To effectively use Visual Studio for C++, start by creating a new project, selecting the appropriate C++ template, and then writing your code in the editor, like this example that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Setting Up Visual Studio for C++
Downloading Visual Studio
To get started on your journey on how to use Visual Studio for C++, the first step is downloading the software. Navigate to the official Microsoft Visual Studio website to find the latest version. While Visual Studio Community is free and offers a robust set of features, Visual Studio Professional and Enterprise provide additional tools and support for teams and enterprises. Choose the version that best suits your needs.
Installing Visual Studio
Once you have the installer, run it and follow the installation prompts. During this process, you will be prompted to select the workloads you want to install. Make sure to choose the "Desktop Development with C++" workload. This ensures that you have all necessary tools for C++ development right from the start.
There are also optional components you can select, such as Windows SDKs and C++ profiling tools. Setting up the IDE correctly at this stage saves time and frustration later on.

Exploring the Visual Studio Interface
Understanding the IDE Layout
To effectively work with Visual Studio, familiarize yourself with its Integrated Development Environment (IDE) layout. The primary components include:
- Menu Bar: Contains menus for file management, editing, and accessing tools.
- Toolbars: Offer quick access to tools and commands you use frequently.
- Solution Explorer: Lets you manage your projects, files, and resources.
- Editor Window: Where you write and edit your code.
Navigating these components skillfully will greatly enhance your productivity.
Customizing the Workspace
A well-tailored workspace can help you focus and work more efficiently. You can change the theme and layout of Visual Studio according to your preferences, providing a more inviting environment. Utilize options to arrange windows for quick access to your most-used functions.
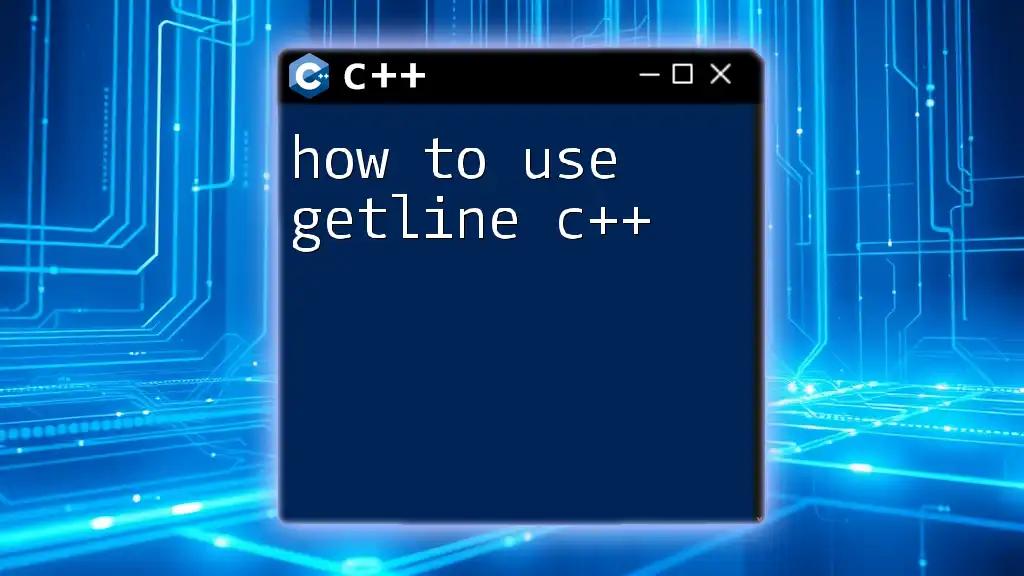
Creating a New C++ Project
Starting a New Project
To begin your first project, navigate to File > New > Project. From the template options, select a C++ template (e.g., "Console App"). This sets up a project structure with all necessary files and settings already in place, allowing you to focus purely on coding.
Configuring Project Settings
Before delving into coding, it’s essential to configure your project properties to suit your workflow. You can manage settings related to the target platform, debug settings, configuration types (Debug/Release), and more. Tailoring these settings at the outset can avoid future headaches.
Understanding the Solution Explorer
The Solution Explorer is your project management hub within the IDE. It helps you see all files in your project and enables you to add existing files or new folders seamlessly. Mastering this tool is critical, as it organizes your C++ resources effectively.
