The Visual C++ Build Tools provide a lightweight package for compiling C++ applications without needing the full Visual Studio IDE, enabling developers to quickly set up a development environment.
Here's a basic example of using a C++ command to compile a simple program:
// hello.cpp
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
And to compile this program using the command line after installing Visual C++ Build Tools, you would use:
cl hello.cpp
What are Visual C++ Build Tools?
Visual C++ Build Tools are essential components in the process of building applications using C++. They include a compiler, linker, libraries, and header files that collectively enable developers to transform their code into executable programs. Unlike comprehensive Integrated Development Environments (IDEs), which generally offer user interfaces for coding, debugging, and building, build tools focus primarily on the compilation and linking stages of software development.
Components Included
- Compiler: Translates C++ source code into machine code that the computer can execute.
- Linker: Combines multiple object files into a single executable, resolving references to functions and variables.
- Libraries: Pre-compiled code that developers can use to perform common tasks without having to write code from scratch.
- Header files: Contain declarations for functions and macros to be used throughout the codebase.
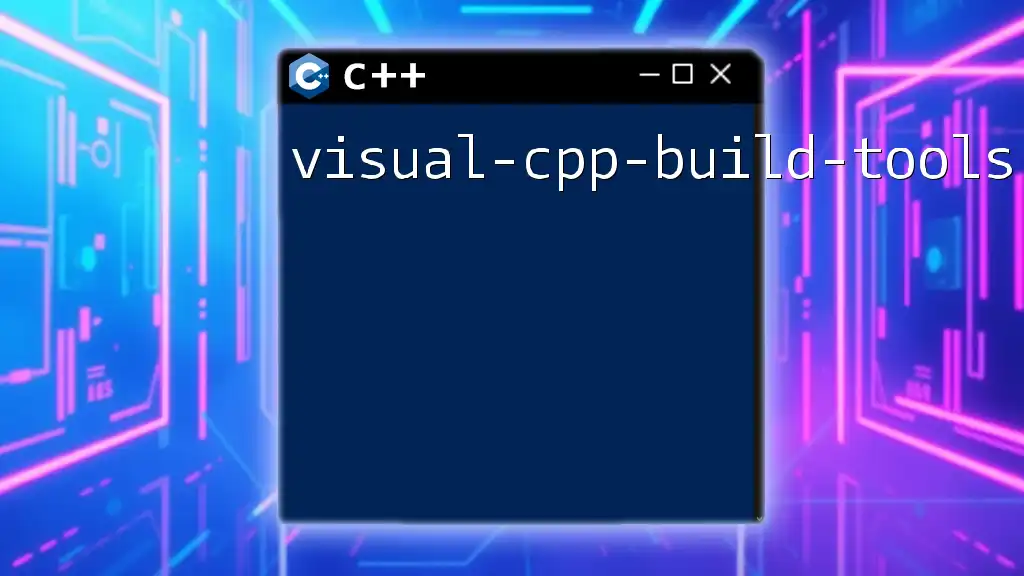
Setting Up Visual C++ Build Tools
System Requirements
Before installing Visual C++ Build Tools, it is important to ensure that your system meets the necessary requirements. The recommended Windows operating systems include Windows 10 and later. Check that you have sufficient storage space, typically requiring several gigabytes for a complete installation.
Installation Process
The installation process is straightforward, but it can be daunting for newcomers. Follow these steps:
- Visit the Official Site: Open the URL [https://visualstudio.microsoft.com/visual-cpp-build-tools/](https://visualstudio.microsoft.com/visual-cpp-build-tools/) to download the installer.
- Run the Installer: After download, run the installer executable.
- Select C++ Build Tools: In the installer, select the C++ build tools workload to include the necessary components.
- Complete Installation: Click install and follow any prompts that appear. This may take several minutes.
Additionally, you might encounter installation issues such as permission errors or conflicts with existing software. If so, ensure your user account has the necessary permissions or try disabling antivirus temporarily.
