"Expert C++ Read Online" provides quick access to essential C++ commands and best practices, allowing learners to harness the power of C++ efficiently.
Here’s a simple C++ function that demonstrates the use of conditional statements:
#include <iostream>
using namespace std;
void checkNumber(int num) {
if (num > 0) {
cout << "The number is positive." << endl;
} else if (num < 0) {
cout << "The number is negative." << endl;
} else {
cout << "The number is zero." << endl;
}
}
Understanding C++
What is C++?
C++ is a powerful general-purpose programming language that was created by Bjarne Stroustrup during the early 1980s. It extends the C programming language, incorporating object-oriented features, making it suitable for a wide range of software development, including systems software, application software, and game development. Key features of C++ include:
- Object-Oriented Programming: This allows developers to create classes that encapsulate data and behavior, promoting code reuse and organization.
- Performance and Efficiency: C++ is designed to give high performance and control over system resources, making it ideal for resource-intensive applications.
Why Learn C++?
There are numerous compelling reasons to learn C++, distinguishing it as an essential language for both novice and experienced programmers alike:
- Versatility in Application Development: C++ is used in various domains such as finance, gaming, and systems programming. Its versatility makes it a valuable addition to any programmer's skill set.
- Job Opportunities in the Tech Industry: With many companies seeking C++ developers for performance-critical applications, mastering this language can significantly enhance your career prospects.
- Significance in System Programming: Understanding C++ is crucial for low-level programming, as it provides a better grasp of how programs interact with hardware.
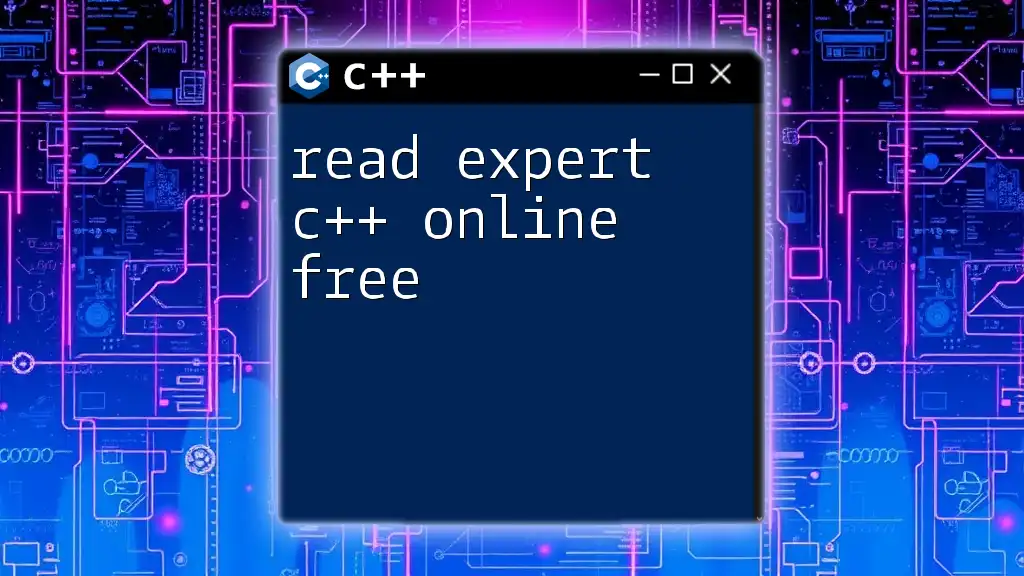
Benefits of Online Learning for C++
Flexibility and Convenience
The primary advantage of online learning is the ability to learn at your own pace. You can access resources anytime and anywhere, allowing you to balance your studies with work or personal commitments.
Diverse Learning Resources
There is a wealth of online resources available for learning C++. This diversity includes various formats such as:
- Video tutorials that make it easy to visualize complex concepts.
- Online courses offering structured curriculums.
- E-books and reference guides for in-depth study and quick reference.