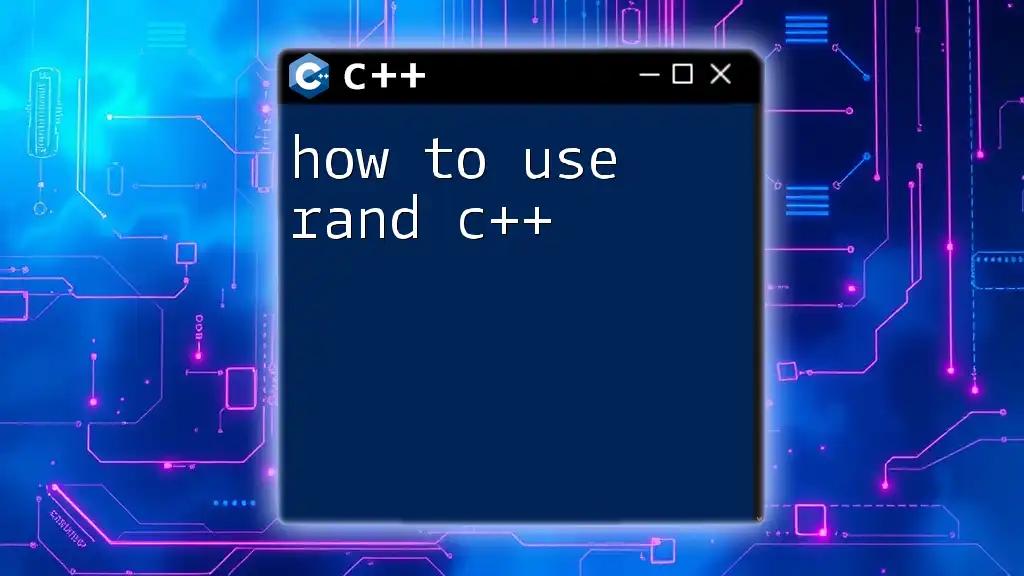
Writing Your First C++ Program
Writing Code in Visual Studio
Now it's time to write some code. Create a new source file named main.cpp. Within this file, input the basic syntax for a simple "Hello, World!" program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This simple program introduces you to the core syntax of C++.
Building the Project
Once you have written your code, you must build your project to turn your source code into an executable program. You can do this by navigating to Build > Build Solution. If successful, you will see a message in the Output Window confirming that the build has completed without errors.
Running Your Program
To execute your program, simply hit Ctrl + F5 or navigate to Debug > Start Without Debugging. The console window will display "Hello, World!" demonstrating that your code runs flawlessly. If any errors occur, return to the code and utilize the Error List for guidance.
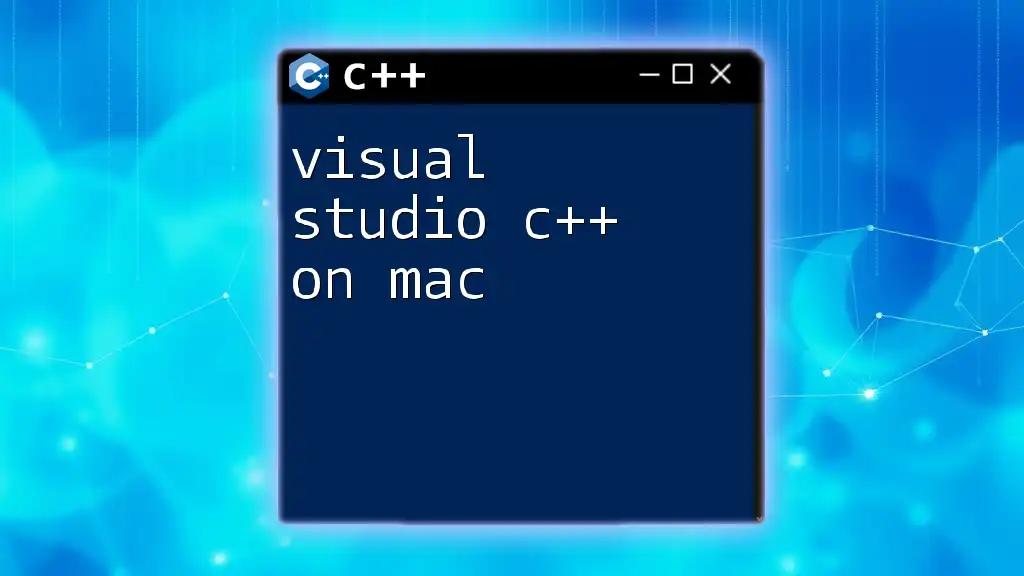
Debugging in Visual Studio
Introduction to the Debugger
Debugging is an essential skill in programming. Visual Studio provides a comprehensive debugger that allows you to identify and resolve issues within your code.
Setting Breakpoints
A valuable feature within debugging is breakpoints. These let you pause execution at certain lines of code, allowing you to examine the state of your program. Set a breakpoint by clicking in the left margin next to a line of code, turning it red.
Step Through your Code
When your program is paused at a breakpoint, use the debugging tools to step through your code. Utilize Step Over (F10), Step Into (F11), and Step Out (Shift + F11) effectively to navigate through your logic and inspect variable values. This approach will help you locate and fix issues more efficiently.
