In C++, you can loop through an array using a `for` loop to access each element in sequence, as shown in the following code snippet:
#include <iostream>
int main() {
int arr[] = {1, 2, 3, 4, 5};
for(int i = 0; i < 5; i++) {
std::cout << arr[i] << " ";
}
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a collection of items stored at contiguous memory locations. This means that each element in an array can be accessed by its index, making data manipulation efficient. Arrays are essential structures in C++ that allow programmers to store multiple values in a single, organized format.
Characteristics of Arrays include:
- Fixed Size: Once an array is declared, its size is determined and cannot be changed dynamically.
- Homogeneous Data Types: All elements in the array must be of the same data type, such as all integers or all floating-point numbers.
Declaring and Initializing Arrays
In C++, declaring an array involves specifying the type of its elements and its size. Here’s how you can declare an integer array:
int arr[5];
You can also initialize an array at the time of declaration. Here are two methods:
- Uniform Initialization: This lets you provide the values directly.
int arr[5] = {1, 2, 3, 4, 5};
2. **Initialization Without Size**: If you don't specify the size, C++ will count the number of initialized elements automatically.
```cpp
int arr[] = {1, 2, 3, 4, 5};
It’s important to remember that accessing elements outside the bounds of the array can lead to undefined behavior.
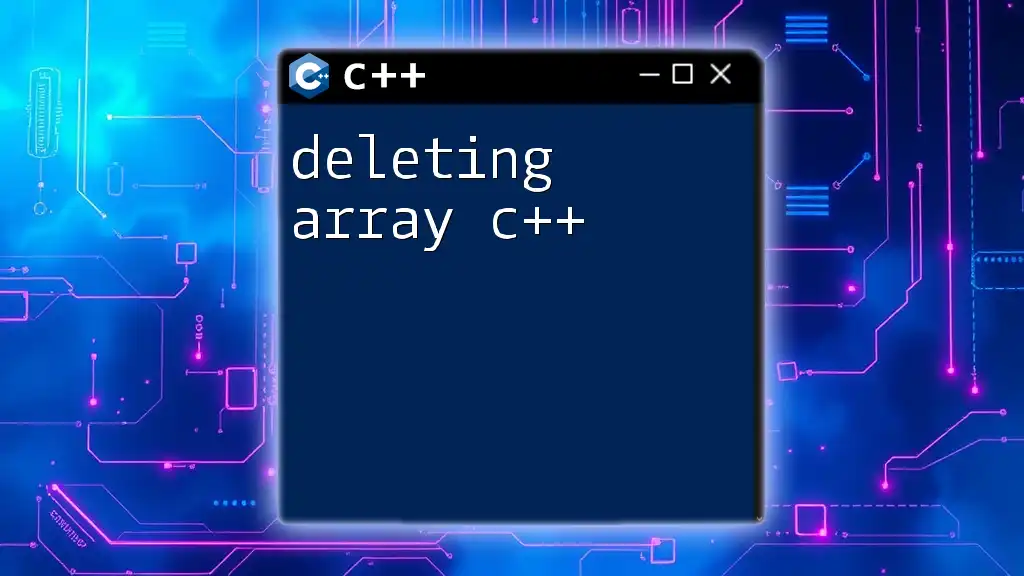
The Basics of Looping Through Arrays in C++
The Need for Looping
Looping through arrays is crucial for data processing. Whether you’re summing values, searching for an item, or modifying the array contents, loops enable this functionality. They provide the control needed to iterate over each element, making it possible to apply operations quickly and efficiently.
Types of Loops in C++
C++ offers several loop constructs including `for`, `while`, and `do-while`. Each loop has its own syntax and use cases, but here we'll focus primarily on the `for` loop.
