To sort an array in C++, you can use the `std::sort` function from the `<algorithm>` header, which efficiently arranges the elements in ascending order. Here's a code snippet demonstrating this:
#include <iostream>
#include <algorithm>
int main() {
int arr[] = {5, 3, 8, 1, 2};
int n = sizeof(arr) / sizeof(arr[0]);
std::sort(arr, arr + n);
for (int i = 0; i < n; i++)
std::cout << arr[i] << " ";
return 0;
}
Understanding Array Sorting in C++
What is Array Sorting?
Sorting an array involves rearranging its elements into a specific order, often ascending or descending. This is fundamental in programming as sorted data can be more efficiently searched and processed. For example, sorting is essential when implementing search algorithms, on data visualizations, and when preparing data for statistical analyses.
Array sorting performs a critical function in various applications, from simple data sorting tasks to complex machine learning algorithms, where sorted arrays can enhance the performance of data manipulation significantly.
Types of Sorting Algorithms
There are several algorithms used to sort arrays, each with its strengths and weaknesses. Here are a few of the most commonly employed sorting techniques:
-
Bubble Sort: A simple comparison-based algorithm. It repeatedly steps through the array, compares adjacent elements, and swaps them if they are in the wrong order. It has a best-case performance of O(n) and a worst-case of O(n²).
-
Selection Sort: This algorithm divides the array into a sorted and unsorted part. It repeatedly selects the smallest (or largest) element from the unsorted subarray and moves it to the sorted section. Its time complexity is O(n²).
-
Insertion Sort: Builds a sorted array one element at a time by comparing and inserting elements into their correct position. Average and worst-case time complexity is O(n²), but it performs well on smaller or mostly sorted datasets.
-
Quick Sort: A highly efficient, comparison-based sorting algorithm that uses a divide-and-conquer approach. Time complexity averages O(n log n) but has a worst-case of O(n²) if not carefully implemented.
-
Merge Sort: This algorithm also uses the divide-and-conquer strategy. It breaks the array into smaller subarrays, sorts them, and then merges them back together. It performs consistently well with a time complexity of O(n log n).
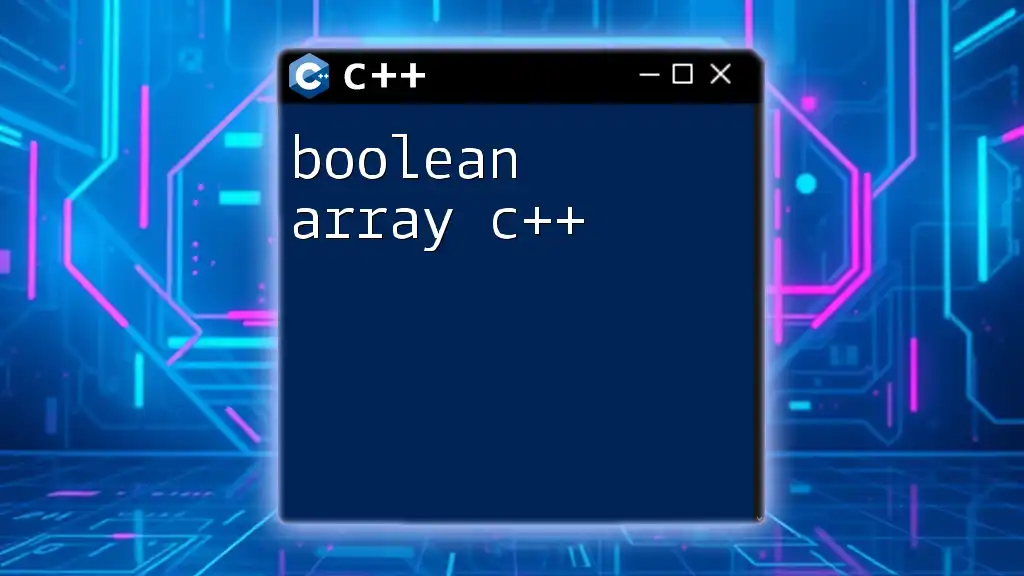
Setting Up Your C++ Environment
Getting Started with C++
Before diving into writing code to sort an array in C++, you need an appropriate development environment. Popular IDEs for C++ include:
- Microsoft Visual Studio: Ideal for Windows users, featuring a robust set of development tools.
- Code::Blocks: A lightweight, cross-platform IDE suitable for beginners.
- CLion: An advanced IDE by JetBrains for professional development.
