In C++, you can initialize a character array by specifying the characters within curly braces, optionally ending with a null terminator to indicate the end of the string. Here's a code snippet demonstrating this:
char myArray[] = {'H', 'e', 'l', 'l', 'o', '\0'};
Understanding Character Arrays
What is a Char Array?
In C++, a char array is a collection of characters that are stored sequentially in memory. The fundamental data type involved here is `char`, which can hold a single character. Character arrays are particularly useful for managing strings, as they allow manipulation of a sequence of characters as a single entity.
One of the striking differences between char arrays and other data types, such as `std::string`, is that char arrays require manual memory management, which can sometimes lead to errors if not handled properly.
Memory Layout of Char Arrays
Understanding how character arrays are laid out in memory is crucial. A char array essentially represents a contiguous block of memory where each character is stored in a sequential manner. When dealing with strings, C++ relies on null-termination to signify the end of the string. This means that after the last character in a char array, a null character (`'\0'`) must be present to indicate that the array has ended.

Initializing Char Arrays in C++
Static Initialization
Static initialization is a straightforward way to initialize char arrays at compile time. You can specify the size of the array and directly input the characters.
For example:
char staticArray[5] = {'H', 'e', 'l', 'l', 'o'};
In this case, the array `staticArray` is explicitly initialized with five characters. However, the programmer must remember to provide enough space for each character, including the null terminator. Thus, if you intend to store a string of "Hello", you should define the array like this:
char staticArray[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
Dynamic Initialization
Using `new` Keyword
Dynamic initialization allows for more flexibility, particularly when the size of the array is not known at compile time. You can use the `new` keyword to allocate memory dynamically.
Here's an example:
char* dynamicArray = new char[6]{'H', 'e', 'l', 'l', 'o', '\0'};
With this example, `dynamicArray` points to a dynamically allocated array that holds the string "Hello". It’s important to remember that with dynamic memory allocation, the programmer is responsible for deallocating the memory using `delete[] dynamicArray` to avoid memory leaks.
Using `std::vector`
As an alternative to raw arrays, you can utilize `std::vector`, which handles memory management itself and offers more safety features.
For instance:
#include <vector>
std::vector<char> vecArray = {'H', 'e', 'l', 'l', 'o', '\0'};
This approach has multiple advantages: `std::vector` manages memory automatically, resizes if needed, and simplifies the process of maintaining variable-size character collections.
Implicit Initialization
C++ also allows implicit initialization of char arrays when defined using string literals. This method is succinct and highly recommended for initializing strings.
Here’s an example:
char implicitArray[] = "Hello";
In this case, C++ automatically adds the null terminator, allowing you to declare a string without needing to specify its size explicitly. The resulting size will be sufficient to hold the string plus the null terminator.
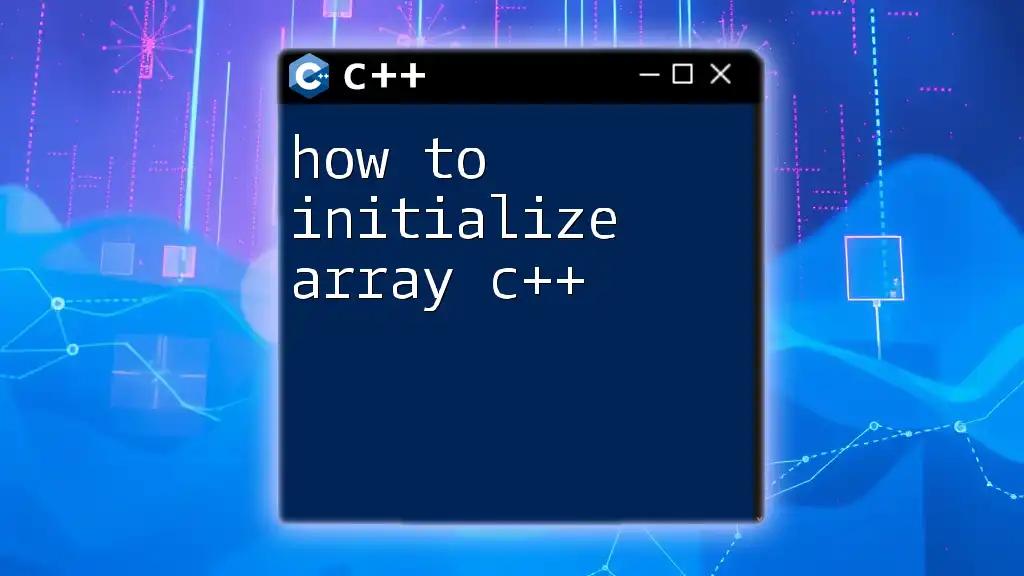
Common Mistakes in Char Array Initialization
Forgetting Null-Termination
One of the most common mistakes made when initializing char arrays is neglecting to include the null terminator. A char array lacking proper termination can lead to undefined behavior and potential crashes during execution, as functions that operate on strings will continue to read memory beyond the intended end.
For example:
char mistakeArray[6] = {'H', 'e', 'l', 'l', 'o'}; // No null-terminator
Over-Allocating or Under-Allocating
Careful attention to the size of the array is crucial. Over-allocating may lead to wasted memory resources, while under-allocating can lead to buffer overflows, which are a significant source of vulnerabilities in software.
To allocate properly, always account for the null terminator, especially when dealing with string inputs or dynamic content.