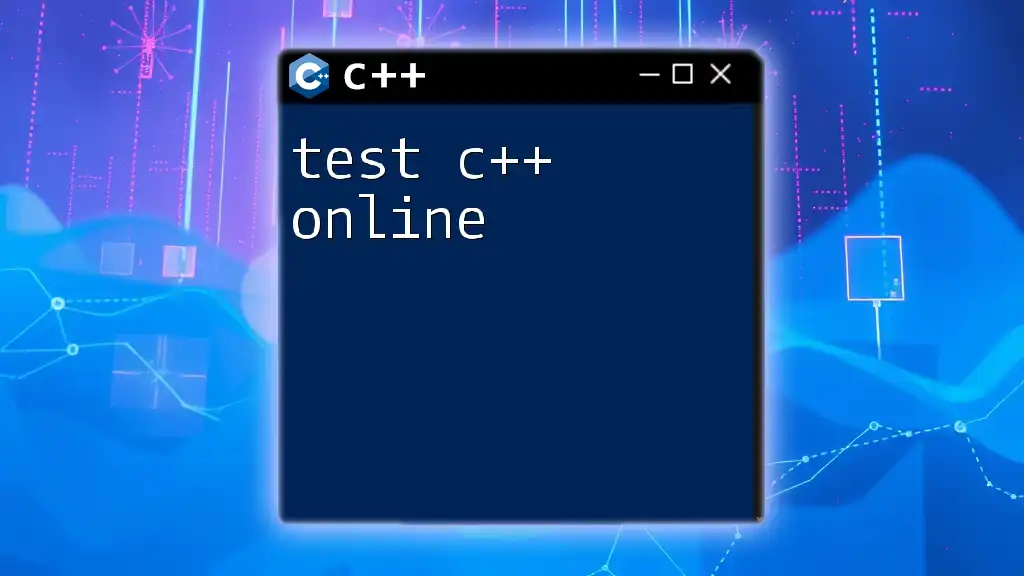
Finding Expert C++ Resources Online
Online Courses
Several popular platforms provide comprehensive courses tailored to different learning levels, including:
- Udemy: Various C++ courses covering beginner to advanced topics, complete with downloadable resources and certificates.
- Coursera: Offers courses from top universities, often focusing on practical applications.
- edX and Pluralsight: Provide a mix of foundational courses and advanced specializations.
When selecting a course, evaluate factors such as course content, structure, reviews, and ratings to ensure quality learning.
Tutorials and Blogs
There are several industry-recognized blogs and websites dedicated to C++. Some recommended resources are:
- CPlusPlus.com: A community-driven site featuring tutorials, references, and forums for asking questions.
- LearnCPP.com: A well-structured tutorial site covering everything from the basics to advanced techniques.
- Stack Overflow: An invaluable resource for problem-solving with a plethora of questions and answers focused on C++.
To gauge the quality of tutorials, always check the depth of content and the quality of code examples provided, as these can greatly influence your learning experience.
YouTube Channels
Visual learners may benefit significantly from YouTube channels dedicated to programming. Some top channels include:
- The Cherno: Offers in-depth videos on C++ concepts, game development, and real-world projects.
- freeCodeCamp: Provides full-length C++ courses available for free, grounded in practical applications.
- ProgrammingKnowledge: A great resource for beginners, with clear explanations of fundamental C++ concepts.
Benefits of Video Learning
Video tutorials can enhance your understanding through visual representation. They often provide additional context through real-life applications and community interaction in the comments section.
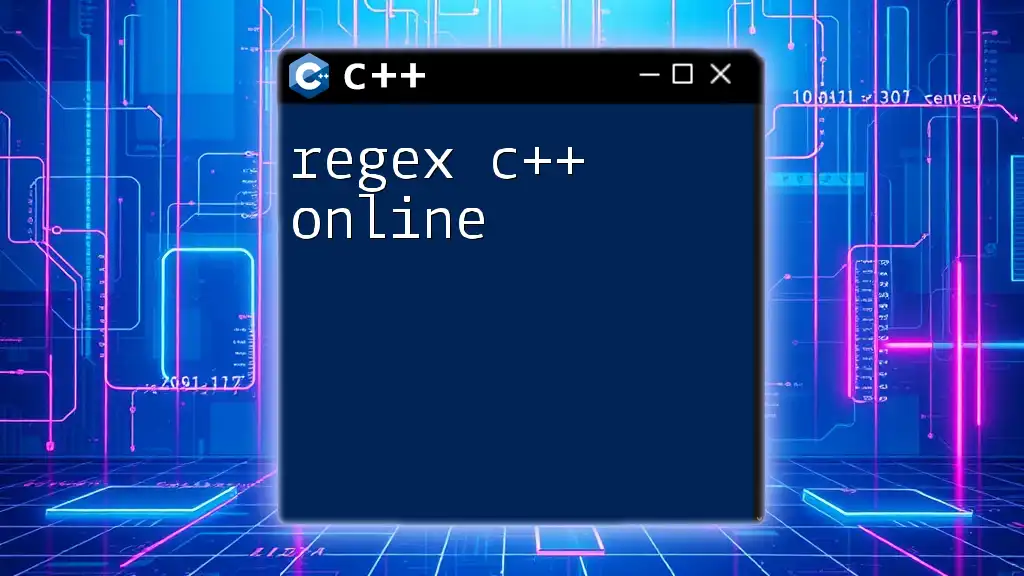
Practical Application of C++
Hands-On Learning
The most effective way to master C++ is through consistent practice. Engage in real-time coding by utilizing resources for coding challenges like:
- LeetCode: Focused on algorithmic challenges and interview prep.
