Visual C++ Build Tools are a set of development tools provided by Microsoft that allow you to compile C++ applications without needing to install the full Visual Studio IDE.
Here's a simple example of using the `cl` command to compile a C++ source file:
cl /EHsc hello.cpp
This command compiles the `hello.cpp` file while enabling exception handling.
What are Visual C++ Build Tools?
Definition and Purpose
Visual C++ Build Tools are a set of tools provided by Microsoft that facilitate the development, building, and debugging of applications written in C++ using the Visual Studio environment. These tools streamline the standard processes involved in compiling source code, linking libraries, and generating executable files or other output formats. They are essential for any C++ developer who aims to build high-quality applications while maintaining efficiency in the development cycle.
Key Features
Visual C++ Build Tools offer a range of crucial features that enhance the C++ development experience:
-
Compiler Support: They include the Microsoft C++ (MSVC) compiler, which is known for its speed and reliability in converting C++ source code into object code.
-
Linker Capabilities: The tools handle linking object files and libraries effectively, enabling developers to create comprehensive applications with numerous dependencies.
-
Debugger Integration: Built-in debugging features facilitate troubleshooting and optimization, allowing developers to inspect variables, system states, and program execution paths.
-
Package Management with NuGet: This feature simplifies the process of managing external libraries and dependencies, helping developers keep their projects updated with the latest versions.
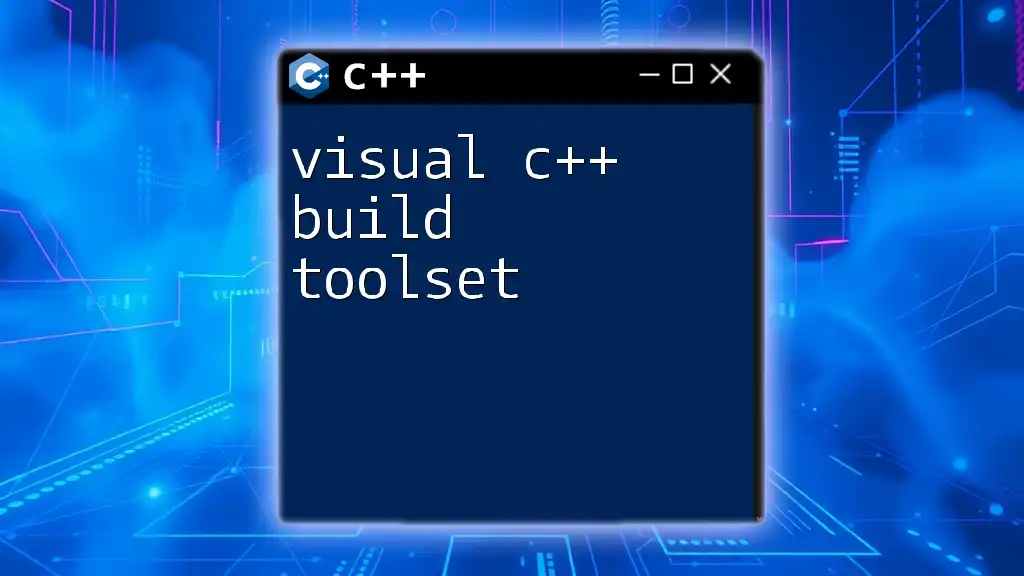
Installing Visual C++ Build Tools
System Requirements
Before installing Visual C++ Build Tools, ensure your system meets the following minimum requirements:
- Operating System: Windows 10 or later, including versions supported by the Visual Studio installation.
- Memory: At least 2 GB of RAM (4 GB or more is recommended for larger projects).
- Hard Disk Space: Minimum of 4 GB of free space (more may be needed based on the components selected).
Step-by-Step Installation Guide
Downloading Visual C++ Build Tools: To start, visit the official Microsoft Visual C++ Build Tools download page and select the appropriate installer.
Installation Process: Follow these detailed instructions:
- Run the installer and choose the "Visual C++ Build Tools" workload.
- Customize the installation by selecting the components that suit your development needs, such as the MSVC compiler, Windows SDK, or other tools.
- Finish the installation by clicking Install.
