To properly free the memory allocated for a dynamic array in C++, use the `delete[]` operator to avoid memory leaks. Here's a code snippet demonstrating this:
int* dynamicArray = new int[10]; // Dynamically allocate an array of 10 integers
// ... (use the array)
delete[] dynamicArray; // Free the dynamically allocated array
Understanding Dynamic Arrays in C++
What is a Dynamic Array?
A dynamic array is an array that is allocated at runtime using the `new` operator. Unlike static arrays, which are fixed in size and determined at compile time, dynamic arrays offer the flexibility of resizing as needed. This capability makes dynamic arrays particularly useful when the size of the array cannot be predetermined.
Dynamic arrays differ fundamentally from static arrays in terms of memory allocation. While static arrays utilize stack memory, dynamic arrays are allocated on the heap, which provides more extensive storage capacity and allows for more complex data structures. Common use cases for dynamic arrays include situations where the required array size can change during program execution, such as when reading data from user input or processing variable-length data.
Memory Management in C++
Memory management is crucial in C++ programming due to its ability to use both stack and heap memory. The stack is used for static memory allocation, while the heap is reserved for dynamic memory allocation. C++ gives developers the power to allocate and deallocate memory as needed, but with that power comes the responsibility of managing that memory properly.
When using dynamic arrays, especially, it is vital to understand that failing to release memory can lead to memory leaks. Conversely, improper deletion can cause undefined behavior and crashes. Thus, proper memory management practices are essential for writing stable and efficient C++ applications.
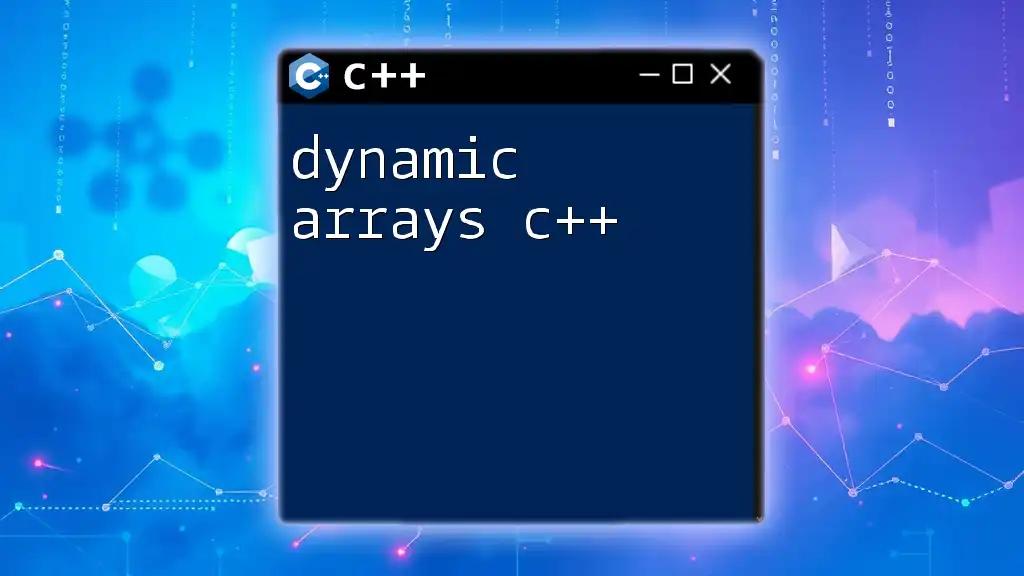
Creating Dynamic Arrays in C++
Syntax for Dynamic Array Creation
Creating a dynamic array in C++ involves using the `new` operator followed by the data type and the size of the array you want to create. The syntax for creating a dynamic array is quite straightforward:
dataType* arrayName = new dataType[arraySize];
Example Code Snippet
int* dynamicArray = new int[5];
In this example, we allocate space for an array of 5 integers. The variable `dynamicArray` now points to the first element of this new array in memory.
Understanding how memory is allocated helps in avoiding pitfalls such as accessing uninitialized memory or going out of bounds. Visualizing this allocation can further clarify how memory is structured and how dynamic arrays operate.

Deleting Dynamic Arrays in C++
Why You Need to Delete Dynamic Arrays
After using a dynamic array, it is critical to delete it to free up the memory allocated on the heap. If you do not delete the dynamic array, the memory remains allocated even after it is no longer needed, leading to memory leaks. These leaks can accumulate over time, causing performance degradation and crashes in long-running applications.
