The `clear` function in C++ is commonly used to remove all elements from a container, such as a vector or list, effectively resetting its size to zero.
Here’s a code snippet demonstrating the `clear` method on a `std::vector`:
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
myVector.clear(); // This will remove all elements from the vector
std::cout << "Size after clear: " << myVector.size() << std::endl; // Output: 0
return 0;
}
Understanding the `clear` Function
What is `clear` in C++?
The `clear` function in C++ is a member function provided by several standard library containers, including `std::vector`, `std::list`, and `std::map`. Its primary purpose is to remove all elements from a container, effectively resetting it to an empty state. This function is crucial for managing resources in your C++ applications.
Key Point: Understanding how `clear` operates is essential for effective memory management and optimizing performance in your programs.
Key Benefits of Using `clear`
-
Memory Management: When objects are stored in a container, they consume system memory. By using `clear`, you release that memory back to the system, ensuring efficient use of resources.
-
Avoiding Memory Leaks: Failing to clear or properly manage the contents of your containers can lead to memory leaks—situations where memory is no longer accessible but not returned to the system. This can cause your program to consume more memory than necessary.
-
Improving Performance: Containers can become bloated with unused objects over time, which can slow down your application. The `clear` function helps keep containers optimized, allowing for faster operations.
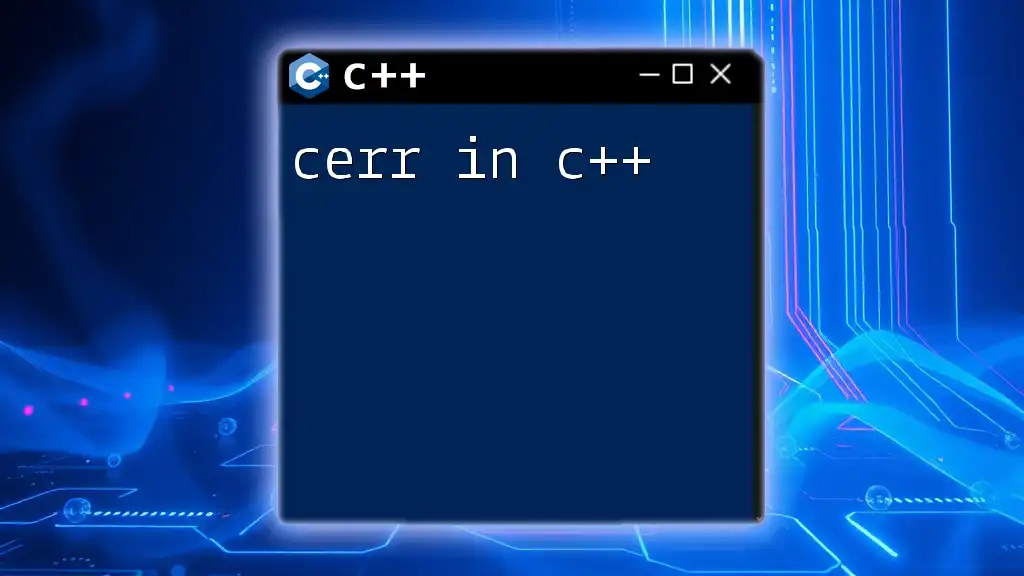
C++ Standard Library Containers with `clear`
Using `clear` with `std::vector`
The `std::vector` is one of the most commonly used containers in C++ due to its flexibility and automatic resizing. Using the `clear` function here is straightforward.
Example: Below is a demonstration of the `clear` function with `std::vector`.
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::cout << "Size before clear: " << numbers.size() << std::endl;
numbers.clear();
std::cout << "Size after clear: " << numbers.size() << std::endl;
return 0;
}
In this example, the initial size of the vector is 5. After calling the `clear` function, the size becomes 0, indicating that all elements have been removed.
Using `clear` with `std::list`
Similarly, `std::list` is a container that allows for the storage of elements in a non-contiguous manner, giving it unique strengths in specific scenarios, such as frequent insertions and deletions. The `clear` function can be applied just as easily with this container.
