In "cpp building 1," we will explore the fundamental process of compiling and executing a simple C++ program using the g++ compiler.
Here's a basic example of a C++ program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile and run the program, use the following commands in your terminal:
g++ hello.cpp -o hello
./hello
Understanding the C++ Build Process
What Does It Mean to Build C++ Code?
In software development, building refers to the process of converting human-readable source code into machine-readable executable files. For C++, building encompasses not only the compilation of source files but also linking object files to create a runnable program.
When you write a C++ program, it typically resides in files with a `.cpp` extension. The building process transforms these source files into object code (.o or .obj files), and finally into an executable that your operating system can run.
Key Terms in the Build Process
Understanding the terminology related to the build process is crucial for grasping how C++ compilation works:
-
Compiler: The tool responsible for transforming C++ code into object code. Popular C++ compilers include g++ (GNU Compiler Collection) and MSVC (Microsoft Visual C++).
-
Linker: This tool combines one or more object files generated by the compiler into a single executable. It resolves references between files and libraries to ensure that calls to functions from other files are successfully linked.
-
Build System: A set of tools and scripts that automate the process of compiling and linking code. Examples of build systems include Make and CMake.
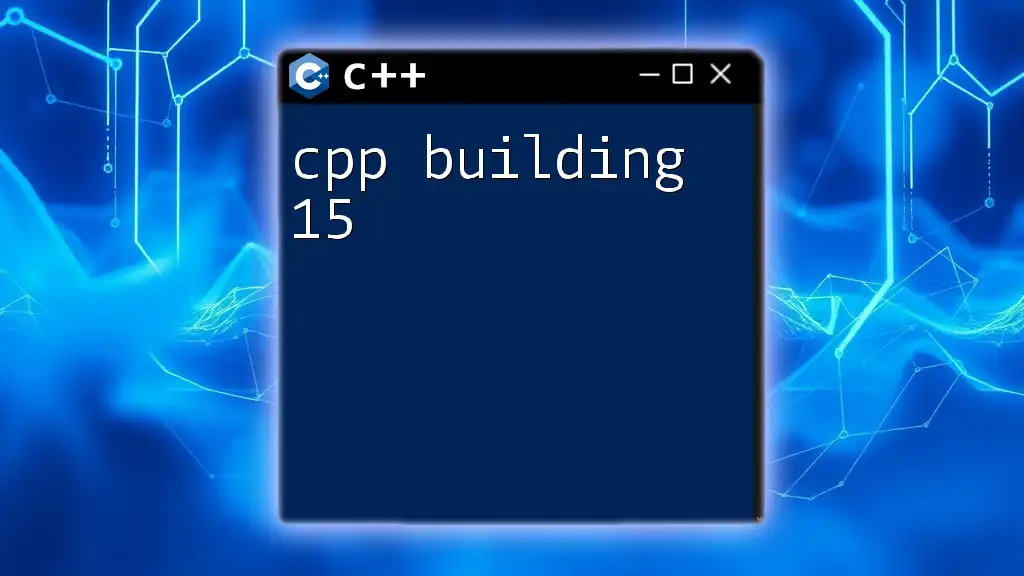
Getting Started with C++ Building
Setting Up Your Environment
To start building C++ applications, you need to set up your development environment. Here are the steps to follow:
-
Install a Compiler: Depending on your operating system, you may choose from various compilers. For instance:
- Windows: Install Visual Studio or MinGW.
- Linux: Usually, g++ is available via your package manager.
- macOS: Use Xcode, which comes with g++.
-
Choose a Text Editor or IDE: While you can use simple text editors, IDEs like Visual Studio, Code::Blocks, or CLion offer integrated tools that facilitate coding, compiling, and debugging.
Writing Your First C++ Program
Let’s write a simple C++ program to illustrate the process:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
In this basic program:
- `#include <iostream>` tells the compiler to include functionalities for input and output.
