Dynamic arrays in C++ are arrays whose size can be determined at runtime, allowing for flexible memory allocation using pointers and the `new` keyword. Here's a simple example:
#include <iostream>
int main() {
int size;
std::cout << "Enter the size of the dynamic array: ";
std::cin >> size;
// Create a dynamic array
int* dynamicArray = new int[size];
// Assign values to the array
for (int i = 0; i < size; ++i) {
dynamicArray[i] = i * 2; // Example assignment
}
// Output the values
for (int i = 0; i < size; ++i) {
std::cout << dynamicArray[i] << " ";
}
// Deallocate the memory
delete[] dynamicArray;
return 0;
}
What is a Dynamic Array?
A dynamic array in C++ is a data structure that allows you to manage collections of variables whose size can change during runtime. Unlike static arrays, which require a fixed size upon initialization, dynamic arrays can easily accommodate varying data needs, making them invaluable for tasks where the amount of data isn't known in advance.
Dynamic arrays provide enhanced flexibility and scalability by allocating memory on the heap rather than the stack, which has its own limitations. They are essential for managing memory effectively and for applications that require dynamic memory use.
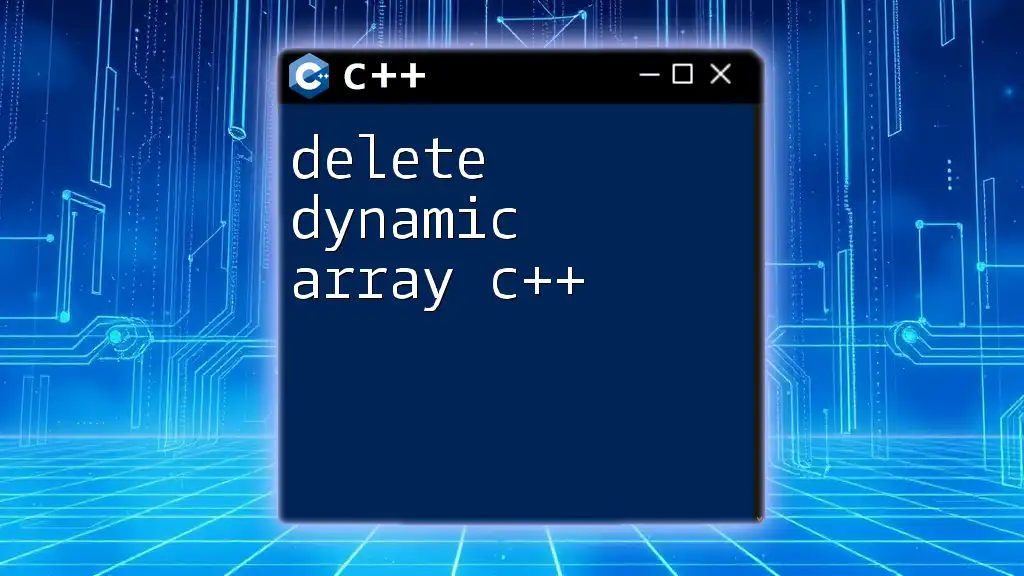
Understanding the Need for Dynamic Arrays
Memory Allocation in C++
Understanding how memory allocation works in C++ is crucial when dealing with dynamic arrays. Memory can be divided into two main regions:
- Stack Memory: Used for static memory allocation, stack memory is fast but has limited size. Once the function call returns, the allocated memory is automatically released.
- Heap Memory: This is the area for dynamic memory allocation, where variables can persist beyond the scope of the function. It allows for more significant allocation, but developers must manage this memory manually.
Advantages of Dynamic Arrays
- Flexibility and Reusability: Dynamic arrays can grow or shrink as needed, allowing for efficient use of memory.
- Efficient Memory Utilization: They help avoid wasteful allocation typical in static arrays, where a fixed size might not always be utilized.
Common Use Cases
Dynamic arrays are particularly useful in scenarios such as:
- Implementing data structures like lists and stacks.
- Storing user inputs where the number of inputs is unknown at compile time.
- Handling variable-length datasets in applications such as image processing or real-time data analysis.
