"Regex in C++ allows developers to perform pattern matching and string manipulation using the `<regex>` library, making it easy to work with text data."
Here's a simple code snippet demonstrating how to use regex in C++ to find all occurrences of a pattern in a string:
#include <iostream>
#include <regex>
#include <string>
int main() {
std::string text = "The rain in Spain stays mainly in the plain.";
std::regex pattern("ain");
std::smatch matches;
while (std::regex_search(text, matches, pattern)) {
std::cout << "Found: " << matches[0] << std::endl;
text = matches.suffix().str();
}
return 0;
}
Understanding Regex
What is Regex?
Regex, or regular expressions, are a sequence of characters that form a search pattern. They are widely used in programming for searching, manipulating, and validating strings. Regex can match specific patterns within text, facilitating various tasks like data extraction, input validation, and formatting modifications.
Importance of Regex in C++
The use of regex in C++ comes with several advantages, making it a valuable tool for developers:
- String Manipulation: Regex provides a powerful syntax for searching and replacing text patterns efficiently.
- Data Validation: Regex makes it easy to confirm that an input string meets certain criteria, such as an email address format.
- Text Processing: Applications often require parsing of strings for meaningful data extraction, and regex excels in handling such tasks.
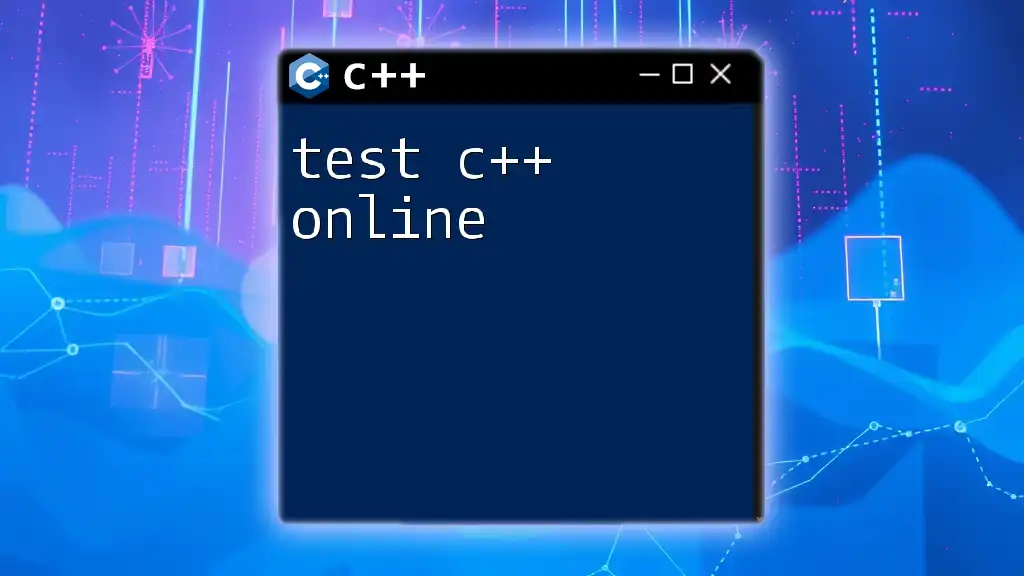
Getting Started with Regex in C++
The Regex Library
C++'s regex capabilities are harnessed through the `<regex>` library, which offers comprehensive functionality for working with regular expressions. To use regex in your C++ program, include the library at the beginning of your code:
#include <regex>
Basic Components of Regex
Regex Syntax Basics
Regex syntax includes characters and metacharacters that enable flexible pattern matching. Here are a few fundamental concepts:
- Characters: Simple letters and digits that match themselves.
- Metacharacters: Special characters (like `.`, `*`, `+`, etc.) that have specific meanings. For instance:
- `.` matches any character except newline.
- `*` matches zero or more occurrences of the preceding element.
- `^` asserts the start of a string.
- `$` asserts the end of a string.
Setting Up Your C++ Environment
For those eager to jump into regex coding, online compilers provide a quick and accessible way to practice without installing any software. Platforms like Repl.it, OnlineGDB, and JDoodle allow you to run C++ code snippets swiftly.
