To check the installed version of C++ on a Linux system, you can use the following command in the terminal:
g++ --version
This command outputs the version of the GNU C++ compiler (g++) currently installed on your system.
Why Check Your C++ Version?
Knowing how to check your C++ version on Linux is crucial for several reasons.
Compatibility Issues
C++ is an evolving language, and each version introduces new features, standard library updates, and differences in compiler behavior. If you're working on complex projects or using libraries that depend on specific C++ features, ensuring your C++ version aligns with the library's requirements is essential. Using deprecated features can lead to bugs and unexpected behavior, and each newer version typically phases out older constructs.
Performance Improvements
With each C++ version, performance enhancements are rolled out that can significantly affect the efficiency of your applications. Features like move semantics in C++11 or concepts in C++20 can improve both speed and memory efficiency, making it critical to target and utilize the latest standards.
Compilation and Development Needs
Some modern C++ features, required for various development frameworks and libraries, won't work with older compilers. Having the right C++ version installed ensures you can compile and run code successfully, thus enabling smoother development processes.
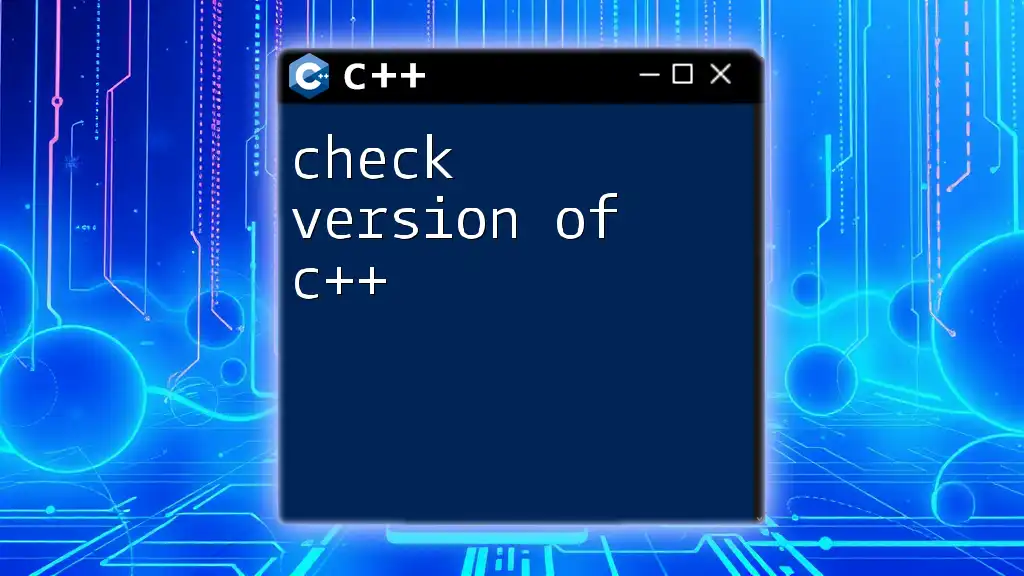
Methods to Check C++ Version in Linux
Using g++ Compiler
One of the most straightforward methods to check your C++ version on Linux is via the `g++` compiler. `g++` is part of the GNU Compiler Collection and is widely used among C++ developers.
Command Line Check
You can easily check the version of `g++` installed on your system by running the following command in your terminal:
g++ --version
The output will display the version of `g++` installed. For example:
g++ (GCC) 9.3.0
This output indicates that you are using GCC version 9.3.0. Each version of `g++` often defaults to a specific C++ standard, so knowing your version helps you understand what C++ features you can use.
Compiler Version Implications
It's essential to note how `g++` version corresponds to the default C++ standard:
- g++ 4.8 and earlier versions default to C++98.
- g++ 4.9 supports C++11 after enabling it explicitly.
- g++ 5.0 onward, C++14 becomes more accessible, and later versions include C++17 and C++20 seamlessly.
This progression indicates that if you want to use specific C++ features, you may have to specify the standard manually in your compilation commands.
Using Predefined Macros
Another effective way to check your C++ version on Linux is through predefined macros during the compilation process.