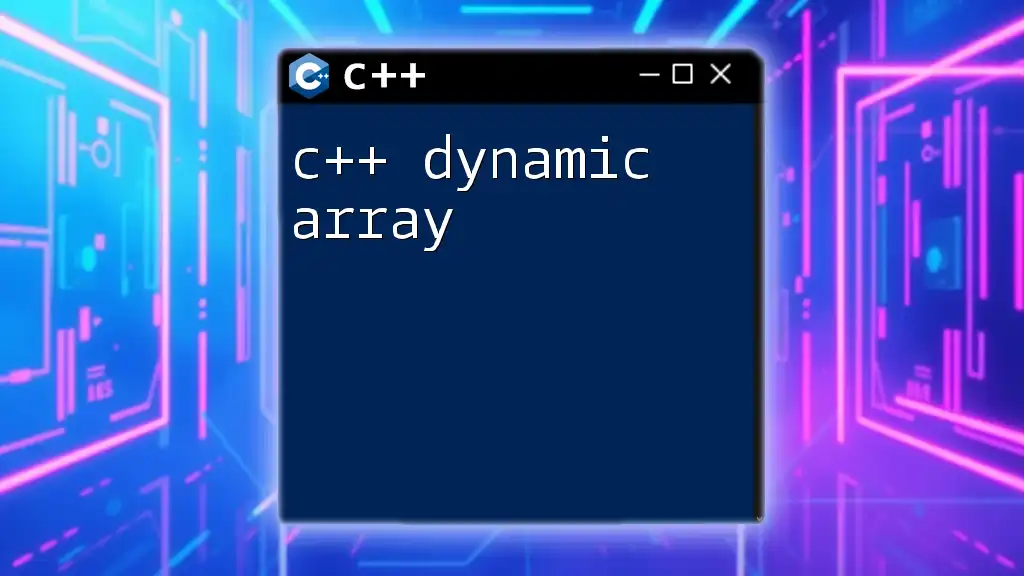
Creating Dynamic Arrays in C++
Using `new` Operator
To create a dynamic array in C++, you use the `new` operator. This operator allocates memory on the heap. The basic syntax looks like this:
int* arr = new int[size];
Here, `size` can be a variable allowing the dynamic declaration of the array's length.
Initializing Dynamic Arrays
You can initialize a dynamic array in several ways:
Default Initialization: If you just allocate memory without initializing the elements, they'll contain indeterminate values.
User-defined Initialization: You can populate your array immediately after allocation:
int size;
std::cout << "Enter the size of the array: ";
std::cin >> size;
int* arr = new int[size];
for (int i = 0; i < size; i++) {
std::cout << "Enter element " << i + 1 << ": ";
std::cin >> arr[i]; // Initializing with user input
}
This way, you obtain a dynamic array filled with user-defined values.
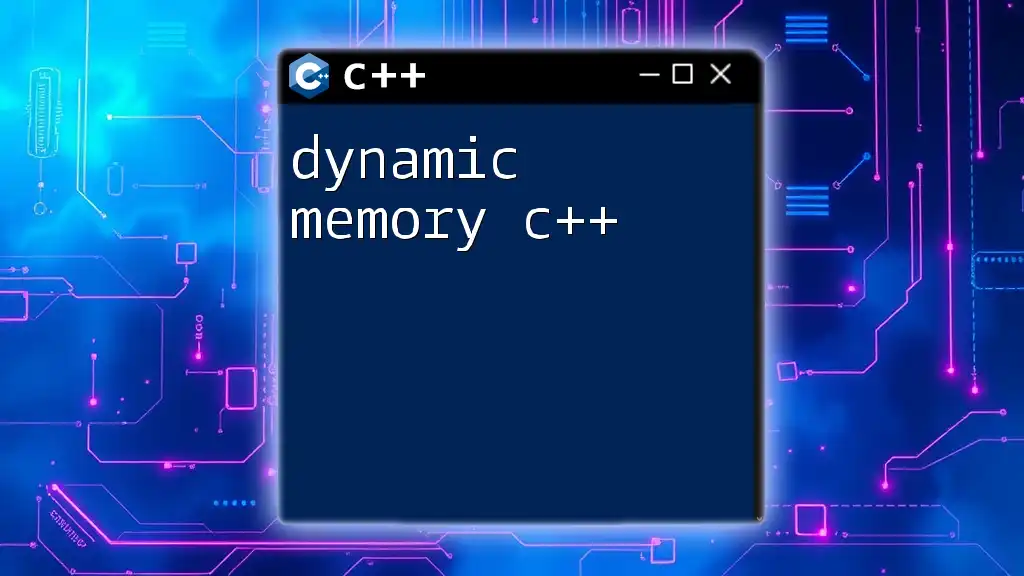
Accessing Elements in Dynamic Arrays
Pointer Arithmetic
In C++, arrays and pointers are closely related. You can access elements of a dynamic array using pointer arithmetic:
int value = *(arr + i); // Accessing the ith element
Example
To modify elements, you can simply use the index notation or pointer arithmetic:
arr[i] = value; // Set the ith element to a new value
By understanding these concepts, you can effectively navigate through dynamic arrays.
