The Vulkan API for C++ provides a low-level, cross-platform graphics and compute API designed to give developers more direct control over GPU resources and performance.
Here's a simple code snippet to initialize the Vulkan instance:
#include <vulkan/vulkan.h>
int main() {
VkInstance instance;
VkInstanceCreateInfo createInfo = {};
createInfo.sType = VK_STRUCTURE_TYPE_INSTANCE_CREATE_INFO;
if (vkCreateInstance(&createInfo, nullptr, &instance) != VK_SUCCESS) {
return -1; // Initialization failed
}
// Application logic here
vkDestroyInstance(instance, nullptr); // Cleanup
return 0;
}
What is Vulkan API?
The Vulkan API is a powerful graphics and compute API designed to provide high-performance, low-overhead access to modern GPUs. It serves as a crucial tool for developers, enabling them to create high-efficiency graphics applications and games. Built as a successor to OpenGL, Vulkan emphasizes direct control over GPU resources and execution, making it an ideal choice for applications requiring advanced rendering techniques.
The evolution of Vulkan stems from the need for higher performance graphics on various platforms. It was developed by the Khronos Group, the same consortium that birthed OpenGL, and has become a standard in the realm of real-time rendering.
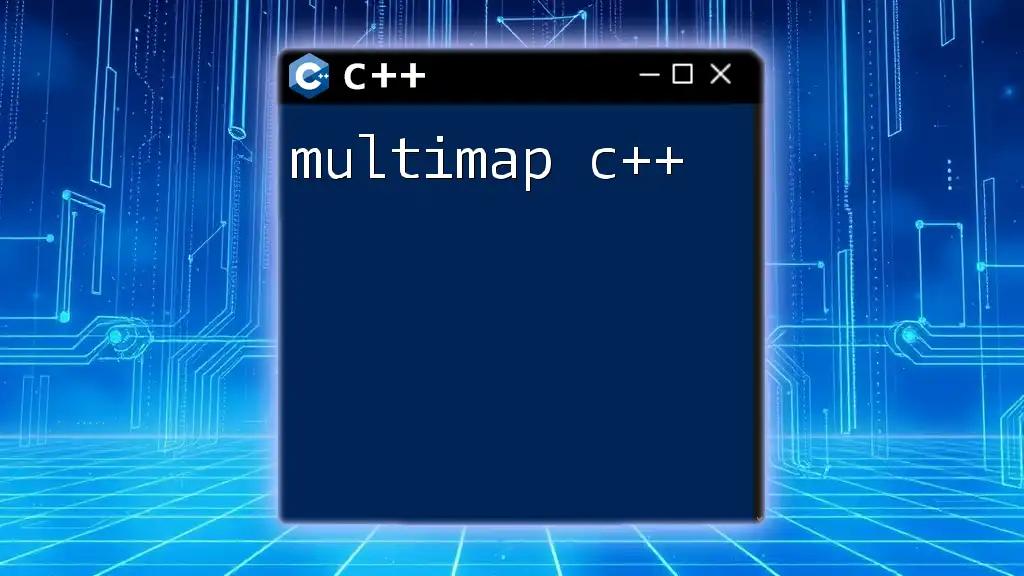
Advantages of Using Vulkan with C++
When considering the use of Vulkan API with C++, several advantages stand out:
- Performance Benefits: Vulkan is designed to minimize CPU overhead, allowing developers to make better use of multi-threading. This translates to smoother graphics and improved frame rates.
- Explicit Control Over GPU: Unlike some older APIs, Vulkan gives developers fine-tuned control over how resources are handled, enabling more efficient memory management and execution.
- Cross-Platform Support: Vulkan is not limited to a single operating system or type of hardware, allowing for the development of applications that run across multiple platforms, including Windows, Linux, and mobile devices.
