"Learn C++ for free by exploring essential commands with practical examples, like the one below that demonstrates a simple 'Hello, World!' program."
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++
What is C++?
C++ is a powerful, high-performance programming language that builds upon the foundations laid by the C programming language. Developed by Bjarne Stroustrup in the late 1970s, C++ has evolved over the decades and has become a staple in software development, systems programming, game development, and even high-performance applications. One of its defining characteristics is its support for object-oriented programming (OOP), which enables developers to create modular and reusable code.
Key Features of C++
C++ has several key features that set it apart from other languages:
-
Object-Oriented Programming: C++ allows developers to define objects and classes, promoting code reuse and a clear organizational structure. Key OOP concepts include inheritance, where a new class can inherit properties from an existing class, and polymorphism, which enables a single function to handle different types of objects.
-
Standard Template Library (STL): The STL provides a collection of generic classes and functions, making common tasks such as sorting and searching far more efficient. By leveraging templates, developers can write code that works with any data type.
-
Performance: C++ is known for its speed and efficiency. By allowing low-level manipulation of data and memory, it’s possible to write code that is highly optimized for performance-critical applications.
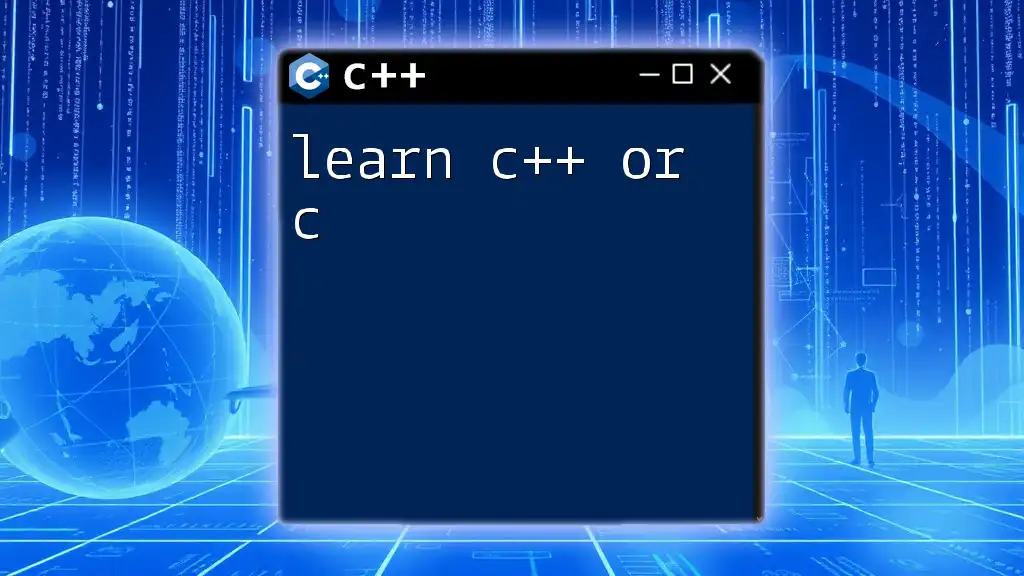
Resources for Learning C++ Free
Online Courses
Many online platforms offer free C++ courses. While resources may vary, consider the following popular options:
- Coursera: Offers courses from universities that introduce C++ in an academic context.
- Udacity: Provides project-based learning modules for a practical approach to C++.
- YouTube Channels: Channels such as The Cherno and ProgrammingKnowledge are particularly good for visual learners looking to grasp C++ concepts effectively through video tutorials.
E-books and Online Textbooks
A wealth of knowledge can be found in freely available e-books and online textbooks. Consider resources like:
- "C++ Primer" is a comprehensive guide that takes you through the language step-by-step if you can find links providing free access.
- Educational institution websites often provide helpful PDFs and open courseware that detail C++ programming.
Interactive Coding Platforms
Incorporate practical learning through coding:
- Codecademy: This interactive platform allows you to write and run C++ code directly in your browser, providing immediate feedback as you learn.
- HackerRank and LeetCode: These platforms offer various coding challenges that can help you solidify your understanding of C++ through practice.
Community and Forums
Joining communities can facilitate learning through discussion and inquiries:
- Platforms like Reddit (r/cpp) and Stack Overflow offer spaces to ask questions, share knowledge, and troubleshoot issues with other developers.
