In C++, a `for` loop is used to execute a block of code a specific number of times, and its syntax includes initialization, condition, and increment in a single line.
Here's a code snippet demonstrating a simple `for` loop that prints numbers 1 to 5:
for (int i = 1; i <= 5; i++) {
std::cout << i << std::endl;
}
What is a For Loop?
A for loop is a control flow statement used to execute a block of code multiple times. It is particularly useful when the number of iterations is known beforehand. The loop follows a specific structure, making it concise and easy to understand. For loops are preferred in many scenarios, especially where initialization, checking a condition, and incrementing a counter variable are all done in a single line.
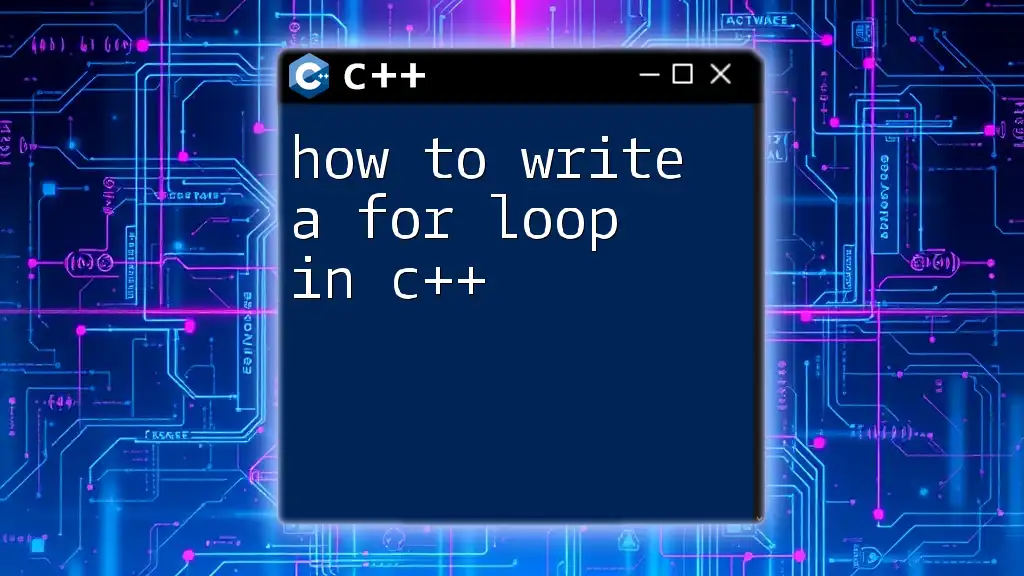
How to Use a For Loop in C++: Syntax Breakdown
The syntax for a for loop in C++ consists of three main parts: initialization, condition, and increment. The general format looks like this:
for(initialization; condition; increment) {
// code to be executed
}
Components of a For Loop
Initialization
Initialization is the first part of the for loop. It sets the starting point of the loop counter. This is crucial as it establishes the parameters of the loop. Typically, a counter variable is declared here.
Example:
for(int i = 0; i < 10; i++) {
// code block
}
In this example, `int i = 0` initializes the counter `i` to 0.
Condition
The condition is evaluated before each iteration of the loop. As long as the condition evaluates to true, the loop will continue to execute. Once the condition evaluates to false, execution of the loop will cease.
Example:
for(int i = 0; i < 10; i++) {
// code block
}
Here, `i < 10` is the condition that dictates how many times the loop will run. When `i` reaches 10, the loop terminates.
Increment/Decrement
The increment (or decrement) updates the loop variable after each iteration of the loop. This is essential to ensure that the loop progresses toward its termination condition.
Example:
for(int i = 0; i < 10; i++) {
// code block
}
In this instance, `i++` increments the value of `i` by 1 in each iteration.