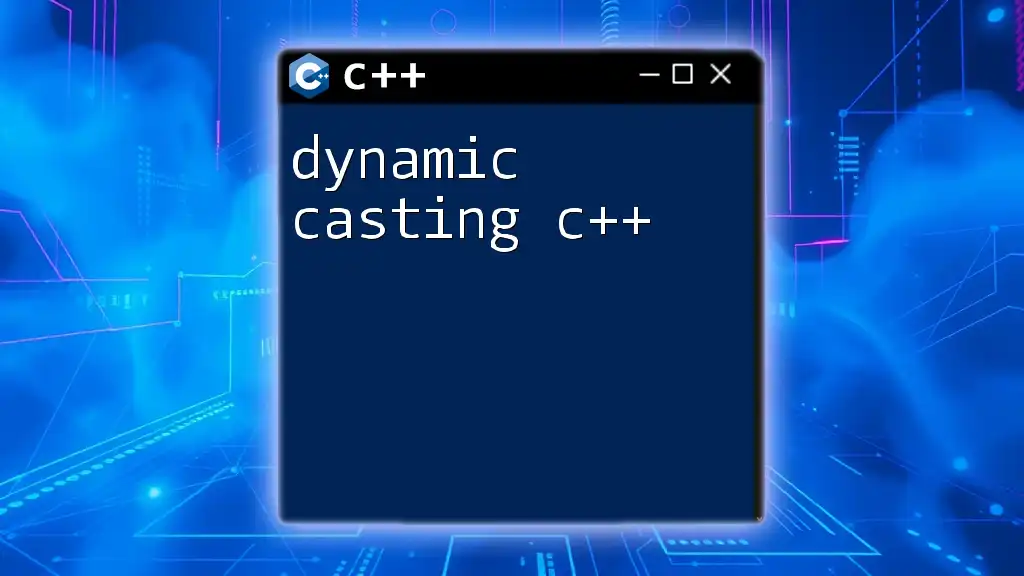
Resizing Dynamic Arrays
Why Resize Dynamic Arrays?
A dynamic array may need to resize when the original capacity is exceeded, which occurs when adding more elements than initially allocated. This resizing ensures your array can adapt to varying data sizes.
Techniques for Resizing
- Manual Resizing: This involves creating a new larger array and copying the data from the original array to the new one, like so:
int newSize = currentSize + additionalSize;
int* newArr = new int[newSize];
// Copying old data to newArr...
for (int i = 0; i < currentSize; i++) {
newArr[i] = arr[i];
}
// Deallocation of old array
delete[] arr;
arr = newArr; // Point the old array to the new array
- Using `std::vector`: The C++ Standard Template Library (STL) provides dynamic arrays in the form of `std::vector`, which automatically handles resizing.
