To write a "Hello, World!" program in C++, you can use the following simple code snippet that includes the necessary header and outputs the text to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the C++ Environment
What You Need
To begin your journey in learning how to write hello world in C++, you will need to set up an adequate environment on your system. The basic tools and software you need include:
- Code Editors: Popular choices like Visual Studio Code or Notepad++ provide user-friendly interfaces for writing and editing C++ code.
- Compilers: C++ code needs to be compiled before execution. You can use GCC (GNU Compiler Collection), Clang, or Microsoft Visual C++.
Setting up your development environment typically involves installing one of these compilers and a code editor that suits your preferences.
Writing Your First C++ Program
Understanding the basic structure of a C++ program is crucial when learning how to write hello world in C++. At the core, every C++ program consists of:
-
Header Files: These are included at the beginning of the program using the `#include` directive. They provide the necessary declarations and definitions for the functions and classes you'll use.
-
Main Function: Every C++ program begins execution from the `main()` function. It serves as the entry point for any C++ application.
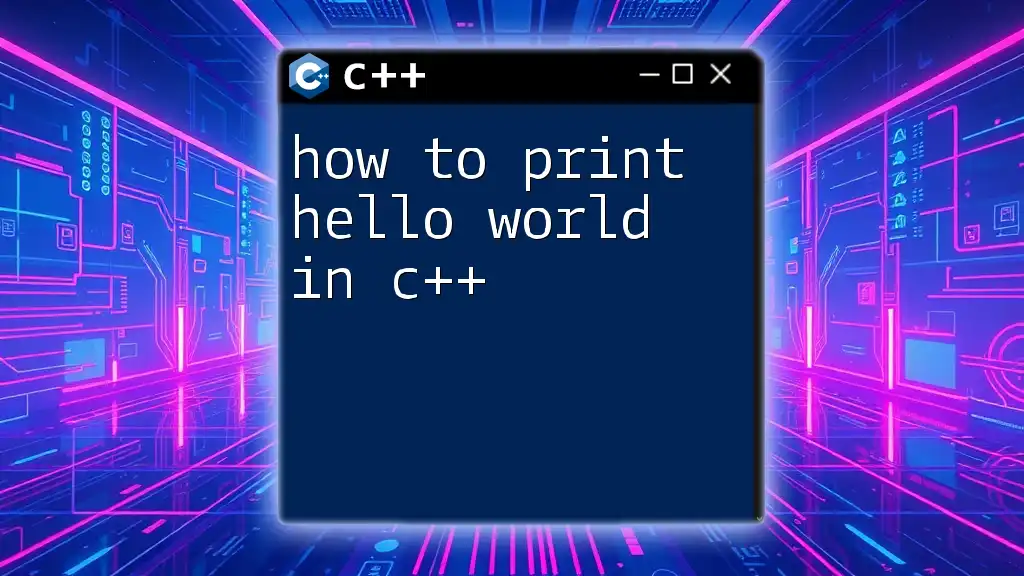
The "Hello, World!" Program
Step-by-Step Breakdown
Writing the Code
Now, let us look at the complete code snippet for the "Hello, World!" program:
#include <iostream> // Include the iostream header
int main() { // Start of the main function
std::cout << "Hello, World!" << std::endl; // Output the text
return 0; // Return statement
}
Code Explanation
-
#include <iostream>: This line includes the iostream header file, which is essential for utilizing input and output streams in C++. It allows you to use `std::cout` to print output to the console.
-
int main(): The `main()` function signifies where the execution of the program begins. In C++, every program must have this function.
-
std::cout: This is the standard output stream in C++. Using `std::cout` followed by the insertion operator (`<<`), you can print text or data to the console.
-
"Hello, World!": This is the string of text that will be printed to the console when the program runs. It serves as the most basic example of output in programming.
-
std::endl: This manipulator is used to insert a newline character in the output, thus moving the cursor to the next line after the text is printed. It also flushes the output buffer.
-
return 0;: This line indicates that the program has executed successfully. Returning zero from the `main()` function is a common convention indicating successful completion.
Compiling and Running Your Program
Using an IDE
To compile and run your C++ code using an IDE, follow these steps:
- Open your preferred IDE (e.g., Visual Studio).
- Create a new C++ project.