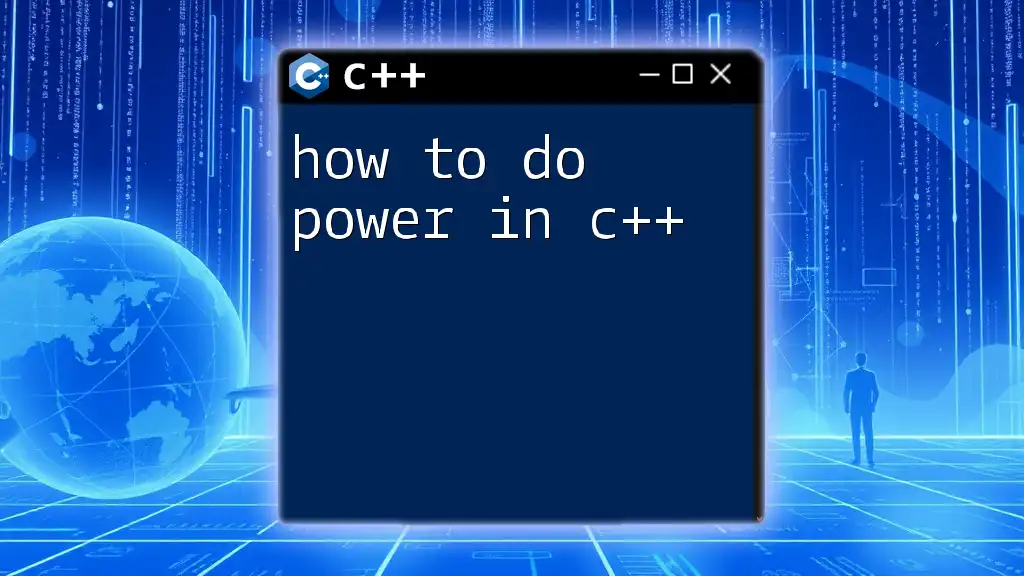
How to Use a For Loop in C++: Step-by-Step Example
Example 1: Printing Numbers from 0 to 9
Let’s look at a simple example that prints numbers from 0 to 9. The following code demonstrates this:
#include <iostream>
using namespace std;
int main() {
for(int i = 0; i < 10; i++) {
cout << i << endl;
}
return 0;
}
In this code, the for loop initializes `i` to 0 and checks if `i` is less than 10. If true, it prints `i` to the console. The counter increments by 1 after each iteration, resulting in the numbers 0 through 9 being printed.
Example 2: Summing Numbers
In this example, we’ll calculate the sum of numbers from 1 to 10:
#include <iostream>
using namespace std;
int main() {
int sum = 0;
for(int i = 1; i <= 10; i++) {
sum += i;
}
cout << "Sum: " << sum << endl;
return 0;
}
Here, we initialize a variable `sum` to 0. The for loop runs from 1 to 10, adding each integer to `sum`. After the loop completion, it prints the total sum, which is 55. This demonstrates the logic behind accumulating values within a loop.
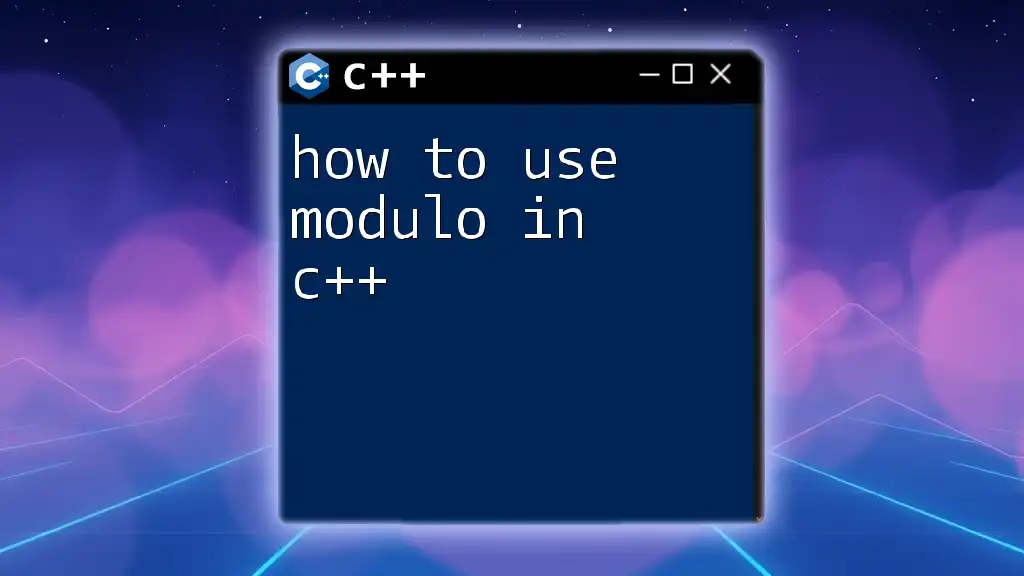
Nested For Loops in C++
Nested for loops are loops within loops, allowing for complex iteration patterns. They are particularly useful when dealing with multi-dimensional data structures, such as matrices.
For example, here’s how you might use nested for loops:
#include <iostream>
using namespace std;
int main() {
for(int i = 0; i < 3; i++) {
for(int j = 0; j < 3; j++) {
cout << "i = " << i << ", j = " << j << endl;
}
}
return 0;
}
This code snippet creates a 3x3 matrix printout. The outer loop iterates through `i`, while the inner loop iterates through `j`. The output will display every combination of `i` and `j`, emphasizing how nested for loops can efficiently handle multiple layers of iterations.