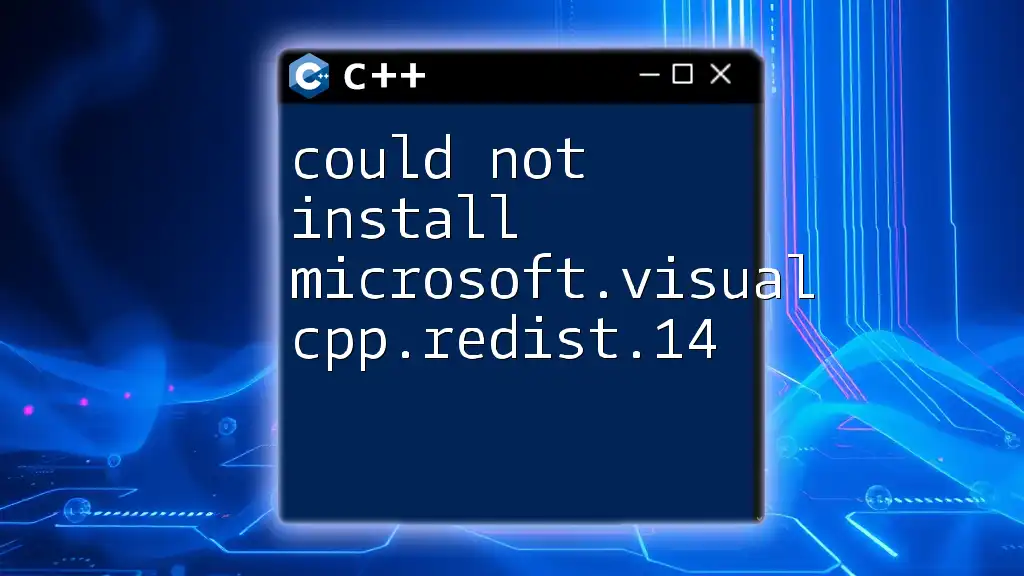
Key Features of Visual C++ Build Tools
Command Line Interface
Using the command line for building C++ applications offers numerous benefits, such as scriptability and automation. It allows for faster workflow, especially when compiling large projects.
To compile a simple program using the command line, you can use the following syntax:
cl /EHsc example.cpp
/EHsc ensures that the compiler handles exceptions safely.
Integration with Visual Studio
Visual C++ Build Tools are designed to work seamlessly with Visual Studio. By enabling the C++ workload within Visual Studio, developers gain access to a rich set of tools that enhance the development experience. To enable the C++ workload:
- Open the Visual Studio Installer.
- Select your installed version.
- Click "Modify" and check the "Desktop development with C++" option.
Environment Variables
Setting environment variables such as PATH allows you to run build tools from any command prompt without needing to specify their full paths.
To set PATH:
- Open System Properties.
- Navigate to Environment Variables.
- Add the path of the Visual C++ Build Tools bin directory to the PATH variable. This allows you to invoke the compiler with `cl` from any command prompt.
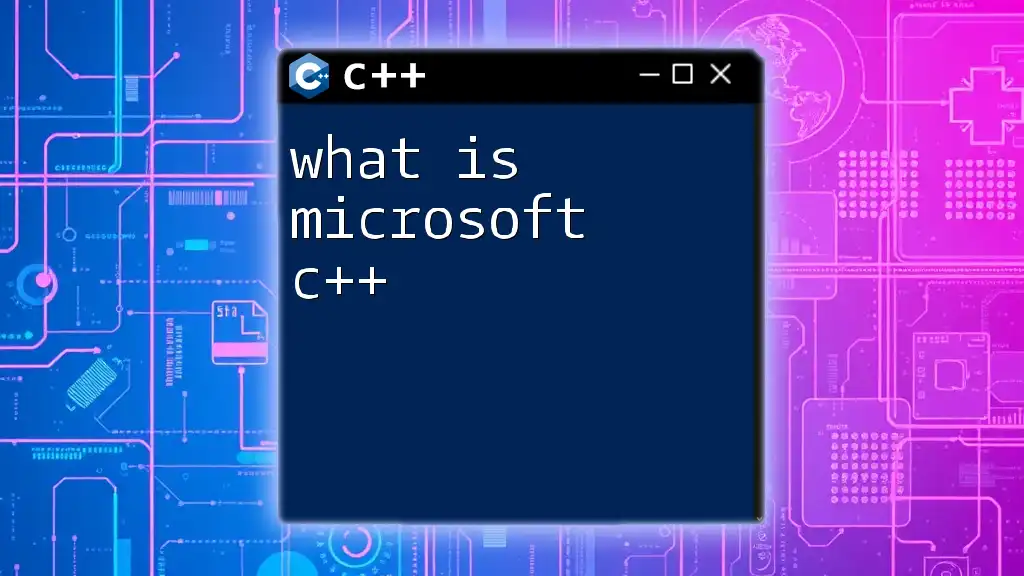
Basic Commands in Visual C++
Compiling a Simple Program
One of the first things you will do when using Visual C++ Build Tools is compiling a simple "Hello, World!" program. Below is a basic example:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
To compile this code, save it as `hello.cpp` and use the following command in the command prompt:
cl hello.cpp
Running the above command generates an executable called `hello.exe`, which you can run directly.