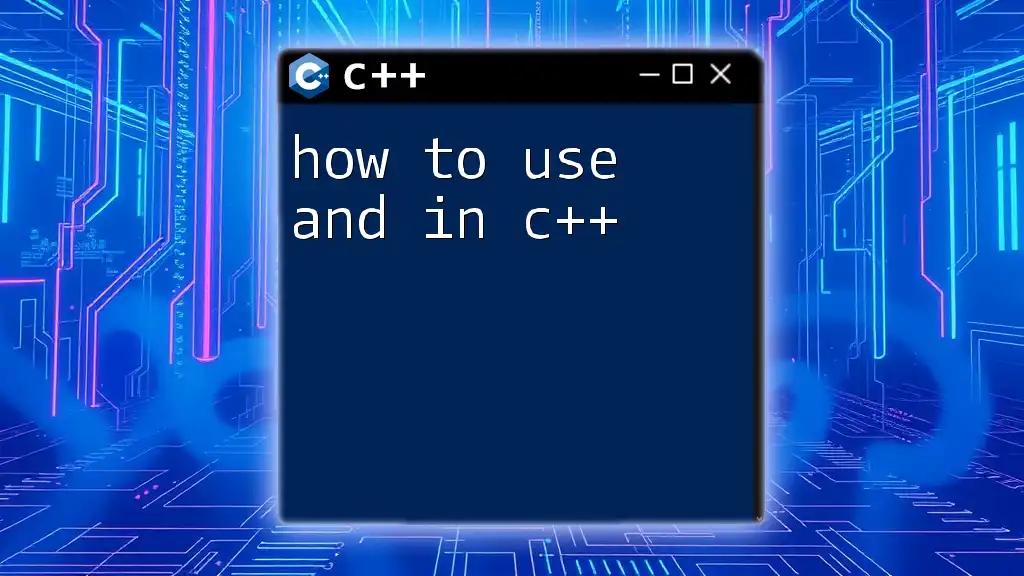
Advanced Features of Visual Studio for C++
Utilizing Code Snippets
Visual Studio offers code snippets that automatically insert commonly used code structures. For example, typing `for` and pressing the Tab key twice generates a for-loop structure. These snippets can save you considerable time while coding.
Using IntelliSense
IntelliSense is another powerful feature that provides dynamic code suggestions as you type. It shows context-aware information about functions, variable types, and more. Try starting a function name; IntelliSense will suggest options and parameters, significantly speeding up the coding process.
Embracing Extensions
To supercharge your C++ development experience, explore options for extensions. Popular extensions include Visual Assist, which enhances code navigation and productivity, and ReSharper C++, which improves code quality through inspections and refactorings. These extensions can be installed directly through the Extensions menu in Visual Studio.
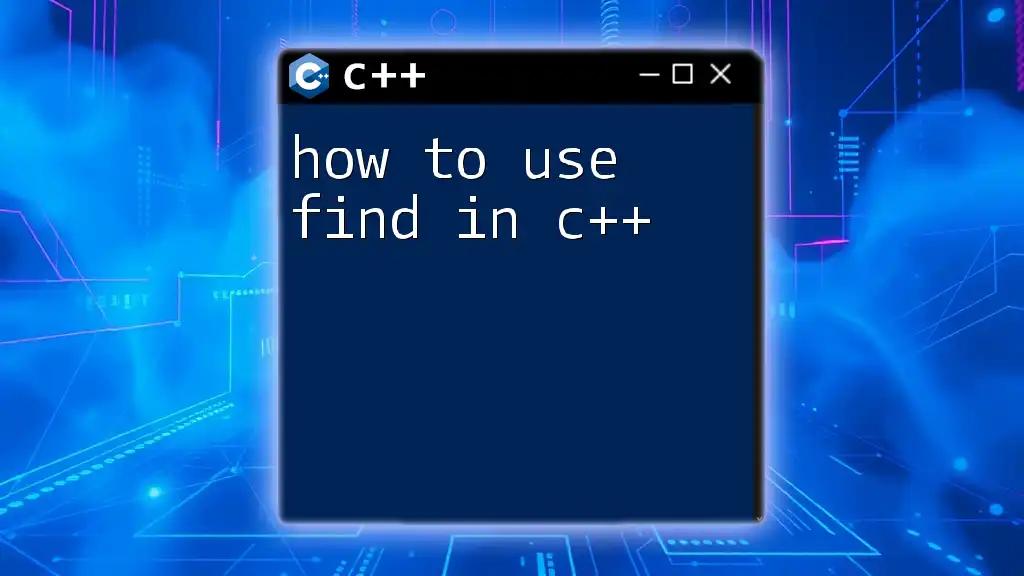
Best Practices for C++ Development in Visual Studio
Organizing Your Code
Keeping your codebase organized cannot be overstated. Use header (.h) and source (.cpp) files to structure your projects logically. This practice helps you compartmentalize functionality and makes your code easier to manage and understand.
