A for loop in C++ allows you to execute a block of code repeatedly for a specific number of iterations, typically using a counter variable.
Here's a simple example:
for (int i = 0; i < 5; i++) {
std::cout << "Hello, World!" << std::endl;
}
Understanding the Basics of a For Loop
What is a For Loop?
A for loop is a control flow statement that allows code to be executed repeatedly based on a given boolean condition. It is a crucial component of programming that enables developers to automate tasks requiring the same operations over multiple iterations, significantly enhancing the efficiency of code execution.
Syntax of a For Loop
The basic structure of a for loop in C++ is as follows:
for (initialization; condition; increment/decrement) {
// Code to be executed
}
Breaking down each component:
- Initialization: This is where you set up your loop variable. Typically, you might start at zero or one, depending on your use case.
- Condition: The loop continues to execute as long as this condition evaluates to true. Once it becomes false, the loop terminates.
- Increment/Decrement: After each iteration, the loop variable is updated. This usually means increasing or decreasing its value by a specified amount.
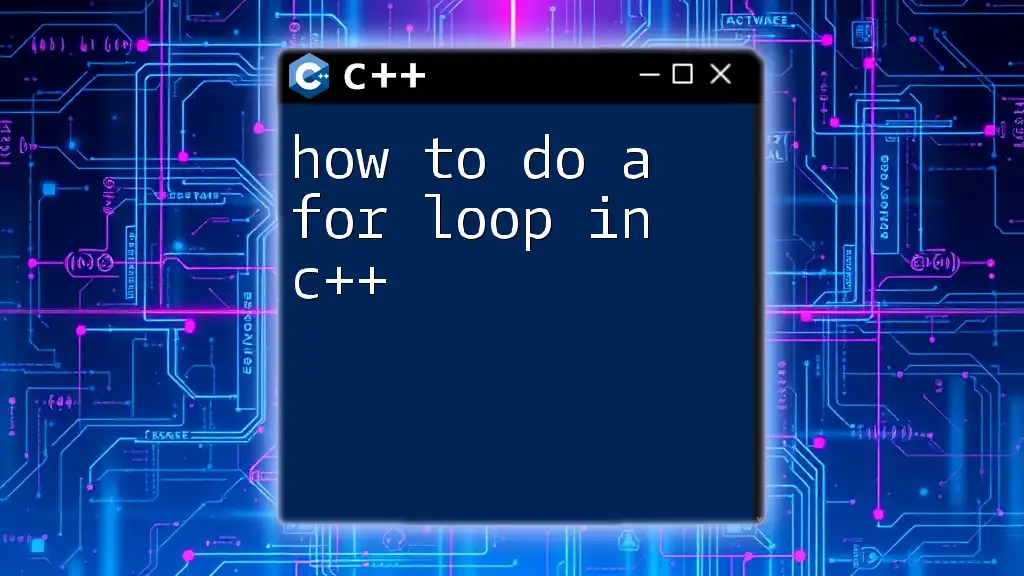
Writing a Simple For Loop
Example: Counting from 1 to 5
Let’s start with a practical example. Below is a simple C++ program that counts from 1 to 5 and displays the numbers.
#include <iostream>
int main() {
for (int i = 1; i <= 5; i++) {
std::cout << i << std::endl;
}
return 0;
}
Explanation:
- The variable `i` is initialized to 1.
- The loop checks if `i` is less than or equal to 5. If this condition holds true, the code inside the loop executes.
- The value of `i` is printed, followed by an increment (`i++`) that adds 1 to `i`.
- This process continues until `i` exceeds 5.
Nested For Loops
What are Nested For Loops?
Nested for loops are loops within loops that are particularly useful for dealing with multi-dimensional data structures, such as matrices. When you need to iterate through each dimension, nested loops provide a way to efficiently manage the process.
Example: Creating a Multiplication Table
Here’s an example of using nested for loops to generate a simple multiplication table.
