To read files in C++ using `ifstream`, you can create an instance of `ifstream`, open the desired file, and then utilize methods like `getline()` to read its contents, like shown in the following code snippet:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
} else {
std::cerr << "Unable to open file" << std::endl;
}
return 0;
}
Understanding ifstream
What is ifstream?
`ifstream` is a C++ class specifically designed for handling input file streams. The term stands for "input file stream," which allows programs to read data from files on the system. Understanding how to use `ifstream` is essential for working with external data and performing tasks such as data analysis, configuration management, or logging.
Including the Required Header
To utilize `ifstream` in your program, you need to include the `<fstream>` header. This header contains the definitions for file stream classes, including both `ifstream` and `ofstream` (used for output file streams). Here’s how to include it in your code:
#include <fstream>
This single line sets the stage for your file-handling capabilities in C++.

Creating and Opening Files with ifstream
Declaring and Initializing ifstream
To read from a file, the first step is to declare and initialize an `ifstream` object. The syntax is straightforward:
std::ifstream inputFile;
This line creates an instance of `ifstream` named `inputFile`, preparing it for use.
Opening a File
Using open()
To open a file for reading, you can use the `open()` method of the `ifstream` class. This method requires the name of the file you wish to open as a parameter:
inputFile.open("example.txt");
If the file exists in the specified directory, the `inputFile` object is now linked to that file, and you can begin reading its content.
Opening a File Directly
Alternatively, you may also open a file directly when declaring the `ifstream` object:
std::ifstream inputFile("example.txt");
This method simplifies the process by combining declaration and opening into a single line, enhancing code readability.

Error Handling with ifstream
Checking if the File is Successfully Opened
Error handling is a crucial aspect when working with file streams. It’s important to ensure that the file has opened successfully before attempting to read from it. This can be accomplished by checking the state of the `ifstream` object:
if (!inputFile) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
In this snippet, if the file fails to open (for reasons such as a missing file or incorrect permissions), a descriptive error message is displayed, and the program exits gracefully. By performing this check, you can prevent runtime errors that might arise from trying to read from an unopened or invalid file.

Reading from Files
Reading Lines with getline()
One of the most efficient ways to read text files in C++ is by using the `getline()` function. This function allows you to read a file line-by-line, which can be particularly useful for processing structured text. Here’s how it works:
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
In this code block, each line read from `inputFile` is stored in the `line` variable and then printed to the standard output. The loop continues until there are no more lines to read, making it an effective and simple way to process all content line-by-line.
Reading Single Words
If you need to read individual words instead of whole lines, you can use the extraction operator (`>>`). This operator reads whitespace-separated words from the file. Here’s a sample of how this works:
std::string word;
while (inputFile >> word) {
std::cout << word << std::endl;
}
Each word found in the file will be printed on a new line. This method is straightforward and especially useful when processing space-separated tokens.
Reading Characters
In some cases, you might want to read files character-by-character. The `get()` function is suitable for this purpose:
char ch;
while (inputFile.get(ch)) {
std::cout << ch;
}
This approach will print each character in the file one after the other, which can be useful for low-level text processing tasks.

Closing the File
Once you are done working with a file, it is essential to close the file stream to free up resources. Closing files also prevents data corruption in some situations. You can close an `ifstream` object using the following code:
inputFile.close();
By including this line at the end of your file processing, you ensure that all resources associated with `inputFile` are properly released.

Example Program: Reading a Text File
Putting it All Together
Here’s a complete example that integrates all the concepts previously mentioned into a single program. This program demonstrates how to use `ifstream` to read lines from a text file:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream inputFile("example.txt");
if (!inputFile) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
return 0;
}
In this program:
- The file `example.txt` is opened.
- It checks if the file opens successfully; if not, an error message is displayed.
- Lines from the file are read and printed until the end of the file.
- Finally, the file is closed to free up resources.
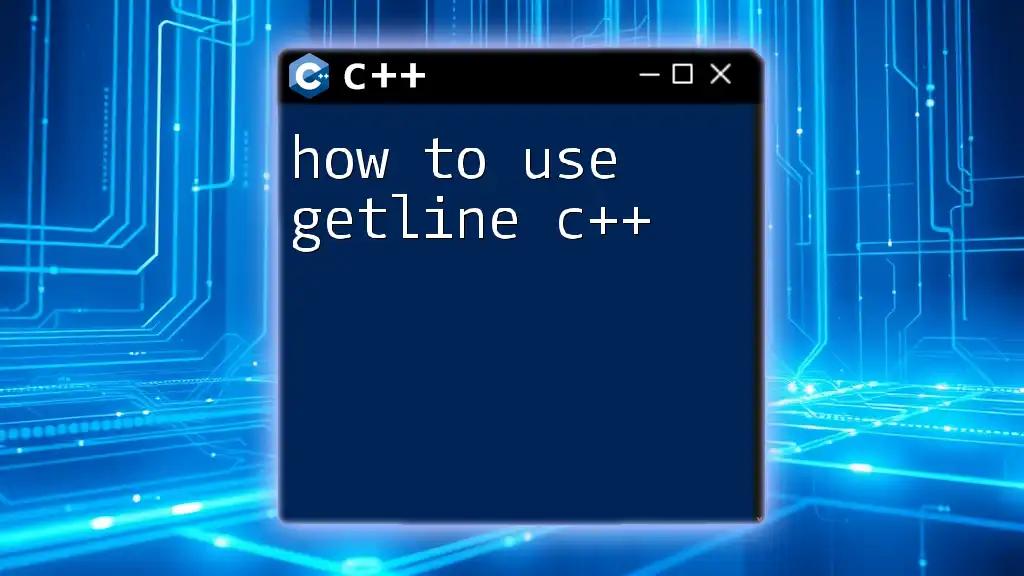
Common Pitfalls and Troubleshooting
Common Errors While Using ifstream
When working with `ifstream`, you may encounter several common issues:
- File not found: Ensure the file path is correct.
- Permission issues: Verify that your application has the right permissions to access the file.
- Uninitialized stream: Always check if the file opened successfully before reading.
Debugging Tips
To troubleshoot issues with file reading:
- Use print statements to confirm the program flow.
- Ensure correct paths and filenames.
- Check your environment configuration if using an IDE.