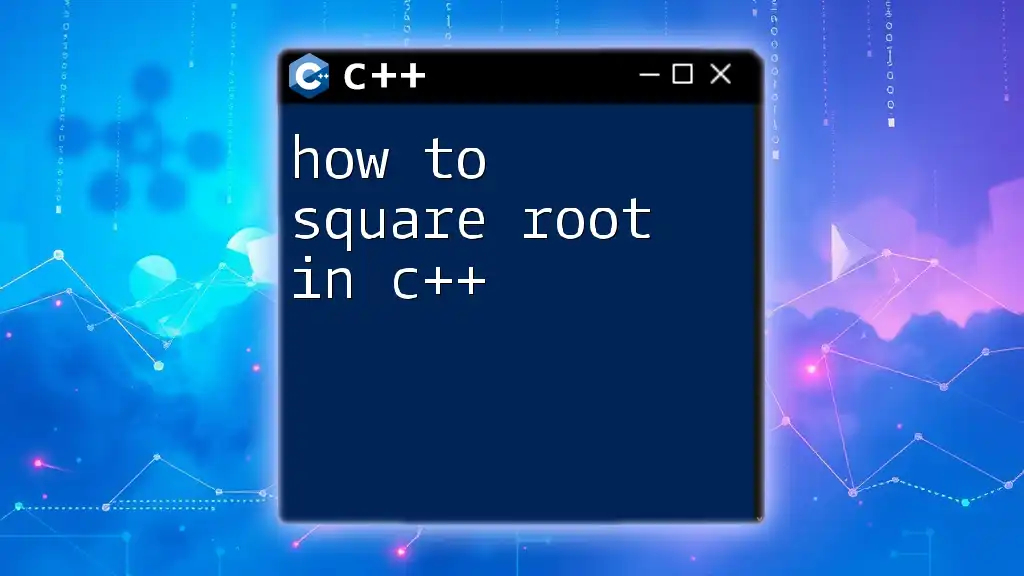
Common Mistakes to Avoid When Using For Loops
While utilizing for loops in C++, some common pitfalls can lead to errors or unexpected behavior.
-
Forgetting to update the loop variable: If you neglect to increment or update the loop variable, you might create an infinite loop that runs indefinitely.
-
Incorrect condition leading to infinite loops: Always ensure that your condition is logically sound; an incorrect condition may cause your loop to run endlessly.
-
Off-by-one errors: Pay attention to your condition. For example, using `i <= 10` versus `i < 10` can dramatically alter the number of iterations.
To illustrate a mistake, consider the following example of an infinite loop:
// Infinite loop example
for(int i = 0; i < 10;) {
cout << i << endl; // This will repeatedly print 0
}
In this case, the loop lacks an increment statement, causing it to execute indefinitely as `i` remains 0.
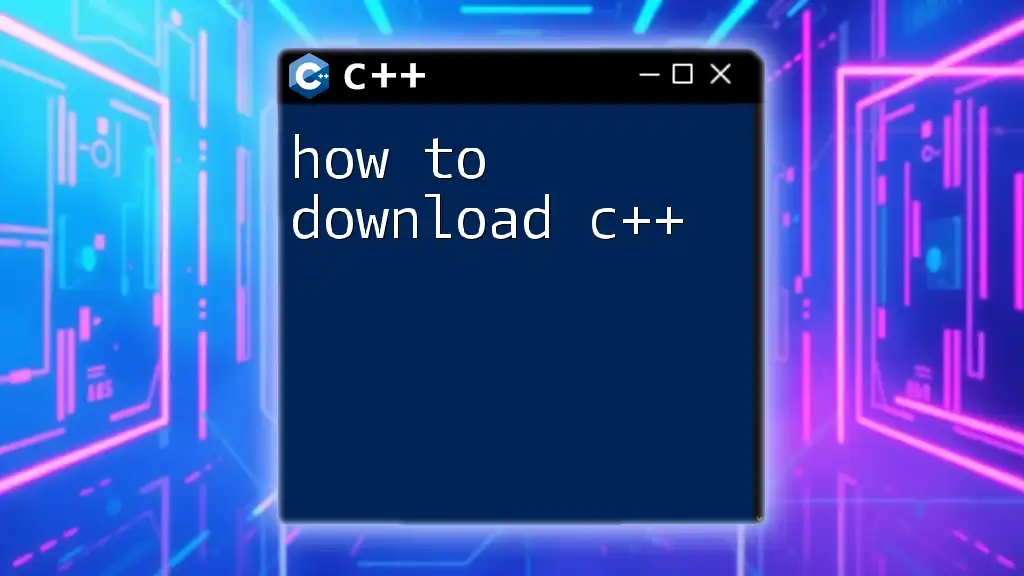
Best Practices for Using For Loops in C++
To write efficient and effective for loops, consider the following best practices:
-
Minimize computations inside the loop: Avoid placing heavy calculations inside the loop, as they can slow down execution. Instead, precompute results when possible.