Verifying Installation: After the installation completes, open a command prompt and type `cl` to check if the compiler is accessible. You should see version information for the Microsoft C++ compiler if the installation was successful.
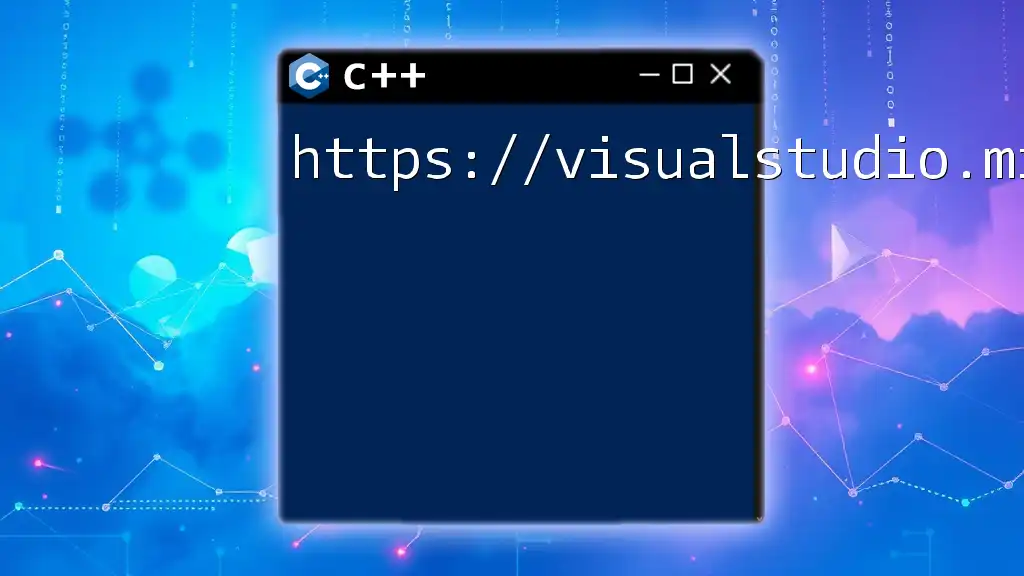
Using Visual C++ Build Tools
Creating a New Project
To create a new C++ project:
- Open Visual Studio and select File > New > Project.
- Choose C++ from the project types and select Console Application as a suitable template.
- Follow the prompts to name your project and select a location for its files.
Your project structure will typically include a source folder for `.cpp` files and potentially a `header` folder for `.h` files, establishing a clean and organized workspace.
Configuring Build Settings
Build Configuration Options
When configuring your build settings, consider the following options:
- Debug vs. Release Modes: Debug mode includes debugging information and is used during development to catch errors, while Release mode produces optimized executables for deployment.
- Set your desired architecture: Select between x86 (32-bit) and x64 (64-bit) based on your target environment.
Command-Line Options
Building a project can also be accomplished using the command line. Here's an example command for building a project named `MyProject.sln` in Release mode:
msbuild MyProject.sln /p:Configuration=Release
Using command-line options not only speeds up the build process but also offers flexibility in automating builds.
Writing and Compiling Code
Writing Code in Visual Studio
Once your project is set up, you can write your source code in the primary editor. Visual Studio provides code highlighting, IntelliSense, and other features that facilitate a productive coding experience.
Compiling Code
The compilation process converts your source code into machine-readable object files. Here’s a simple C++ code snippet demonstrating a basic program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile your code, simply click on Build > Build Solution in Visual Studio. If successful, this will generate an executable file in the specified output directory.
Linking Libraries
Static vs. Dynamic Linking
In C++, you can link libraries either statically (including all required code in the executable) or dynamically (linking to shared libraries at runtime). Choose static linking for smaller, standalone executables, and dynamic linking when you want to share libraries among different applications.
Adding Dependencies
To add external libraries to your project:
- Right-click on your project in the Solution Explorer and select Properties.
- Navigate to Configuration Properties > Linker > Input.
- Add your library files (e.g., `.lib` files) in the Additional Dependencies section.
This process helps maintain cleaner code and integrates third-party functionalities seamlessly.