- HackerRank: Offers various problems with immediate feedback.
- Codewars: Engages users through gamified coding challenges.
Open Source Projects
Participating in open-source projects can significantly deepen your understanding of C++. These projects often require contributions, allowing you to interact with experienced developers.
Benefits of Contributing to Open Source
- Real-World Experience: You gain practical experience and exposure to coding standards.
- Networking: Opportunities to engage with professionals and build a digital footprint.
Notable C++ Projects
Examples of significant C++ projects you can consider for contributions include Mozilla Firefox, MySQL, and Blender. A common beginner contribution could include fixing a minor bug or adding a feature, which can boost your confidence and skills.
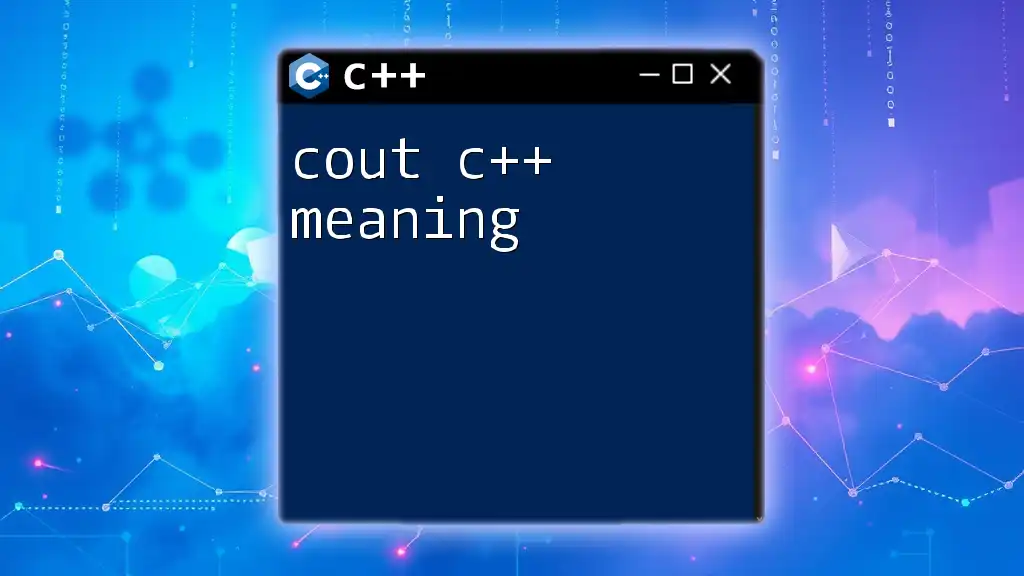
Expert Tips for Mastering C++
Build a Strong Foundation
A solid understanding of the foundational concepts of C++ is crucial for advanced learning. Focus on:
- Syntax: The rules that define the structure of the code.
- Loops, Conditionals, and Functions: Basic constructs to control flow and modularize code.
As an initial coding example, consider the following simple C++ program:
#include <iostream>
using namespace std;
int main() {
int a = 5;
cout << "Hello, C++ World!" << endl;
return 0;
}
Code Regularly
Set aside time each day for coding practice. Engaging consistently with programming will solidify your understanding and increase comfort with syntax and concepts.
Join C++ Communities
Engaging with online forums and developer networks can provide you with valuable insights and support. Recommended channels include:
- Reddit: Subreddits dedicated to C++ discussions and queries.
- Discord: Join servers focused on C++ for live chats and events.
- Stack Overflow: Don’t hesitate to ask questions and attempt to answer others – this is a great way to learn.