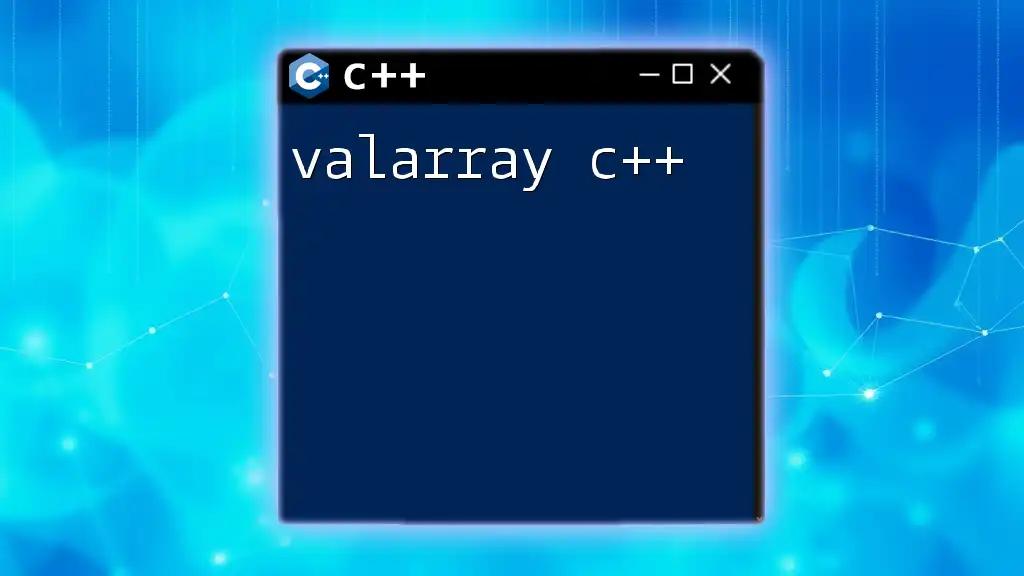
Getting Started with Vulkan and C++
Setting Up Your Development Environment
Before diving into Vulkan programming with C++, you must properly set up your development environment. Here are the essentials:
-
Required Tools and Libraries:
- Download and install the Vulkan SDK, which includes all necessary libraries and debugging tools.
- Ensure your graphics driver supports Vulkan. If not, you must update it.
-
Configuring Your IDE:
- If you use Visual Studio or any other IDE, add the Vulkan include directory to your project settings, and link against the Vulkan library. This is crucial for compiling your Vulkan projects without issues.
Creating Your First Vulkan Project
Once everything is set up, you can create your first Vulkan project. A basic project structure typically includes directories for shaders, assets, and source code. This organization will help in scaling your project later.
Basic Code Snippet: Initial Setup
#include <vulkan/vulkan.h>
VkInstance instance;
VkApplicationInfo appInfo = {};
appInfo.sType = VK_STRUCTURE_TYPE_APPLICATION_INFO;
appInfo.pApplicationName = "Hello Vulkan";
appInfo.applicationVersion = VK_MAKE_VERSION(1, 0, 0);
This snippet demonstrates how to create and initialize a Vulkan instance, the core component for any Vulkan application.
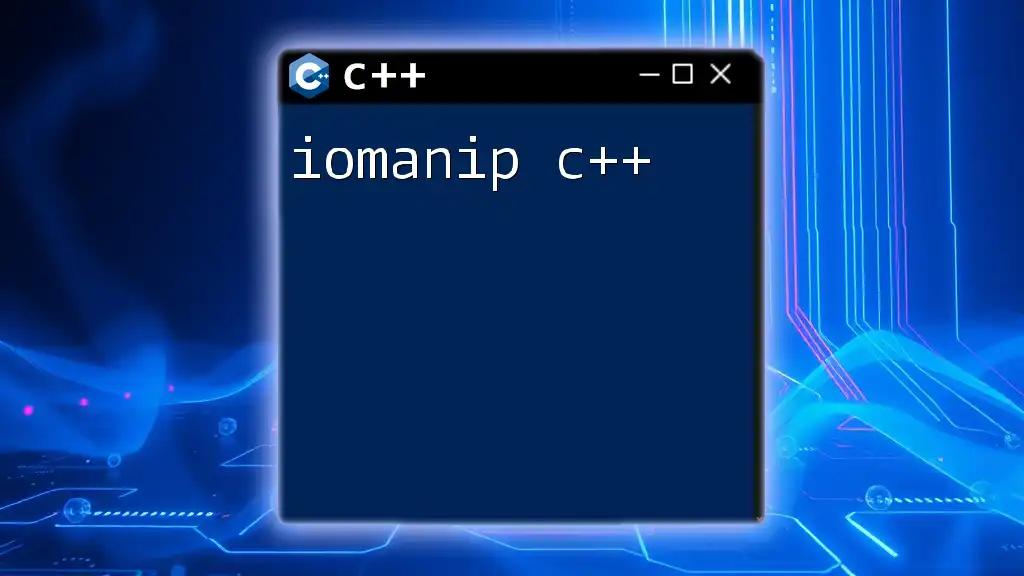
Understanding Vulkan Architecture
Core Components of Vulkan
Vulkan Instance
The Vulkan instance acts as a foundation for your Vulkan application. You create a new instance by providing information about your application and the Vulkan API version you intend to use.