- The `main()` function is the entry point of all C++ applications.
- `std::cout` is used to print text to the console.
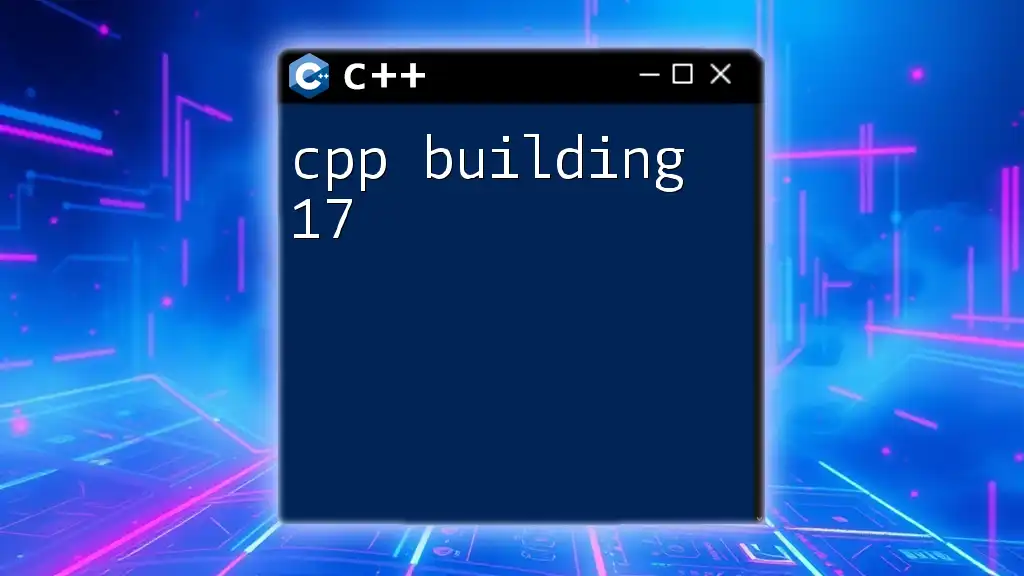
Compiling Your C++ Code
Using the Command Line to Compile
To compile your C++ program from the command line, follow these steps:
- Open your terminal or command prompt.
- Navigate to the directory where your source code resides.
- Use the following command to compile:
g++ -o hello hello.cpp
Here, `-o hello` specifies the name of the output executable (`hello`), and `hello.cpp` is the source file you want to compile.
Common Compilation Errors
When compiling, you may encounter several errors. Some common ones include:
-
Syntax Errors: These occur due to incorrect C++ syntax. The compiler will inform you of the line number and may give a hint about the issue.
-
Undefined References: If you misspell a function name or forget to include necessary headers, you will see this error during linking.
To troubleshoot, examine the error messages carefully, as they often guide you toward the solution.
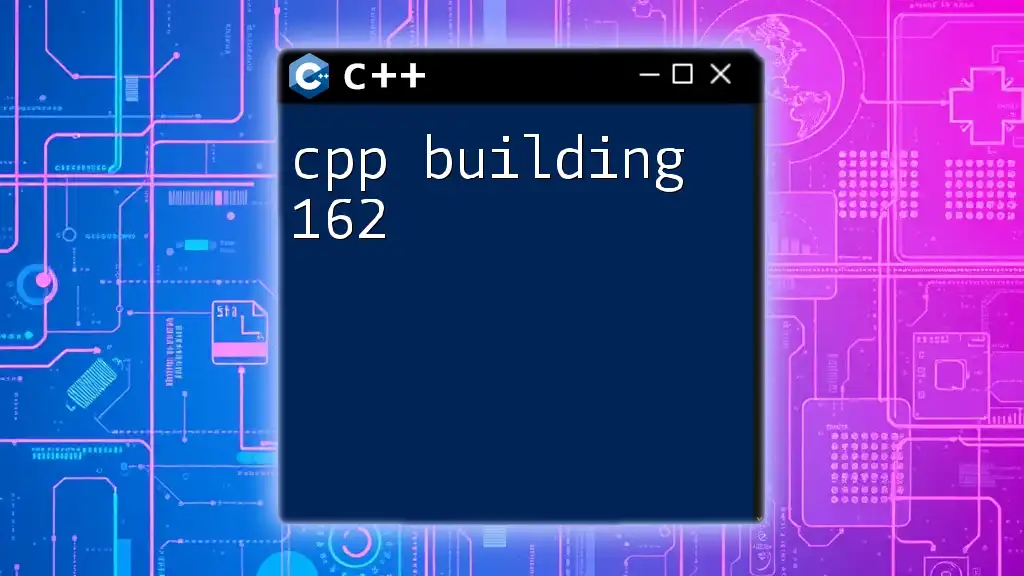
Linking: Combining Object Files
Understanding the Linking Process
After compilation, the linker combines different object files to create a full executable. This process resolves references so that the compiled code can make function calls across different files.