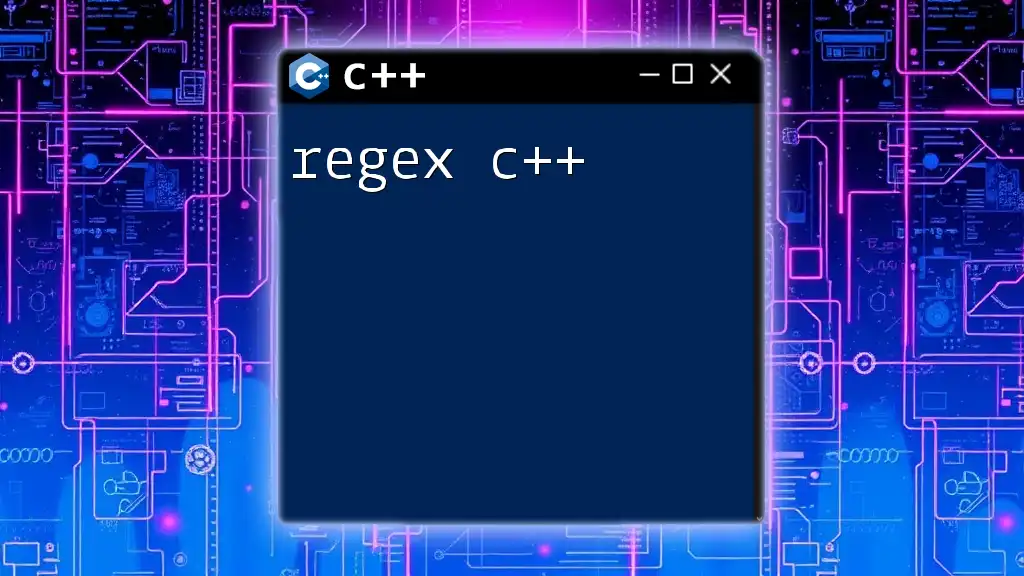
Core Regex Commands in C++
Creating a Regex Object
To utilize regex in C++, you need to create a `std::regex` object which encapsulates the regular expression pattern. Here’s how to declare and initialize a regex object:
std::regex myRegex("[a-zA-Z]+");
This pattern matches sequences of one or more letters, both uppercase and lowercase.
Matching Patterns
`std::regex_match` vs. `std::regex_search`
In C++, two key functions are used to match patterns:
- `std::regex_match`: This function checks if an entire string matches a pattern.
- `std::regex_search`: This function looks for a match of the pattern anywhere within the string.
Here’s a code snippet that illustrates both functions:
std::string text = "Hello World";
std::regex pattern("Hello");
bool fullMatch = std::regex_match(text, pattern); // false
bool partialMatch = std::regex_search(text, pattern); // true
Replacing Text
The `std::regex_replace` function allows you to replace matched patterns with another string, making it useful for text formatting or sanitization. Here’s an example that removes all digits from a string:
std::string input = "Report 123 has 4 errors.";
std::regex digitPattern("[0-9]+");
std::string result = std::regex_replace(input, digitPattern, "");
std::cout << result; // Output: "Report has errors."
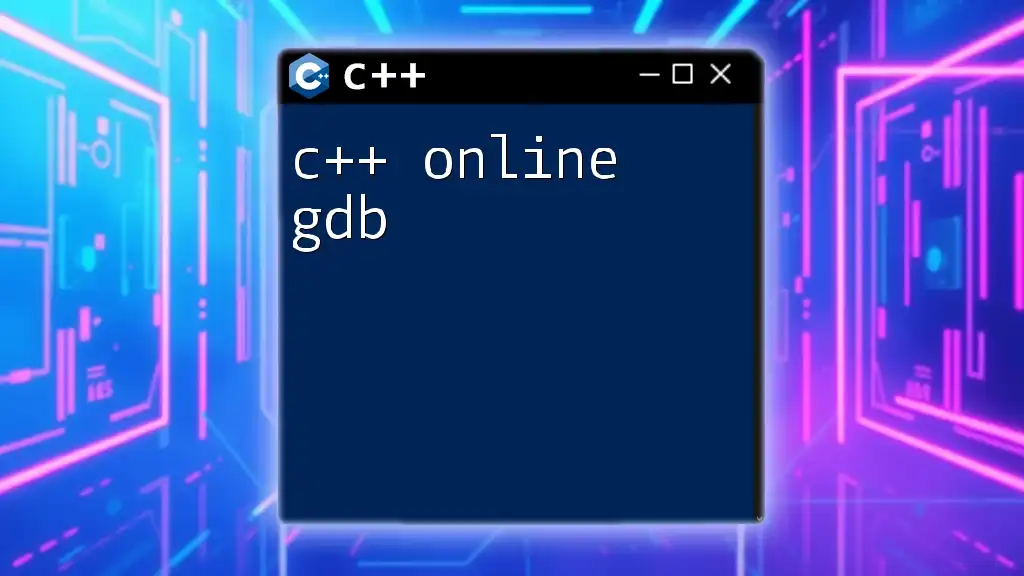
Advanced Regex Techniques
Using Capture Groups
Capture groups enhance regex capabilities by allowing you to extract specific portions of a match. Grouping is achieved using parentheses `()`. For instance, consider extracting the area code and the phone number:
