In C++, `async` and `await` facilitate writing asynchronous code, allowing functions to run concurrently without blocking the main thread, implemented through the `std::async` function and future handling.
#include <iostream>
#include <future>
#include <chrono>
int asyncTask() {
std::this_thread::sleep_for(std::chrono::seconds(2)); // Simulate a long task
return 42; // Return result
}
int main() {
std::future<int> result = std::async(std::launch::async, asyncTask);
std::cout << "Doing other work...\n";
// Wait for the result
std::cout << "The answer is: " << result.get() << '\n';
return 0;
}
Understanding Asynchronous Programming in C++
What is Asynchronous Programming?
Asynchronous programming refers to the execution of tasks without blocking the progress of other tasks. Instead of waiting for a task to complete, your program can continue executing other operations, which is crucial for improving performance and responsiveness, especially in applications that require concurrency.
Advantages of asynchronous programming include:
- Improved Performance: Non-blocking tasks allow programs to perform other operations while waiting, reducing idle time.
- Enhanced User Experience: In user interfaces, async operations keep applications responsive, preventing them from freezing during long processing tasks.
- Efficient Resource Utilization: By using threads and asynchronous patterns, resources can be better allocated, avoiding timeouts and enhancing throughput.
Comparison with Synchronous Programming In synchronous programming, tasks are performed sequentially. Each task must complete before the next begins, which can lead to inefficient use of resources and a laggy user experience. Asynchronous programming resolves these issues, allowing for multitasking and overall better application performance.
C++ and Multithreading
Multithreading has been a fundamental part of C++ development since the introduction of the C++ Standard Library. With improvements in C++11 and later versions, especially C++20, developers gained more robust tools for handling concurrency.
The Standard Library provides components like `std::thread`, `std::async`, and more that simplify the implementation of asynchronous operations.
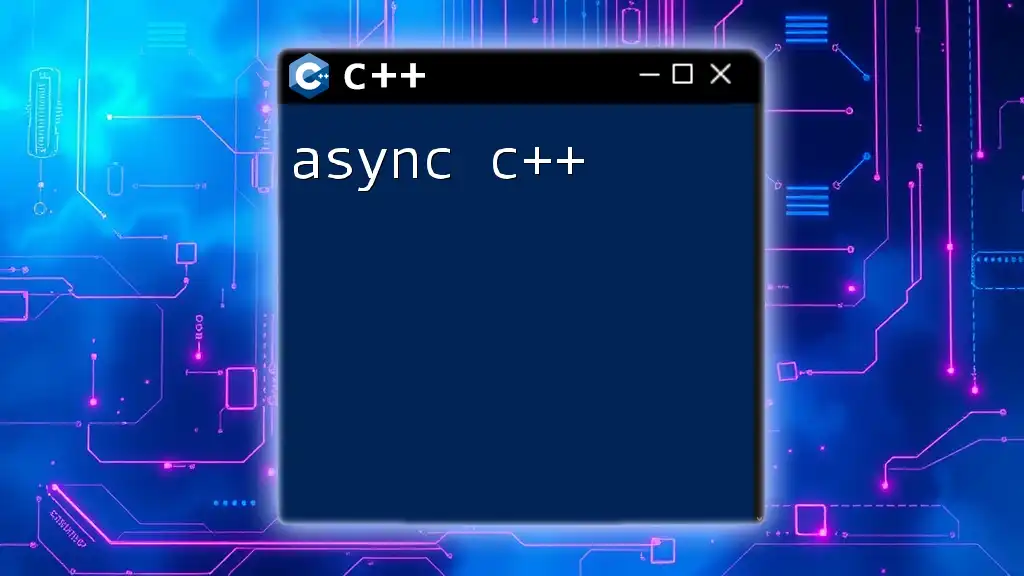
The Concept of Async Await in C++
Introduction to Async and Await in C++
The `async` and `await` keywords are common in modern programming paradigms, allowing developers to write asynchronous code that remains easy to understand. While C++ does not have these keywords directly, its `std::async` and coroutines in C++20 effectively perform the same functions.
