Yes, C++ is still widely used today in various domains such as game development, system software, and high-performance applications due to its efficiency and versatility.
Here’s a simple C++ snippet demonstrating the classic "Hello, World!" program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
The Evolution of C++
Brief History of C++
C++ was developed in the early 1980s by Bjarne Stroustrup at Bell Labs as an enhancement to the C programming language. It introduced object-oriented concepts, which marked a significant shift in software development practices at that time. Over the years, C++ has undergone several major iterations, each adding features that have kept the language relevant in an ever-evolving tech landscape. Notable versions include C++98, C++11, C++14, C++17, C++20, and the upcoming C++23, each contributing enhancements such as smart pointers, lambda expressions, and modules.
Key Features of C++
C++ remains popular due to its unique set of features:
- Object-Oriented Programming: C++ allows developers to model real-world entities through classes and objects, enhancing code reusability and scalability.
- Performance and Execution Speed: C++ is renowned for its performance. Unlike many higher-level languages, C++ provides fine-grained control over system resources and memory management, which is crucial for performance-critical applications.
- Compatibility with C: C++ maintains backward compatibility with C, allowing developers to leverage existing C libraries and code.
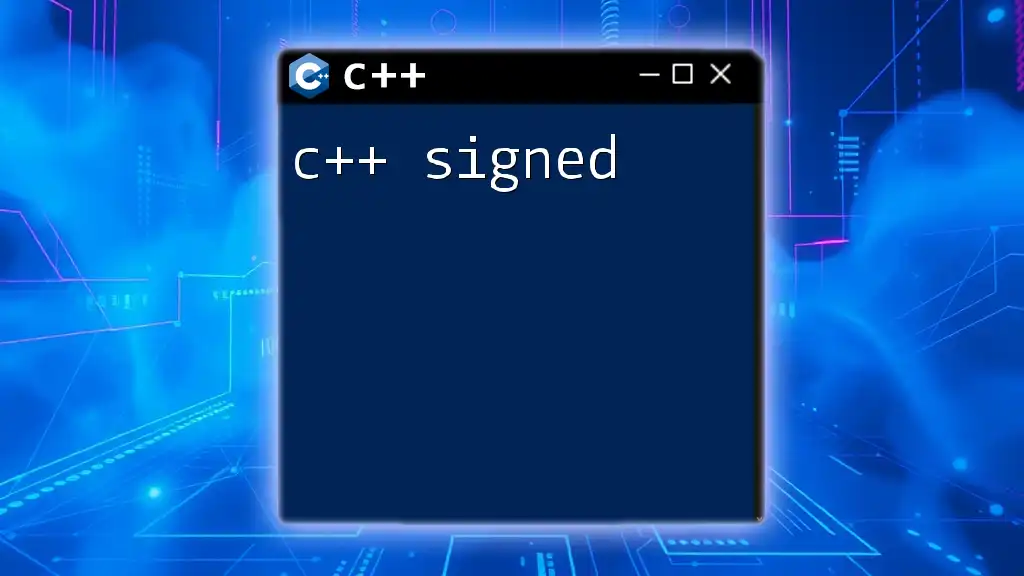
Current Usage and Trends
Industries Utilizing C++
C++ finds applications across numerous industries, showcasing its versatility and resilience.
- Finance and Banking: High-performance trading platforms often rely on C++ due to its speed. Systems designed for high-frequency trading need to process data with minimal latency, where C++ excels.
- Game Development: Leading game engines, such as Unreal Engine, are built with C++. This reliance is largely due to C++'s ability to manage resources effectively and deliver high frame rates, crucial for an immersive gaming experience.
- Automotive and Embedded Systems: C++ is instrumental in developing real-time systems for automotive applications, where reliability and performance are non-negotiable.
C++ in Modern Software Development
In the ever-connected world of software development, C++ remains relevant for cross-platform development, often interfacing with languages like Python or Java for various projects. Furthermore, its strengths in system programming and high-performance computing make it indispensable for tasks that require direct hardware manipulation or intensive computational capabilities.
Trends in Programming Languages
According to various metrics, including the TIOBE Index and the RedMonk rankings, C++ continues to hold a pivotal position amidst popular languages like Python, Java, and C#. While newer languages shine in ease of use or specific applications, C++ remains a choice for performance-oriented tasks that require meticulous control.
