C++ offers powerful capabilities that can be executed in a single line, allowing for concise and efficient coding, such as in this example of a simple addition operation:
#include <iostream>
int main() { std::cout << "Sum: " << (5 + 3) << std::endl; return 0; }
Understanding C++ One-Line If Statements
What is a One-Line If Statement?
A one-line if statement in C++ is a minimalist way of expressing a conditional evaluation. These statements are used to simplify code, making it more concise and readable when the conditions being evaluated are straightforward. Unlike traditional multi-line if statements, one-liners can often capture the essence of the logic without excessive verbosity.
Basic Syntax of C++ One-Liner If
The basic syntax comprises the condition followed by a 'ternary operator'. The structure is simple:
condition ? expression_true : expression_false;
This structure allows developers to assign or execute expressions based on a condition, all succinctly captured in a single line.
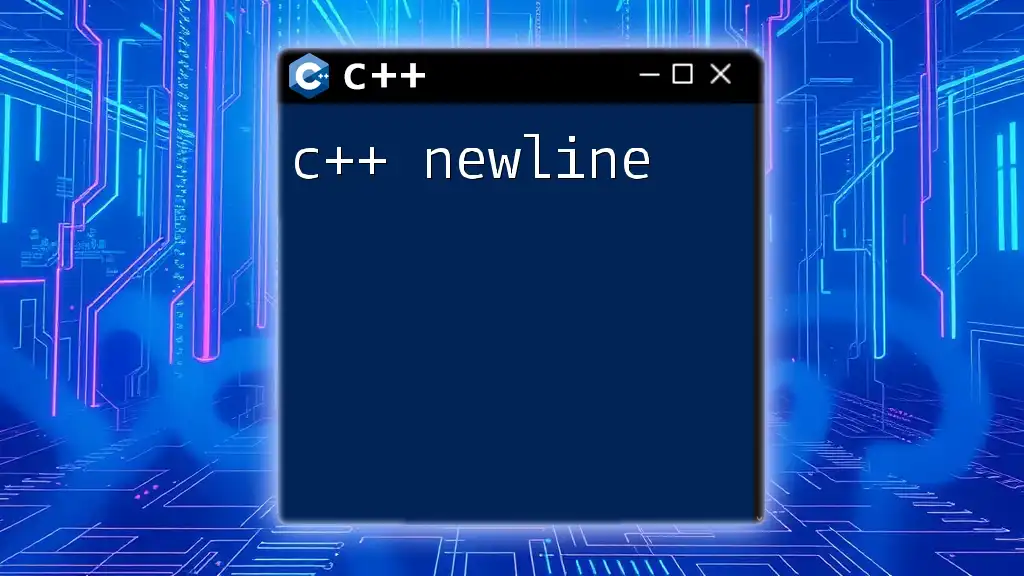
Using If Else in One Line
The One-Line If Else Statement
The one-line if else statement is an extension of the one-line if, where you evaluate a condition and return one of two expressions based on whether the condition is true or false. This is similarly formatted:
condition ? expression_true : expression_false;
This method can replace lengthy if-else blocks when the operations are simple enough.
Practical Examples of One-Line If Else
-
Simple Conditional Assignment
Consider a scenario where you want to check if someone is eligible to vote based on their age:int age = 20; std::string canVote = (age >= 18) ? "Yes" : "No";
Here, `canVote` is assigned either "Yes" or "No" based on the condition checked.
-
Function Return Based on Condition
In this example, we can illustrate a basic function that returns the maximum of two numbers:int max(int a, int b) { return (a > b) ? a : b; }
The function checks if `a` is greater than `b`, returning `a` if true and `b` otherwise.