Benefits of using async await functionality include:
- Simplifying the complexity of multithreaded programming.
- Allowing for cleaner separation of business logic from orchestration concerns.
C++20 and Coroutines
C++20 introduced coroutines, a powerful feature for asynchronous programming. Coroutines enable functions to suspend execution, allowing for cooperative multitasking. With the introduction of keywords like `co_await`, `co_return`, and `co_yield`, developers can manage asynchronous operations seamlessly.
- `co_await`: Used to suspend execution until the awaited operation completes.
- `co_return`: Indicates the result of the coroutine.
- `co_yield`: Allows yielding multiple values over time from a coroutine.
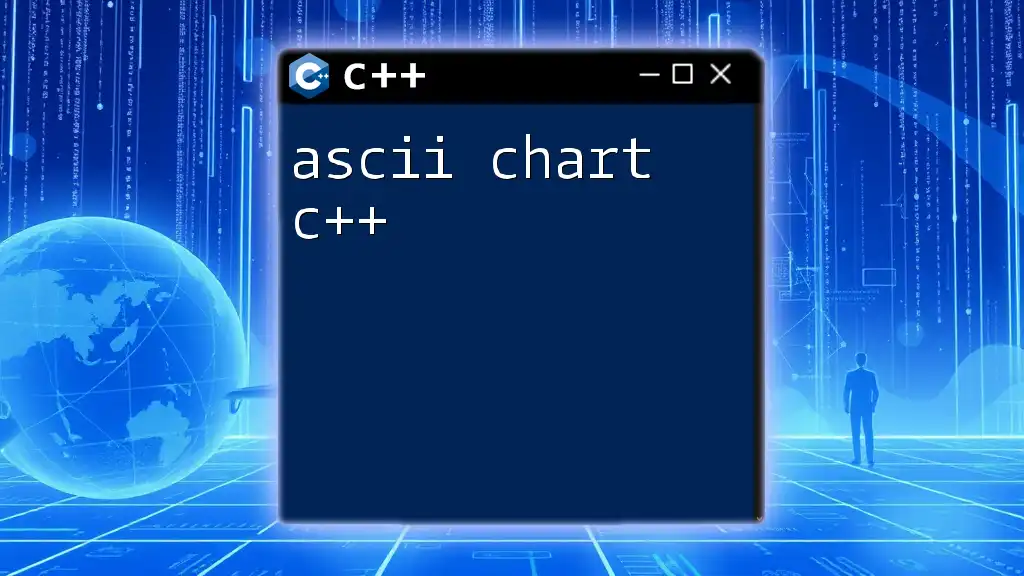
Getting Started with Async Await in C++
Setting Up Your C++ Environment
To get started with async await in C++, you need the appropriate tools and compiler that supports C++20 features. The following options are popular for their compatibility:
- GCC: Ensure you use version 10 or newer.
- Clang: Same as GCC, anything from version 10 or above.
- MSVC (Visual Studio): Ensure you have Visual Studio 2019 (version 16.8 or later).
Make sure to enable C++20 features in your project settings, as each environment has specific flags for this purpose.
Basic Syntax of Async Await
In C++, the simplest way to introduce asynchronous functions is by using `std::async`. Here’s an example demonstrating how to create and call an async function:
#include <iostream>
#include <future>
int asyncFunction() {
// Simulate a time-consuming task
std::this_thread::sleep_for(std::chrono::seconds(2));
return 42;
}
int main() {
std::future<int> futureValue = std::async(asyncFunction);
std::cout << "Waiting for result...\n";
std::cout << "Result is: " << futureValue.get() << std::endl;
return 0;
}
In this code snippet, `std::async` kicks off `asyncFunction()` in a separate thread, allowing the main thread to continue executing. The call to `futureValue.get()` will block until the async function is completed, but because it’s running separately, the overall system stays responsive.