Example: Here's how to use `clear` with `std::list`.
#include <iostream>
#include <list>
int main() {
std::list<std::string> items = {"apple", "banana", "cherry"};
std::cout << "List size before clear: " << items.size() << std::endl;
items.clear();
std::cout << "List size after clear: " << items.size() << std::endl;
return 0;
}
In this scenario, the size of the list decreases from 3 to 0 after the `clear` operation is employed.
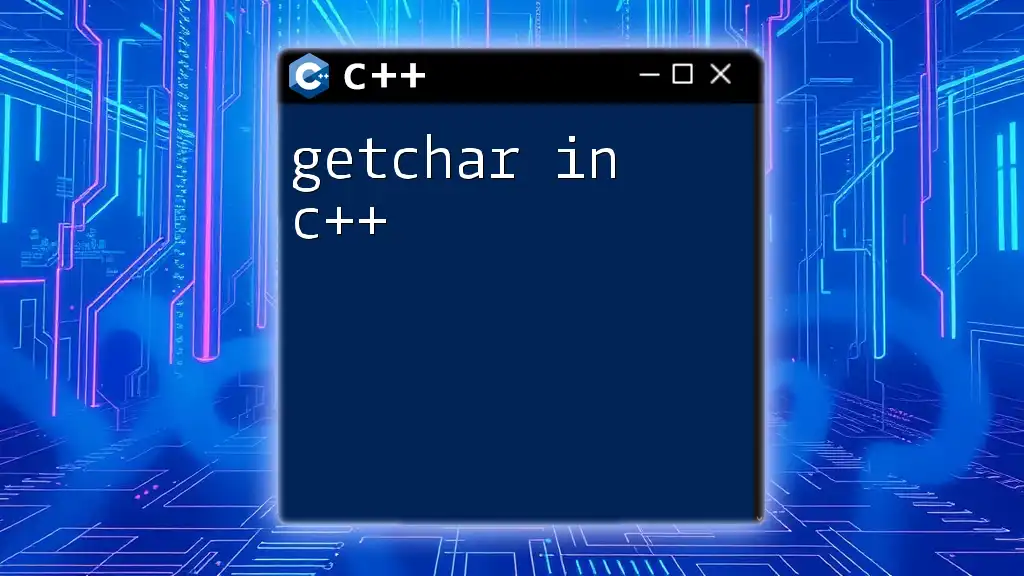
Using `clear` in Custom Classes
Implementing `clear` in Your Own Data Structures
When creating custom data structures, it’s essential to maintain a clear state for optimal resource management. Implementing a `clear` function in your custom classes can help manage the lifecycle of the object’s data effectively.
Best Practice: When writing your own `clear` method, ensure it resets all member variables to their initial states. This promotes clarity and prevents unintended behavior.
Example of a Custom Class with `clear`
Consider the following example of a `Student` class, which includes a `clear` method to reset its properties.
#include <iostream>
#include <string>
class Student {
public:
std::string name;
int age;
void clear() {
name.clear();
age = 0; // Reset age
}
};
int main() {
Student student;
student.name = "John";
student.age = 25;
student.clear();
std::cout << "Name: " << student.name << ", Age: " << student.age << std::endl;
return 0;
}
In this code, the `Student` object resets its `name` to an empty string and `age` to 0 when the `clear` function is called, illustrating the importance of defining a clear state.
