To create a header file in C++, simply define your functions and classes in a `.h` file and include it in your main program using the `#include` directive. Here's an example:
// ExampleHeader.h
#ifndef EXAMPLE_HEADER_H
#define EXAMPLE_HEADER_H
void myFunction();
#endif // EXAMPLE_HEADER_H
What is a Header File?
A header file is a crucial component of C++ programming that encapsulates declarations of functions, classes, variables, and constants that can be shared between multiple source files. This modular approach facilitates organized code structure and improves maintainability.
Common Extensions
Header files are generally saved with the extensions `.h` or `.hpp`. While both can be used interchangeably in many scenarios, `.hpp` tends to be associated more with C++ implementations. Understanding these extensions allows developers to quickly identify file types and their intended purpose.
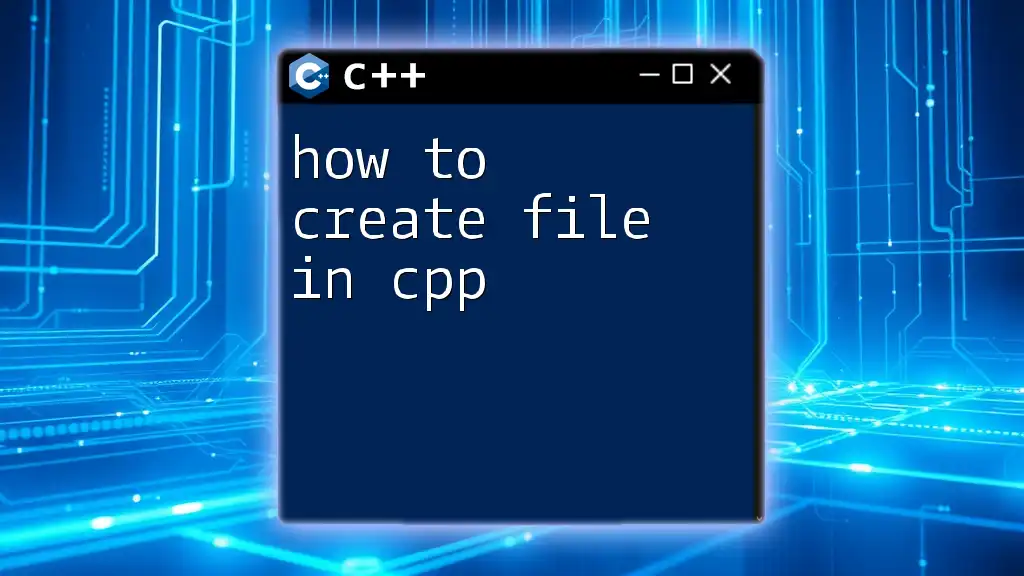
How to Create a Header File in C++
Creating a header file in C++ is a straightforward process that involves several key steps. Below is a detailed guide.
Step-by-Step Guide
-
Opening a Code Editor: Use any code editor or Integrated Development Environment (IDE) such as Visual Studio, Code::Blocks, or even simple text editors like Notepad++.
-
Creating a New File: Once your code editor is open, create a new file.
-
Naming Conventions for Header Files: Follow a consistent naming convention. Use descriptive names that specify what the header file encapsulates, such as `myheader.h` or `mathfunctions.hpp`.
Header File Structure
A header file typically includes declarations that define functionality without implementing it. Here’s an example to illustrate:
// myheader.h
#ifndef MYHEADER_H
#define MYHEADER_H
// Declarations of functions, classes, variables
void myFunction();
#endif // MYHEADER_H
In this example, `myheader.h` contains a single declaration for a function named `myFunction`.
Explanation of Include Guards
Include guards are essential for preventing multiple inclusions of the same header file, which can lead to compilation errors. The preprocessor directives `#ifndef`, `#define`, and `#endif` wrap around the header's content. If the file is included multiple times, the first inclusion defines the macro (in this case, `MYHEADER_H`), and subsequent inclusions check this definition, effectively excluding the file’s content from being processed again.