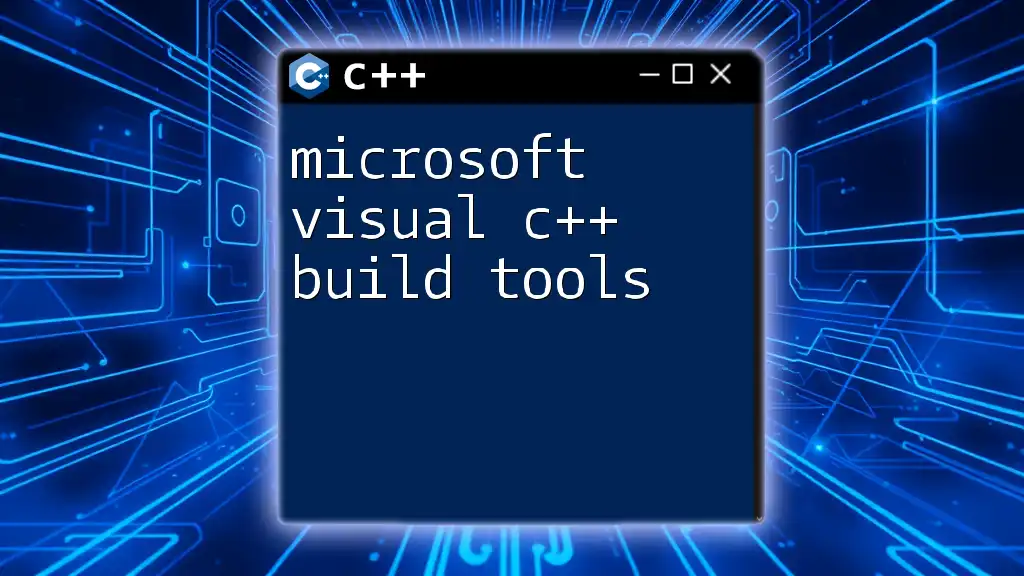
Debugging with Visual C++ Build Tools
Introduction to Debugging
Debugging is an invaluable part of software development, especially in C++. It helps identify and fix bugs, optimize code, and ensure your application runs as intended. Visual C++ Build Tools have powerful debugging functions integrated to support developers throughout the process.
Setting Breakpoints and Watching Variables
Setting breakpoints allows you to pause execution at a specific line of code, making it easier to inspect the program's state. To set a breakpoint, simply click in the left margin next to the line number in the code editor.
You can also monitor variables while debugging by adding them to the Watch Window. By right-clicking on a variable and selecting Add to Watch, you can track its value at runtime.
Using the Debugger
Once your breakpoints are in place, start debugging by clicking Debug > Start Debugging or pressing F5. You can step through your code line by line, check the call stack for function calls, and inspect variable values at different execution points. This comprehensive approach helps identify common bugs, such as logic errors and memory leaks.
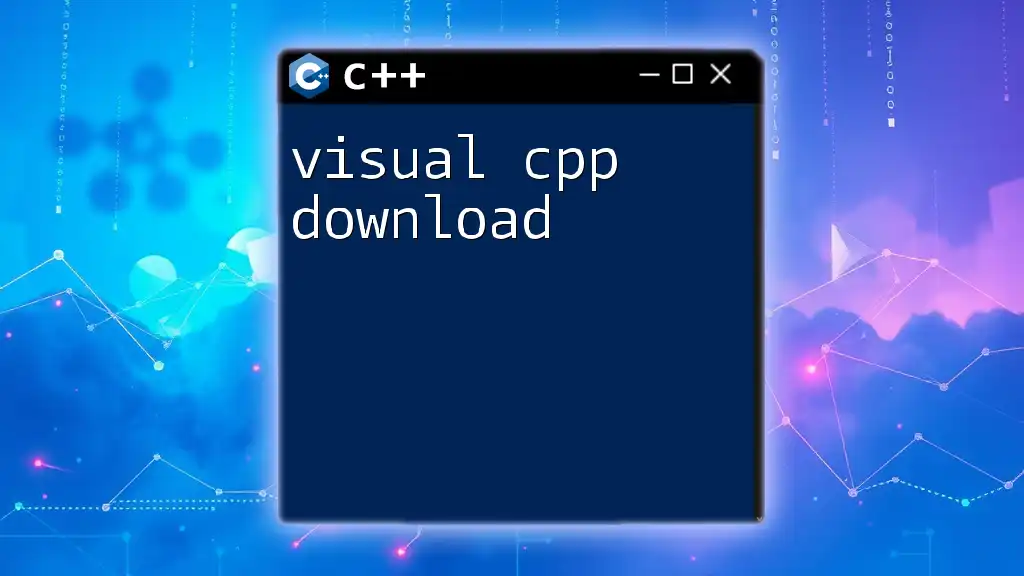
Package Management with NuGet
What is NuGet?