Consider this example, where your program consists of multiple files:
// hello.cpp
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
// main.cpp
void greet();
int main() {
greet();
return 0;
}
To compile and link these files together, use:
g++ -o hello main.cpp hello.cpp
This command tells the compiler to produce an executable named `hello` from both `main.cpp` and `hello.cpp`.
Static vs. Dynamic Linking
In C++ building, linking can be either static or dynamic.
Static Linking
In static linking, all the required libraries are combined into the executable at compile time. This makes deployment simpler since the executable contains everything it needs to run.
Dynamic Linking
Dynamic linking, on the other hand, incorporates libraries at runtime. While this reduces the size of the executable and allows sharing of common libraries, it can cause issues if the dynamic library is missing or incompatible with the executable.
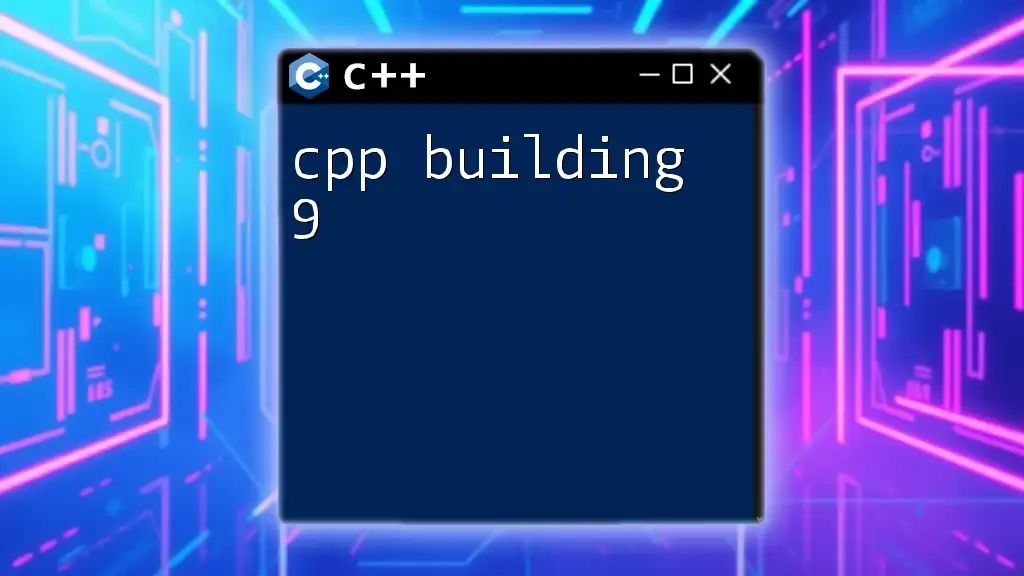
Using Build Systems
Introduction to Build Systems
Build systems are tools that automate the compilation and linking of code, which is especially helpful for larger projects. They help manage dependencies, streamline the build process, and handle the complexities of compiling multiple files.
