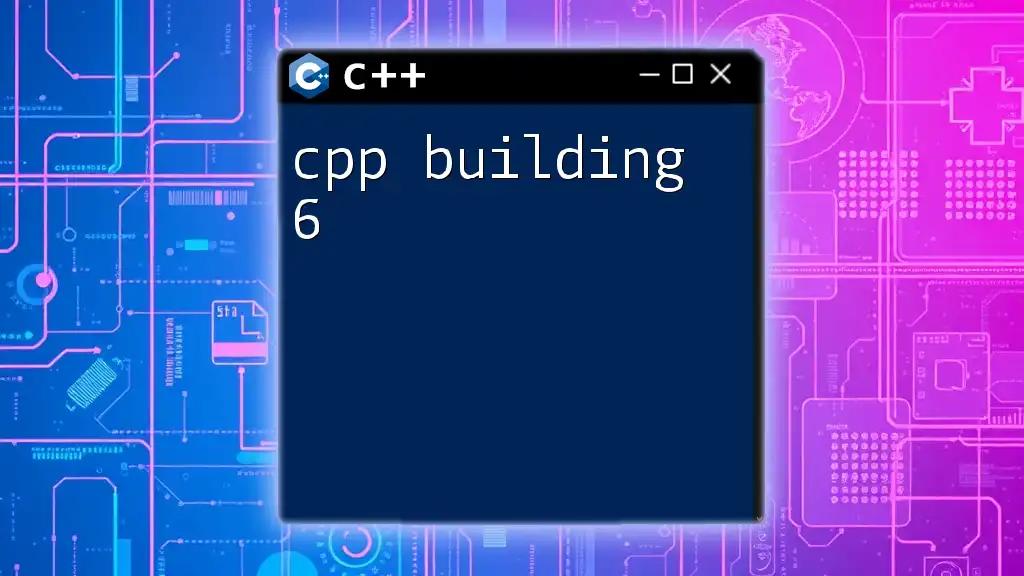
Additional Resources
Explore official C++ documentation, recommended books, online courses, and community forums to continue your learning journey and deepen your grasp of C++17 and beyond. Happy coding!