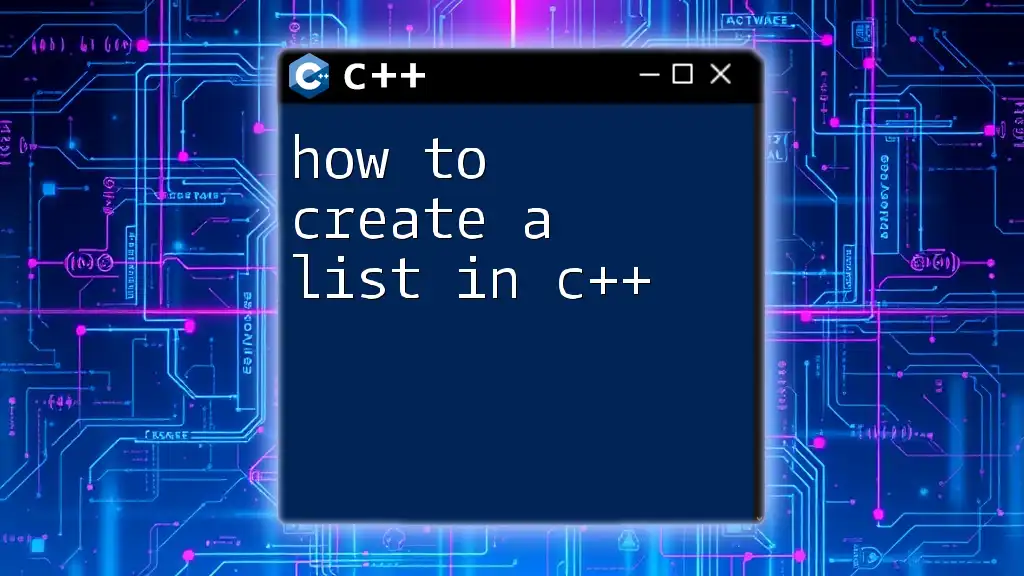
How to Include a Header File in C++
To utilize a header file created with the previous steps, you need to include it in your source file.
The Include Directive
The `#include` directive is used for this purpose. Use double quotes for user-defined header files (`#include "myheader.h"`) and angle brackets for system header files (`#include <iostream>`).
Example of Including a Header File
Here’s how to include your newly created header file in a source file:
// main.cpp
#include "myheader.h"
int main() {
myFunction();
return 0;
}
In this example, the `main.cpp` file includes `myheader.h`, allowing it to call `myFunction`.
Compilation Process
During the compilation process, the C++ compiler reads the contents of included header files and compiles them into the object code. As a result, any functions or variables declared in the header file become accessible within the source file.
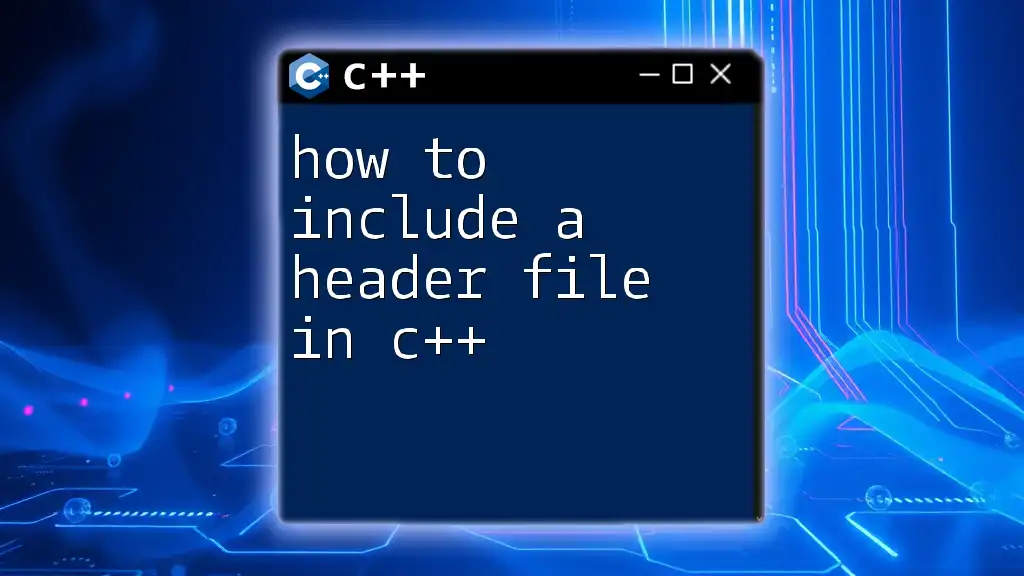
Best Practices in Header File Creation
Creating a header file is not solely about writing code, but also about coding effectively. Here are some best practices to follow.
Organizing Code with Header Files
Utilizing header files allows you to organize your code logically. For larger projects, structure your header files by functionality or module, thereby improving code readability and maintenance.
Minimizing Dependencies
It’s crucial to keep header files independent. Make use of forward declarations to minimize dependencies instead of including other header files directly. This prevents excessive coupling between components:
// Forward declaration
class MyClass;
// Function declaration using forward declaration
void useMyClass(MyClass* obj);
Comments and Documentation
Adding comments is vital for efficient collaboration and future reference. Document your functions clearly, describing parameters and return types. For standardized documentation, consider using Doxygen, which generates documentation from annotated source files. Here’s an example of properly documented code:
/**
* @brief Function that does something
*
* Detailed description of function behavior.
