To print "Hello, World!" in C++, you can use the following code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding the Basics of C++
What is C++?
C++ is a versatile, high-performance programming language that builds on the foundation of the C programming language. It incorporates features such as object-oriented programming, making it a powerful choice for software development, game programming, and system-level programming. C++ has evolved significantly since its inception in the 1970s, establishing itself as a staple in the programming community.
Why Start with "Hello World"?
The "Hello World" program is often the first step in learning a new programming language. It introduces essential programming concepts in a straightforward manner, helping beginners understand the syntax and structure of C++. By creating a simple program to display "Hello, World!", you learn about:
- Syntax: The specific rules for writing valid C++ code.
- Compilation: The process through which your human-readable code is transformed into machine code.
- Execution: Running your program to see the outcome.

Setting Up Your Environment
Installing a C++ Compiler
Before you can learn how to print "Hello World" in C++, you'll need to set up your development environment by installing a C++ compiler. Here are some popular options:
- GCC (GNU Compiler Collection): Widely used in Unix-based systems.
- MSVC (Microsoft Visual C++): A powerful option for Windows users within Visual Studio.
- Clang: A compiler for C/C++/Objective-C, known for its modularity and performance.
Each of these compilers has its own installation guide, but usually involves downloading an installer or package manager command.
Choosing an Integrated Development Environment (IDE)
An Integrated Development Environment (IDE) can significantly enhance your coding experience. Here are popular IDEs suitable for C++ development:
- Visual Studio: Feature-rich and user-friendly, ideal for Windows applications.
- Code::Blocks: Lightweight and simple, works on various platforms.
- CLion: A premium IDE that offers advanced features, including refactoring and debugging tools.
For each IDE, follow their specific installation instructions, and create a new project to start coding.

Writing the Hello World Program
Structure of a Basic C++ Program
Understanding the structure of a basic C++ program is vital. Every C++ program typically consists of:
- Headers: Lines that include libraries, such as `iostream`, that provide essential functions.
- Main Function: The entry point of every C++ program, designated by `int main()`.
- Statements: Lines of code that perform tasks, like printing text to the console.
C++ Code for Hello World
Here is the simple code to print "Hello, World!" in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Code Breakdown:
- `#include <iostream>`: This line tells the compiler to include the input-output stream library, which defines the `std::cout` object for outputting data.
- `int main()`: Defines the main function where the execution of the program begins.
- `std::cout`: This is the standard output stream, which allows you to print text to the console.
- `"Hello, World!"`: This string literal is what will be printed when the program runs.
- `std::endl`: This manipulator adds a new line and flushes the output stream, ensuring that everything printed appears immediately.
- `return 0;`: Indicates successful program termination.
Compiling and Running Your Program
Once you have written your program, it's time to compile and run it. Here’s how:
Using Command Line:
- Open your terminal or command prompt.
- Navigate to your project directory where the C++ file is saved.
- Use the following command to compile:
- For GCC: `g++ hello_world.cpp -o hello_world`
- For MSVC: `cl hello_world.cpp`
- Run the program:
- On command line, type `./hello_world` (or just `hello_world.exe` on Windows).
Using an IDE:
- Simply hit the "Run" button, which compiles and executes the program for you.
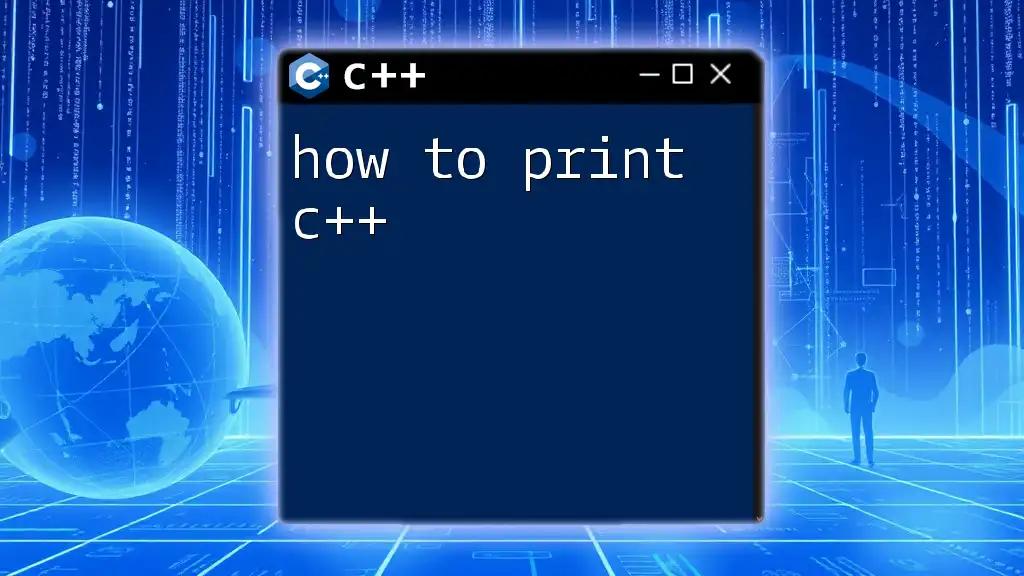
Common Errors and Troubleshooting
Syntax Errors
When learning how to print "Hello World" in C++, syntax errors are common. Some typical mistakes include:
- Missing Semicolons: Every statement in C++ must end with a semicolon.
- Incorrect Case: C++ is case-sensitive; `cout` must be `std::cout`.
- Unmatched Brackets: Ensure all brackets are properly opened and closed.
Be sure to double-check your code and refer to any error messages from the compiler for guidance.
Runtime Errors
Runtime errors can occur when the program is running. Common issues may include:
- Input Issues: If you prompt for user input but don’t handle it properly, it could lead to unexpected crashes.
- Uninitialized Variables: Using variables that haven't been assigned a value can result in unpredictable behavior.
Pay careful attention to error messages as they provide invaluable clues for diagnosing issues.