-
Use meaningful variable names: Clearly naming your loop variables can clarify the intent and context of your loop, making your code easier to read and maintain.
-
Keep it simple: Aim for clarity in your loops. If it becomes overly complex, consider breaking it into smaller functions.
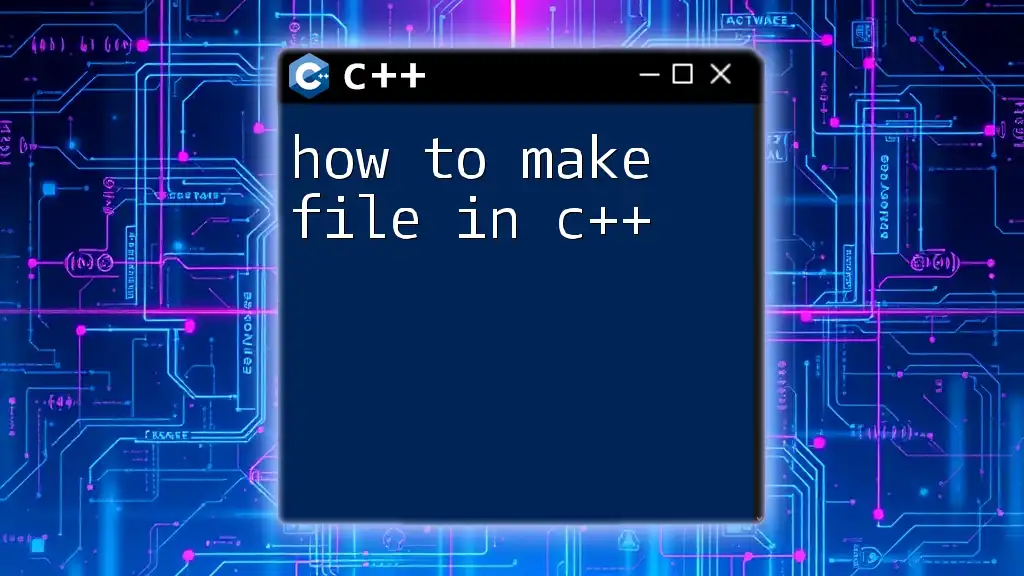
Conclusion
In summary, mastering the for loop in C++ is essential for any programmer. Its structure allows for clear and efficient handling of repetitive tasks. By understanding how to do a for loop in C++ through initialization, conditions, and increments, alongside practical examples, you can effectively utilize loops in your coding practice.
As you grow in your programming journey, actively seek to practice these concepts and apply them in various scenarios. Keep coding, and you will excel!
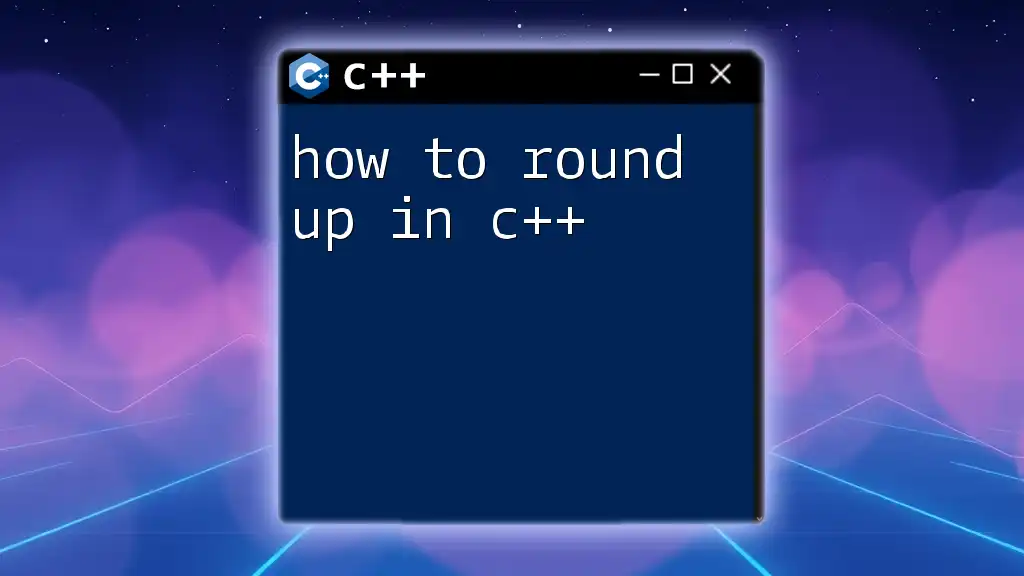
Call to Action
We encourage you to subscribe to our blog for more programming tips, examples, and exercises that will deepen your understanding of C++ and enhance your coding skills. If you have questions or would like to share your experiences with for loops, feel free to leave a comment below!