*
* @return int status of the function
*/
int myFunction();
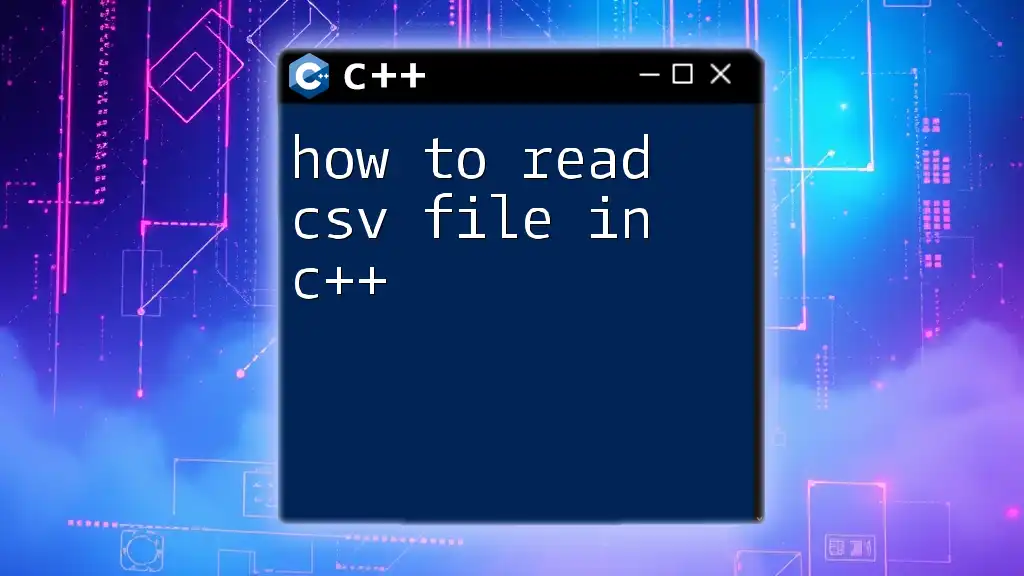
Common Mistakes When Creating Header Files
Even experienced programmers can make errors while dealing with header files. Below are some frequent pitfalls to avoid.
Forgetting Include Guards
Neglecting to include guards can lead to multiple definition errors, particularly in larger projects where files are included multiple times. Always ensure to use guards to maintain cleanliness in code.
Circular Dependencies
Circular dependencies occur when two or more header files include each other, resulting in confusion for the compiler. To resolve this, rely on forward declarations, and carefully structure your includes to avoid these cycles.
Over-Complexity
Keep header files focused on a specific purpose. Overly complex header files can confuse users and defeat their modular purpose. Aim for conciseness and clarity.
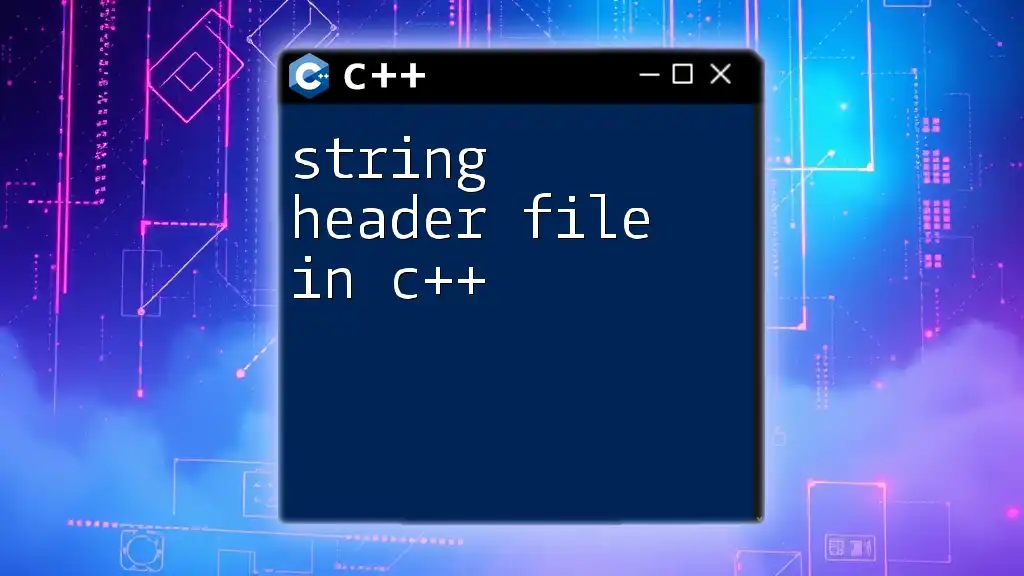
Conclusion
In summary, knowing how to create a header file in C++ is a key skill that enhances your programming practice. Header files not only allow you to reuse code but also foster better organization within your projects. Each time you define a header file, you're improving not just the current project but also your overall programming capability.