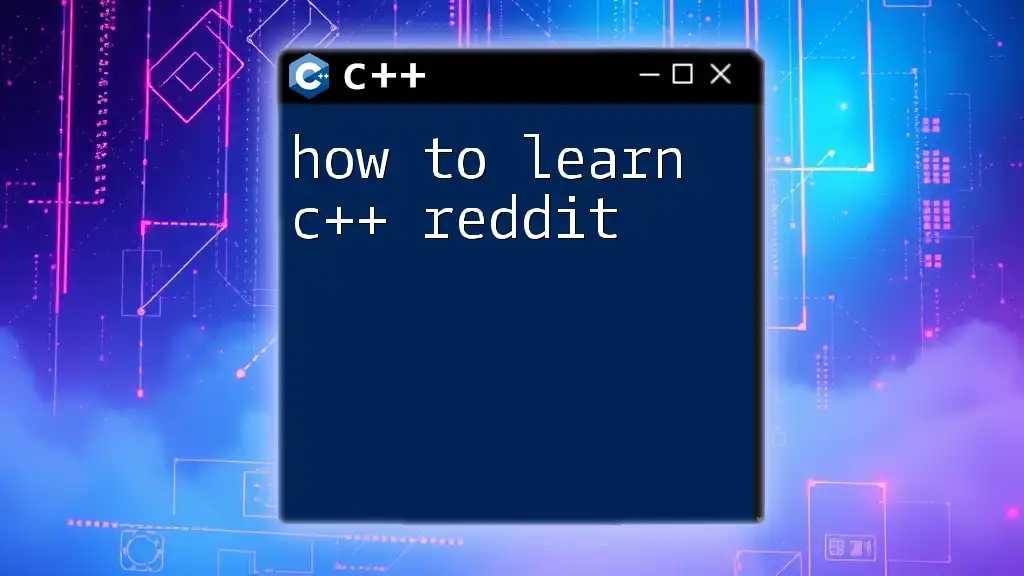
Getting Started with C++
Setting Up Your C++ Environment
To start programming in C++, you need the right development environment. There are several IDEs (Integrated Development Environments) and compilers available to choose from:
- Code::Blocks: A user-friendly IDE that’s particularly good for beginners.
- Visual Studio: A comprehensive development environment ideal for Windows users.
- g++: A widely used C++ compiler that can be set up through various command-line interfaces.
Follow a step-by-step guide to install your chosen IDE. Once installed, you can test your setup by writing a simple "Hello World" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Learning Basic Syntax
Understanding the basics of C++ syntax is crucial for starting your journey in learning C++.
Variables and Data Types
C++ supports several data types:
- int: Used for integers.
- float: For floating-point numbers.
- char: For characters.
Declaring variables is straightforward:
int age = 25;
float salary = 45000.50;
char initial = 'A';
Control Structures
Control structures like if statements and loops dictate how your code behaves.
If Statements: The basic syntax for an if statement is as follows:
if (age > 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Not an adult" << std::endl;
}
Loops: Loops help execute a certain block of code multiple times. Here’s an example of a `for` loop:
for(int i = 0; i < 5; i++) {
std::cout << i << " ";
}
Functions in C++
Functions are reusable blocks of code that perform specific tasks.
Function Declaration and Definition
Defining a function requires specifying the return type, function name, and parameters. Here's an example of a factorial function:
int factorial(int n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
Passing Arguments to Functions
Understanding how to pass arguments to your functions is essential. You can pass by value or by reference, each having its specific use cases.