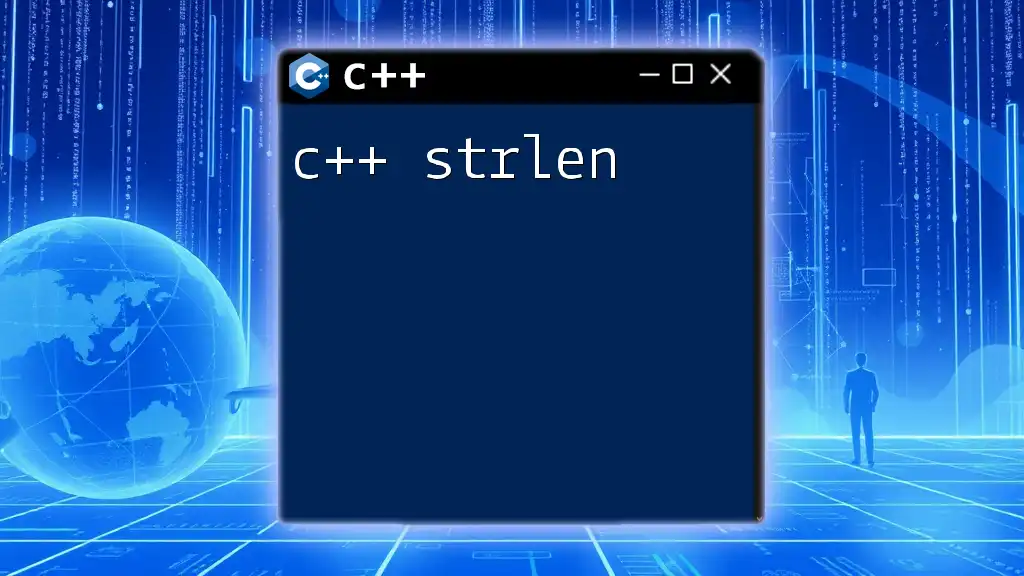
Case Studies: C++ in Action
C++ in Game Development
A notable implementation of C++ is within game development. The Unreal Engine showcases this by leveraging C++ to create complex, high-performance games. A snippet demonstrating a simple character class illustrates how easily developers can manage game mechanics:
// A simple example of a character class in C++
class Character {
public:
void jump() {
// Jump logic here
std::cout << "Character jumps" << std::endl;
}
};
The focus on performance and memory management ensures seamless gameplay and responsive interactions, key components for modern gaming experiences.
C++ in Financial Applications
C++ is also paramount in financial services, where real-time performance is essential. A simplified trading algorithm written in C++ can exhibit its speed and precision:
// Simplified trading algorithm in C++
void executeTrade(float price) {
if (price < 100) {
std::cout << "Buying at " << price << std::endl;
// Buy logic
} else {
std::cout << "Selling at " << price << std::endl;
// Sell logic
}
}
This small snippet reflects how decisions need to be made promptly, validating C++'s application in high-stakes environments.
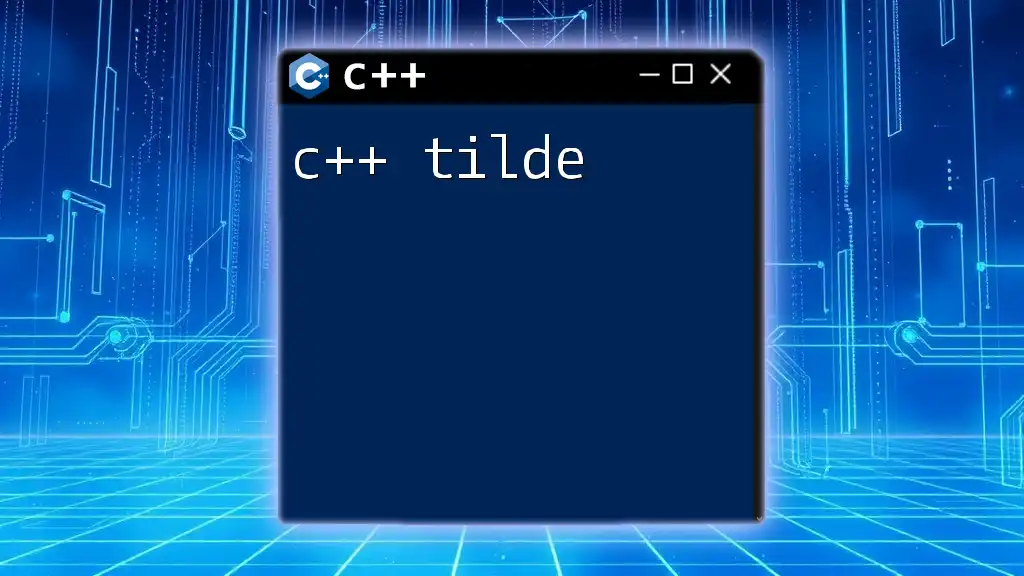
Advantages of Using C++
Performance and Efficiency
C++ is widely praised for its performance. Unlike languages that handle memory management automatically, C++ allows developers to manage memory manually, optimizing for speed and efficiency, which is crucial in resource-constrained environments like embedded systems.