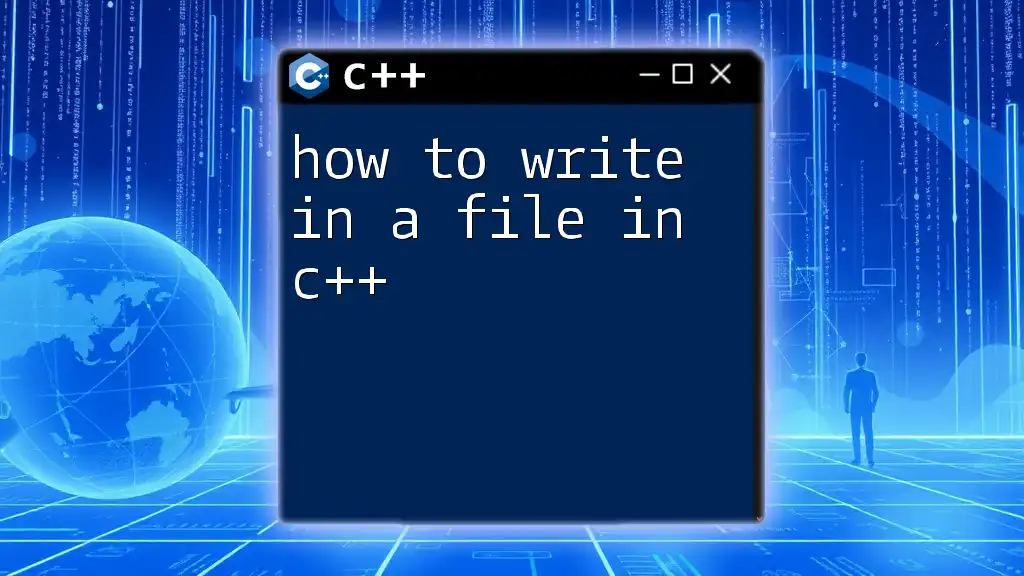
Additional Resources
To dive deeper into C++ programming and header files, consider exploring recommended books and online tutorials that delve into practical applications. Engaging with community forums can also offer additional insights and support for challenging concepts.
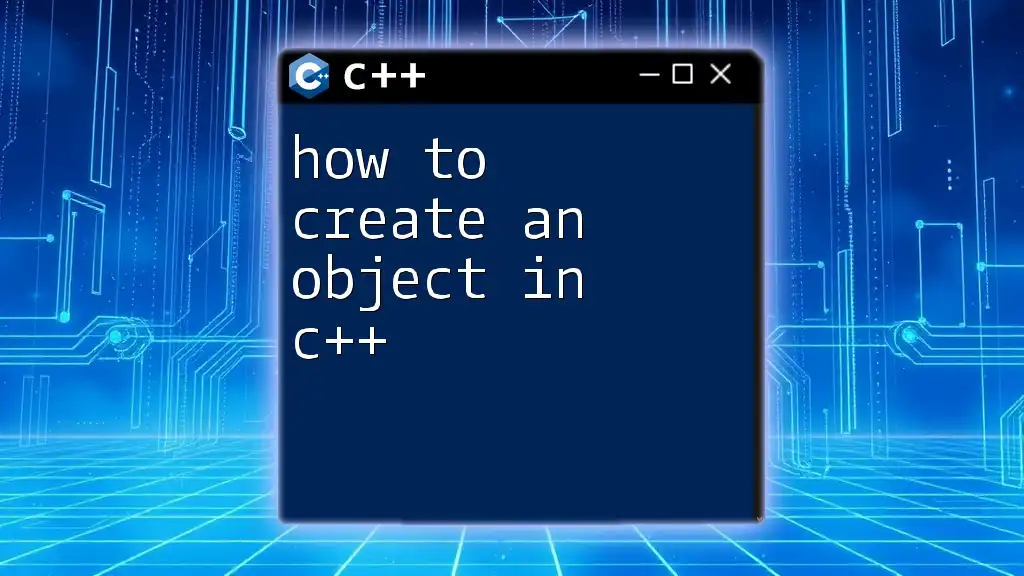
Call to Action
If you're interested in further expanding your C++ knowledge, join our courses! We offer structured learning paths focusing on practical coding skills, including how to create and efficiently manage header files in your projects.