C++ is considered a mid-level language because it combines high-level features like object-oriented programming with low-level capabilities for direct memory manipulation.
Here’s a simple code snippet demonstrating pointer usage in C++:
#include <iostream>
int main() {
int value = 42;
int* ptr = &value; // Pointer to an integer
std::cout << "Value: " << *ptr << std::endl; // Dereferencing the pointer
return 0;
}
Understanding Language Levels
What Are Low-Level Languages?
Low-level languages are programming languages that provide little or no abstraction from a computer's instruction set architecture. This means they allow for direct manipulation of hardware and memory. These languages are often hardware-specific and consist of simple instructions that correspond closely to machine language.
- Examples of low-level languages: Assembly language and Machine Language.
- Characteristics of low-level languages:
- Closer to hardware, giving programmers detailed control over system resources.
- More complex and harder to learn compared to higher-level languages.
- Typically used for system programming and performance-sensitive applications.
What Are High-Level Languages?
In contrast, high-level languages are designed to be easy for humans to read and write. They abstract away much of the complexity of the hardware, allowing developers to focus on programming logic rather than low-level details.
- Examples of high-level languages: Python, Java, and Ruby.
- Characteristics of high-level languages:
- Greater abstraction, using syntax more similar to natural languages.
- Easier to learn and more productive, allowing developers to write complex applications more quickly.
- Often come with built-in libraries for extensive functionality.