- Copy and paste the "Hello, World!" code into the main source file.
- Click on the "Build" or "Compile" option.
- Run the program using the "Run" button or terminal within the IDE.
Command Line Compilation
If you prefer using the command line for compiling C++ code, here is how you can do it:
- Open your command line interface.
- Navigate to the directory where your code file (let's say it's named `hello_world.cpp`) is saved.
- Use the following commands to compile and run the program:
g++ hello_world.cpp -o hello_world
./hello_world
- The command `g++ hello_world.cpp -o hello_world` compiles the source code into an executable named `hello_world`.
- The command `./hello_world` runs the generated executable.
Common Errors and Troubleshooting
While writing your "Hello, World!" program, you might encounter some common mistakes. Here are a few troubleshooting tips:
- Missing Semicolon: C++ requires a semicolon at the end of each statement. Forgetting this can lead to compilation errors.
- Incorrect Includes: If you forget to include the iostream header, you will encounter an error related to `std::cout`.
- Typographical Errors: Always double-check for spelling errors in your code. Even a small typo can generate an error message.
Understanding these errors can help you efficiently troubleshoot and get back on track when coding.
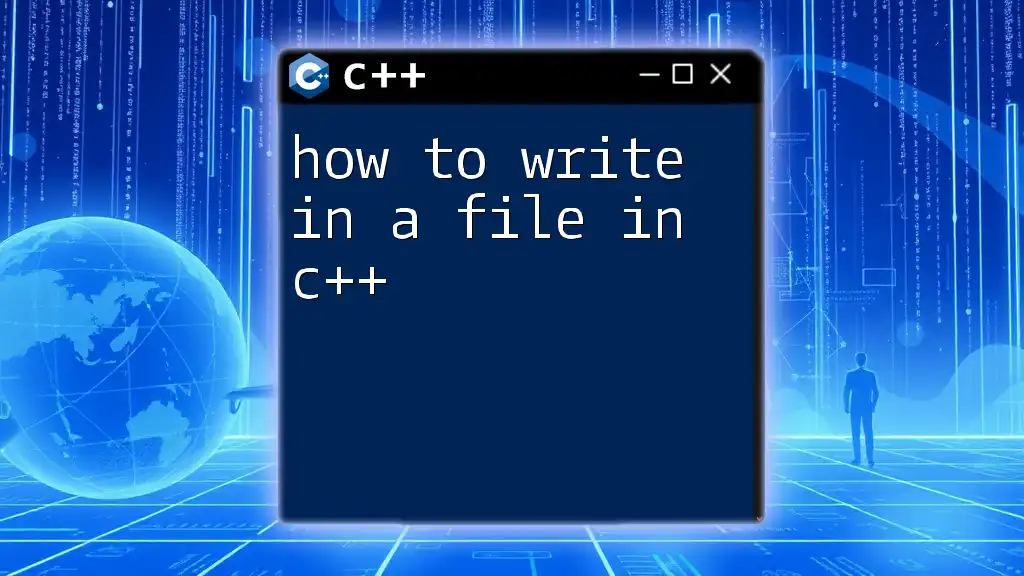
Expanding the Basics
Variants of "Hello, World!"
Using Different Output Methods
You can also demonstrate different methods of output. For example, using the `printf` function from the C standard library:
#include <cstdio>
int main() {
printf("Hello, World!\n"); // Using printf instead
return 0;
}
This shows an alternative way to print output, which is also valid in C++ but comes from the C language standards.
Using Input Statements
Your "Hello, World!" program can also be made interactive by allowing user input. Below is an example where the user is prompted to enter their name:
#include <iostream>
int main() {
std::string name;
std::cout << "Enter your name: ";
std::cin >> name; // Taking input from the user
std::cout << "Hello, " << name << "!" << std::endl; // Greeting the user
return 0;
}
In this code, we added functionality to read an input string from the user and then include that in a personalized greeting.
