To sort a vector in C++, you can utilize the `std::sort` function from the `<algorithm>` header to arrange its elements in ascending order. Here’s how to do it:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {4, 1, 3, 9, 7};
std::sort(vec.begin(), vec.end());
for (int num : vec) {
std::cout << num << " ";
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
A vector in C++ is a dynamic array provided by the Standard Template Library (STL). Unlike traditional arrays, vectors can change size dynamically as elements are added or removed. This characteristic makes them incredibly flexible and efficient for various programming tasks.
Common Use Cases for Vectors
Vectors are beneficial in various situations, such as:
- Dynamic sizing: You can easily add and remove items without worrying about preset sizes.
- Efficient insertion and deletion at both ends of the container.
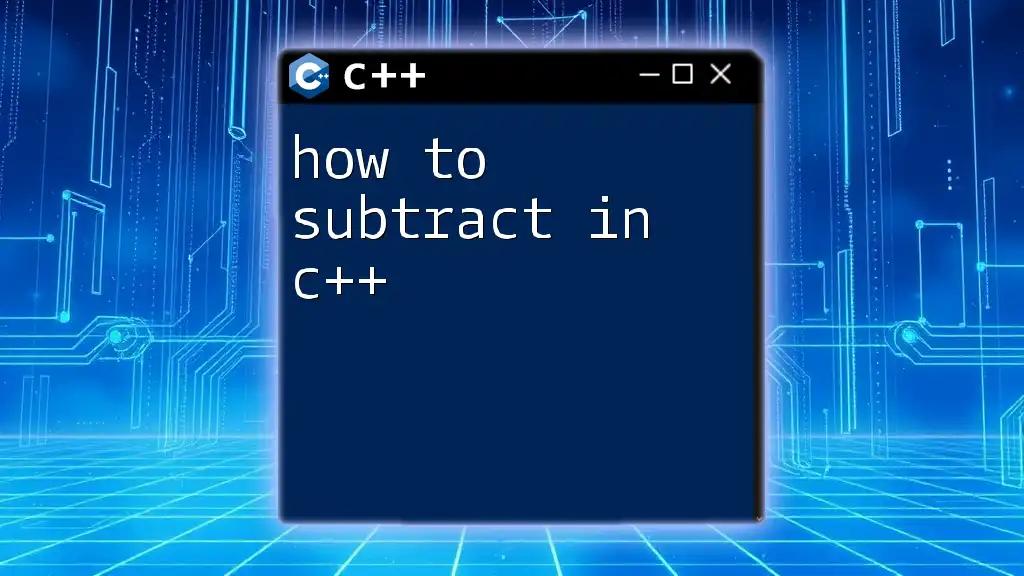
The Basics of Sorting in C++
What is Sorting?
Sorting refers to the process of arranging elements in a particular order, typically either ascending or descending. This fundamental concept is crucial for efficient data retrieval and processing.
Why Sort a Vector?
Sorting helps improve the performance of other operations such as searching and merging datasets. Sorted data allows algorithms to operate more efficiently, reducing time complexity and improving overall program performance. Real-world applications range from quick data analysis to organizing information in databases.

How to Sort a Vector in C++
Using the Standard Library
Introduction to `std::sort`
C++ provides a built-in function called `std::sort`, which sorts the elements in a range. It's part of the `<algorithm>` header and is highly optimized for performance.
Basic Syntax of `std::sort`
Here’s a simple example of using `std::sort`:
#include <algorithm>
#include <vector>
std::vector<int> numbers = {5, 3, 8, 1, 4};
std::sort(numbers.begin(), numbers.end());
Detailed Step-by-Step Guide
Step 1: Include Necessary Headers
To use `std::sort`, you must include the `<algorithm>` header:
#include <algorithm>
Step 2: Initialize Your Vector
You can initialize your vector with a set of integers, for example:
std::vector<int> myVector = {7, 2, 6, 4, 1};
Step 3: Call `std::sort()`
Invoke the `std::sort` function. The parameters `myVector.begin()` and `myVector.end()` specify the range of elements to be sorted:
std::sort(myVector.begin(), myVector.end());
Step 4: Resulting Sorted Vector
After sorting, you might want to display the sorted vector. Here’s how you can print the elements:
for (int num : myVector) {
std::cout << num << " ";
}

Sorting in Different Orders
Sorting in Ascending Order
The default behavior of `std::sort` is to sort in ascending order. For the example provided in the previous section, the sorted output will now be:
1 2 4 6 7
Sorting in Descending Order
To sort in descending order, you'll need to provide a custom comparison function. You can use `std::greater<int>()` from the `<functional>` header:
#include <functional> // Include for std::greater
std::sort(myVector.begin(), myVector.end(), std::greater<int>());

Custom Sorting Criteria
Introduction to Lambda Functions
With C++, you have the capability to define lambda functions, which are essentially unnamed functions defined at the point of use. They can be very useful for specifying custom sorting conditions.
Example of Custom Sort with Lambda
You can implement a custom sort by passing a lambda function to `std::sort`. Below is an example of sorting a vector in a custom way:
std::sort(myVector.begin(), myVector.end(), [](int a, int b) {
return a < b; // Custom criteria: Sort in ascending order
});

Performance Considerations
Time Complexity of `std::sort`
`std::sort` is highly efficient, with an average time complexity of O(n log n). However, in the worst-case scenario, it could degrade to O(n²) for certain types of data.
When to Use `std::sort()` vs. Other Sorting Methods
While `std::sort()` is usually the best choice due to its efficiency, sometimes `std::stable_sort()` may be preferable. The latter preserves the relative order of equal elements, making it suitable for certain applications.

Conclusion
In this guide, we've thoroughly explored how to sort a vector in C++ using the powerful `std::sort` functionality. We discussed the preparation needed, how to implement sorting, customize sorting criteria, and considered the performance aspects. Sorting vectors is an essential skill that enhances data manipulation capabilities in C++. The next step is to practice sorting vectors with diverse datasets to solidify your understanding further.

Additional Resources
Books and Online Courses
Explore literature and courses dedicated to C++ and sorting algorithms to deepen your knowledge.
Community Forums and Documentation
Engaging with communities focused on C++ can provide additional insights, coding tips, and collaboration opportunities.

Frequently Asked Questions (FAQs)
What is the difference between `std::sort` and `std::stable_sort`?
`std::sort` does not guarantee the relative order of equal elements, while `std::stable_sort` preserves it.
How do I sort a vector of objects or structures?
You can sort a vector of objects by defining a custom comparison function that specifies the sorting criteria based on object properties.
Can I sort vectors of strings or characters?
Yes, `std::sort` can sort vectors of strings and characters in the same way it sorts integers.
How does sorting affect the performance of my program?
Sorting can be a computationally intensive operation; however, efficient sorting helps improve performance in subsequent operations like searching.