C++ is not considered a scripting language; rather, it is a compiled programming language that requires a compiler to execute code, which allows for more complex and performance-driven applications.
Here’s a simple C++ code snippet demonstrating a basic program:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding Scripting Languages
What is a Scripting Language?
A scripting language is typically defined as a programming language designed for integrating and communicating with other programming languages. It is often interpreted rather than compiled and provides a simpler and more concise syntax that facilitates rapid development.
Common characteristics of scripting languages include:
- Interpreted Execution: Scripting languages are usually executed directly from the source code, which means they can be run without the need for a separate compilation step.
- Ease of Use: They tend to have user-friendly syntax that enables quick learning and application, making them ideal for novices and for tasks requiring speed.
- Dynamic Typing: Many scripting languages employ dynamic typing, where type checking is performed at runtime, allowing for greater flexibility in programming.
Examples of well-known scripting languages are Python, JavaScript, and Ruby, each of which excels in specific domains such as web development, automation, and data analysis.
Key Differences between Compiled and Interpreted Languages
Compiled languages transform source code into machine code through a compilation process, resulting in an executable file. In contrast, interpreted languages execute instructions directly from the source code. Here are essential distinctions:
- Compilation Stage: Compiled languages require a separate compilation step, which typically results in faster execution during runtime. Examples include C and C++.
- Runtime Flexibility: Interpreted languages allow scripts to be changed and executed on the fly, enabling easier testing and debugging.
Understanding the execution model is crucial in defining what a language is, and this brings us to explore how C++ fits into this paradigm.
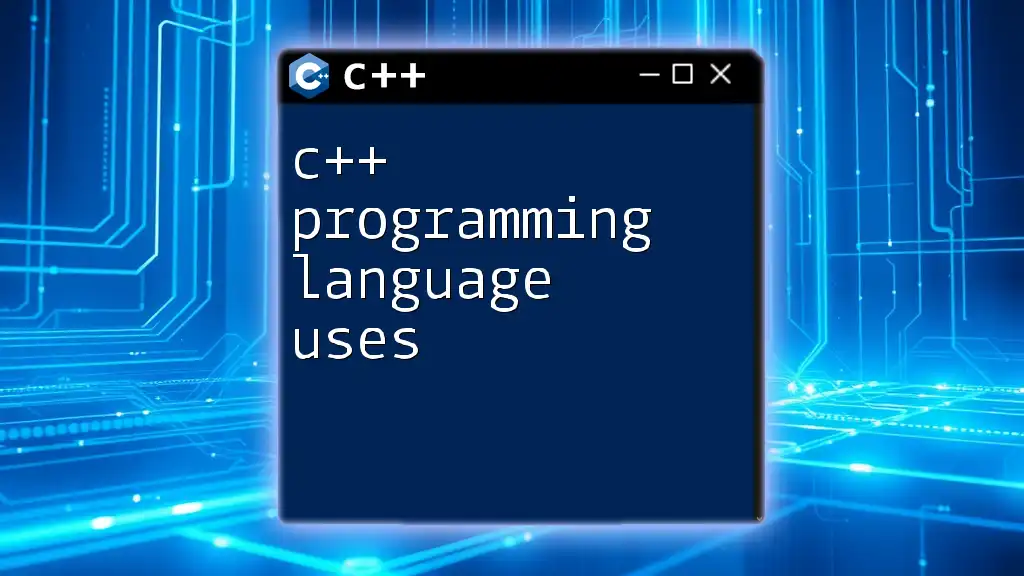
C++ Overview
What is C++?
C++ is an extendable procedural programming language that supports object-oriented, generic, and functional programming paradigms. Developed in the early 1980s by Bjarne Stroustrup, C++ was designed as an extension of the C programming language with the objective of improving code efficiency and facilitating complex software design.
With features like classes, inheritance, and polymorphism, C++ allows developers to create scalable and maintainable codebases. It is primarily utilized for system software, game development, and high-performance applications.