Example Code Snippet for Instance Creation
VkInstanceCreateInfo createInfo = {};
createInfo.sType = VK_STRUCTURE_TYPE_INSTANCE_CREATE_INFO;
createInfo.pApplicationInfo = &appInfo;
if (vkCreateInstance(&createInfo, nullptr, &instance) != VK_SUCCESS) {
throw std::runtime_error("failed to create Vulkan instance!");
}
Logical Device
Once your instance is created, the next step is to create a logical device, which allows you to communicate with the physical device (GPU).
Example Code Snippet for Logical Device Creation
VkDevice device;
VkDeviceCreateInfo deviceCreateInfo = {};
deviceCreateInfo.sType = VK_STRUCTURE_TYPE_DEVICE_CREATE_INFO;
if (vkCreateDevice(physicalDevice, &deviceCreateInfo, nullptr, &device) != VK_SUCCESS) {
throw std::runtime_error("failed to create logical device!");
}
Command Buffers
Command buffers are essential in Vulkan programming. They store commands before execution, enabling efficient rendering.
Recording Commands in Buffers
VkCommandBuffer commandBuffer;
vkBeginCommandBuffer(commandBuffer, &beginInfo);
vkEndCommandBuffer(commandBuffer);
This snippet outlines how to record commands within a command buffer, a crucial aspect of rendering in Vulkan.
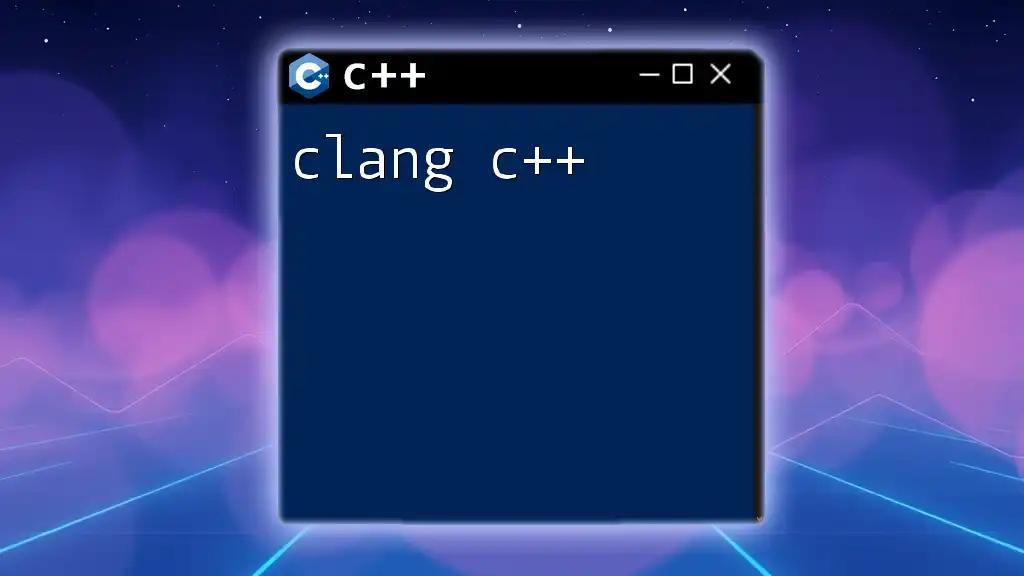
Setting Up a Rendering Pipeline
Overview of the Rendering Pipeline
The rendering pipeline is a series of steps that your graphics card follows to process data and produce images. Vulkan's pipeline consists of fixed-function stages and programmable stages.
Creating Shader Modules
Shader modules are integral to the rendering pipeline, as they define how your graphics are processed.
Writing and Compiling a Basic Shader in GLSL
Here's a simple vertex shader example written in GLSL:
#version 450
layout(location = 0) in vec3 inPosition;
void main() {
gl_Position = vec4(inPosition, 1.0);
}
You need to compile this shader using a tool like `glslangValidator` before using it as a shader module in Vulkan.
Configuring Pipeline States
The graphics pipeline state is configurable to optimize rendering as per your application's requirements.
Example Code Snippet for Pipeline Creation
VkGraphicsPipelineCreateInfo pipelineInfo = {};
pipelineInfo.sType = VK_STRUCTURE_TYPE_GRAPHICS_PIPELINE_CREATE_INFO;
// Additional pipeline state configurations
This code snippet shows how you'd begin setting up the graphics pipeline state, which is fundamental to rendering.