Versatility
The versatility of C++ spans various domains, including artificial intelligence, virtual reality, and systems programming. Its ability to deliver performance without compromising functionality makes it suitable for diverse applications, from game engines to scientific computing.
Community and Learning Resources
The robust community surrounding C++ contributes to its longevity. Numerous libraries, frameworks, and educational resources are available for both beginners and seasoned developers, reinforcing the idea that C++ is here to stay.
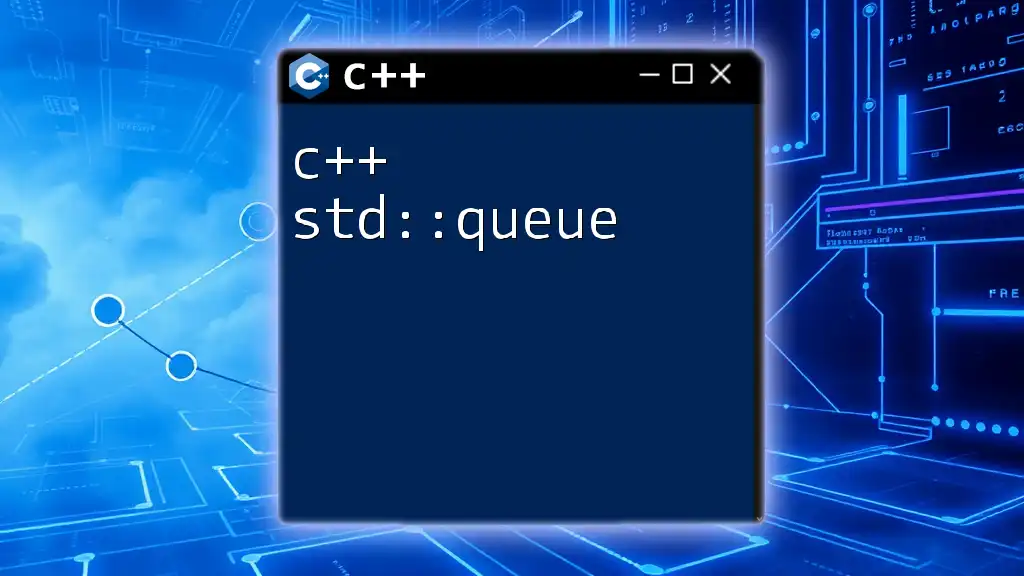
Criticisms and Challenges
Complexity of C++
Despite its advantages, C++ has a steep learning curve. New developers often find the language's nuanced syntax and complex concepts challenging. Common pitfalls include memory leakage and undefined behavior, which can result from manual memory management and pointer arithmetic.
Alternatives to C++
While C++ is a powerhouse, languages such as Python and Rust have emerged as appealing alternatives, especially for rapid development and safety concerns. These languages simplify many aspects of programming, making them more accessible for newcomers. In specific scenarios—such as quick scripting tasks or applications prioritizing safety—these alternatives may outperform C++.