NuGet is a package manager designed for managing third-party libraries and dependencies in .NET projects, including C++ applications. It simplifies the process of importing and updating libraries, thereby streamlining the development workflow for complex applications.
Installing Packages using NuGet
To install a package using NuGet:
- Open the Package Manager Console found in Tools > NuGet Package Manager.
- Execute the command to install a desired package. For instance, to install the JSON library, you would type:
nuget install jsoncpp
This adds the specified package to your project, enabling you to utilize its functionalities immediately.
Updating and Managing Packages
Regularly checking for updates of the packages in use is crucial for maintaining security and performance. You can do this from the Package Manager Console by executing:
Update-Package
This command updates all installed packages to their latest versions, ensuring your codebase benefits from the latest improvements and bug fixes.
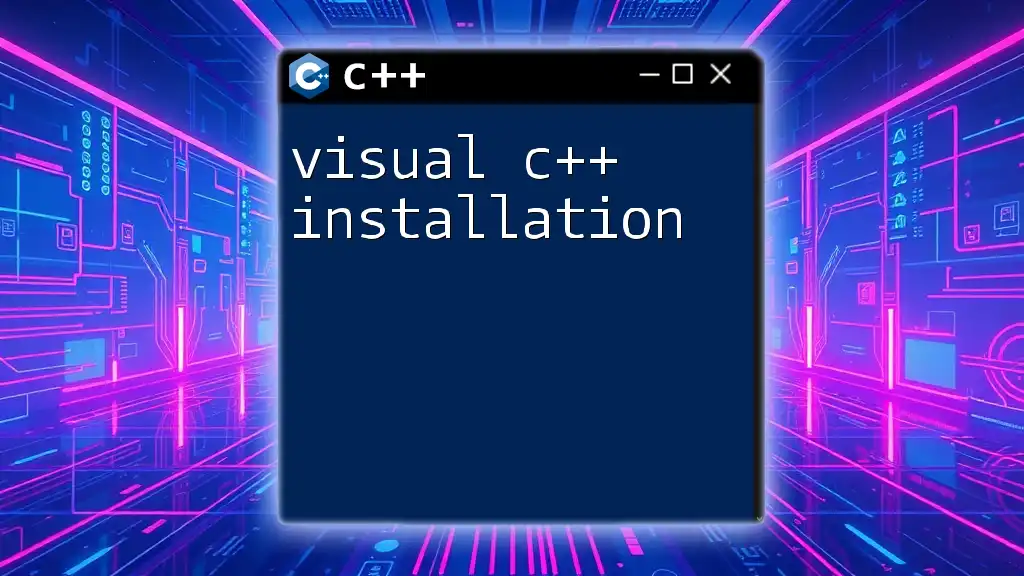
Common Issues and Solutions
Trouble with Installation
One of the most common issues during installation is failing to meet system requirements. Ensure your system has adequate hardware resources and is running a compatible version of Windows.
Build Failures
Build errors may occur due to various reasons, such as syntax errors in the code or misconfigured paths to libraries. Pay close attention to the error messages provided in the output window. Common solutions include:
- Checking for typos in code.
- Verifying that all required libraries are correctly linked.
- Ensuring that all paths are properly configured in project settings.