Conclusion
In conclusion, understanding how to use ifstream to read files in C++ is vital for any developer. By following the techniques discussed, such as opening files, handling errors, and reading content, you can efficiently manage file input in your programming endeavors. Practice these concepts regularly to become proficient in file handling.
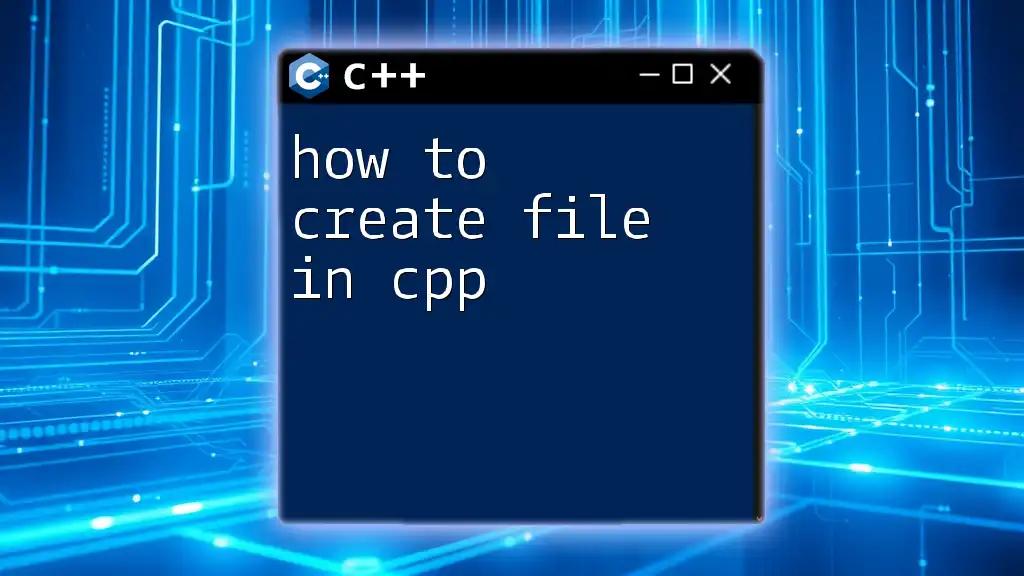
Additional Resources
For further learning, consider exploring books such as "The C++ Programming Language" by Bjarne Stroustrup, or online platforms like Codecademy and Coursera for interactive C++ courses. Practice files available on GitHub or educational repositories can provide excellent hands-on experience.
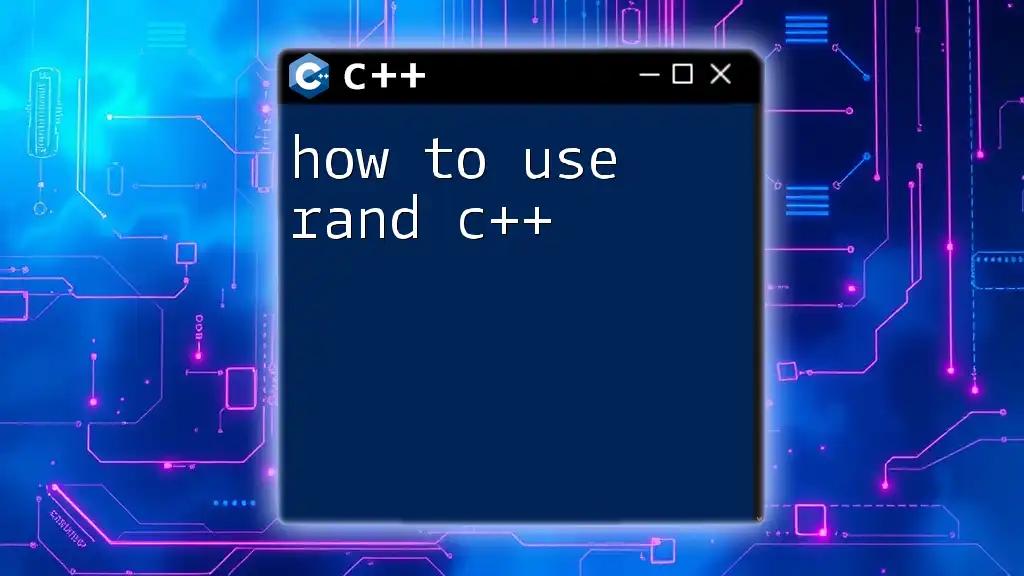
Call to Action
Feel free to share your experiences or ask questions related to file handling in C++. We would love to hear your thoughts and help you enhance your programming skills! Follow us for more tutorials and insights to improve your understanding of C++ and its features.