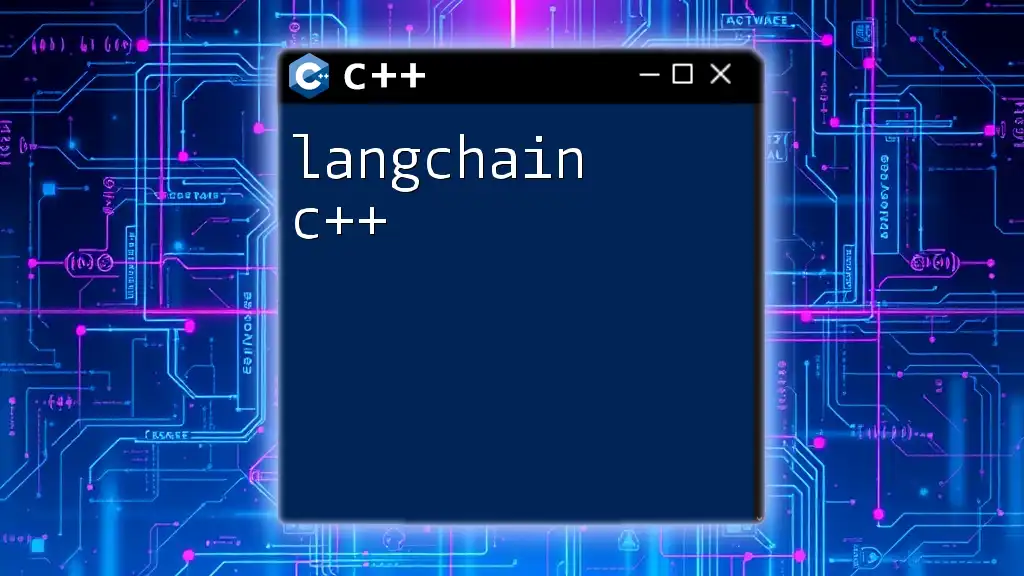
Advanced Async Await Techniques in C++
Implementing C++ Coroutines
With C++20, coroutines become a fundamental aspect of writing async code. The following code illustrates a basic coroutine setup:
#include <iostream>
#include <coroutine>
struct MyAwaiter {
bool await_ready() { return false; }
void await_suspend(std::coroutine_handle<>) {}
int await_resume() { return 42; }
};
struct MyCoroutine {
struct promise_type {
MyCoroutine get_return_object() { return {}; }
std::suspend_never initial_suspend() { return {}; }
std::suspend_never final_suspend() noexcept { return {}; }
void unhandled_exception() {}
void return_void() {}
};
};
MyCoroutine asyncFunction() {
int result = co_await MyAwaiter();
std::cout << "Result: " << result << std::endl;
}
In this illustration, `MyAwaiter` serves as a custom awaitable type, which enables `asyncFunction()` to suspend execution and resume later. Understanding the mechanics of coroutines, especially the promise and awaitable types, is vital for leveraging their full potential in C++.
Creating Custom Awaitable Types
Developers can create custom awaitable types to define new asynchronous behaviors. This involves implementing certain methods that dictate how the awaitable interacts with the coroutine mechanism. Here is a brief overview of the necessary methods:
- `await_ready`: Determines if the operation can complete immediately without suspension.
- `await_suspend`: Handles what happens when the coroutine is suspended.
- `await_resume`: Retrieves the result once the operation is complete.
Error Handling in Async Operations
When writing asynchronous code, error handling is crucial to ensure robustness. Here are some best practices:
- Use Exception Handling: Leverage try-catch blocks within coroutines to catch exceptions and handle them gracefully.
- Check Future States: When using `std::async`, check if the future has valid results before accessing them.
- Graceful Shutdown: Implement proper cleanup and resource deallocation when an operation fails.