Best Practices for Using Char Arrays
Choosing the Right Initialization Method
The choice between static and dynamic initialization largely depends on the requirements of your program. For fixed-size strings known at compile-time, static initialization (with carefully considered array sizes) is often the best route. Conversely, if you anticipate variable-length strings or require more complex manipulation, opting for `std::vector` or dynamic arrays gives you the needed flexibility.
Avoiding Common Pitfalls
To ensure safe and effective usage of char arrays:
- Regularly check for buffer overflows: Always verify that the data you are writing does not exceed the allocated size.
- Include null-termination: Always ensure strings are null-terminated; this is critical for correct string operations in C++.
- Stay vigilant with dynamic memory: Always remember to free dynamically allocated arrays to prevent memory leaks.

Conclusion
Understanding how to initialize char arrays in C++ is foundational for effective programming with strings. By mastering static and dynamic initialization methods, recognizing common mistakes, and adopting best practices, you can manipulate character data safely and efficiently. Remember, proper initialization is not just a coding requirement; it directly affects the reliability and security of your programs.
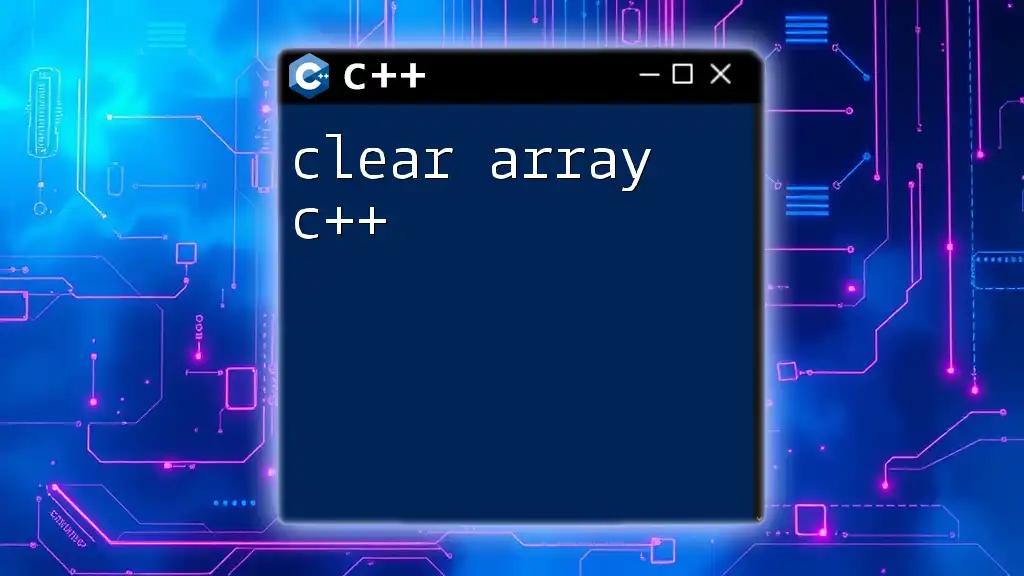
Additional Resources
Explore C++ documentation and tutorials to deepen your understanding of character arrays and other essential programming concepts. Online platforms like LeetCode and Codecademy can provide practical coding experience, while books such as “The C++ Programming Language” can enhance your learning further.

Call to Action
We encourage you to share your experiences with char arrays and initialization in the comments below. Don’t forget to subscribe for more insightful C++ tips and tricks!