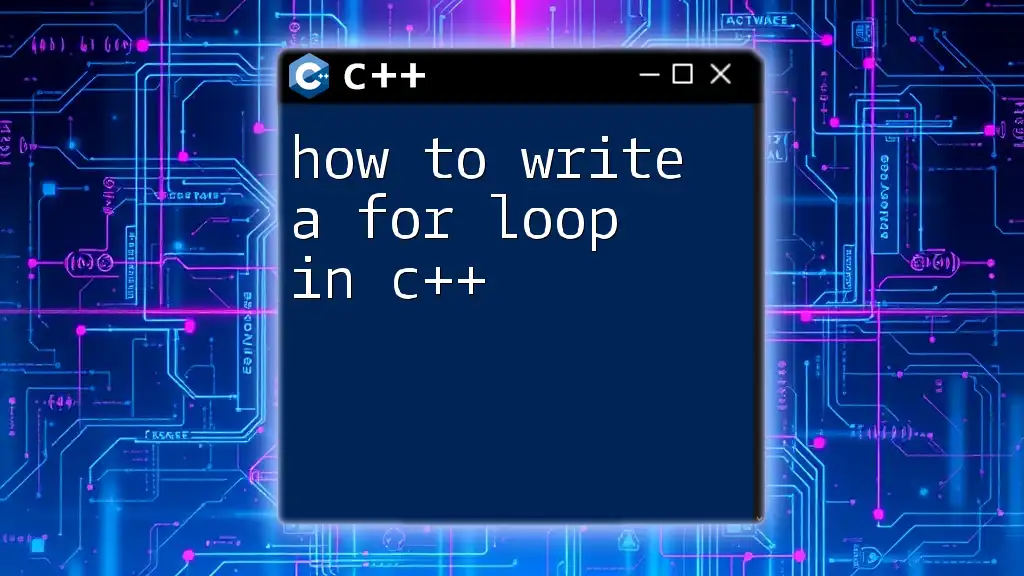
Conclusion
Learning how to write hello world in C++ is an important milestone in your programming journey. This simple program not only showcases the basic syntax of C++ but also sets the stage for more complex concepts you'll face as you advance. Don't hesitate to experiment with variations of this program to strengthen your understanding of the language.
As you embark on your coding adventure, remember that consistent practice and exploration of new features will significantly enhance your C++ skills.
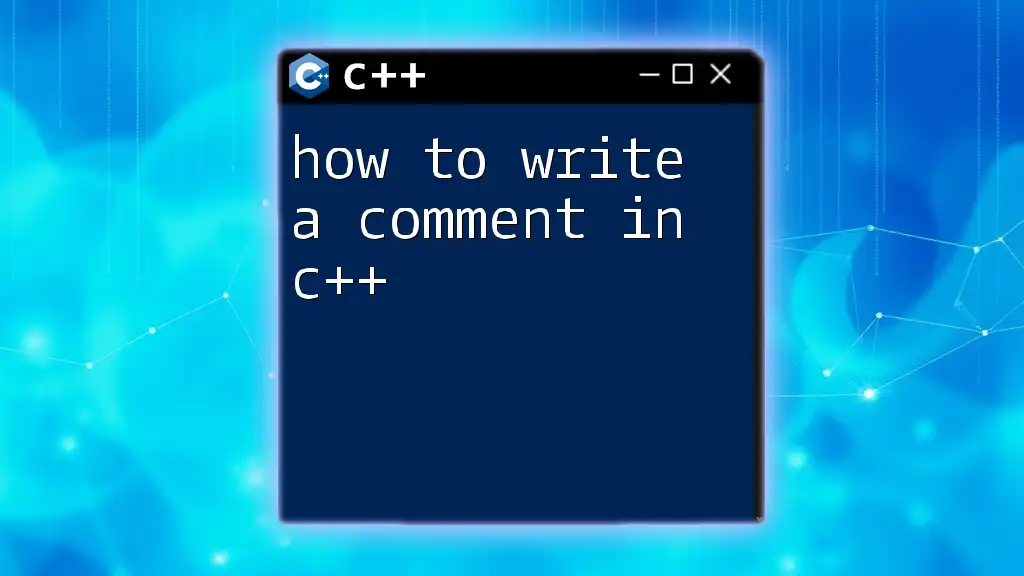
Additional Resources
To continue your learning, consider checking out some of the following resources:
- Recommended books and online courses specific to C++.
- Best practices for writing clean and efficient C++ code.
- Websites and forums where you can engage with other C++ learners and experts.
By integrating these resources into your study routine, you'll expand your knowledge and become proficient in C++. Happy coding!