Example
When using vectors, resizing happens implicitly:
#include <vector>
std::vector<int> vec;
vec.push_back(value); // Automatically resizes
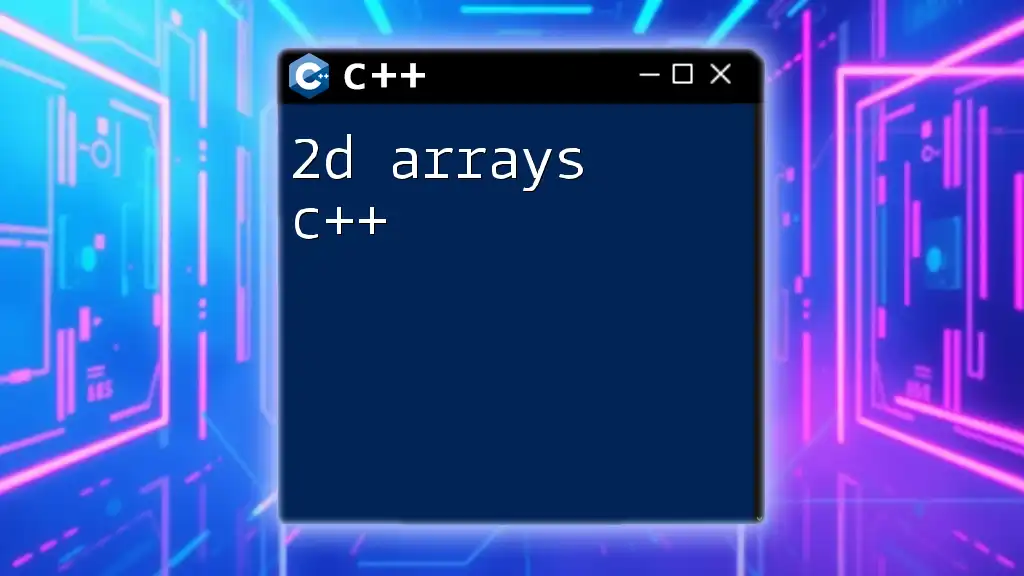
Deallocating Memory
Importance of Memory Management
Manual memory management is critical in C++ to avoid memory leaks, which occur when allocated memory isn't released. Properly managing memory helps ensure efficient use of resources.
Deleting Dynamic Arrays
When you're finished using a dynamic array, you need to deallocate the memory using the `delete` operator, specifically for arrays:
delete[] arr;
Example of Proper Memory Management
Here’s a complete example demonstrating memory allocation and deallocation:
int size;
std::cout << "Enter the size of the dynamic array: ";
std::cin >> size;
int* arr = new int[size]; // Dynamic array creation
//... (Use the array)
delete[] arr; // Proper deletion
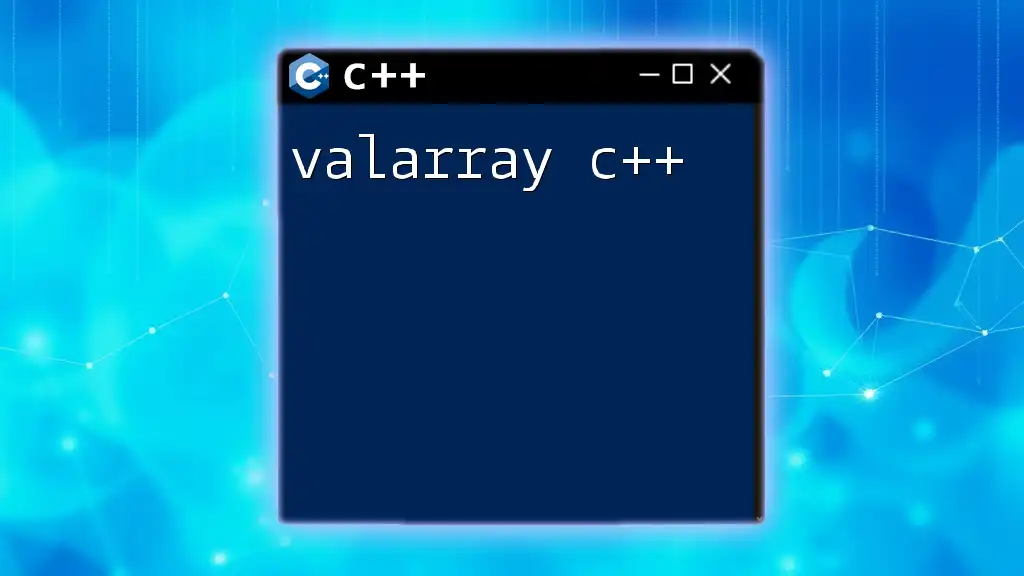
Dynamic Arrays in STL with `std::vector`
Introduction to `std::vector`
`std::vector` is a part of the C++ Standard Template Library that abstracts away manual memory management, providing a dynamic array implementation that handles allocation and resizing automatically.