#include <iostream>
int main() {
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= 5; j++) {
std::cout << i * j << "\t"; // Tabbed output for formatting
}
std::cout << std::endl; // New line after each row
}
return 0;
}
Explanation:
- The outer loop iterates through numbers 1 to 5 for the first factor.
- The inner loop does the same for the second factor, calculating the product of the two variables (i and j).
- The result is printed in a tab-separated format, and after each completion of the inner loop, a new line is outputted, creating a table format.
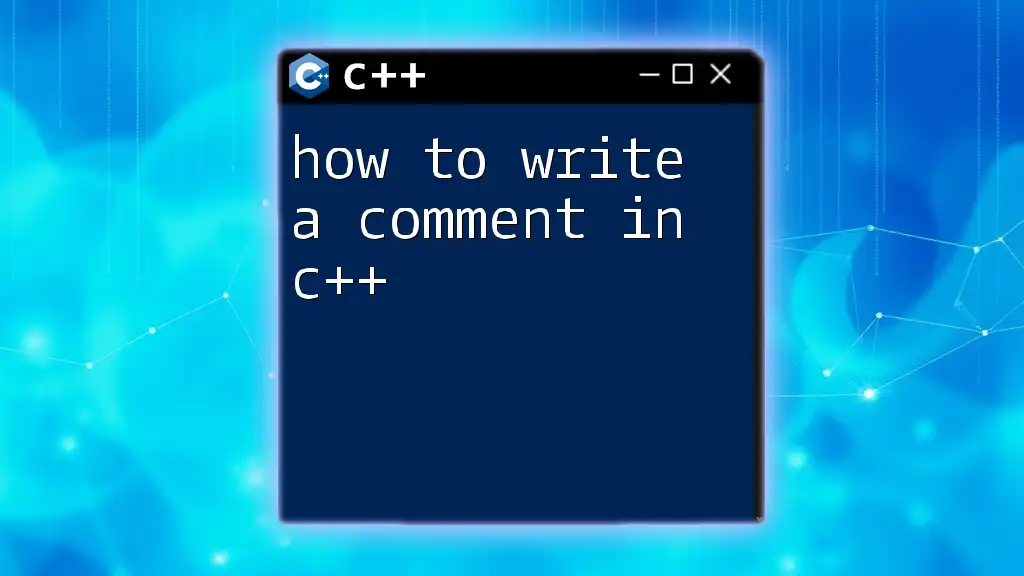
Common Errors When Working with For Loops
Off-By-One Errors
One common mistake with for loops is the off-by-one error. This happens when the loop iterates one time too many or one time too few. Here’s an example that demonstrates this issue:
for (int i = 0; i <= 5; i++) { // This should be i < 5
std::cout << i << std::endl;
}
In the above loop, including `i <= 5` means that the loop executes six times, outputting numbers 0 through 5, which may be erroneous depending on the intention.
Infinite Loops
Another common pitfall is creating infinite loops, where the loop never terminates. This typically occurs when the increment or decrement statement is omitted.
for (int i = 0; i < 5; ) { // Missing increment
std::cout << "Hello" << std::endl;
}
This loop continuously outputs "Hello" because `i` is never updated, causing the condition to remain true indefinitely.