Linking Multiple Source Files
When developing larger applications, you will likely need to compile and link multiple source files. For instance, if you have two source files, `file1.cpp` and `file2.cpp`, you can compile both and link them together with:
cl file1.cpp file2.cpp
This command will generate a single executable that includes components from both source files.
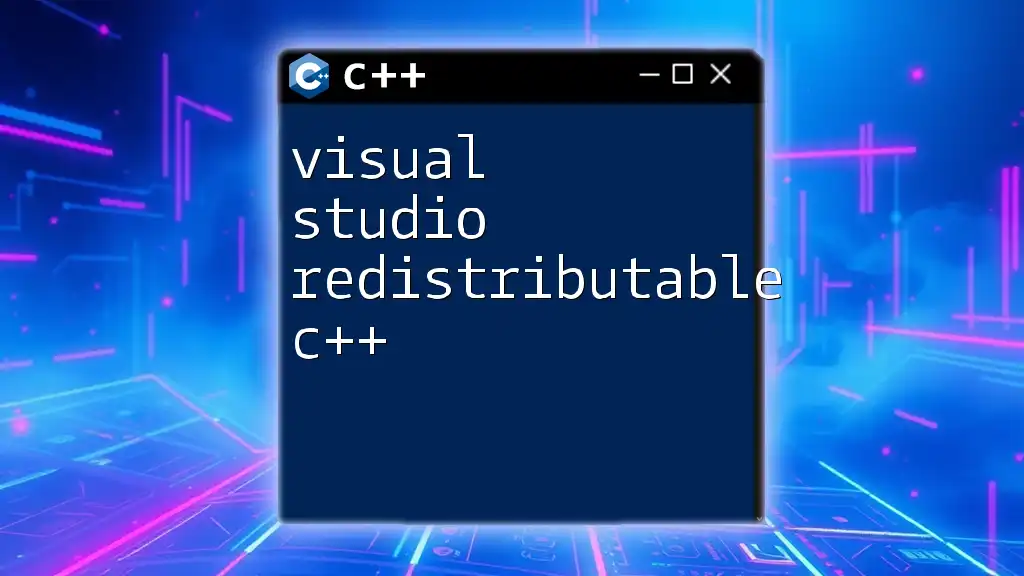
Beyond Basics: Advanced Usage
Static vs. Dynamic Libraries
Understanding the difference between static and dynamic libraries is crucial for effective C++ programming. Static libraries are linked at compile-time and become part of the executable, meaning they do not require the library to be present on the target machine. Dynamic libraries (DLLs), on the other hand, are linked at run-time and can save space but require the library to be accessible at execution.
To create a static library, use:
lib /OUT:myLib.lib mySource.obj
To use a dynamic library, you would compile your code with:
cl /LD mySrc.cpp
This generates a DLL that can be shared across multiple applications.
Custom Build Configurations
For most projects, you will want to create different build configurations, such as Debug and Release. These configurations often use different compiler flags for optimization or debugging.
You can set up a custom build configuration with specific flags:
cl /EHsc /Zi /Fe:myProgram.exe mySource.cpp
In this case, /Zi generates debugging information, which is essential for code debugging in the development phase.
Makefiles and CMake
Using Makefiles or CMake can streamline your build process for larger projects. Here is a basic example of a Makefile:
CC = cl
CFLAGS = /EHsc
TARGET = myProgram.exe
SOURCES = main.cpp helper.cpp
$(TARGET): $(SOURCES)
$(CC) $(CFLAGS) $(SOURCES) /Fe:$(TARGET)
This Makefile compiles multiple source files into a single executable, automating much of the command-line work.