To run C++ code, ensure you have the necessary compilers installed (e.g., MinGW for Windows or g++ on Unix-based systems). Once your environment is set up, you'll be ready to write your first sorting algorithm.
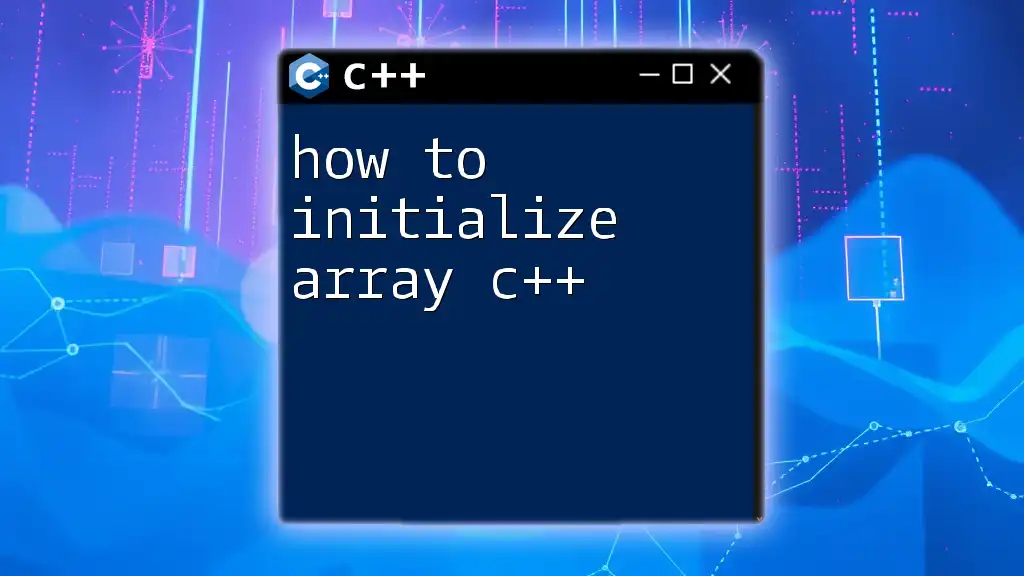
How to Sort an Array in C++
Using the Standard Library's `std::sort` Function
The simplest way to sort an array in C++ is by using the `std::sort` function from the `algorithm` header. This function is optimized and leverages powerful sorting algorithms behind the scenes.
Here's how you can use `std::sort`:
#include <iostream>
#include <algorithm> // Header for std::sort
int main() {
int arr[] = {5, 2, 9, 1, 5, 6};
int n = sizeof(arr) / sizeof(arr[0]);
std::sort(arr, arr + n);
std::cout << "Sorted array: ";
for (int i = 0; i < n; ++i) {
std::cout << arr[i] << " ";
}
return 0;
}
In this example, `std::sort` takes two parameters: the beginning and the ending pointer of the range to be sorted. After sorting, the array will be displayed in ascending order.
Implementing Custom Sorting Functions
Sometimes, you might want to sort an array based on custom criteria. You can easily achieve this by defining a custom comparison function.
For instance, if you want to sort in descending order, you can do it like this:
bool customCompare(int a, int b) {
return a > b; // Sorts in descending order
}
int main() {
int arr[] = {5, 2, 9, 1, 5, 6};
int n = sizeof(arr) / sizeof(arr[0]);
std::sort(arr, arr + n, customCompare);
std::cout << "Sorted array in descending order: ";
for (int i = 0; i < n; ++i) {
std::cout << arr[i] << " ";
}
return 0;
}
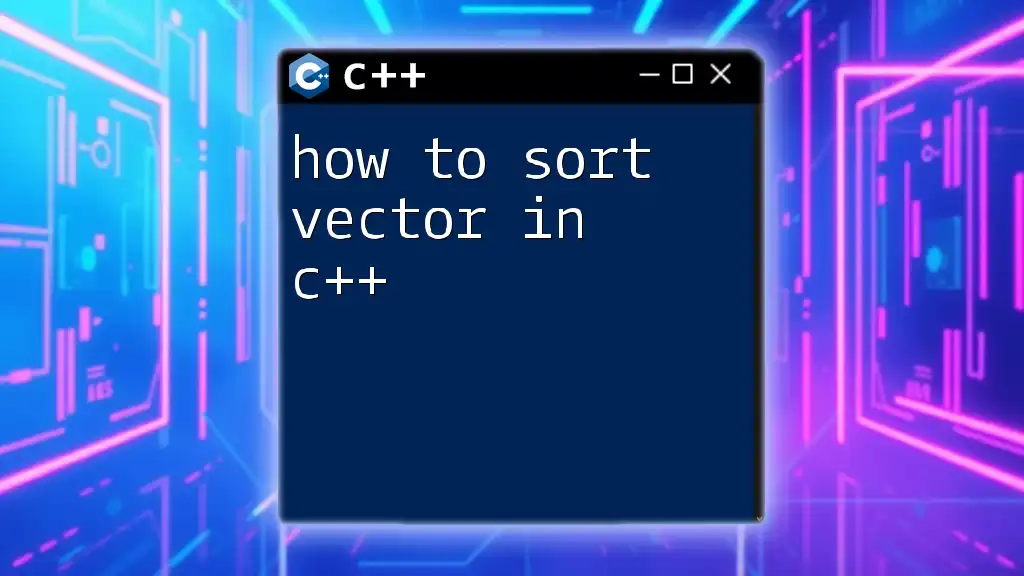
Manual Implementation of Sorting Algorithms
Bubble Sort Algorithm
The Bubble Sort algorithm is one of the simplest sorting techniques. Here's how it works:
- Repeat for the entire array.