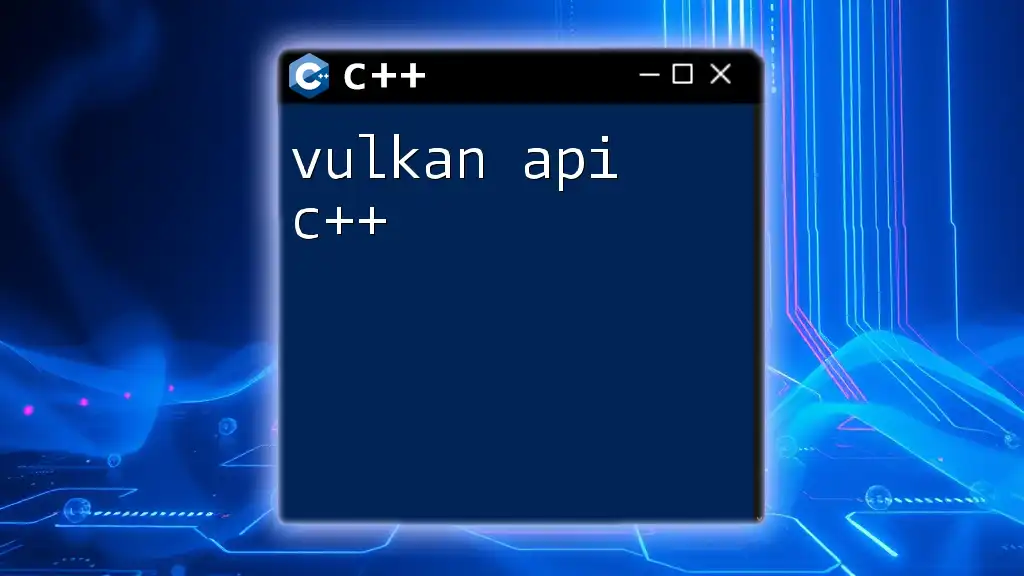
Practical Applications of Async Await in C++
Use Cases for Async Await in C++
Async await patterns are suitable for various applications, including:
- Networking Applications: Handle multiple connections, data transfers, and responses in a non-blocking manner.
- GUI Applications: Improve responsiveness by performing time-consuming tasks in the background, allowing users to interact with the application smoothly.
- Real-Time Systems: Gaming and simulations benefit from async operations that manage multiple tasks simultaneously without lag.
Real-World Example: Building an Async HTTP Client
A practical demonstration of async await in C++ can be seen in implementing an asynchronous HTTP client. Here's a pseudo code snippet:
#include <iostream>
#include <future>
#include <curl/curl.h>
std::string fetchData(const std::string& url) {
// Use libcurl to fetch data
// (Attach actual implementation here)
return "Fetched Data";
}
std::future<std::string> asyncFetch(const std::string& url) {
return std::async(std::launch::async, fetchData, url);
}
int main() {
auto data = asyncFetch("http://example.com");
std::cout << "Waiting for data...\n";
std::cout << data.get() << std::endl;
return 0;
}
In this example, the function `fetchData` would perform an HTTP request and return the result asynchronously, maintaining responsiveness throughout the task.
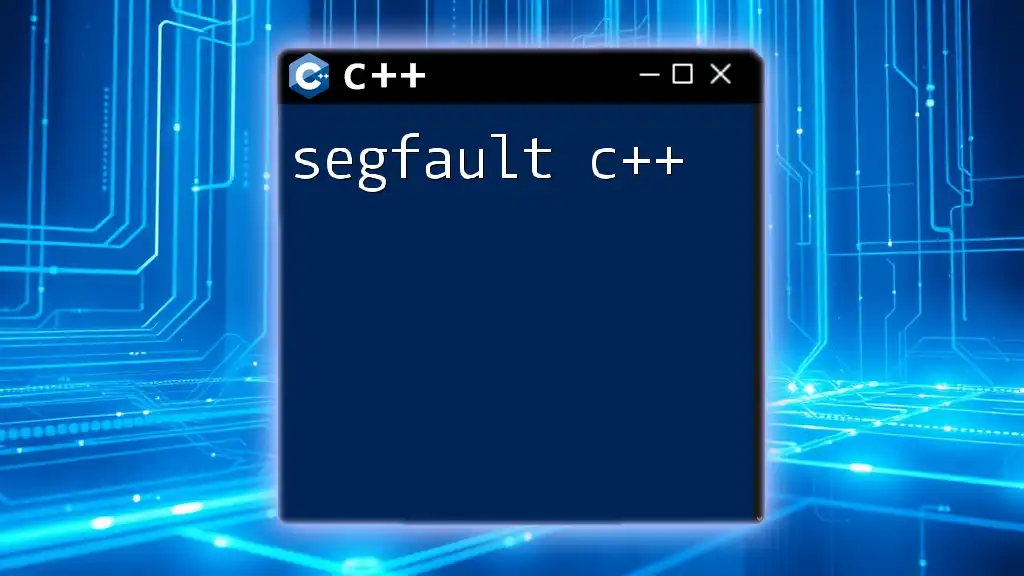
Conclusion
Recap of Async Await in C++
Async await functionality enhances C++ capabilities and allows developers to write non-blocking code effectively. By leveraging features introduced in C++20, developers can manage asynchronous tasks with more straightforward syntax and improved readability.