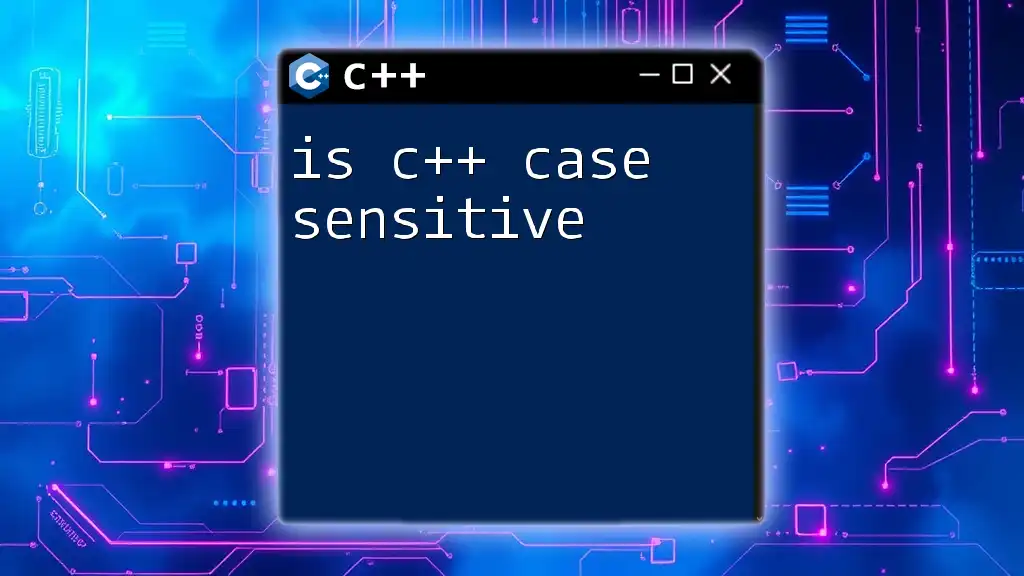
The Future of C++
New Standards and Features
C++ continues to evolve, with the latest standards introducing features that address modern challenges in programming. C++20 introduced concepts, ranges, and coroutines, enhancing the ability to write cleaner and more efficient code. The upcoming C++23 promises additional improvements that keep it aligned with contemporary programming practices.
C++ in Emerging Technologies
C++ is well-poised to play a key role in future technologies, including artificial intelligence, Internet of Things (IoT), and blockchain. A simple example demonstrating compatibility with IoT devices illustrates this potential:
// Example of C++ interacting with IoT devices
#include <iostream>
class IoTDevice {
public:
void sendData() {
std::cout << "Sending data to the server" << std::endl;
// Send data logic here
}
};
With its performance attributes and growing relevance in new tech fields, C++ is expected to stay at the forefront of software development.
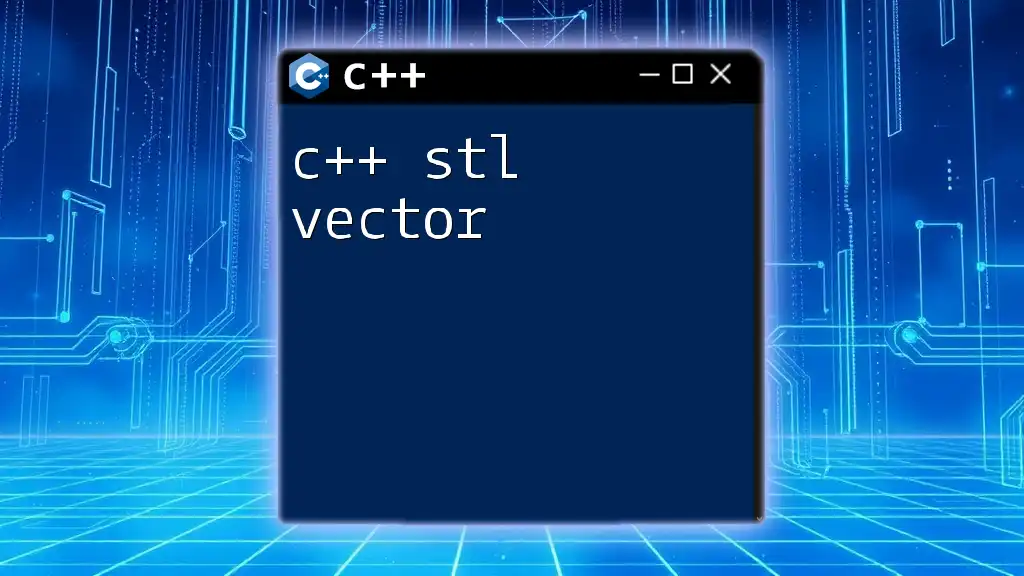
Conclusion
When asking "Is C++ still used?", the answer is a resounding yes. Its rich history, robust community, and versatile applications underscore its ongoing relevance in various industries. Despite challenges and emerging alternatives, C++ remains an essential skill for developers, providing the tools necessary to build performance-critical applications. As you consider diving deeper into C++, consider exploring resources offered by our company that will guide you through mastering C++ and its commands.