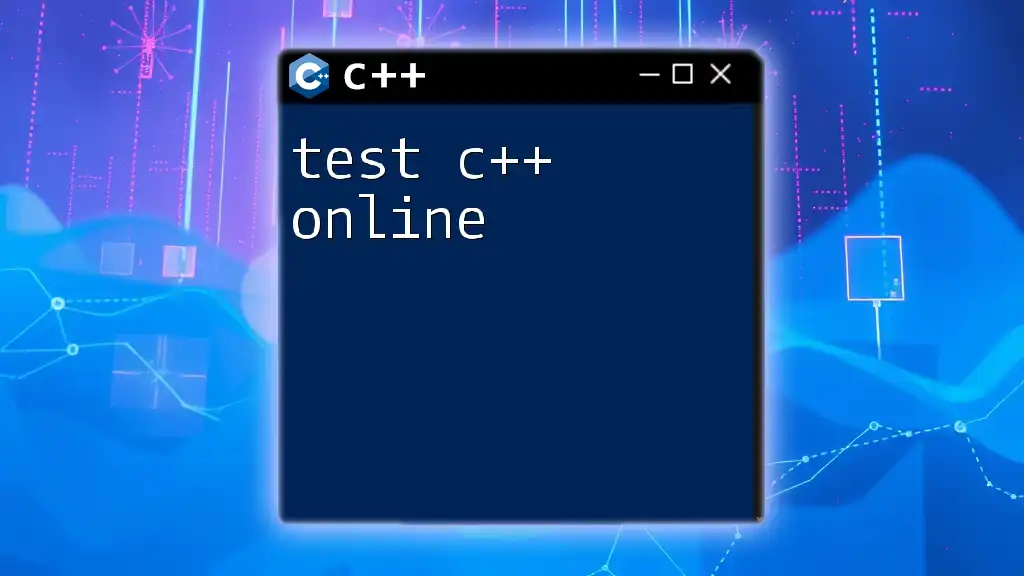
Implementing One-Line If Statements in Different Contexts
Using One-Line If Statements in Loops
One-liners can also enhance clarity in loops for simple operations. For instance, you can print whether numbers are even or odd in a single line:
for (int i = 0; i < 10; i++)
(i % 2 == 0) ? std::cout << i << " is even\n" : std::cout << i << " is odd\n";
This implementation efficiently uses the one-liner to categorize and print results without cluttering the loop.
One-Line If Statements with Functions
One-liners can be particularly useful in functions to evaluate and return values quickly. For example:
bool isPositive(int number) {
return (number > 0) ? true : false;
}
In this case, the function checks if a number is greater than zero, returning `true` if it is and `false` otherwise.
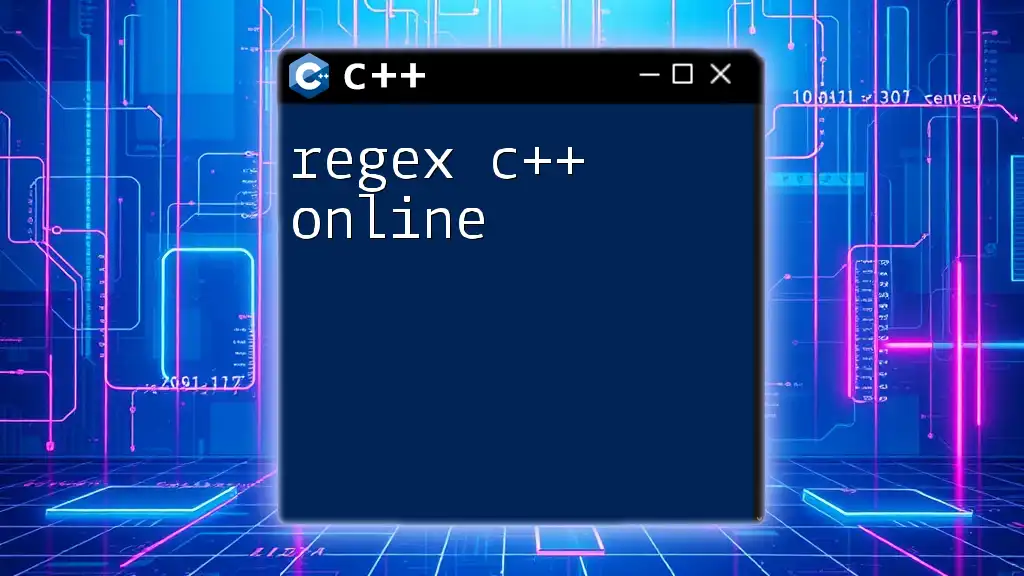
Best Practices for Using One-Line If Statements
When to Use One-Line Statements
When deciding whether to use one-liners, maintain a balance between conciseness and clarity. They work best in scenarios where the logic is straightforward and doesn't require extensive processing. If readability is compromised, it may be better to use traditional multi-line if statements.
Common Pitfalls to Avoid
One of the most significant mistakes developers make is misusing one-liners leading to over-complexity. Here’s an example of poor readability:
(x > 10) ? (y < 5) ? a = 1 : a = 0 : (y == 5) ? a = 2 : a = 3;
This convoluted structure can confuse readers and make debugging difficult. Avoid nesting multiple conditional checks unnecessarily.