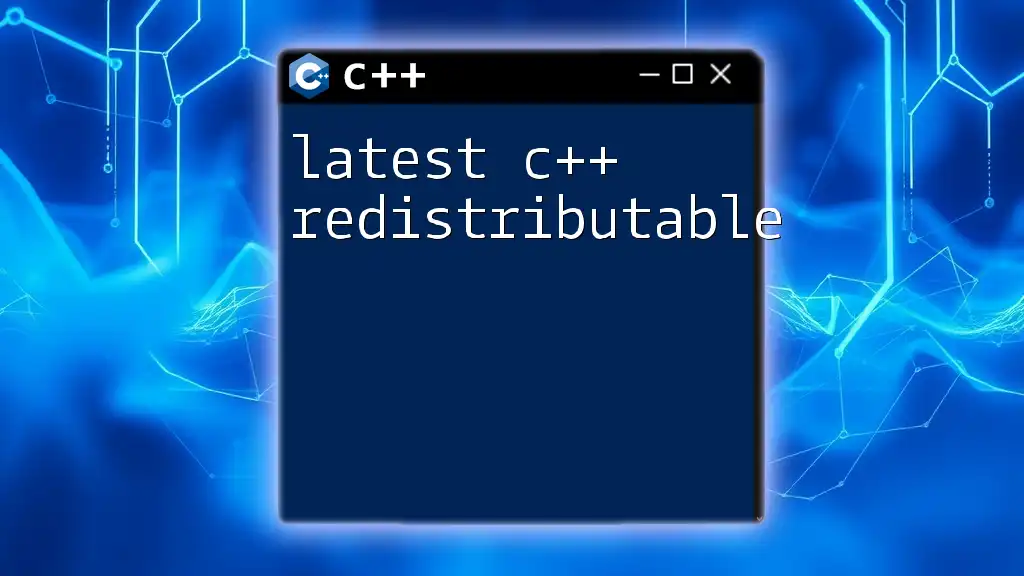
Common Challenges in Learning C++
Overcoming Resource Overload
With limitless online content available, it can be easy to feel overwhelmed. To combat this, prioritize high-quality content and set achievable learning goals. Define what you want to focus on each week to create a structured learning path.
Debugging Skills Development
Mastering debugging is essential in programming. Tools like GDB (GNU Debugger) can help identify issues in your code. Pair your learning with practical exercises to enhance your debugging skills, as this will greatly improve your problem-solving capabilities.
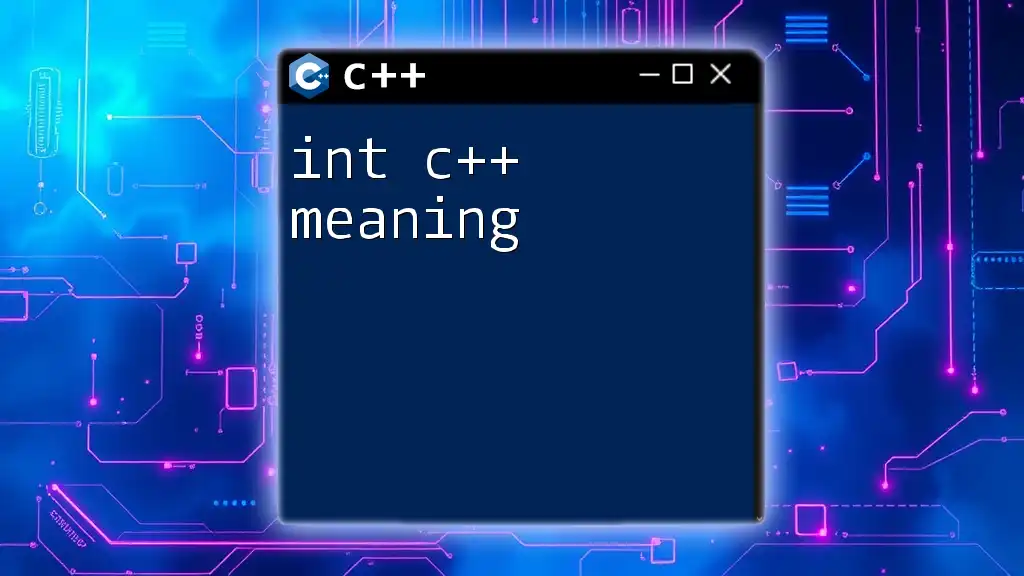
Conclusion
By using reliable online resources to learn C++, you can build a solid foundation and gain practical skills essential for your programming career. Whether you’re a beginner or looking to deepen your existing knowledge, the journey to becoming proficient in C++ is filled with opportunities for growth. So, take the first step today and start utilizing these expert resources to master C++ online!