Syntax for Deleting Dynamic Arrays
To delete a dynamic array, you must use the `delete[]` operator, which specifically indicates that you are freeing an entire array:
delete[] arrayName;
Example Code Snippet
delete[] dynamicArray;
This line of code releases the memory held by `dynamicArray` back to the system. It is essential to use `delete[]` when deallocating dynamic arrays instead of `delete`, as the latter is meant for single object deletion.
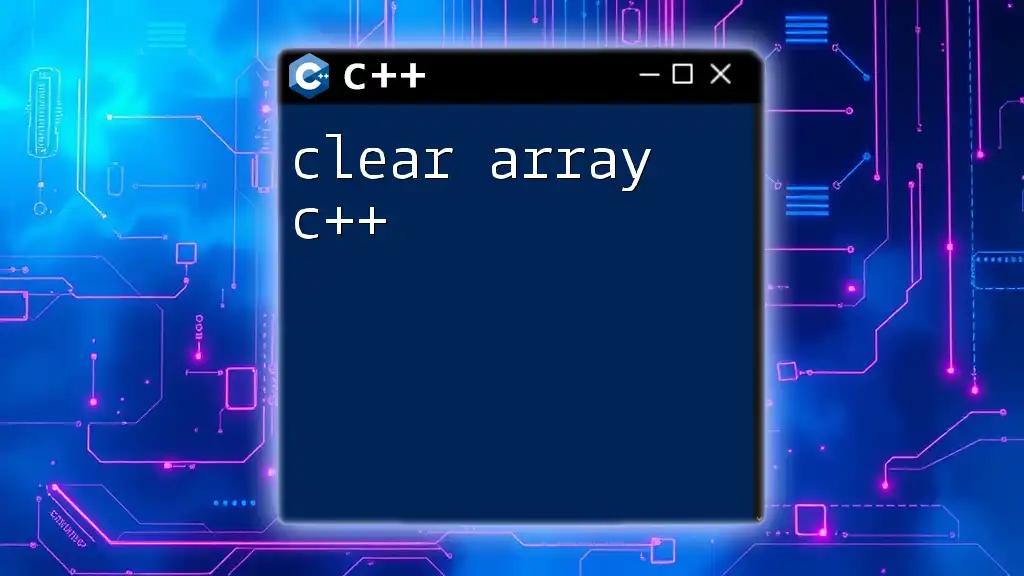
Common Mistakes When Deleting Dynamic Arrays
Forgetting to Delete Allocated Memory
One of the most common mistakes is forgetting to delete the allocated dynamic memory. This oversight can lead to memory leaks, which are difficult to troubleshoot and can significantly diminish the performance of an application.
Double Deleting
Another common pitfall is double deleting. This occurs when you try to delete a pointer that has already been deleted. It can lead to undefined behavior, causing your program to crash or produce erratic results. To avoid this mistake, it is essential to set pointers to `nullptr` after deletion and ensure that deletion occurs only once.

Best Practices for Deleting Dynamic Arrays
Always Match Allocation and Deallocation
It is vital to maintain a clear and consistent relationship between allocation and deallocation. Always ensure that every `new` has a corresponding `delete[]`. This practice not only prevents memory leaks but also promotes clearer and more maintainable code.
Utilize Smart Pointers (C++11 and Beyond)
With the advent of C++11, smart pointers provide an excellent solution for safer memory management. Using smart pointers like `std::unique_ptr` or `std::shared_ptr`, you can handle dynamic memory automatically, eliminating the need for manual deletion.
Using `std::unique_ptr`, for example, could look like this:
#include <memory>
std::unique_ptr<int[]> dynamicArray(new int[5]);
In this situation, `std::unique_ptr` automatically takes care of memory when it goes out of scope, thus preventing leaks and other memory issues.