Utilize Predefined Macros
C++ provides a macro named `__cplusplus`, which evaluates as a long integer corresponding to the version of the standard being used at compile time. Here’s an example code snippet to demonstrate how to check the C++ version programmatically:
#include <iostream>
int main() {
std::cout << "C++ version: " << __cplusplus << std::endl;
return 0;
}
In this code, `__cplusplus` will yield different values based on the C++ standard in use:
- `201103L` for C++11
- `201402L` for C++14
- `201703L` for C++17
- `202002L` for C++20
This method is particularly helpful when you're unsure of the compiler settings or when working in an environment where multiple compilers are installed.
Using pkg-config
For projects that rely on specific libraries, `pkg-config` can be valuable in determining the required C++ version.
Checking Specific Libraries
You can use the following command to check the C++ flags needed for a library:
pkg-config --cflags --cxxflags <library-name>
Replace `<library-name>` with the actual library you are interested in. The output will often include compiler flags indicating which C++ version it is expecting or supporting, thus helping you align your compiler settings accordingly.
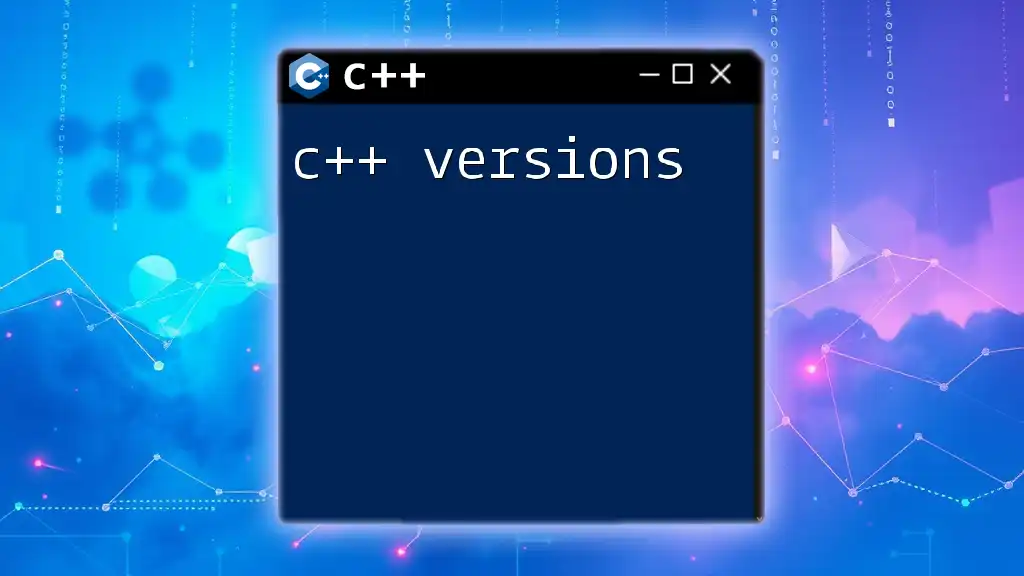
Common C++ Compilers and Their Versions
GCC (GNU Compiler Collection)
As one of the most popular compilers for C++ on Linux, GCC is constantly updated. To check the version of GCC specifically, use:
gcc --version
The output will be similar to that of `g++`, confirming which version you are currently running.
Clang
Another highly efficient compiler is Clang. To check the version of Clang, simply run:
clang --version
This compiler is recognized for its speed and error reporting, and knowing the Clang version can be crucial for projects that prefer this compiler.
