Yes, C++ is case-sensitive, meaning that it treats identifiers with different casing as distinct entities. Here's a simple code snippet illustrating this concept:
#include <iostream>
int main() {
int variable = 5;
int Variable = 10;
std::cout << "variable: " << variable << std::endl; // Outputs 5
std::cout << "Variable: " << Variable << std::endl; // Outputs 10
return 0;
}
Understanding Case Sensitivity in Programming
What is Case Sensitivity?
Case sensitivity refers to the distinction between uppercase and lowercase letters in programming languages. In a case-sensitive language, identifiers such as variable names, function names, and class names are differentiated based on their letter casing.
For example, in case-sensitive languages like C++, `myVariable` and `MyVariable` are considered two distinct identifiers. This is in contrast to case-insensitive languages like SQL, where `myvariable`, `MyVariable`, and `MYVARIABLE` are interpreted as the same entity.
Importance of Case Sensitivity
Understanding case sensitivity is crucial for several reasons:
-
Code Consistency: When writing code, maintaining a consistent use of case helps improve readability and organization. It becomes easier for other developers (or even yourself later) to understand and navigate the codebase.
-
Common Errors: Many errors arise from case-sensitive confusion. A developer might inadvertently try to access a variable using an incorrect casing, leading to compilation errors or unexpected behavior.
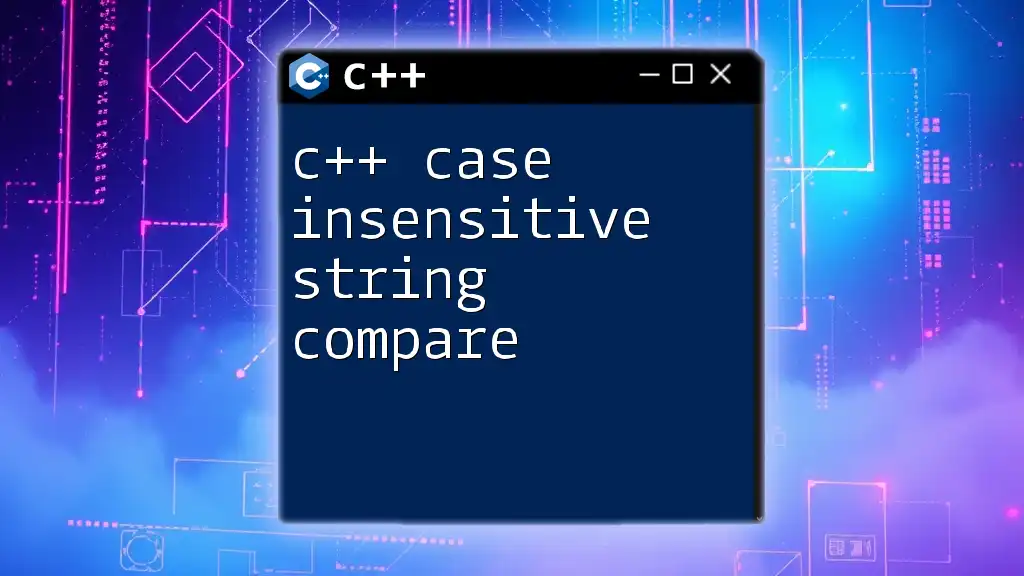
C++ and Case Sensitivity
Is C++ Case Sensitive?
Yes, C++ is a case-sensitive programming language. This means that all identifiers, including variables, functions, and classes, are treated distinctly based on their letter casing. Consequently, this feature has significant implications for how C++ code is written and interpreted.
Examples of Case Sensitivity in C++
To illustrate C++'s case sensitivity, consider the following examples:
-
Variable Names: In C++, you can declare variables with names differing only in their casing:
int myVariable = 5; int MyVariable = 10; int MYVARIABLE = 15;
In this case, `myVariable`, `MyVariable`, and `MYVARIABLE` are treated as three separate variables. It is vital to use the correct casing when referencing them to avoid confusion or errors.
-
Function Names: Similar to variables, function names in C++ are also case-sensitive:
void myFunction() { // Function code } void MyFunction() { // Different function code }
Here, both functions can coexist in the same scope without any conflict. This characteristic can be both advantageous and risky depending on how the code is structured.
-
Class Names: Classes also follow case sensitivity in C++:
class MyClass {}; class myclass {}; // Completely different
This means that `MyClass` and `myclass` are two distinct classes. It's crucial to maintain consistency in naming to prevent errors when instantiating classes.