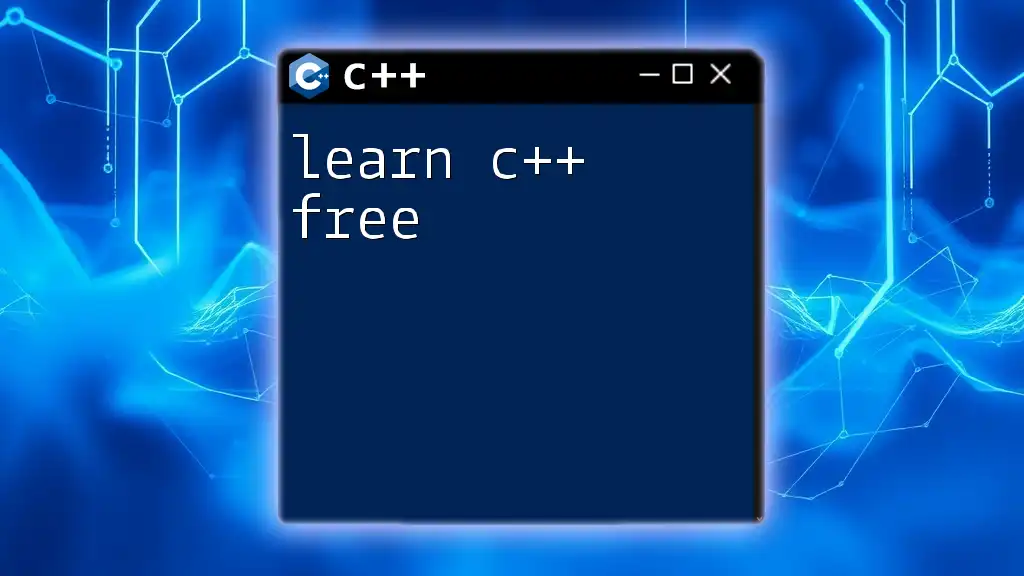
Common Misconceptions About `clear` in C++
Many new programmers may confuse the `clear` function with destructors. It's critical to understand that `clear` does not delete the container itself; instead, it removes the contents while keeping the container structure intact.
In Summary: While destructors free memory occupied by the entire object, `clear` only affects the elements stored within the container. Understanding this distinction is key to effective memory management in C++.
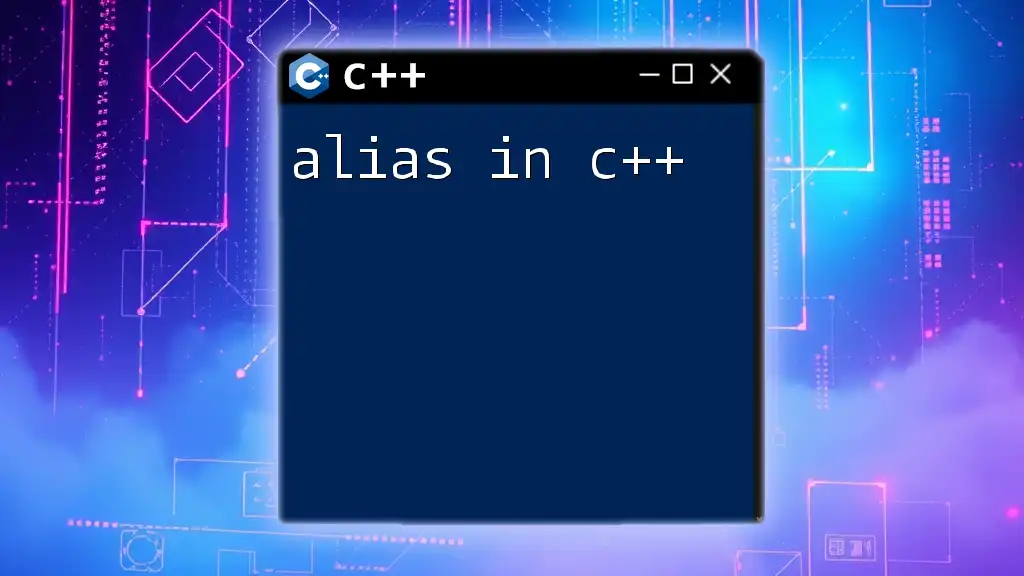
Conclusion
In C++, mastering the `clear` function is crucial for maintaining clarity in both data structures and memory management. By effectively utilizing `clear`, you can ensure that your programs remain efficient and free from common pitfalls like memory leaks.
By practicing the use of `clear` in various contexts, you will reinforce your understanding and proficiency in C++ programming. Remember, effective resource management leads to better performance, greater maintainability, and a more robust application design.
Happy coding!