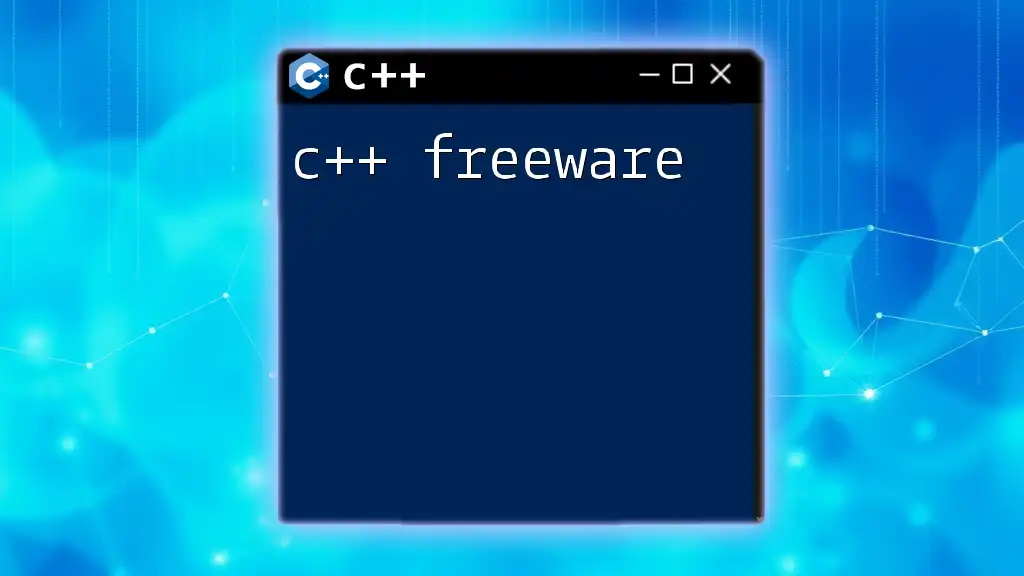
Advanced Topics in C++
Object-Oriented Programming Concepts
To harness the full potential of C++, it’s imperative to understand OOP concepts.
Classes and Objects
A class in C++ is essentially a blueprint for creating objects. Here’s how you would define a simple class:
class Car {
public:
string brand;
void start() {
std::cout << "Car started." << std::endl;
}
};
Inheritance and Polymorphism
Inheritance allows one class to inherit properties of another. For example:
class Vehicle {
public:
void honk() {
std::cout << "Beep!" << std::endl;
}
};
class Bike : public Vehicle {
};
Memory Management in C++
Memory management is an important aspect of programming in C++, particularly when dealing with dynamic data allocation.
Pointers and References
Understanding pointers is crucial, as they allow direct memory access:
int* ptr = new int; // dynamically allocate memory
*ptr = 10; // assign value
delete ptr; // free memory
Understanding Smart Pointers
Smart pointers like `unique_ptr` and `shared_ptr` help manage memory automatically and prevent leaks.
#include <memory>
std::unique_ptr<int> ptr = std::make_unique<int>(10);
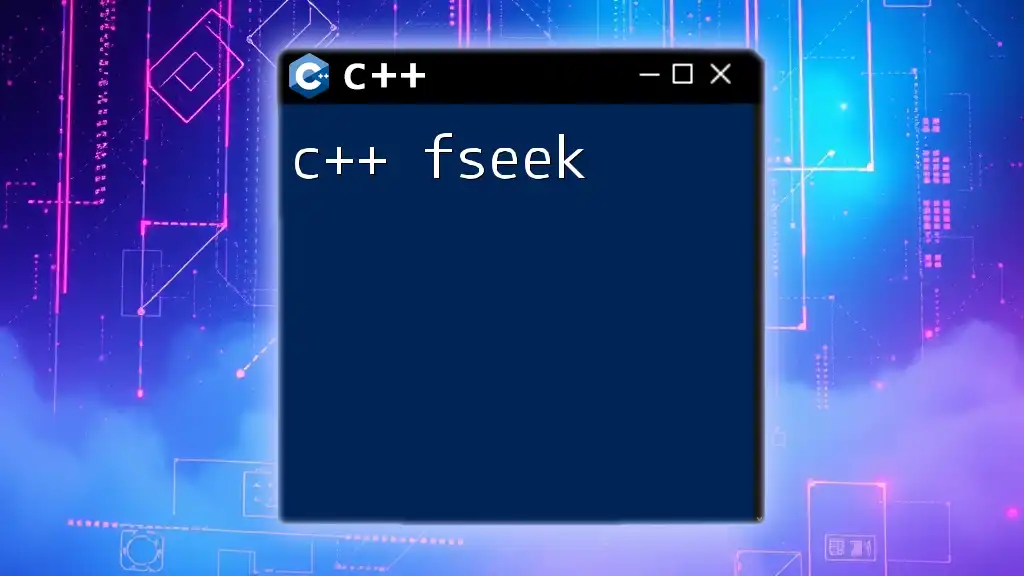
Practical Projects to Enhance Your Skills
Building a Simple Calculator
Creating a command-line interface (CLI) calculator is an excellent way to apply theoretical knowledge. Start by defining basic operations like addition, subtraction, multiplication, and division, and build functions for each.
Creating a Text-Based Game
Developing a simple text-based game can help reinforce many C++ concepts while enhancing your programming mindset. Define the game’s mechanics, such as turns and choices, then implement the game logic using C++ constructs you’ve learned.