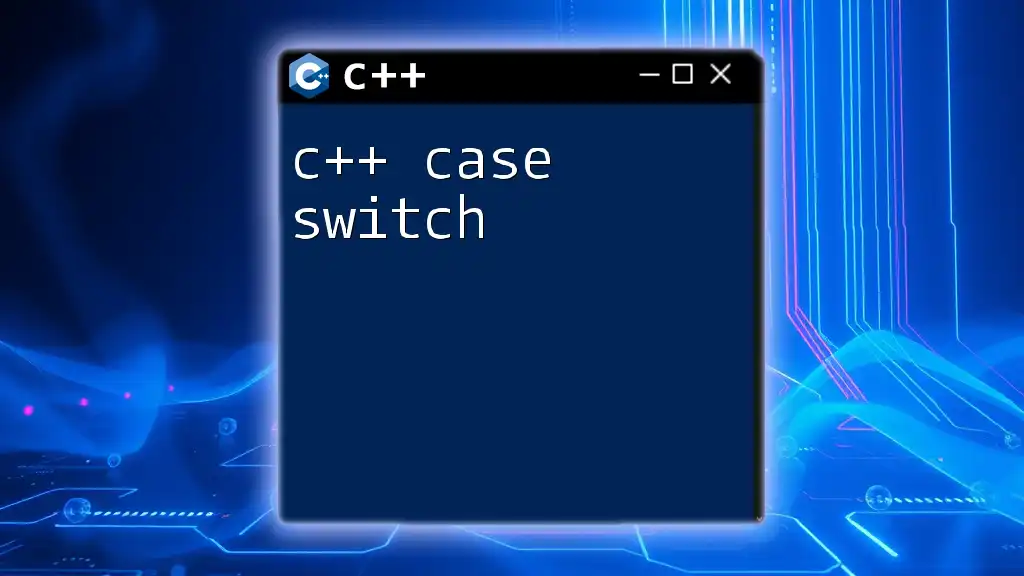
Common Pitfalls Related to Case Sensitivity
Examples of Mistakes
With the unique nature of case sensitivity, errors can easily arise. A practical example would look like this:
int age = 30;
std::cout << Age; // Error: 'Age' was not declared in this scope
In this instance, when the variable `age` is referenced with an incorrect casing (`Age`), the compiler throws an error indicating that the variable has not been defined. This type of mistake can lead to frustration while debugging.
Best Practices to Avoid Errors
To mitigate errors related to case sensitivity, consider adopting the following best practices:
-
Tip for Beginners: Establish a consistent naming convention—whether it's camelCase, snake_case, or another style—and stick to it throughout your codebase.
-
Using IDE Features: Leverage the features of Integrated Development Environments (IDEs) to your advantage. Many IDEs offer code highlighting, auto-completion, and error detection that can help you spot case-related mistakes before running your code.
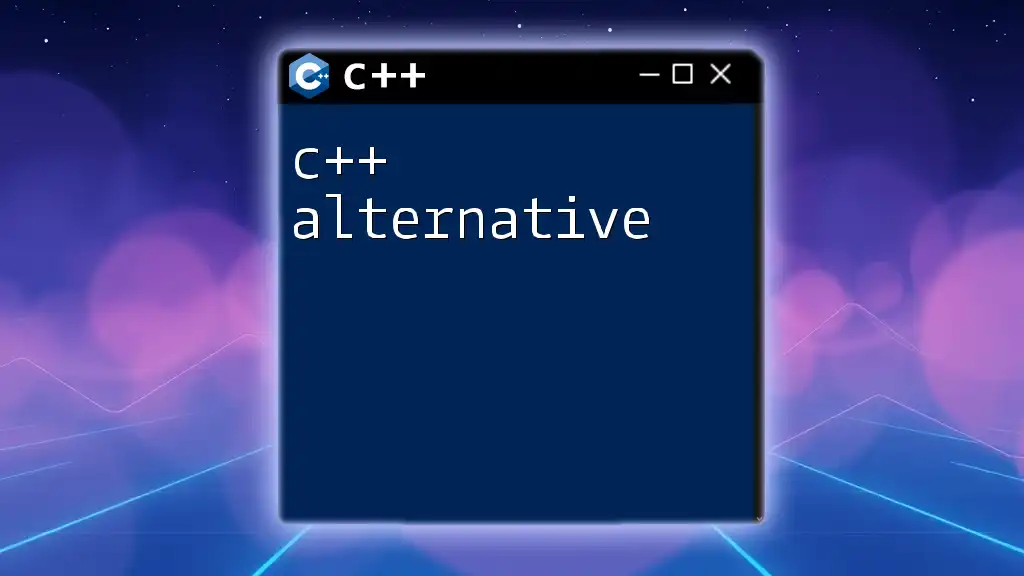
Tools and Resources
Development Environments
When working with C++, employing a reliable Integrated Development Environment (IDE) can greatly enhance productivity and reduce the chances of errors related to case sensitivity. Some recommended IDEs include:
-
Visual Studio: A powerful IDE with extensive features for C++ development, offering code suggestions and error detection.
-
Code::Blocks: An open-source IDE that provides a simple interface for coding in C++, making it beginner-friendly while still supporting advanced features.
Further Reading and Resources
For those looking to deepen their understanding of C++ and case sensitivity, consider exploring the following resources:
-
Books: Look for comprehensive C++ programming books that provide in-depth discussions on naming conventions and best practices.
-
Online Courses: Platforms like Coursera, Udemy, and edX offer courses that cover C++ programming fundamentals, including important concepts related to case sensitivity.