- Compare each pair of adjacent elements and swap them if they are in the wrong order.
- Continue this process until no swaps are required, indicating that the array is sorted.
Here's an implementation of Bubble Sort:
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
std::swap(arr[j], arr[j + 1]);
}
}
}
}
Pros and cons of using Bubble Sort:
While easy to implement, its time complexity makes it inefficient for large lists, making it more suitable for small datasets.
Selection Sort Algorithm
Selection Sort works by repeatedly selecting the smallest or largest element from the unsorted segment and moving it to the sorted segment.
void selectionSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
std::swap(arr[minIndex], arr[i]);
}
}
Pros and cons of using Selection Sort:
Though not the fastest method (time complexity of O(n²)), it has the advantage of doing minimal swaps, which could be beneficial when dealing with larger data types.
Insertion Sort Algorithm
Insertion Sort builds a sorted array one number at a time. It works well for small data sets and is adaptive, meaning it performs better on already sorted data.
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
Pros and cons of using Insertion Sort:
This algorithm is efficient for small datasets (O(n) when the array is mostly sorted) but becomes slow with larger lists.
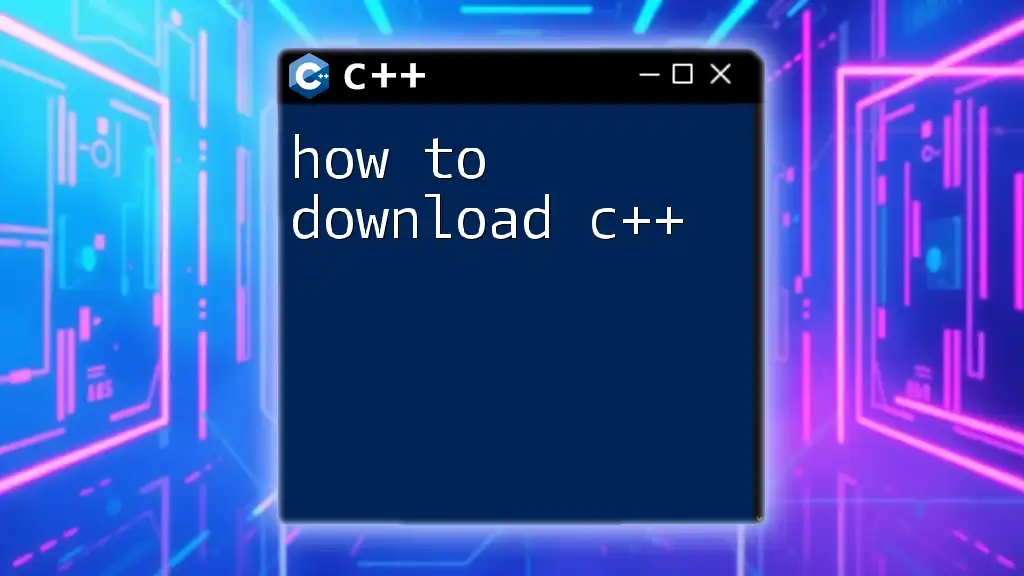
More Advanced Sorting Algorithms
Introduction to Quick Sort
Quick Sort is a divide-and-conquer algorithm. The basic idea is to select a 'pivot' element from the array and partition the other elements into two sub-arrays. Here's how to implement it:
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
std::swap(arr[i], arr[j]);
}
}
std::swap(arr[i + 1], arr[high]);
return (i + 1);
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
Pros and cons of using Quick Sort:
It is generally faster and requires less space than other algorithms, but its worst-case performance can degrade to O(n²) if not implemented with care.