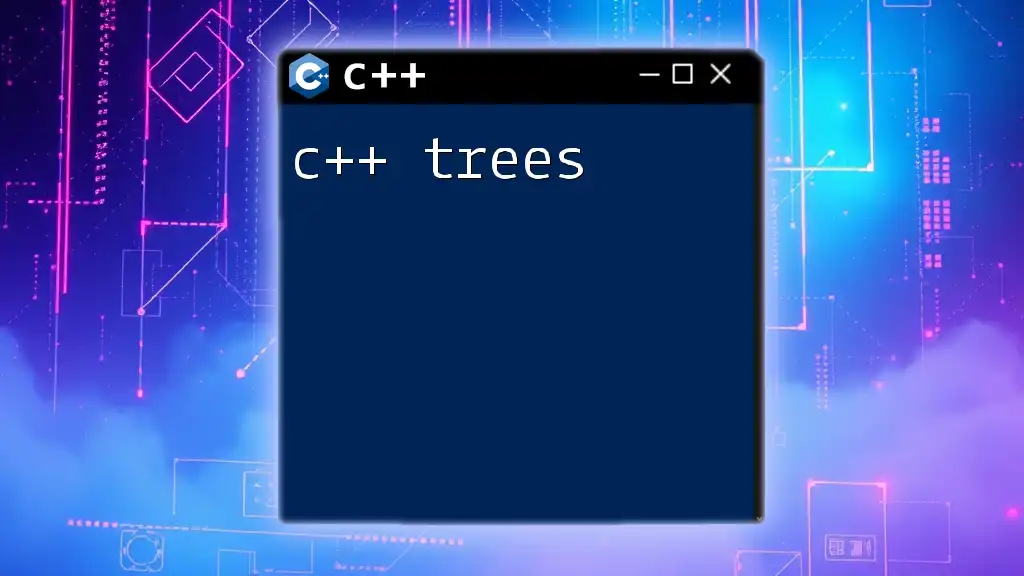
Additional Tips for Learning C++
Best Practices
Writing clean and maintainable code is vital for any programmer. Pay attention to formatting, use comments judiciously, and follow naming conventions.
Keeping Updated with C++ Innovations
The C++ language continues to evolve, and it’s important to stay informed about new standards and features. Follow C++ development through industry blogs, conferences, and community forums.
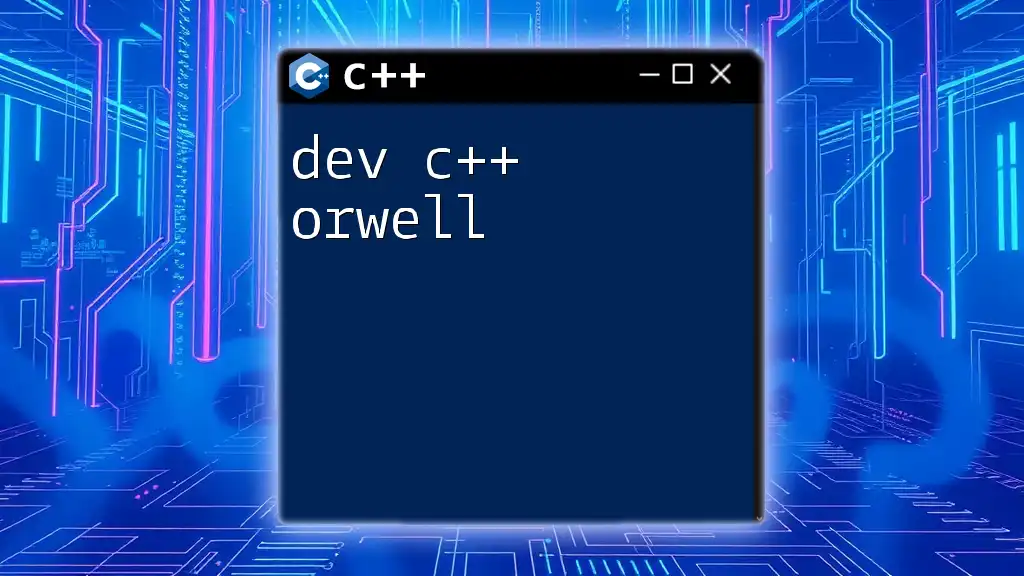
Conclusion
Throughout this guide, you have explored the myriad resources available to learn C++ free. Whether you engage with online courses, interactive platforms, or coalesce learning through community forums, a consistent practice of coding and problem-solving will solidify your skills. Embrace the journey, explore advanced topics, and become part of the thriving C++ community.