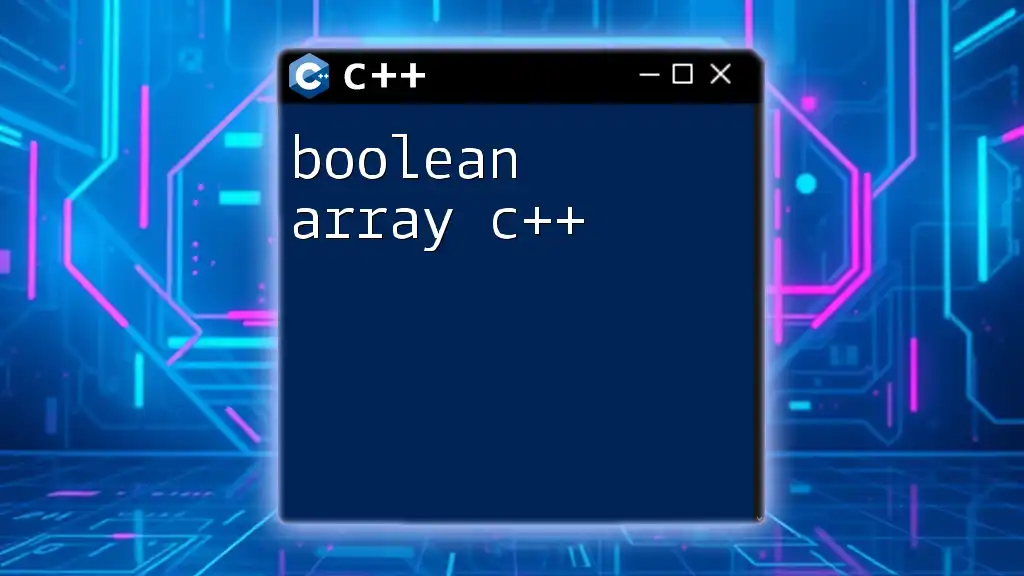
Looping Through Arrays: The For Loop
Using the C++ For Loop Array
The `for` loop is one of the most common methods to iterate through arrays. Its syntax is quite straightforward:
for(initialization; condition; increment) {
// Statements to execute
}
Example: Looping Through an Array
Here's a simple example demonstrating how to loop through an array using a `for` loop:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
for(int i = 0; i < 5; i++) {
cout << arr[i] << " ";
}
return 0;
}
In this code:
- The loop initializes `i` to `0`.
- It checks the condition `i < 5` to ensure that it doesn’t exceed the array limits.
- It increments `i` with each iteration, allowing you to access each element sequentially.
Looping with Dynamic Arrays
Dynamic arrays in C++ allow for more flexibility by using the `new` keyword to allocate memory at runtime. This advantage is especially useful when the size of the array is not known during compilation. Here's how to work with a dynamic array:
#include <iostream>
using namespace std;
int main() {
int n;
cout << "Enter number of elements: ";
cin >> n;
int* arr = new int[n];
for(int i = 0; i < n; i++) {
cin >> arr[i];
}
for(int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
delete[] arr; // Important to free memory
return 0;
}
In this code:
- Memory is dynamically allocated using `new`.
- It is crucial to use `delete[]` to free the allocated memory, preventing memory leaks—a common concern with dynamic arrays.
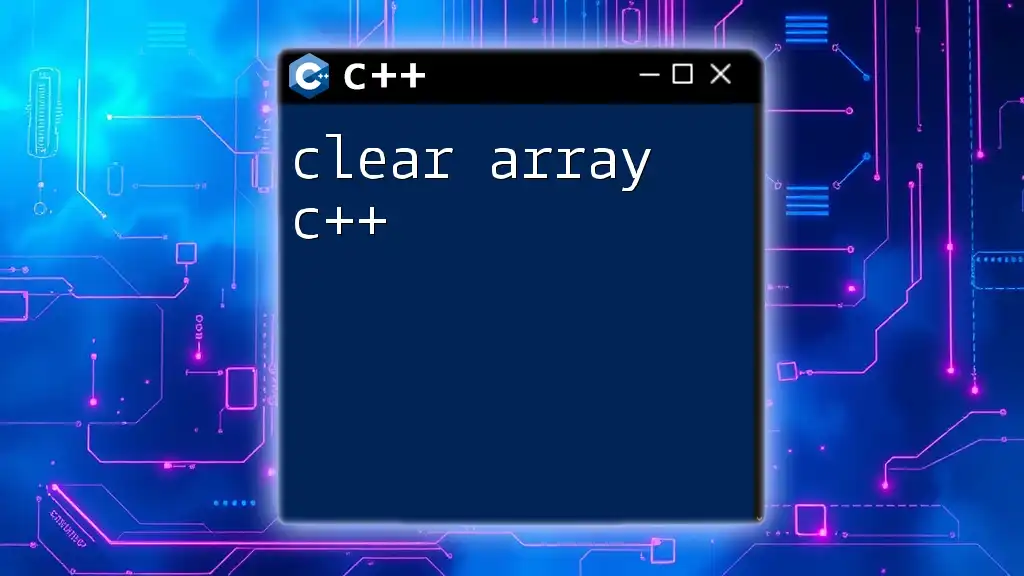
Looping Through Arrays with Range-Based For Loops
What is a Range-based For Loop?