Leveraging Version Control
As your projects grow, so does the need for version control. Visual Studio integrates seamlessly with Git, allowing you to manage changes, collaborate with others, and maintain project history. Familiarize yourself with basic Git commands through the Team Explorer to make collaboration a breeze.
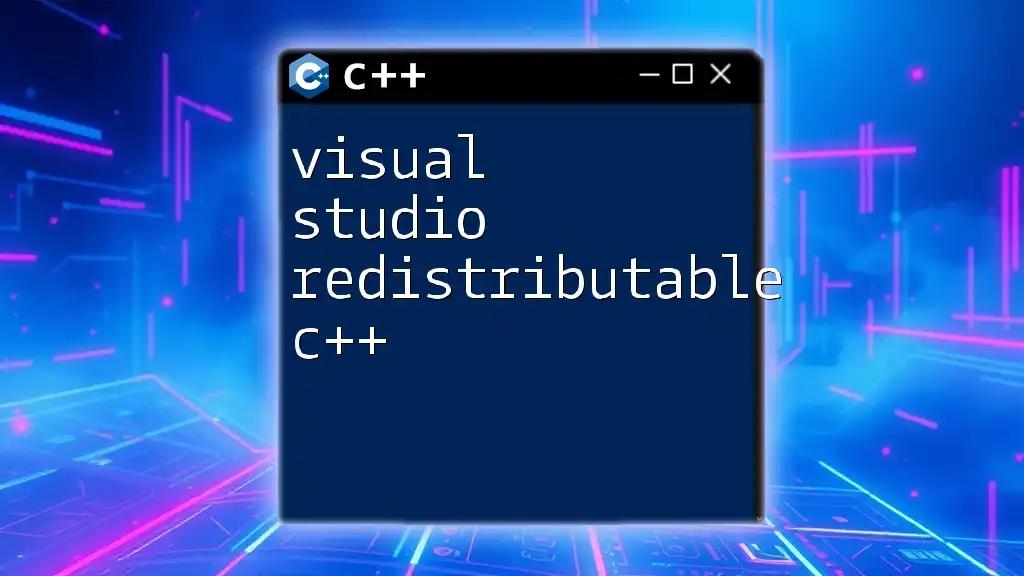
Conclusion
Understanding how to use Visual Studio for C++ is key to enhancing your programming skills. With its powerful features and user-friendly interface, Visual Studio can significantly augment your workflow and productivity.

Additional Resources
For further exploration, check out the official Visual Studio documentation. There are numerous C++ programming books and online courses that can complement your learning journey. Don’t forget to engage with communities and forums where you can ask questions and learn from other C++ developers.
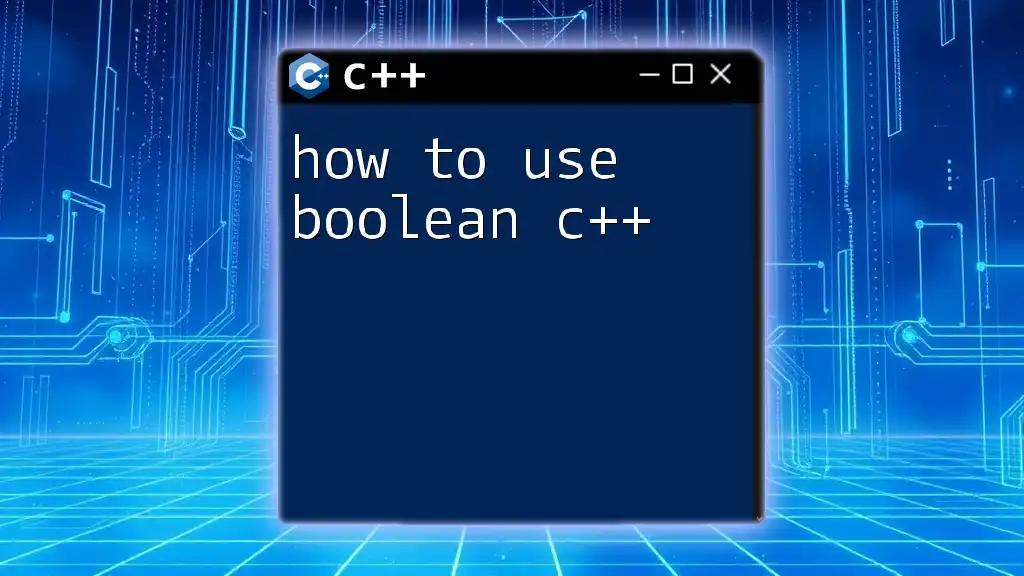
Call to Action
Now that you have a thorough guide on how to use Visual Studio for C++, I encourage you to dive in, experiment, and share your experiences. Subscribe to stay updated for more insights and tips to further enhance your C++ programming journey.