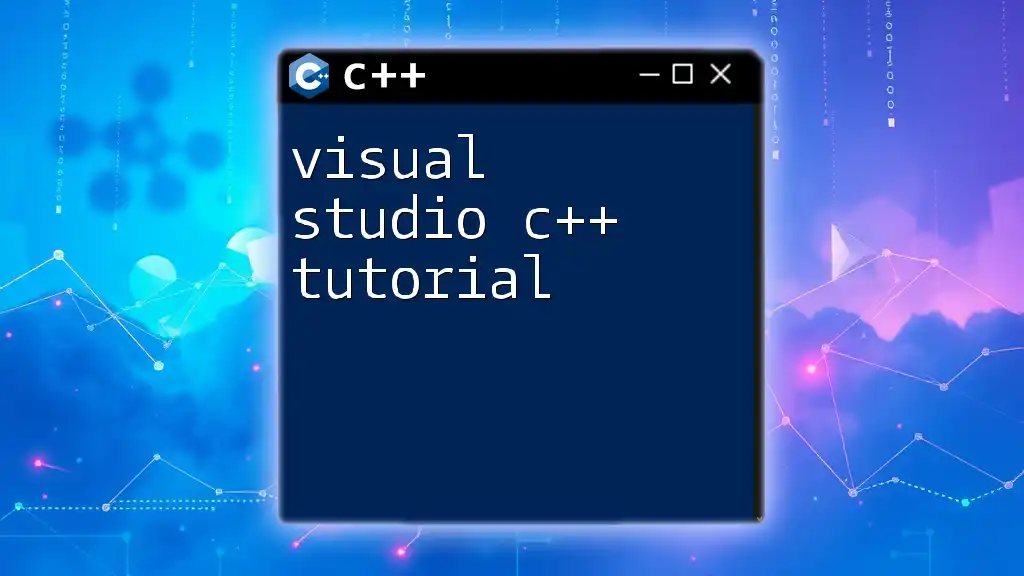
Troubleshooting Common Issues
Compilation Errors
When starting with Visual C++, you may encounter compilation errors such as Syntax Errors or Unknown Type Names. These problems can often be resolved by checking your code for typos or ensuring that all required header files are included.
Linking Errors
Linking errors may arise if the compiler and linker cannot find specified functions or variables. Common causes include mismatched function signatures or missing object files. Always double-check your code and ensure that all necessary files are being linked.
Deployment Issues
Deployment can introduce its own set of challenges. It's crucial to correctly package all required libraries, especially for dynamic libraries. Using tools such as `windeployqt` can help streamline this process.
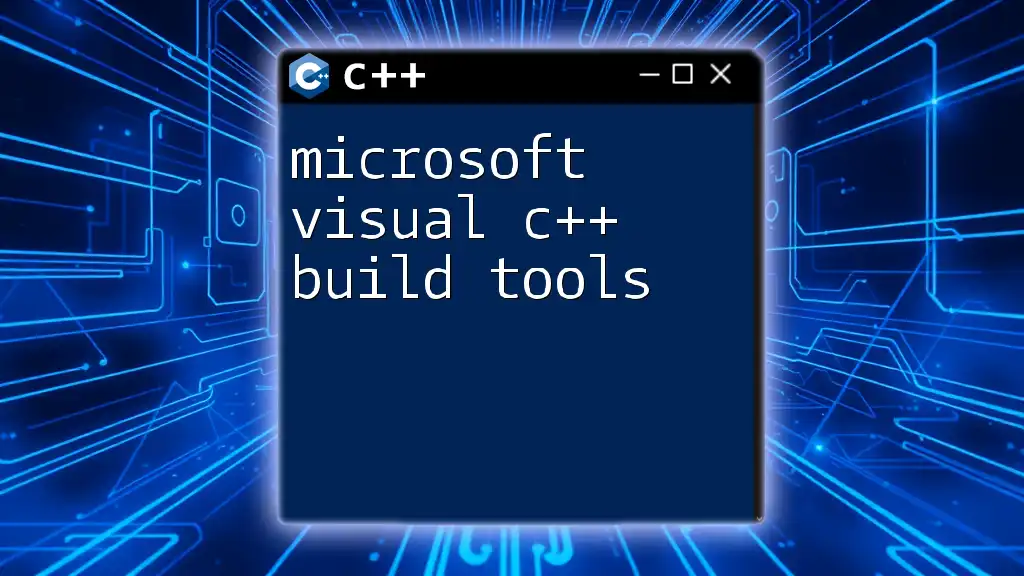
Tips for Efficient Usage
Scripting Builds
Another effective approach for working with Visual C++ Build Tools is scripting the build process. You can create batch files that automate compilation:
@echo off
cl /EHsc main.cpp helper.cpp /Fe:myProgram.exe
pause
Running this batch file will compile your program with a single command, saving time and reducing human error.
Performance Optimization
To enhance performance, consider using precompiled headers. This technique can significantly reduce compilation times for larger projects that use extensive headers.
To create a precompiled header file:
// pch.h
#include <iostream>
#include <vector>
Compile it with the following command:
cl /Ycpch.h /Fp precompiled.h
Utilizing this can greatly speed up your build times.