Introduction to Merge Sort
Merge Sort is another divide-and-conquer algorithm. It divides the array into halves, sorts the halves, and merges them back together. An example implementation would look like this:
void merge(int arr[], int left, int mid, int right) {
int i, j, k;
int n1 = mid - left + 1;
int n2 = right - mid;
int *L = new int[n1], *R = new int[n2];
for (i = 0; i < n1; i++)
L[i] = arr[left + i];
for (j = 0; j < n2; j++)
R[j] = arr[mid + 1 + j];
i = 0;
j = 0;
k = left;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k] = L[i];
i++;
} else {
arr[k] = R[j];
j++;
}
k++;
}
while (i < n1) {
arr[k] = L[i];
i++;
k++;
}
while (j < n2) {
arr[k] = R[j];
j++;
k++;
}
delete[] L;
delete[] R;
}
void mergeSort(int arr[], int left, int right) {
if (left < right) {
int mid = left + (right - left) / 2;
mergeSort(arr, left, mid);
mergeSort(arr, mid + 1, right);
merge(arr, left, mid, right);
}
}
Pros and cons of using Merge Sort:
It has a stable O(n log n) performance, which makes it appealing for large datasets, but it can use additional memory for merging.
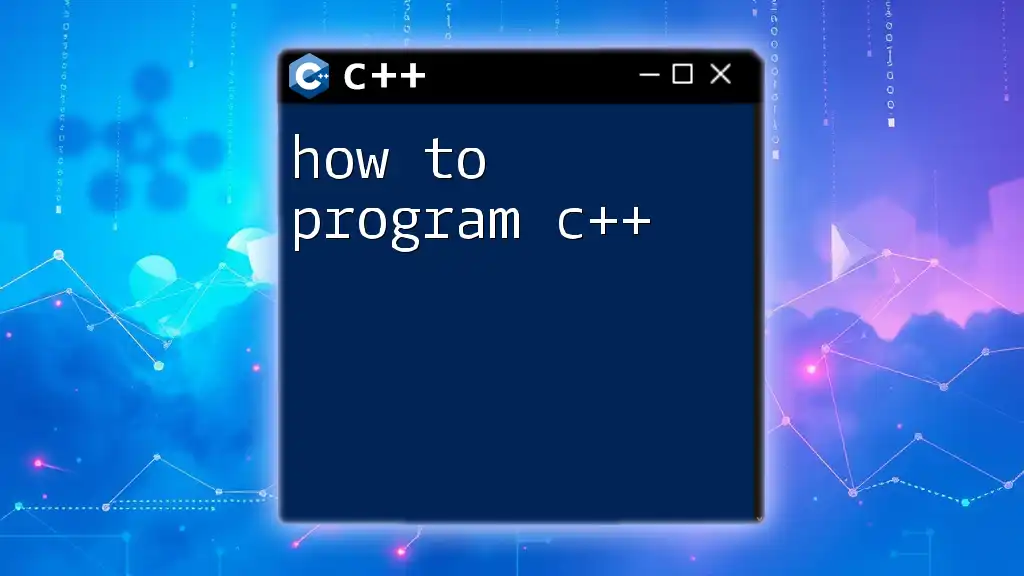
Performance Comparison of Sorting Algorithms
Time Complexity Breakdown
Understanding the time complexity of sorting algorithms is crucial:
- Bubble Sort: O(n²) – inefficient for large datasets.
- Selection Sort: O(n²) – better than Bubble Sort in terms of swaps.
- Insertion Sort: O(n²) – efficient for smaller or partially sorted arrays.
- Quick Sort: Average O(n log n), worst-case O(n²).
- Merge Sort: O(n log n) – consistently good performance.
Space Complexity Overview
Most algorithms operate in-place, but some, like Merge Sort, require additional space, making it essential to balance time and space efficiency depending on the intended use case.