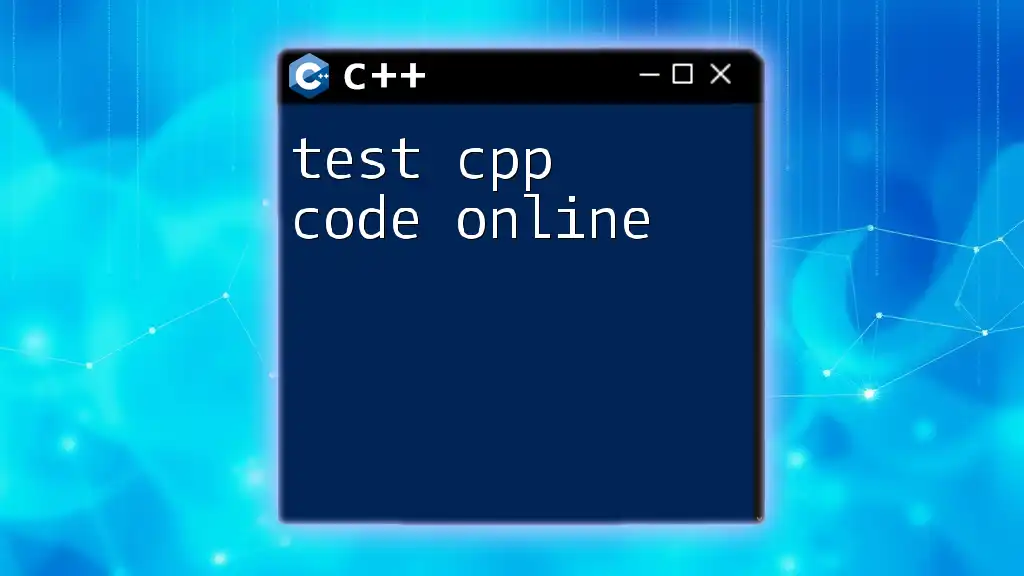
Additional Resources
You might also want to explore further books or platforms such as:
-
Books for Further Reading
- "C++ Primer" by Stanley B. Lippman offers an excellent foundation for beginners.
- "Effective C++" by Scott Meyers provides advanced tips and best practices.
-
Online Practice Sites
- Codecademy for interactive learning.
- GitHub for practicing version control and contributing to collaborative projects.
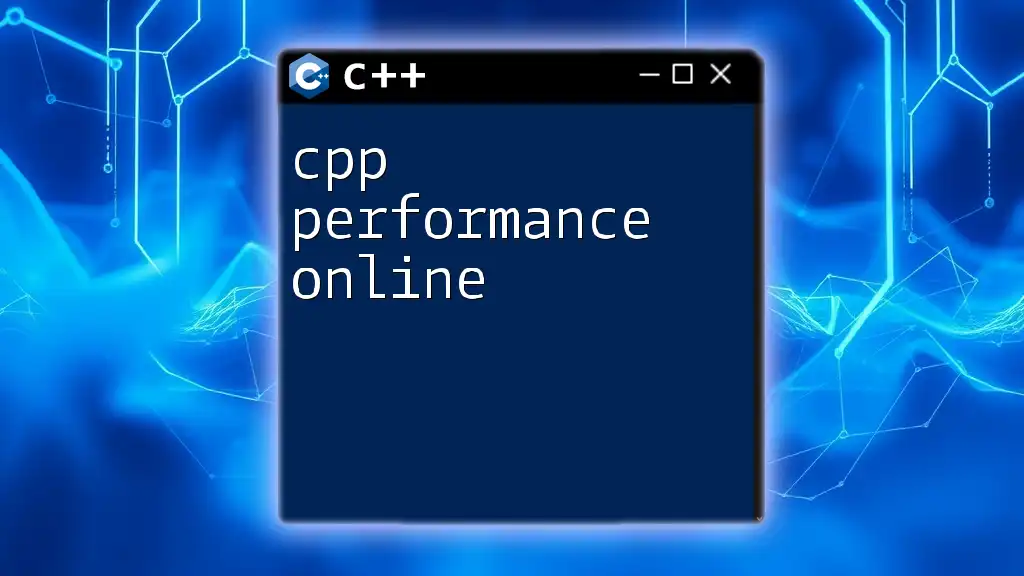
Frequently Asked Questions (FAQs)
What is C++ used for?
C++ is widely used in various domains such as game development (e.g., Unreal Engine), system/software development (e.g., operating systems), and embedded programming.
How long does it take to learn C++?
The time required can vary based on several factors: prior programming experience, learning pace, and depth of focus. However, with consistency, you can grasp the fundamentals in a matter of weeks.
Can I learn C++ with no programming background?
Absolutely! While prior programming knowledge can be beneficial, numerous resources are tailored specifically for beginners, helping them build a strong foundation in C++.