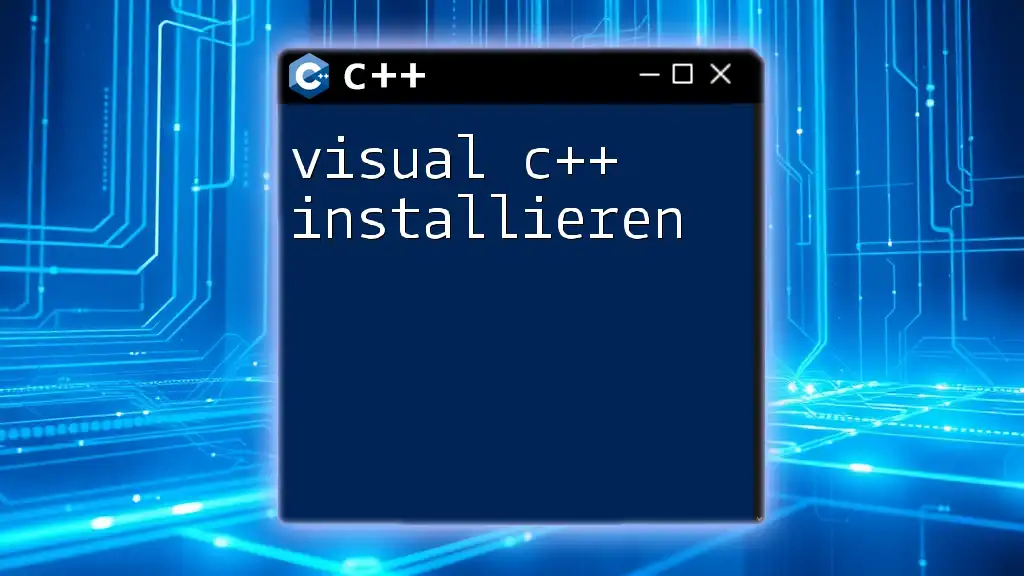
Conclusion
Visual C++ Build Tools are an essential component of C++ software development, providing invaluable support for compiling, linking, and debugging applications. By leveraging these tools, developers can greatly enhance productivity and ensure the quality of their code. We encourage you to explore the capabilities of Visual C++ Build Tools and share your experiences in your development journey.
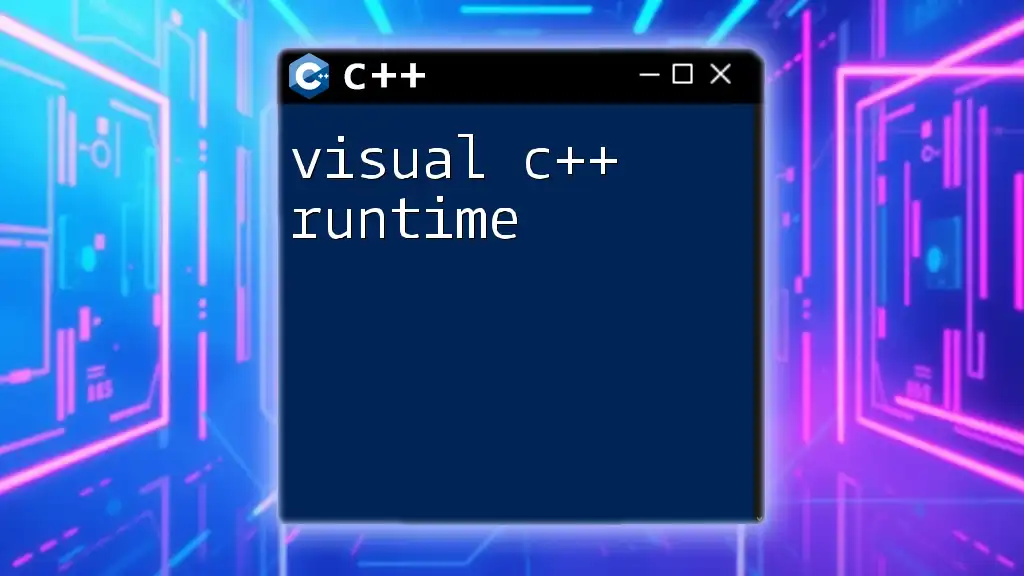
Additional Resources
For further learning and support, refer to the official Microsoft documentation for Visual C++ Build Tools, as well as tutorials and community forums that offer assistance and insights from experienced developers.