Further Reading and Resources
To deepen your knowledge of async await in C++, consider exploring additional resources:
- C++ Coroutines Documentation: Official reference for coroutines in C++20.
- Advanced C++ Programming: Books focusing on concurrency and multithreading concepts.
- Online Courses: Platforms offering courses on modern C++ practices.
Encouraging Practice
It is essential to practice implementing async await patterns in your projects. Understanding these concepts will significantly enhance your programming skills and contribute to the development of responsive, high-performing applications. Engaging in coding challenges, contributing to open-source projects, and developing personal projects will accelerate your learning journey.
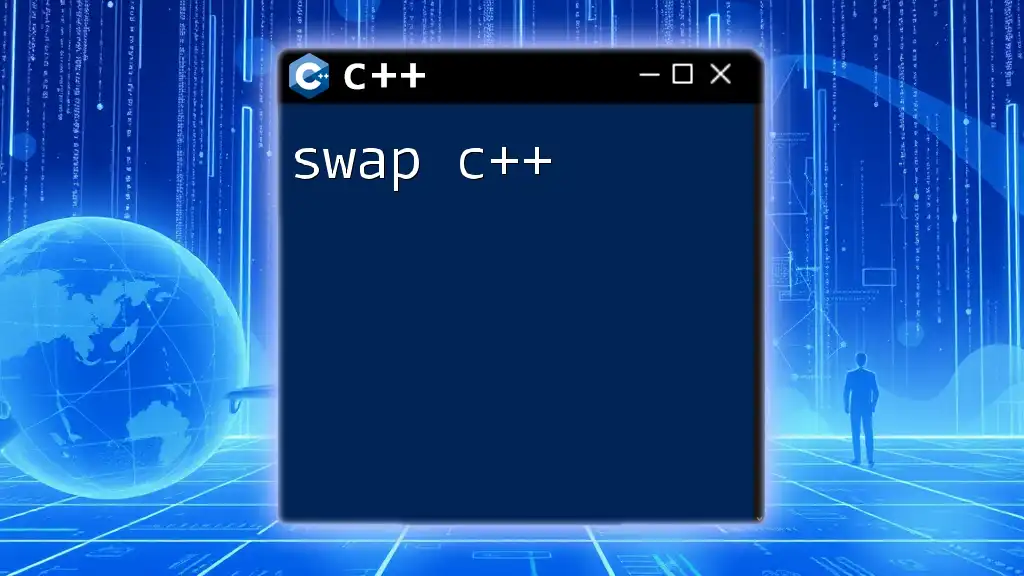
FAQ Section
What are the main advantages of using async await in C++?
The primary advantages include improved responsiveness, more efficient resource utilization, and simplified error handling in asynchronous tasks.
Is async await supported in all C++ compilers?
While most modern compilers have started supporting C++20 features, you should ensure that you are using versions that support coroutines. Tools like GCC, Clang, and MSVC that are updated to the latest standards implement async await capabilities.
Are there any limitations when using async await in C++?
Some limitations include the complexity of managing multi-threaded operations, potential pitfalls with shared resources, and the need for careful exception management to prevent crashes or undefined behavior.
By embracing async await in C++, developers can build more efficient and responsive applications, harnessing the full power of modern C++.