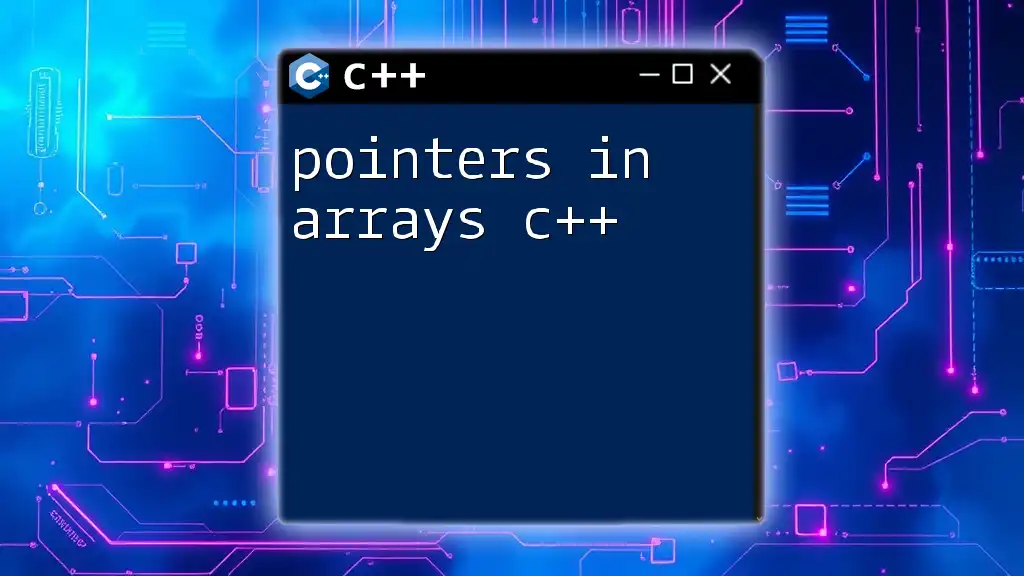
Conclusion
Sorting is a fundamental concept in programming, especially within C++. Understanding how to sort an array in C++ not only enhances your knowledge of data structures but also equips you with the skills to write more efficient and optimized programs. By choosing the right sorting algorithm and applying C++ functionalities effectively, you’ll streamline your data processing tasks.
Exploring different algorithms not only builds your programming arsenal but also gives insight into how you can optimize performance across various applications. With practice and experimentation, you can become adept at implementing sorting algorithms, enhancing your problem-solving skills within the realm of programming.
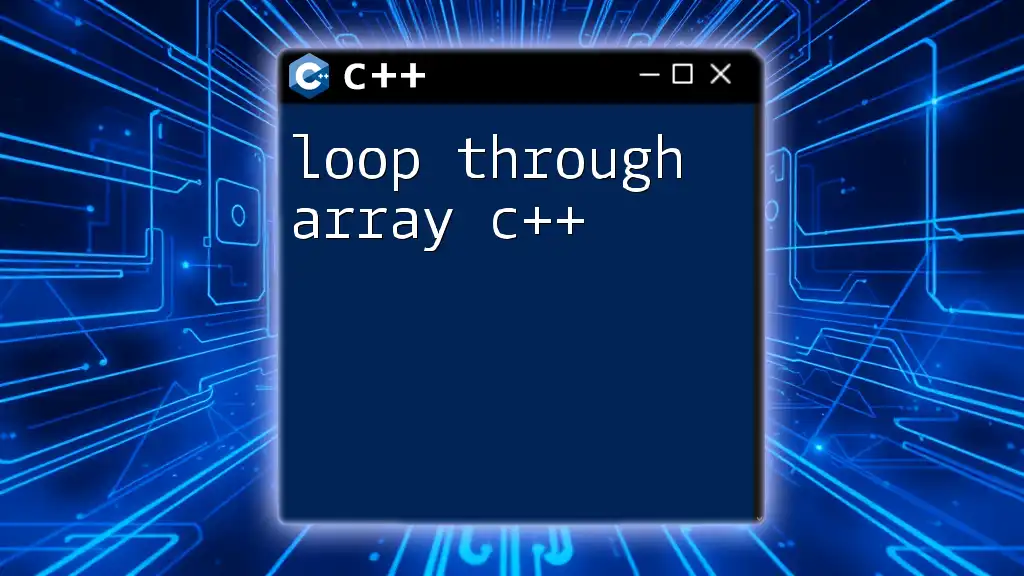
Additional Resources
To further expand your knowledge on C++ and sorting algorithms, consider browsing through:
- Books: "C++ Primer" by Lippman, "Effective C++" by Scott Meyers
- Online Courses: Platforms like Coursera, Udacity, or Khan Academy offer C++ programming courses.
- Documentation: The C++ Standard Library documentation contains in-depth explanations and examples.
Engage with these resources, and continue growing your expertise with C++ and algorithms!