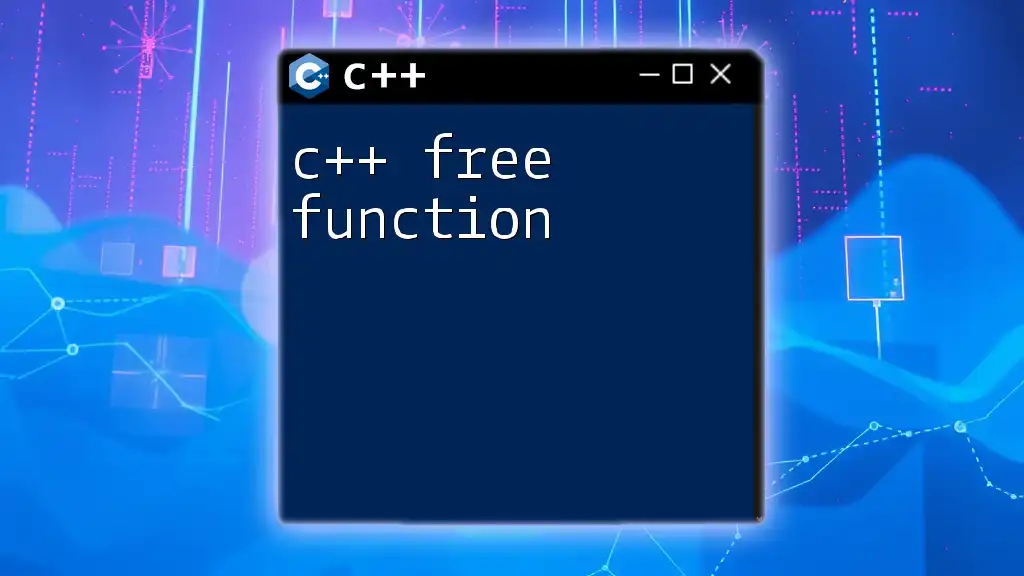
FAQs
As you pursue your journey in C++, you may encounter common questions. Feel free to explore forums and community spaces to find answers or seek assistance.
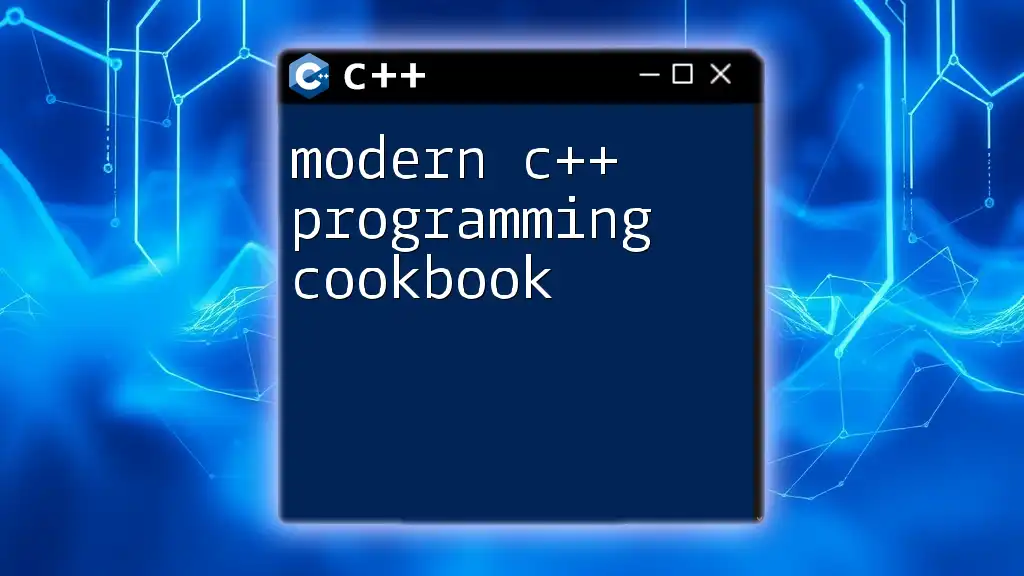
References
For more information, consider the resources linked throughout the article, which collectively form a robust platform for your C++ learning adventure.