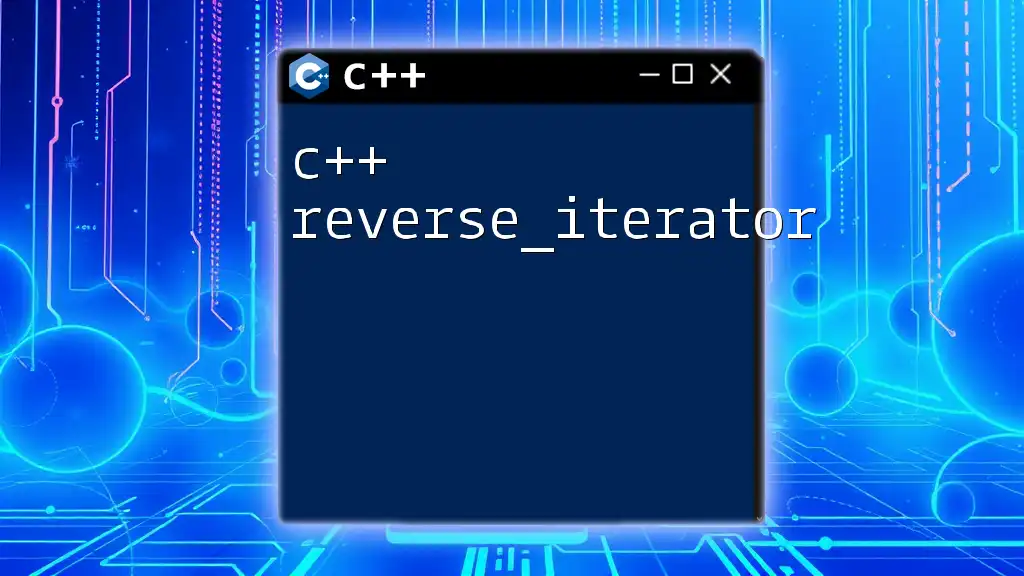
Conclusion
In summary, C++ is indeed case-sensitive, and knowing this is crucial for writing effective and error-free code. The distinct treatment of identifiers based on their casing can significantly impact code readability and maintainability. By practicing careful naming conventions and utilizing available tools, developers can navigate the challenges posed by case sensitivity in C++ more efficiently.
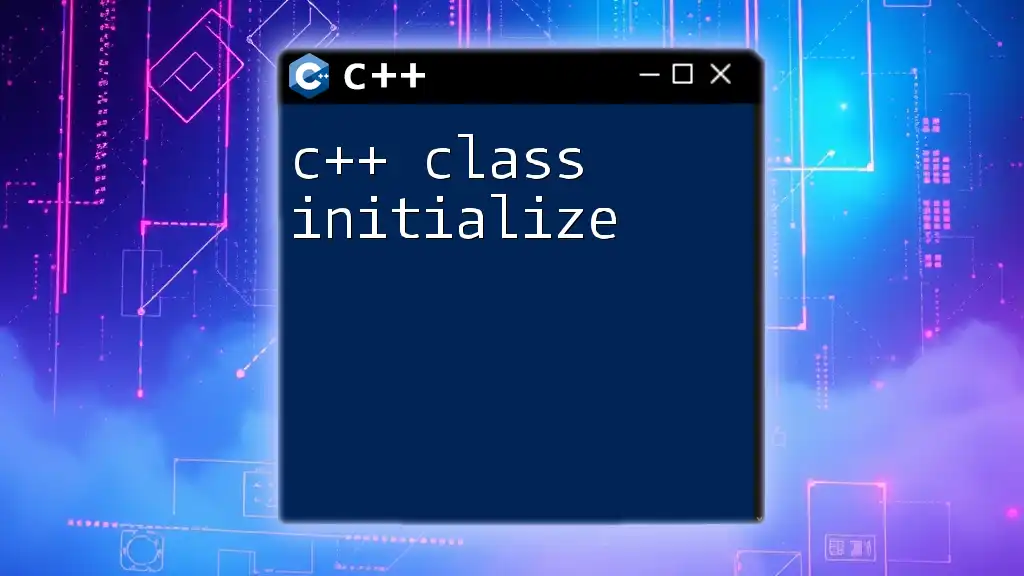
FAQs
Is C++ the only language that is case sensitive?
No, C++ is not the only case-sensitive language. Others like Java, C#, and JavaScript also exhibit case sensitivity. This feature, while common, varies across programming languages, and understanding it is vital to successfully coding in multiple environments.
What are some common naming conventions?
Common naming conventions include:
- camelCase: `myVariableName`
- snake_case: `my_variable_name`
- PascalCase: `MyVariableName`
Selecting a standard naming convention and adhering to it improves code clarity and reduces the likelihood of errors.
How can I prevent case-sensitive errors?
To prevent errors stemming from case sensitivity, always ensure consistent use of names throughout your code. Utilize IDE features to highlight inconsistencies and engage in practices such as code reviews, where team members can spot potential case-related mistakes in each other's code.
By keeping these pointers in mind, developers can navigate the intricacies of C++ effectively, ensuring both functionality and readability in their projects.