Basic Operations with `std::vector`
You can create, add, and retrieve elements easily. Here’s a brief on some fundamental operations:
-
Creating a vector:
std::vector<int> vec;
-
Adding elements:
vec.push_back(value);
-
Accessing elements:
int val = vec[i];
Example
Here's a simple example using `std::vector`:
#include <vector>
std::vector<int> vec;
vec.push_back(10);
vec.push_back(20);
for (size_t i = 0; i < vec.size(); i++) {
std::cout << vec[i] << " "; // Outputs: 10 20
}
This code snippet shows how intuitive working with `std::vector` can be compared to raw dynamic arrays.
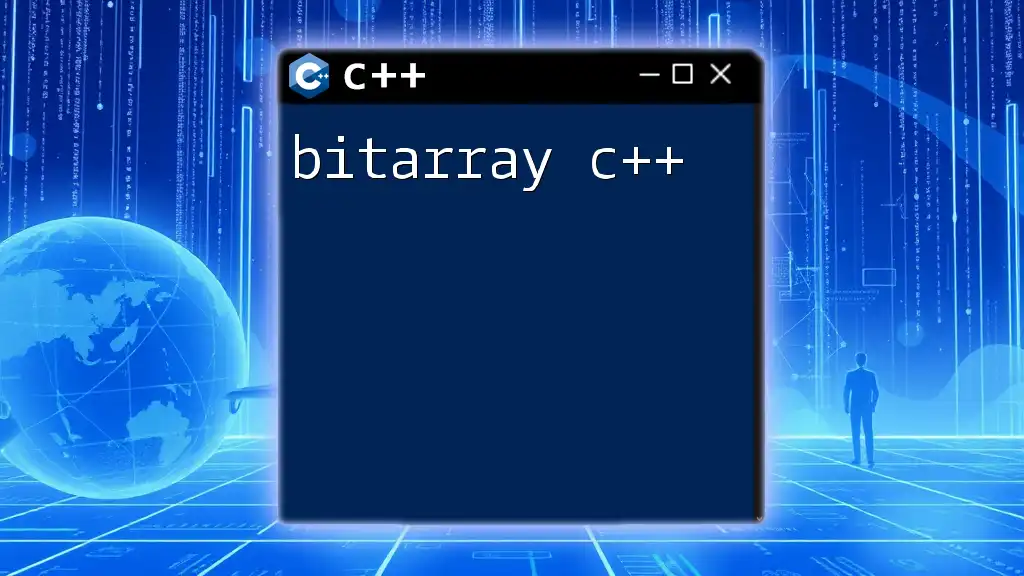
Common Pitfalls with Dynamic Arrays
Memory Leaks
One of the most prevalent issues is memory leaks, which occur when you forget to free memory allocated for a dynamic array. This can cause applications to run out of memory over time.
To prevent this, always ensure to deallocate memory using `delete[]` for dynamic arrays, especially in scenarios with multiple allocations.