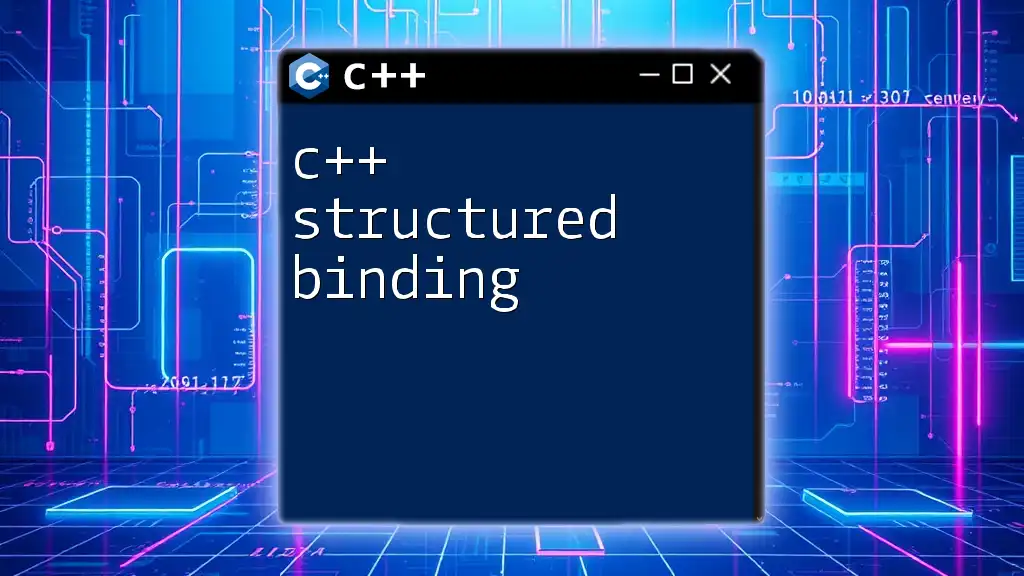
Additional Resources
To further your learning, consider exploring recommended books, online courses, and community forums that specifically cater to C++. These resources will enhance your understanding and application of this powerful programming language.
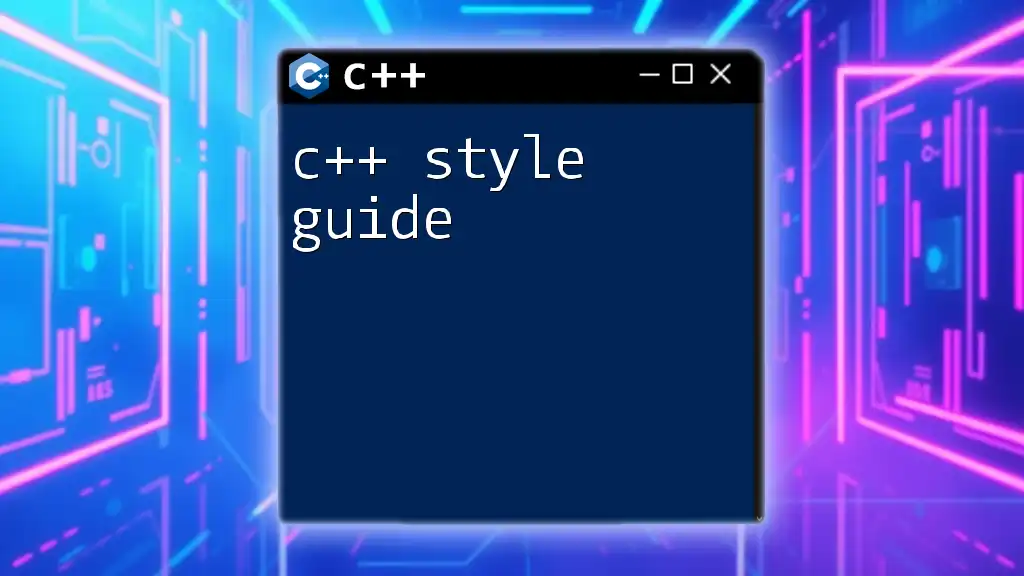
FAQs
In this section, we'll address common queries about C++ usage, its future, and how to start learning effectively.