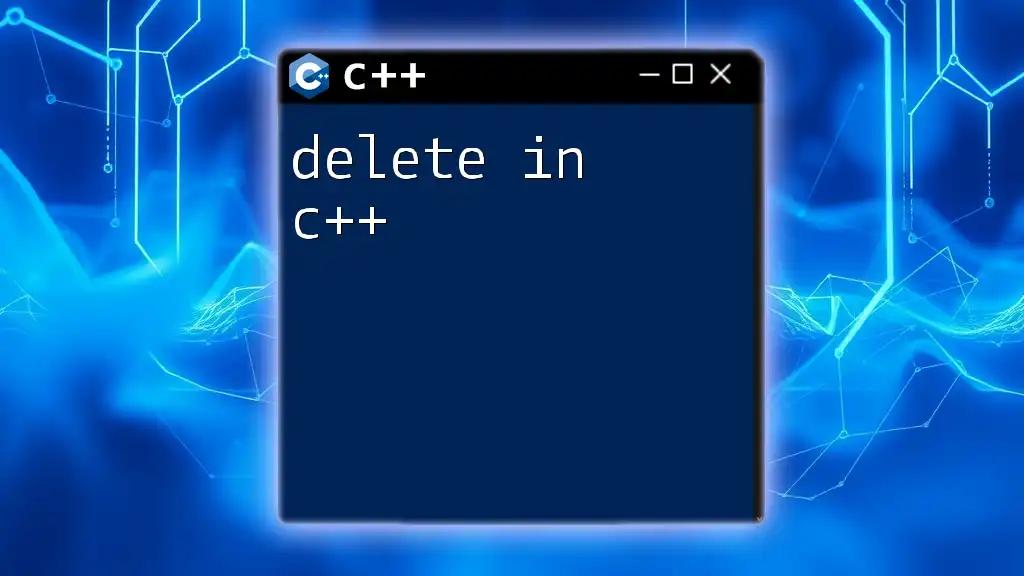
Example Scenario: Using Dynamic Arrays
Step-by-Step Example
Let’s consider a complete example that demonstrates the creation, usage, and deletion of a dynamic array in one cohesive program.
Complete Code Snippet
#include <iostream>
using namespace std;
int main() {
int size;
cout << "Enter size of dynamic array: ";
cin >> size;
// Creating dynamic array
int* myArray = new int[size];
// Fill and process the array
for (int i = 0; i < size; ++i) {
myArray[i] = i * 10; // Example operation
}
// Display the contents
for (int i = 0; i < size; ++i) {
cout << myArray[i] << " ";
}
cout << endl;
// Deleting dynamic array
delete[] myArray;
return 0;
}
This code snippet exemplifies how to interact with a dynamic array in C++. It first prompts the user for the size of the array, allocates memory for it, fills it with values, displays those values, and finally deallocates the memory.
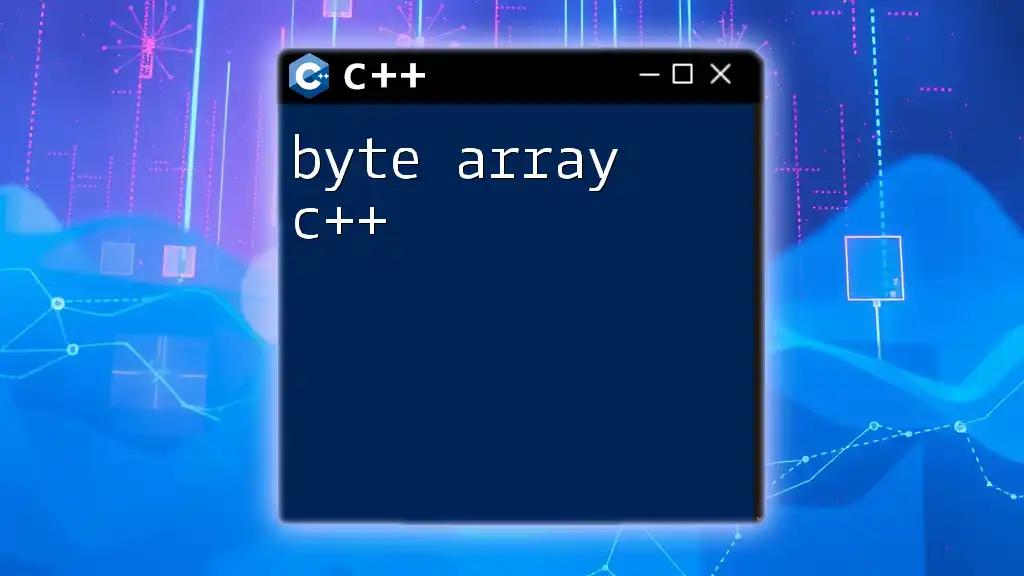
Conclusion
Recap of Key Points
In this comprehensive guide to delete dynamic array C++, we explored the fundamentals of dynamic arrays, covering their creation and deletion. We discussed common mistakes and best practices, emphasizing the importance of memory management to maintain application performance and stability.
Final Thoughts on Dynamic Arrays in C++
C++ offers powerful tools for working with dynamic arrays, but with great power comes great responsibility. By adhering to best practices and properly managing memory, you can harness dynamic arrays effectively to create robust applications. Start implementing these techniques today, ensuring safe and efficient coding in your projects!