Accessing Out of Bounds
Accessing elements outside the allocated limits of your dynamic array can lead to undefined behavior, crashes, or data corruption. Always check array bounds before trying to access elements:
if (index >= 0 && index < size) {
// Safe to access arr[index]
}
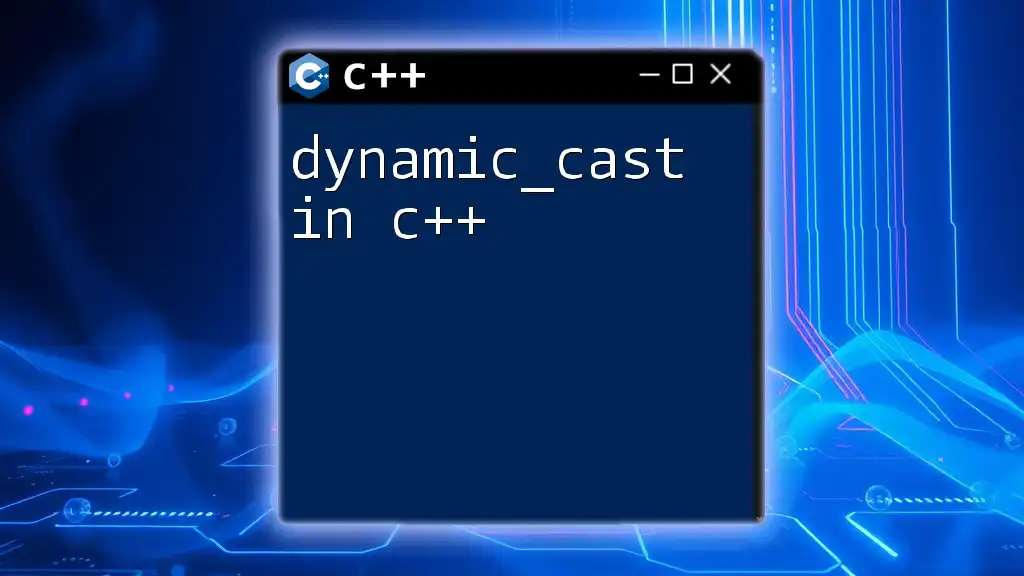
Conclusion
Dynamic arrays in C++ are powerful tools that offer flexibility and efficiency in memory management. Understanding their creation, manipulation, and deallocation is essential for writing robust C++ applications. Experimentation with different scenarios will deepen your understanding and proficiency with dynamic arrays in C++.
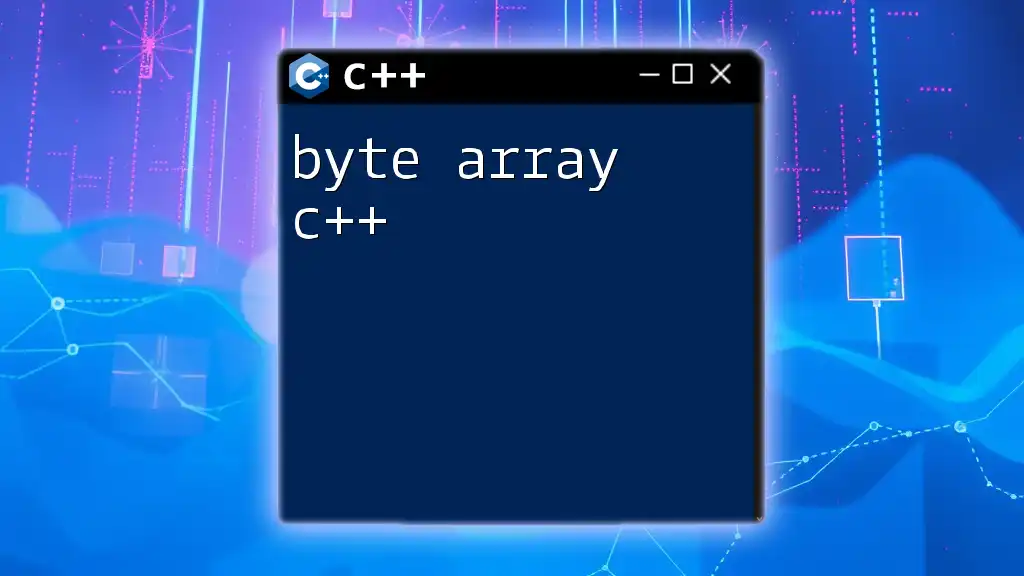
Additional Resources
To further your knowledge, consider exploring books and online tutorials focused on C++ dynamic arrays, and engage with programming communities for discussions and support. The C++ documentation is also an excellent resource for understanding details about pointers, memory management, and the STL.