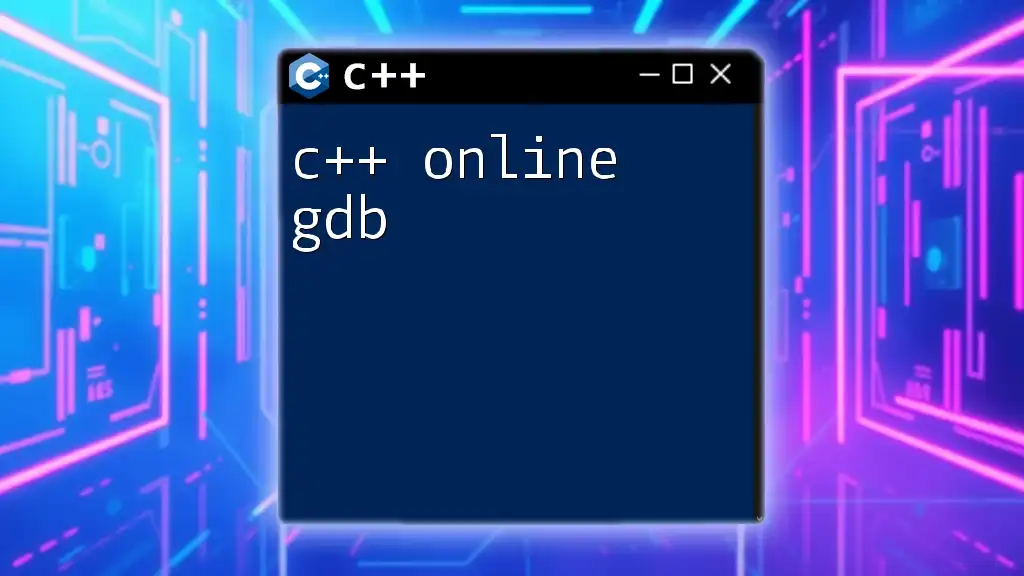
Enhancing Readability in One-Line Statements
Use of Parentheses
Adding parentheses can significantly aid clarity, especially in more complex one-liners. For instance:
// Without parentheses
(x > 0) ? a = 1 : a = 0;
// With parentheses
(x > 0) ? (a = 1) : (a = 0);
In the second example, the use of parentheses clearly delineates the assignment operations tied to the condition, enhancing readability.
Commenting on Complex One-Liners
When one-liners become intricate, adding comments can greatly aid understanding. Here's an example:
(x > 10) ? (y < 5) ? a = 1 : a = 0 : (y == 5) ? a = 2 : a = 3; // Determine a's value based on x and y
By adding a succinct comment, future readers (or your future self) can quickly grasp the core logic behind a complicated expression.
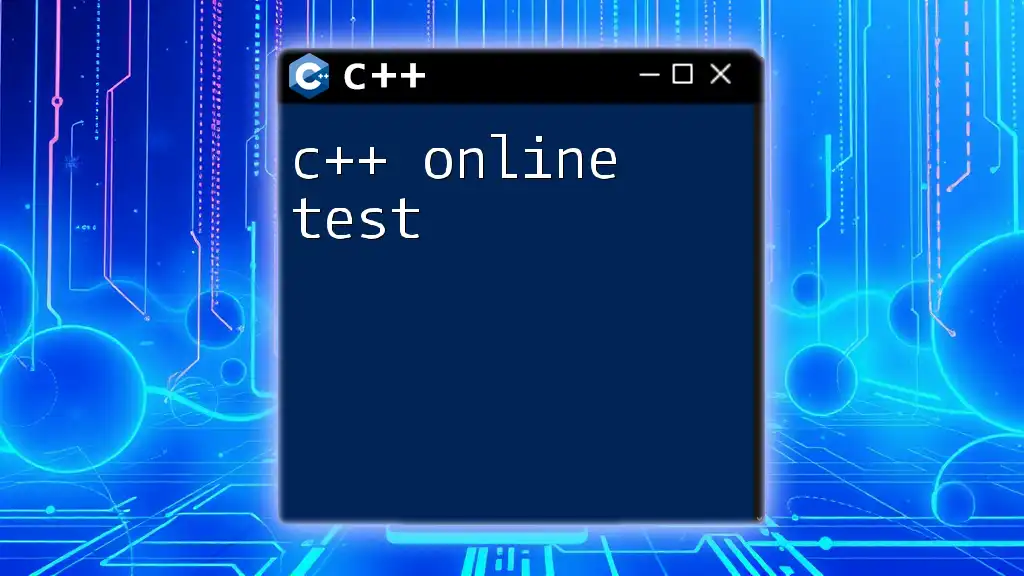
Conclusion
In summary, using one-line if statements in C++ provides a powerful tool for concise conditional logic. They allow for cleaner code when used correctly, enhancing both the readability and maintainability of your codebase. However, it is crucial to practice caution — remember that clarity often trumps brevity. Test your understanding by practicing writing your own one-liners, and feel free to reach out with questions or for further discussion on this topic!