Creating a Simple CMake Project
Installing CMake
CMake is a popular cross-platform build system. Install it from the official website or through your package manager.
Writing a CMakeLists.txt File
A `CMakeLists.txt` file guides CMake on how to build your project. Here’s a simple example for a project called "HelloWorld":
cmake_minimum_required(VERSION 3.10)
project(HelloWorld)
add_executable(hello hello.cpp main.cpp)
This file includes:
- `cmake_minimum_required`: Specifies the minimum version of CMake required.
- `project`: Defines the name of your project.
- `add_executable`: Lists the source files to be compiled into the executable `hello`.
Building with CMake
To build a project with CMake:
- Create a build directory:
mkdir build
cd build
- Run CMake to configure the project:
cmake ..
- Compile the project using:
make
This will generate the executable specified in the `CMakeLists.txt`.
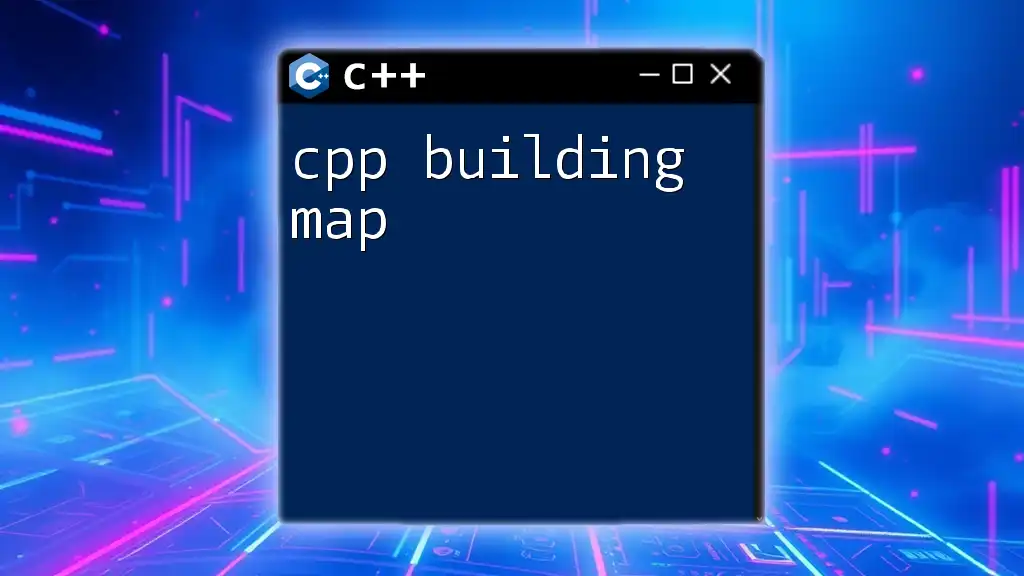
Best Practices for Building C++ Applications
Organizing Your Code for Easier Builds
Proper organization of your code can simplify the building process. Consider using:
- Separate Directories: For source code and headers.
- Consistent Naming Conventions: This helps in identifying files quickly.
Utilizing header files to declare functions can also minimize dependencies across your source files.
Using Version Control Systems
Version control, like Git, is essential for managing changes in your codebase. It allows you to track revisions, rollback changes when necessary, and collaborate with other developers efficiently. Integrating version control into your build process ensures you always have a record of changes leading up to a successful build.