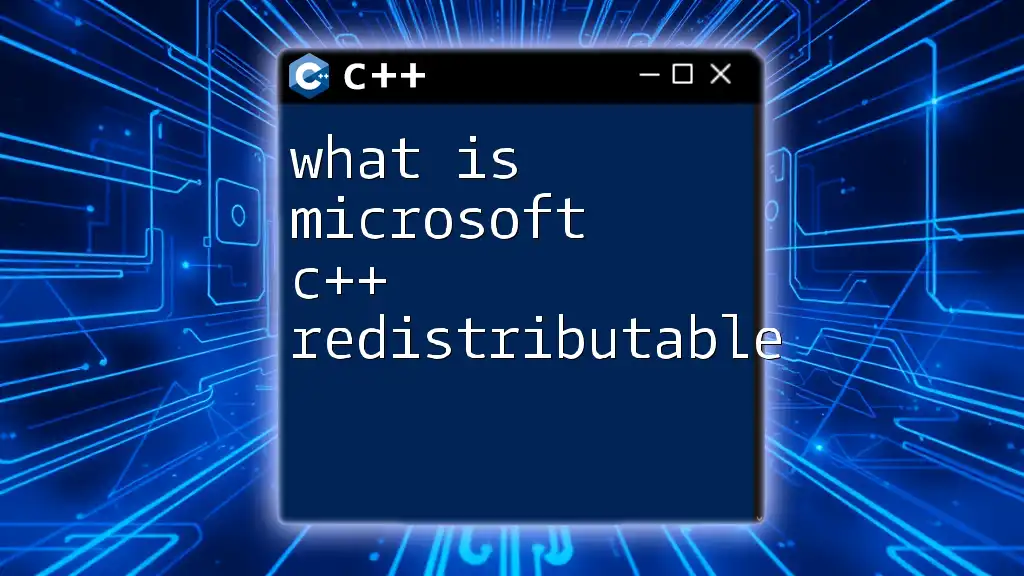
Conclusion
Mastering Visual C++ Build Tools is an important step for anyone serious about C++ development. Understanding the ins and outs of these tools will not only streamline your workflows but also enhance your overall programming efficiency. Diving deeper into advanced features and best practices can further increase your proficiency with Visual C++.
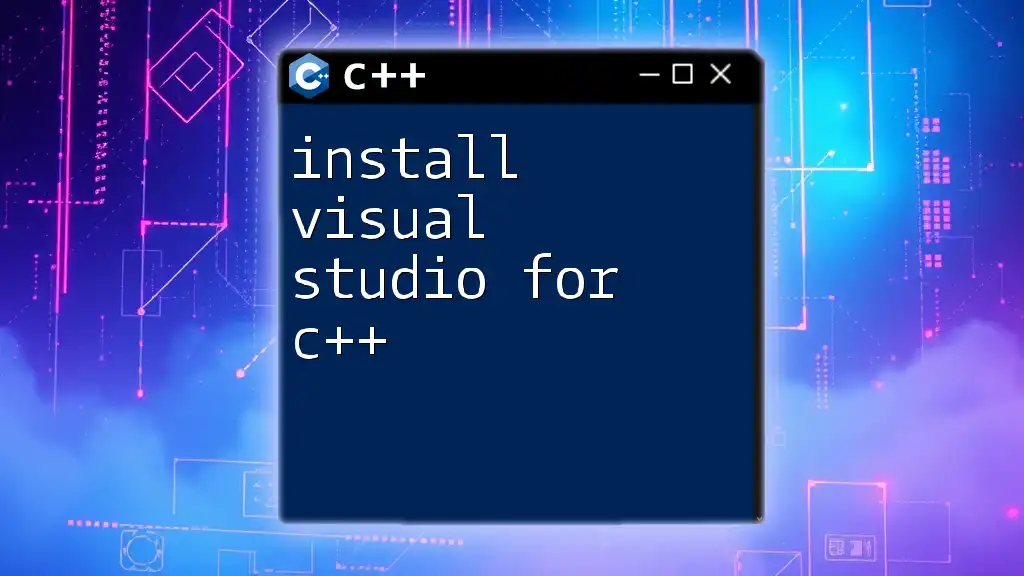
FAQs
What are the differences between Visual C++ Build Tools and a full IDE?
Visual C++ Build Tools focus solely on compilation and linking, providing a command-line interface for building applications. In contrast, a full IDE like Visual Studio offers a complete development environment, including a graphical interface for coding, debugging, and visual design capabilities.
Can I use Visual C++ Build Tools on other operating systems?
Currently, Visual C++ Build Tools are tailored for Windows environments. For cross-platform C++ development, alternative compilers and build systems such as GCC or Clang may be more appropriate.
Where can I find additional resources or tutorials?
The official Microsoft documentation, forums, and numerous online courses offer extensive resources for learning more about Visual C++ Build Tools. Engaging with the community can also provide valuable insights and assistance.