Is C++ a Low-Level Language?
C++ and Its Relationship to Machine Language
To understand is C++ low level, it's essential to recognize how C++ interacts with machine language. C++ code must be transformed into machine code that the computer's processor can understand. C++ inherits features from both low-level and high-level languages.
Consider the following example where we utilize pointers in C++:
int main() {
int x = 5;
int* p = &x; // Pointer to the address of x
return 0;
}
In this example, `p` is a pointer that gives us direct access to the memory address of `x`. This demonstrates C++'s ability to handle low-level operations, such as memory management and direct hardware manipulation, which align with low-level languages.
C++ as an Abstraction Layer
Despite its low-level capabilities, C++ incorporates numerous features that provide higher abstraction. This includes object-oriented programming principles such as classes and inheritance.
For instance, using classes enhances code organization and encapsulation:
class Calculator {
public:
int add(int a, int b) {
return a + b;
}
};
Here, the `Calculator` class encapsulates the addition operation, making it easier to use and understand. This showcases how C++ allows programmers to work at a higher level of abstraction, despite its ability to interact with hardware directly.
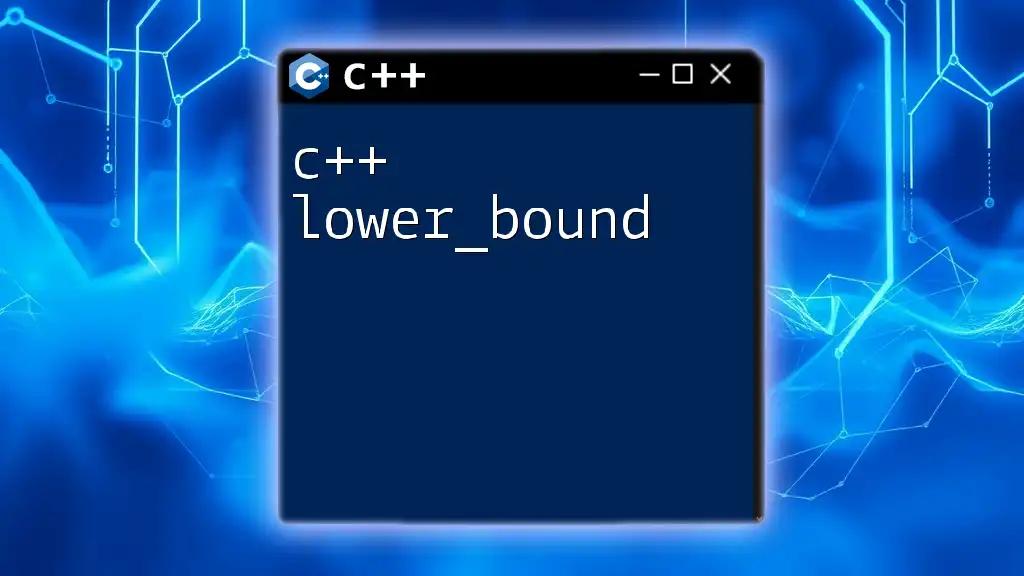
Is C++ a High-Level Language?
Features of C++ That Align with High-Level Concepts
C++ has a rich standard library that supports a wide range of functionalities, making it suitable for high-level programming tasks. The Standard Template Library (STL) provides templates for data structures and algorithms, making it much easier to write complex applications.
For example, consider this snippet demonstrating the use of the STL:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
std::cout << number << std::endl; // High-level functionality
}
return 0;
}
In this example, we are utilizing a vector—an abstract data type offered by C++—to handle a dynamic array of integers. This feature is reminiscent of high-level languages that abstract away memory management complexities.
Comparisons with Other High-Level Languages
C++ retains features that are also common in other high-level languages, such as robust error handling mechanisms. The code below shows how C++ handles exceptions:
try {
int result = 10 / 0;
} catch (const std::exception &e) {
std::cerr << "Error: Division by zero!" << std::endl;
}
This ability to manage errors gracefully is a hallmark of high-level programming. Thus, C++ seamlessly integrates these features, enhancing its appeal as a high-level language while still functioning as a low-level one.
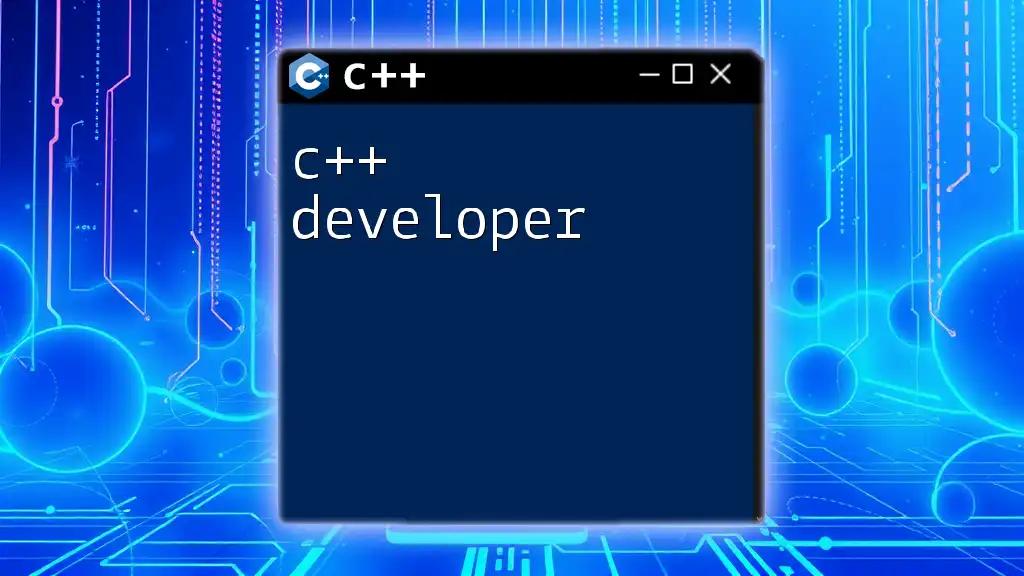
The Middle Ground: C++ as a Multi-Level Language
C++ Characteristics That Make It Unique
C++ stands out in the programming landscape as a hybrid language encompassing both low-level and high-level features. It is designed for system-level programming tasks, such as OS development and performance-intensive applications while being equipped with rich abstraction layers that support application-level programming.
This unique combination allows developers to write efficient code that operates closer to the hardware while also taking advantage of high-level constructs that boost productivity.
Practical Applications of C++
C++ is employed in a wide range of applications that require both performance and high-level programming capabilities. For instance:
- System-level programming: C++ is often used in developing operating systems, embedded systems, and hardware drivers.
- Application-level programming: It’s equally popular for creating desktop applications, game development, and real-time simulations.
This versatility underscores C++ as a multi-level language capable of addressing diverse programming needs effectively.
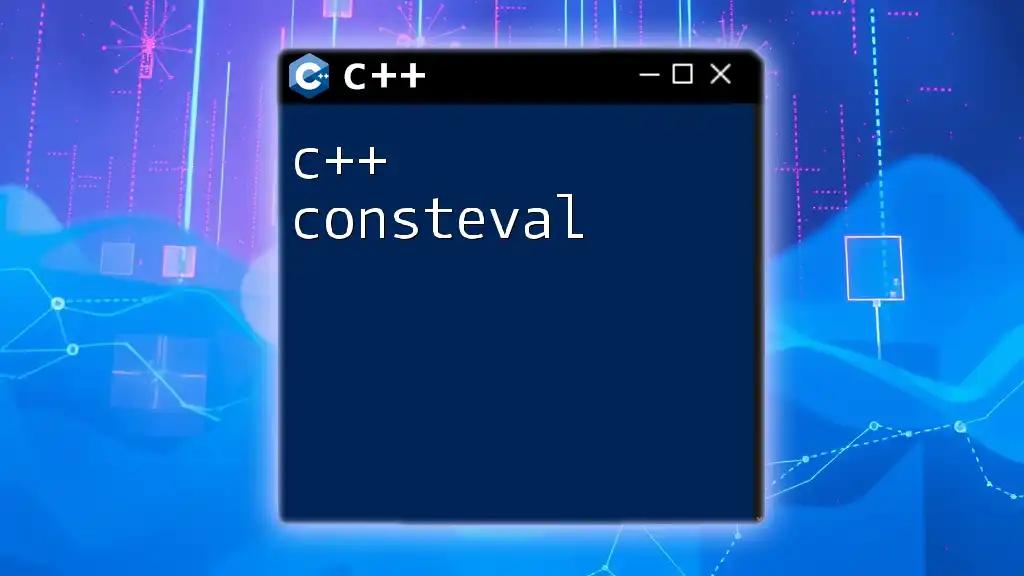
Summary and Conclusion
In conclusion, the question is C++ low level? reveals that C++ does not fit neatly into a single category. Instead, it encompasses both low-level and high-level characteristics, making it suitable for various programming tasks.
C++ gives programmers the tools necessary to access hardware directly while providing high-level constructs that enhance abstraction and ease of use. Its unique blend of properties has solidified C++ as one of the most powerful and versatile programming languages in use today.

Additional Resources
For those interested in deepening their understanding of C++, consider exploring recommended books and online courses dedicated to C++ programming. Familiarizing yourself with the official C++ documentation and best practices can also provide additional insights into this versatile language.

Call to Action
Engage with our company to participate in courses focused on C++ commands and explore this dynamic language further. Embrace the flexibility that C++ offers and enhance your programming skills today!