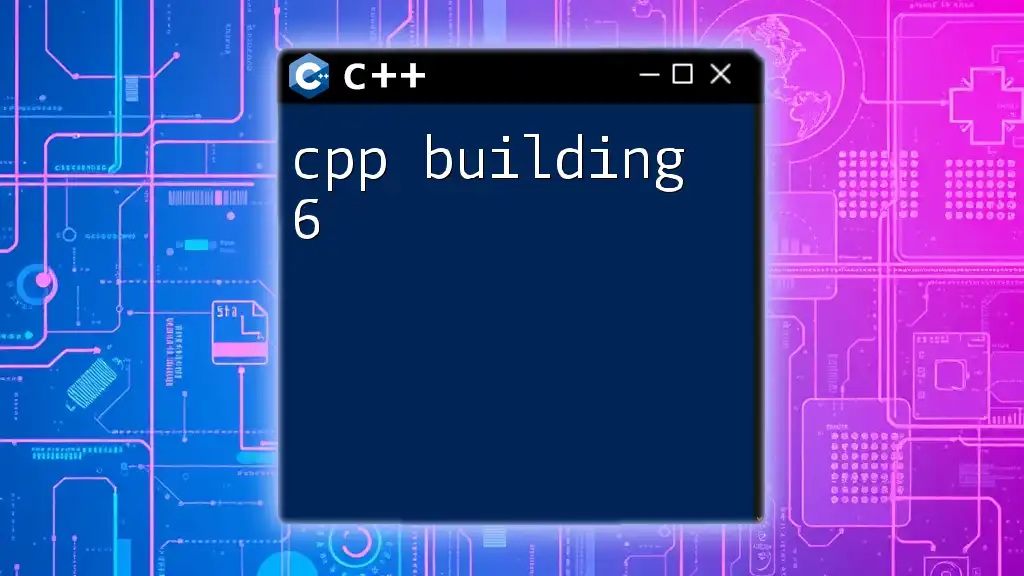
Conclusion
Understanding the C++ building process is fundamental to developing robust applications. Familiarizing yourself with compilation, linking, and build systems lays a strong foundation for your C++ programming journey. Practice building projects and experiment with various tools introduced in this guide. By doing so, you’ll gain confidence and proficiency in C++ development, paving the way for further exploration into advanced topics.
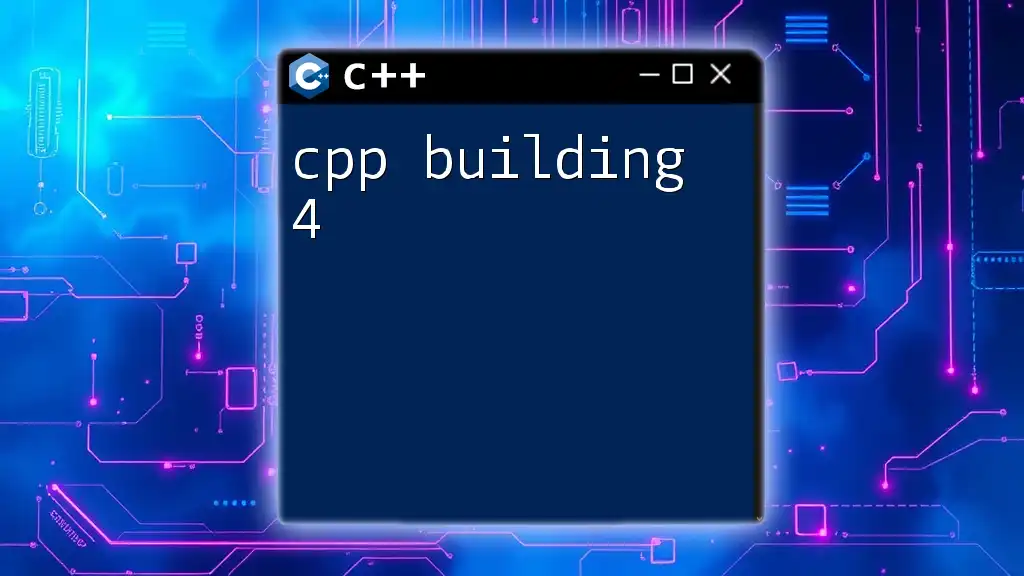
Additional Resources
Explore books, online courses, and additional documentation to reinforce your learning. Don’t hesitate to reach out with questions or share your experiences with C++ building.