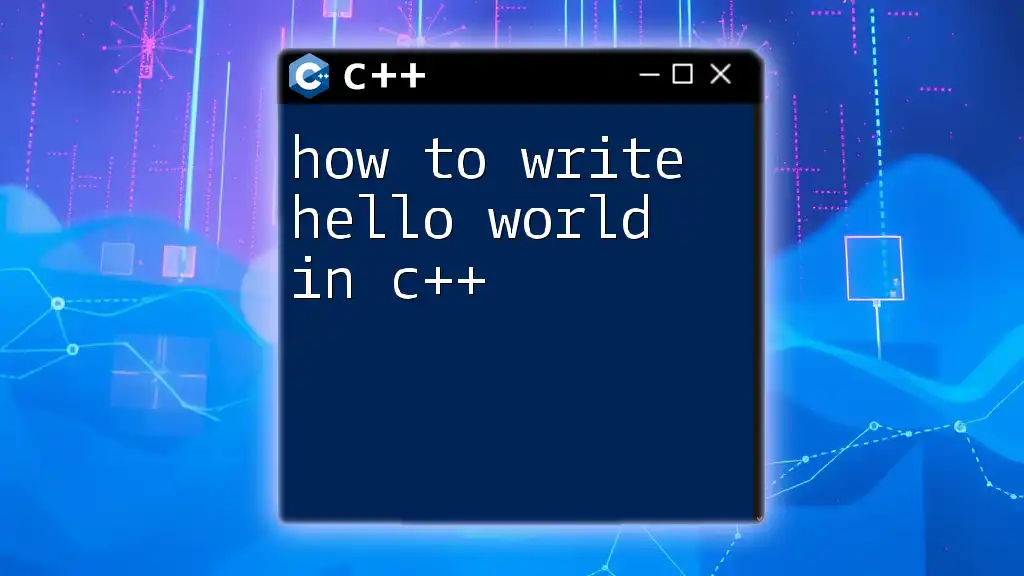
Best Practices for Using For Loops
Keep It Concise
One of the essential practices when writing for loops is to maintain conciseness. A well-structured loop should be easy to read; thus, unnecessary complications should be avoided. A clearer loop leads to easier maintenance and readability.
Avoid Complex Conditions
Avoid convoluted conditions within your loops. Complex conditions can lead to confusion and bugs in the program. If conditions become too intricate, consider breaking them out into separate functions that enhance readability.
Variable Scope
Correct variable scope is vital in for loops. It’s important to understand that any variables defined within the loop are only accessible within that block.
for (int i = 0; i < 10; i++) {
// 'i' is only accessible within this block
}
// std::cout << i; // This will cause an error
In this example, attempting to access `i` outside of the loop block will lead to an error, as it goes out of scope once the loop ends.
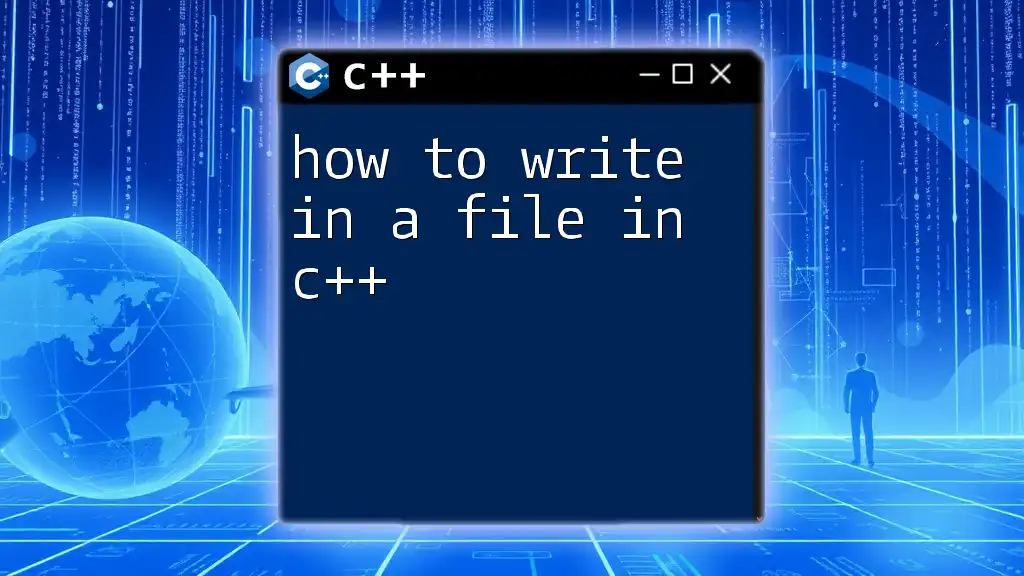
Conclusion
In this guide, we've explored how to write a for loop in C++, covering the fundamental syntax, practical examples, common pitfalls, and best practices. This foundational knowledge can significantly enhance how you approach programming in C++. As you continue to familiarize yourself with loops, remember to practice writing your own and experimenting with variations.