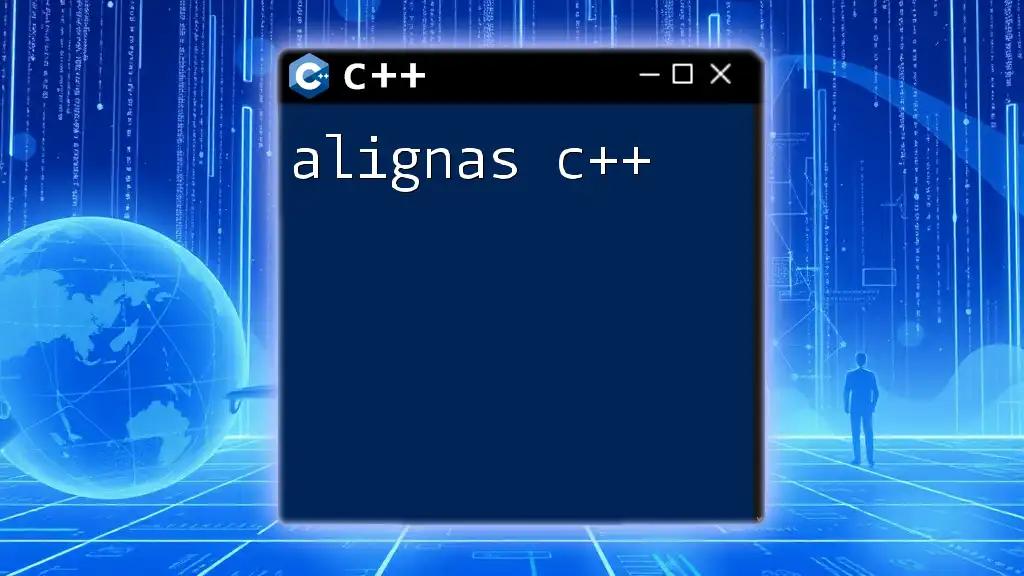
Drawing to the Screen
Framebuffers and Swapchains
Framebuffers and swapchains are closely linked in defining how rendered images are presented to the user.
-
Understanding Framebuffers: Framebuffers are collections of image resources that hold the rendered content before they are displayed.
-
Setting Up a Swapchain: The swapchain is an abstraction that manages presentation; it allows rendered images to be displayed on the screen.
Example Code Snippets for Swapchain Configuration
VkSwapchainCreateInfoKHR swapchainInfo = {};
swapchainInfo.sType = VK_STRUCTURE_TYPE_SWAPCHAIN_CREATE_INFO_KHR;
// Additional swapchain configurations
Drawing with Vulkan
To render your scene, you must submit command buffers and present images from the swapchain to the screen.
Example Code Snippet for Command Buffer Submission
vkQueueSubmit(graphicsQueue, 1, &submitInfo, nullptr);
This command submits your recorded command buffer to the graphics queue for processing.

Advanced Vulkan Concepts
Synchronization in Vulkan
Synchronization is critical when working with Vulkan, as multiple processes often run concurrently.
Understanding Synchronization Primitives
- Semaphores signal the completion of one operation before starting another.
- Fences ensure command buffer execution before proceeding.
Example Code Snippet for Synchronization
vkWaitForFences(device, 1, &fence, VK_TRUE, UINT64_MAX);
Memory Management
Effective memory management is vital in Vulkan due to its explicit control nature.
Allocating and Managing Memory in Vulkan
Vulkan requires you to explicitly allocate memory for buffers and images. This involves selecting the right memory type suited for your operation.
Example Code Snippets Detailing Memory Usage
VkMemoryAllocateInfo allocInfo = {};
allocInfo.sType = VK_STRUCTURE_TYPE_MEMORY_ALLOCATE_INFO;
// Additional memory allocation configurations

Debugging and Optimization
Common Vulkan Debugging Tools
For effective debugging, utilize Vulkan Validation Layers, which help catch errors during the development process.
Example Usage Scenarios
To enable validation layers, configure your instance create info to include them. This step will help in identifying issues quickly during development.
Performance Optimization Tips
To enhance the performance of your Vulkan applications:
- Profile your application at regular intervals.
- Follow best practices such as minimizing state changes, batching draw calls, and managing memory allocations smartly.
Example Dos and Don’ts for Developers
- Do utilize command buffers wisely.
- Don’t overuse synchronization primitives, which can lead to performance bottlenecks.

Conclusion
Recap of What You Learned
In this guide, we’ve explored the basics of the Vulkan API in C++, including setting up a development environment, understanding the graphics pipeline, and the essentials of rendering with Vulkan.
Next Steps in Your Vulkan Journey
For those keen to deepen their knowledge, consider exploring more advanced topics like compute shaders, ray tracing, or Vulkan’s extensions. Many resources are available, from online courses to community forums.
Call to Action
Now that you have a foundational understanding of using Vulkan API with C++, it's time to embark on your development journey! Start building your own projects and harness the full power of modern graphics programming.