std::string phone = "(123) 456-7890";
std::regex phonePattern("\\((\\d{3})\\) (\\d{3})-(\\d{4})");
std::smatch matches;
if (std::regex_search(phone, matches, phonePattern)) {
std::cout << "Area code: " << matches[1] << "\n"; // Output: Area code: 123
}
Flags and Modifiers
Using flags such as `std::regex::icase` enables case-insensitive matches, allowing `regex` to match patterns regardless of their case. Here’s an example demonstrating this feature:
std::string input = "Hello World";
std::regex pattern("hello", std::regex::icase);
bool matches = std::regex_match(input, pattern); // true
Error Handling with Regex
Managing exceptions is crucial when using regex, as improper patterns or unsupported operations can lead to runtime errors. Key exceptions include `std::regex_error`, which can indicate issues with invalid patterns. Here’s how to handle such exceptions:
try {
std::regex wrongPattern("[");
} catch (const std::regex_error& e) {
std::cerr << "Regex error: " << e.what();
}
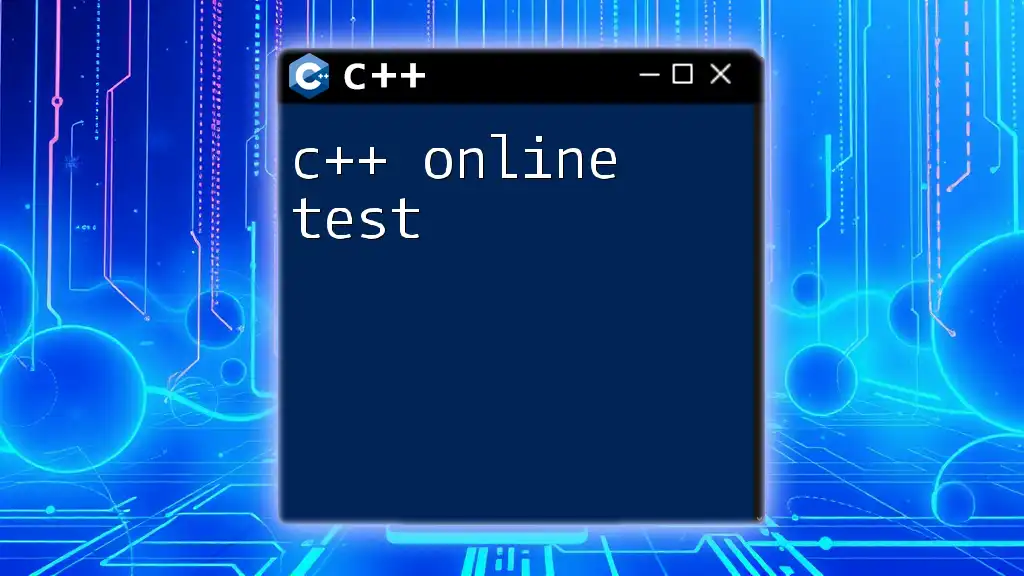
Practical Applications of Regex in C++
Data Validation
You're often required to validate user input against specific requirements. Regex excels in confirming whether an email address is formatted correctly. Here’s a typical email validation regex:
std::string email = "example@domain.com";
std::regex emailPattern("^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}$");
bool isValid = std::regex_match(email, emailPattern); // true if valid
Log File Analysis
Extracting meaningful data from log files is another practical use of regex, particularly for monitoring systems. Consider a regex snippet to capture log entries that include the error level:
std::string log = "[ERROR] File not found at path /home/user.";
std::regex logPattern(R"(\[(\w+)\] (.+))");
std::smatch results;
if (std::regex_search(log, results, logPattern)) {
std::cout << "Error Level: " << results[1] << ", Message: " << results[2] << "\n";
}
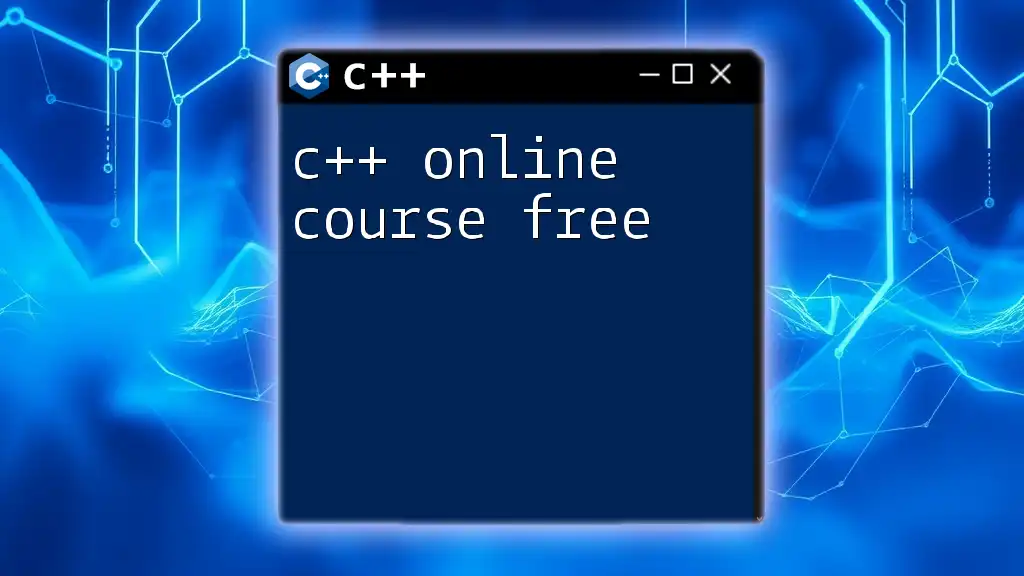
Testing and Debugging Regex Patterns
Regex Testing Tools
When developing regex patterns, using online tools like regex101 or RegExr can significantly boost productivity. These platforms provide real-time results and detailed explanations for regex patterns, ensuring thorough testing.
Common Pitfalls
While working with regex, here are common mistakes to watch out for:
- Misunderstanding Greediness: By default, quantifiers are greedy. Use `?` to make them non-greedy if needed.
- Wrong Escaping: Be aware of which characters need escaping (like `\` and `(`).
- Patterns Too Broad or Too Specific: Fine-tune regex patterns to fit your use case without being either too loose or overly restrictive.
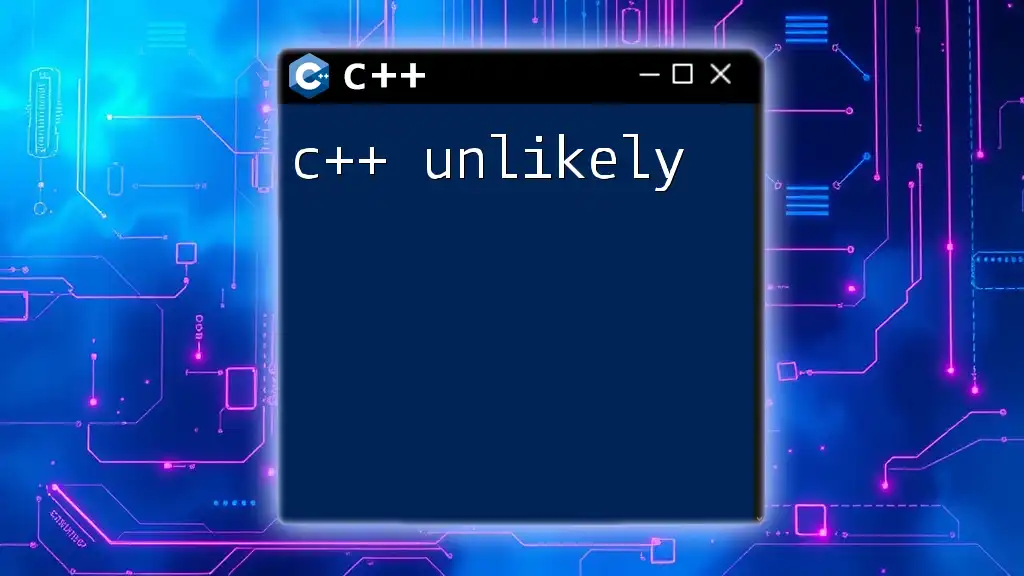
Conclusion
Key Takeaways
Mastering regex in C++ opens numerous opportunities for efficient text processing and data validation. By understanding regex syntax and best practices, you can enhance your coding toolkit significantly.
Resources for Continued Learning
To further develop your regex skills, consider checking out recommended books, online courses, and comprehensive tutorials that delve deeper into advanced regex techniques and best practices. This continued exploration will ensure you remain proficient in using regex in your C++ projects.