Enhancing Your Hello World Program
Adding User Input
Once you've mastered the basics, consider modifying your "Hello World" program to make it interactive. Here's how you can accept user input:
#include <iostream>
#include <string>
int main() {
std::string name;
std::cout << "Enter your name: ";
std::getline(std::cin, name);
std::cout << "Hello, " << name << "!" << std::endl;
return 0;
}
This code sample prompts the user for their name and welcomes them. You will learn about string types and handling input effectively.
Using Functions
As you grow in your coding journey, you'll likely want to write more organized and reusable code. Here's how to structure a function for your program:
#include <iostream>
void printHello(std::string name) {
std::cout << "Hello, " << name << "!" << std::endl;
}
int main() {
printHello("World");
return 0;
}
This snippet demonstrates the concept of functions, allowing you to define the action of printing greetings separately and call it with any name.
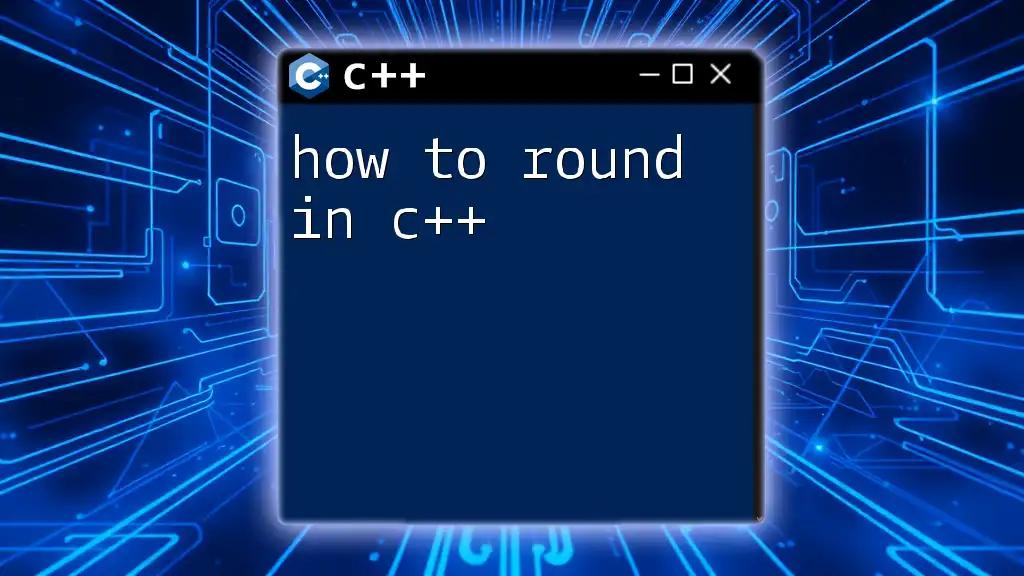
Conclusion
In this article, we've explored how to print "Hello World" in C++. We've learned about the structure of a C++ program, written our code, and compiled and executed it. Armed with this fundamental knowledge, you're well on your way to delving deeper into more complex C++ topics and programs.

Additional Resources
To further enrich your learning experience, I recommend the following resources:
- Books: "C++ Primer" by Lippman, "Effective C++" by Scott Meyers.
- Websites: Codecademy, LeetCode, and W3Schools.
- Courses: Udacity’s C++ Nanodegree, Coursera’s C++ for C Programmers.

Call to Action
Try creating your version of the Hello World program, play around with variations, and share your experiences. Questions are welcomed in the comments, and I encourage you to connect with fellow learners in your programming community!