Introduced in C++11, the range-based for loop simplifies the process of iterating over arrays and other collections. It eliminates the need for an index and makes the code cleaner and more readable.
Example of Range-based For Loop
You can use a range-based for loop as follows:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
for(int num : arr) {
cout << num << " ";
}
return 0;
}
In this example:
- Each element in the array `arr` is accessed directly through the variable `num`, making the code visually simpler and less error-prone.
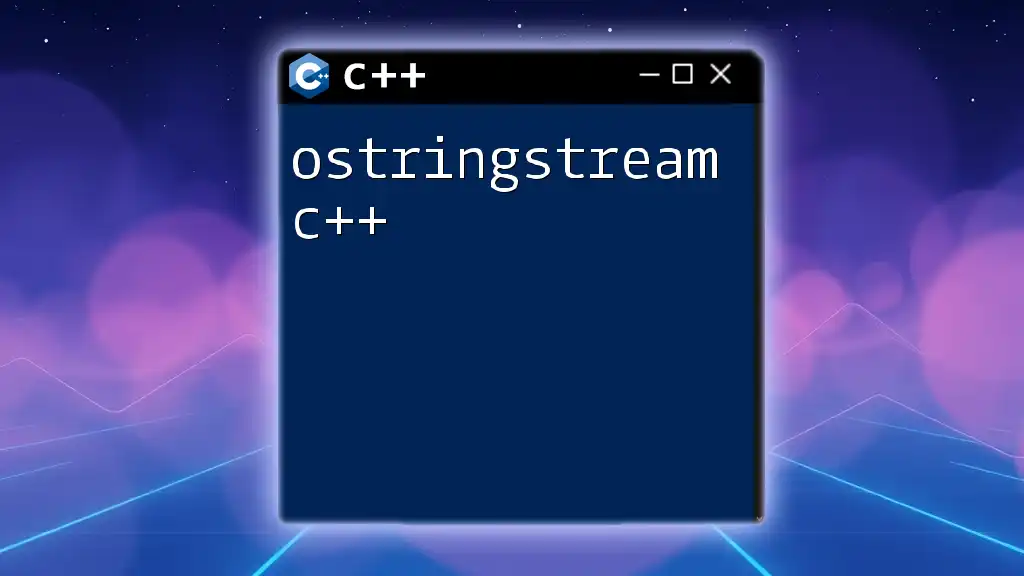
Looping Arrays in Different Scenarios
Summing Elements in an Array
Looping can also be used to perform calculations, such as summing all elements in an array. Here’s a quick snippet demonstrating how to accomplish this:
int sum = 0;
for(int i = 0; i < size; i++) {
sum += arr[i];
}
Searching for an Element in an Array
Another common operation is searching for a specific element in an array. Here’s how you can implement a simple search function:
bool findElement(int arr[], int size, int target) {
for(int i = 0; i < size; i++) {
if(arr[i] == target) return true;
}
return false;
}
Modifying Elements in an Array
You can easily modify elements within an array. For instance, the following code demonstrates how to increment each element by 1:
for(int i = 0; i < size; i++) {
arr[i] += 1; // Increment each element by 1
}
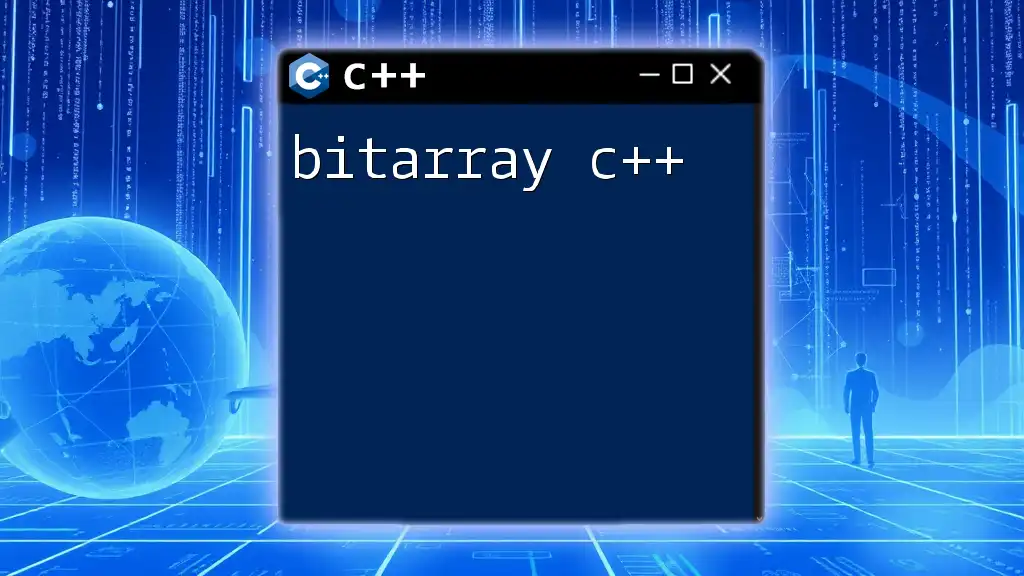
Common Errors When Looping Through Arrays
Out-of-Bounds Access
One of the most critical issues when working with arrays is accessing indices that do not exist. This can lead to undefined behavior and hard-to-trace bugs. Always ensure that the index you are accessing is within the array boundaries.
Infinite Loops
An infinite loop may occur if the loop condition is never met. This can happen due to a mistake in the ending condition or increment operation. Always debug your loop conditions to ensure they terminate as expected.
Uninitialized Arrays
Attempting to loop through an uninitialized array can result in accessing garbage values. Ensure that all arrays are properly initialized before use to avoid unexpected outcomes.
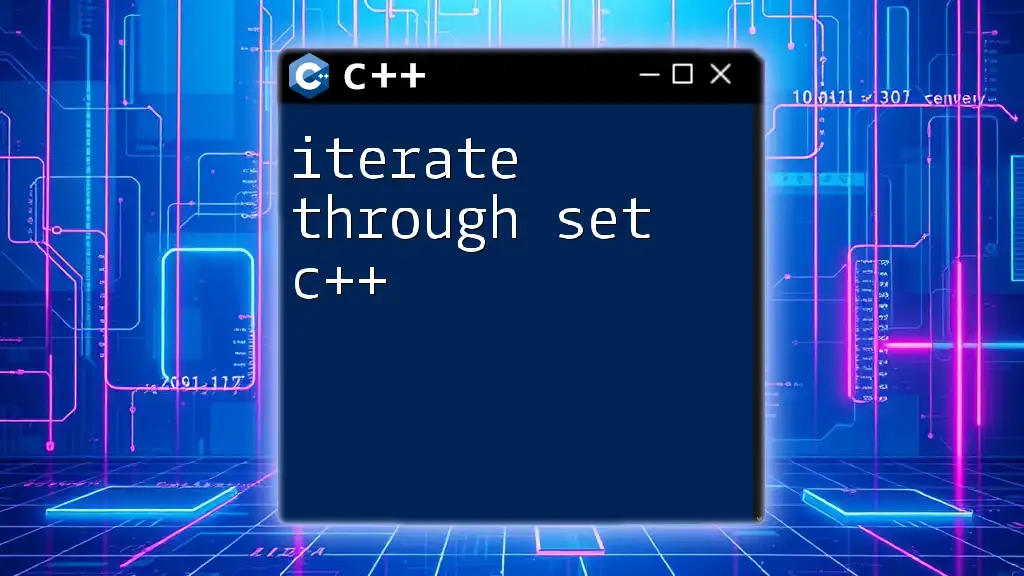
Best Practices for Looping Through Arrays
- Use range-based for loops whenever applicable to enhance readability and reduce the risk of off-by-one errors.
- Always check array bounds before accessing elements to prevent crashes or unintended behaviors.
- Avoid hardcoding the sizes; use constants or variables to make your code adaptable.