Other Compilers
-
Intel C++ Compiler: Aimed at performance-critical applications. To check its version, you can run:
icpc --version
-
Oracle Developer Studio: Known for its robust support for enterprise applications. You can check its version similarly by using:
g++ --version
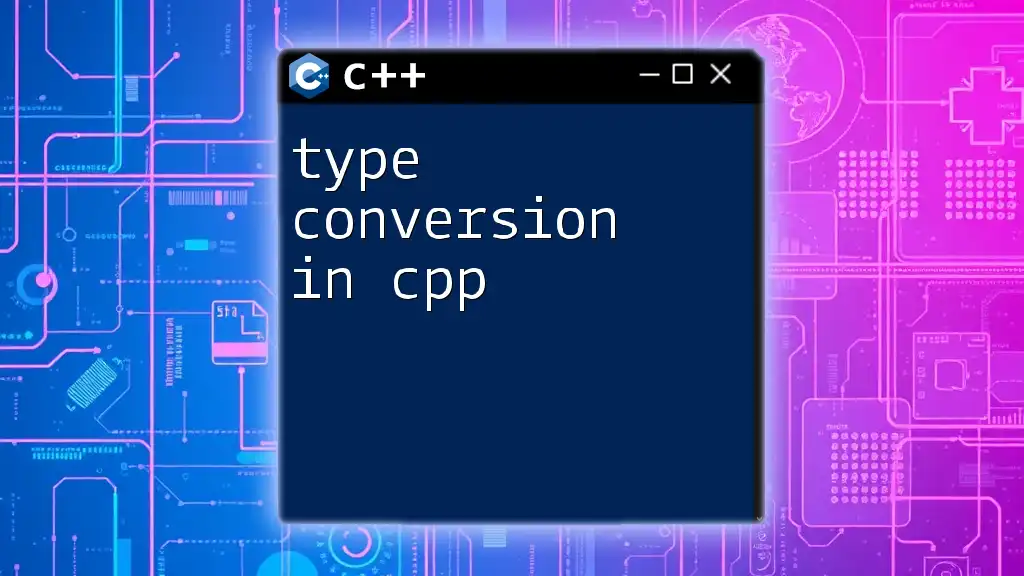
Upgrading Your C++ Compiler on Linux
If you discover that your C++ version is outdated, it’s essential to upgrade. Here’s how you can do that on popular Linux distributions.
Installation Process
For Ubuntu/Debian-based Distributions
To install the latest version of the GCC compiler, use the following commands:
sudo apt update
sudo apt install g++
This command will fetch the latest `g++` from the repository.
For Red Hat/Fedora-based Distributions
On these distributions, you can upgrade with the following command:
sudo dnf install gcc-c++
Verifying the Update
After installing or upgrading, it’s essential to re-run the version check commands you learned earlier. This ensures that you're using the intended version:
g++ --version
You should also test the predefined macro method with the example code provided to ensure you can utilize the necessary C++ features.
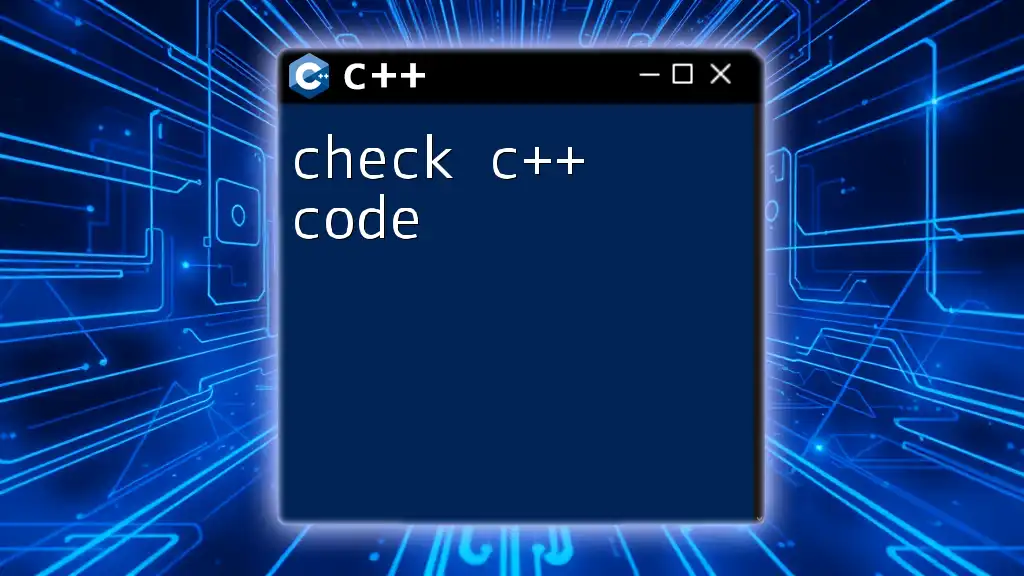
Conclusion
Understanding how to check your C++ version on Linux is vital for effective C++ programming and project compatibility. Regularly updating your compiler not only enhances performance but also allows you to leverage new features that can simplify complex tasks.