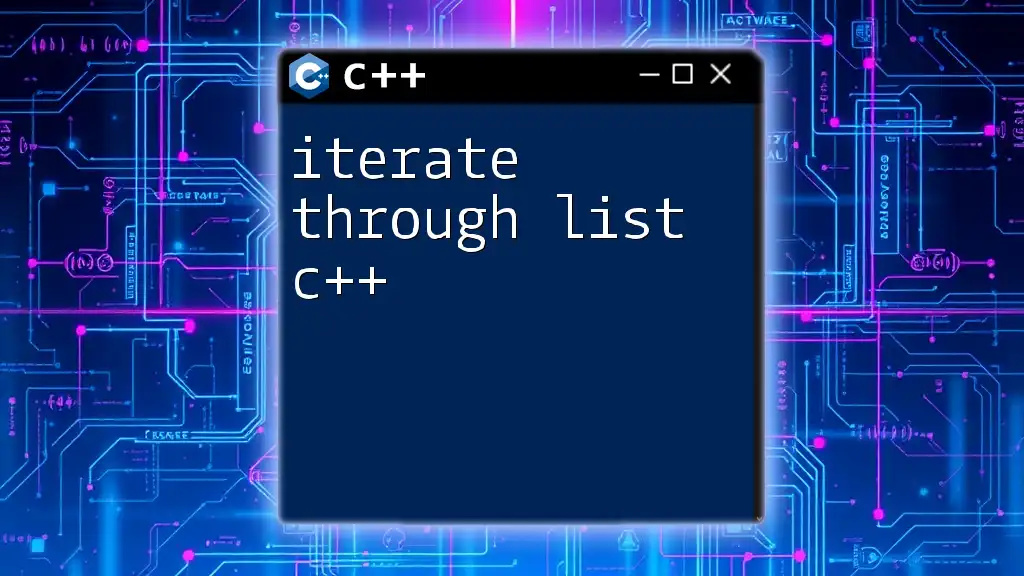
Conclusion
Looping through arrays in C++ is an indispensable skill for efficient data manipulation. With various loop constructs available, you can easily traverse and operate on arrays to meet your programming needs. As you practice and become comfortable with these techniques, you'll discover that efficient data management forms the backbone of effective programming.
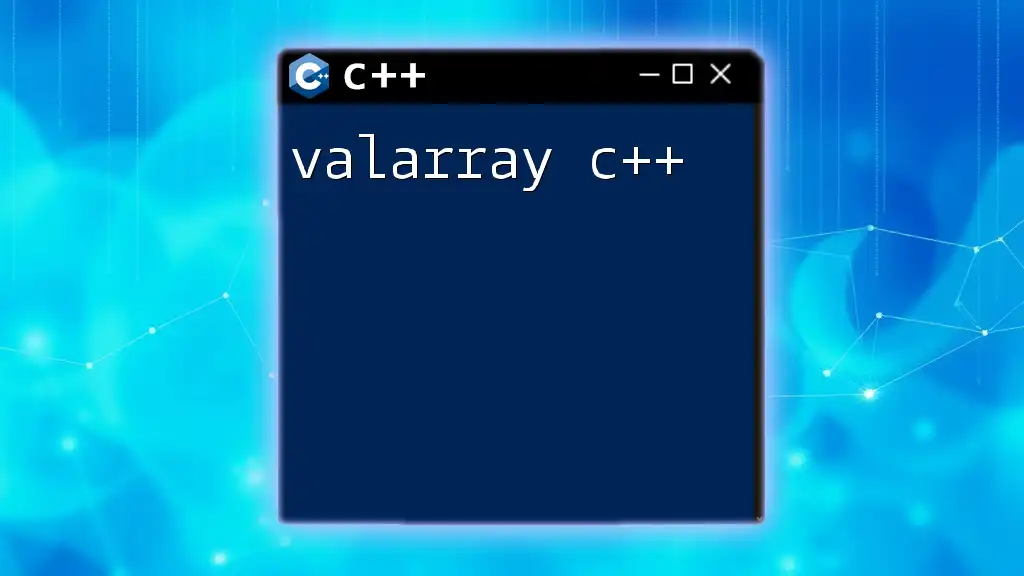
Further Reading and Resources
Continued learning is vital. You may wish to explore additional resources about arrays and loops, including:
- Online tutorials and documentation for C++.
- Books specifically focused on C++ programming concepts.
- Courses covering advanced data structures and algorithms in C++.