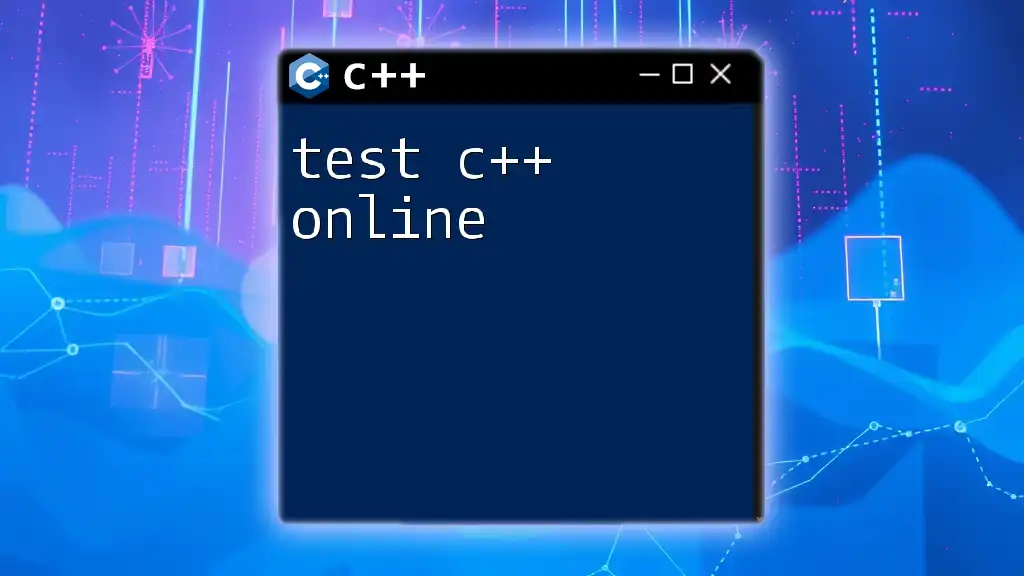
Frequently Asked Questions
What happens if I use an outdated C++ version?
Using an outdated version can lead to compatibility issues, a lack of access to modern features, and potential bugs related to deprecated features. This can significantly hamper your development efficiency.
Can I run multiple versions of C++ on the same machine?
Yes, utilizing version managers, containers, or even different compiler installations can help manage multiple C++ versions on the same machine. This flexibility allows developers to work across various projects with differing standards.
Why is my code not compiling with a specific C++ version?
Compilation errors often arise from feature incompatibility. Older versions may not support newer constructs or features, leading to confusion and additional debugging efforts. Always ensure your code matches the capabilities of the C++ version you are targeting.
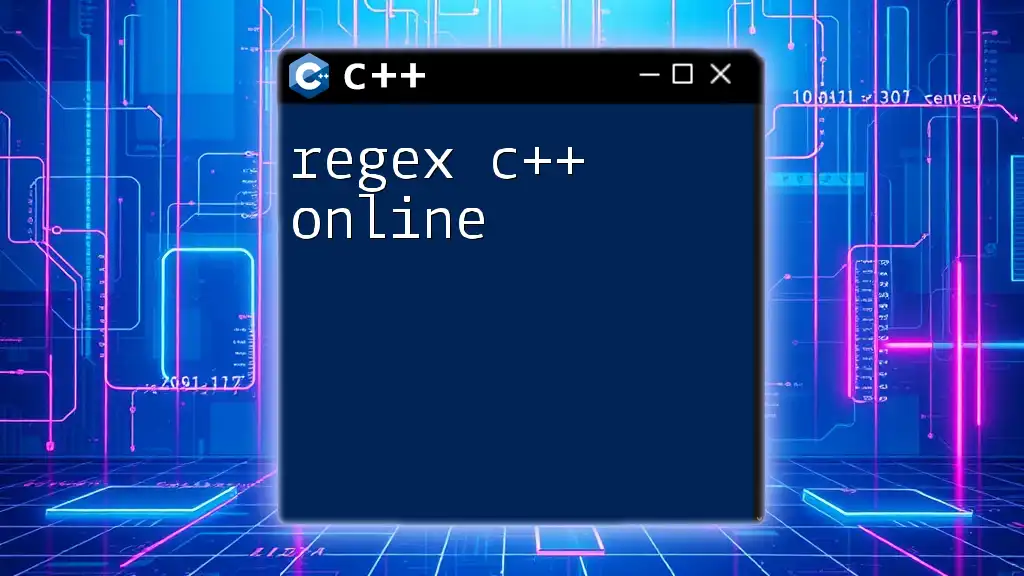
Additional Resources
For further exploration of the topic, consider checking out the official documentation for the GCC and Clang compilers, enrolling in online C++ courses, or participating in community forums for troubleshooting and support. These resources can solidify your understanding and improve your proficiency in using C++.