How C++ is Different from Scripting Languages
One of the fundamental differences between C++ and scripting languages lies in its execution methodology. As a compiled language, C++ necessitates a compilation step, converting human-readable source code into machine code before execution. This contrasts with the direct execution model of traditional scripting languages.
Additionally, C++ is statically typed, meaning data types must be declared explicitly, while scripting languages often employ dynamic typing, where variable types can change at runtime. This distinction often makes C++ more performant but can impose a steeper learning curve for newcomers.
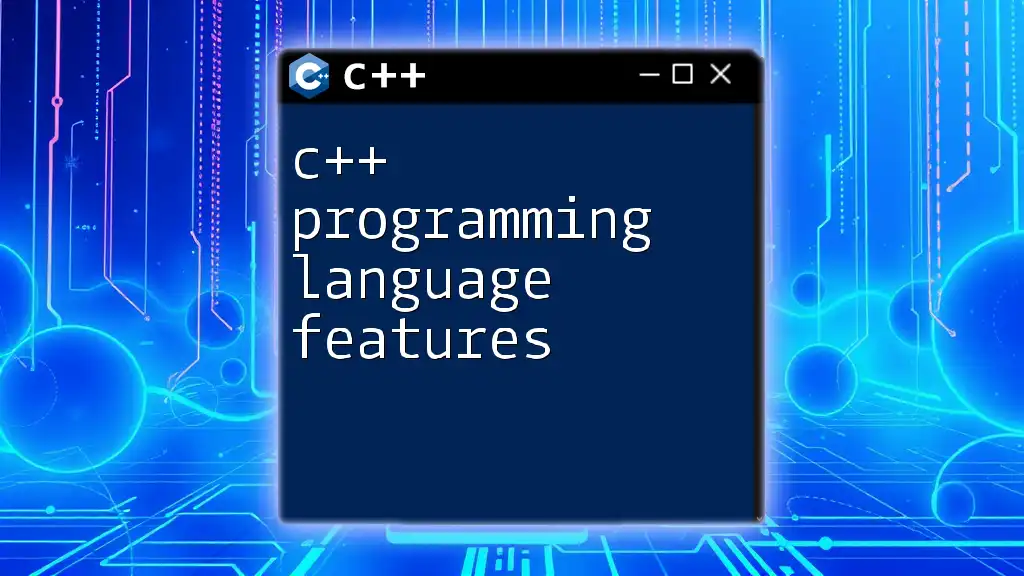
C++ in the Context of Scripting
Can C++ Be Used for Scripting?
While C++ is primarily known as a systems programming language, it has capabilities that can be leveraged for scripting tasks. Some frameworks allow developers to use C++ in a scripting context. For instance, frameworks such as ChaiScript and Squirrel provide mechanisms for incorporating C++ into scripts.
C++ is frequently employed in systems-level scripting and in application scripting when performance is of utmost concern. For example, game engines often integrate C++ to handle critical real-time operations while allowing other components to be scripted in more user-friendly languages.
Examples of C++ as a Scripting Language
Example 1: Using C++ for Game Scripting
In many game development scenarios, C++ can serve as the backbone for performance-critical operations. For instance, consider the following function that manipulates player positions:
// Simple example of scripting in a game environment
void movePlayer(float x, float y) {
player.position.x += x;
player.position.y += y;
}
In this example, C++ is used to manage the speed and efficiency required for real-time gameplay, showcasing its capability to function effectively in a scripting role.
Example 2: Automating Tasks with C++
Beyond gaming, C++ can streamline automation tasks. The following is a simple example of a function designed to rename files, demonstrating how C++ can interact with system-level processes:
#include <iostream>
#include <string>
// Example of a script to automate file handling
void renameFile(const std::string& oldName, const std::string& newName) {
// Implementation to rename files
}
This code illustrates how C++ can simplify tedious tasks, acting akin to a scripting language when quick, performance-oriented solutions are necessary.
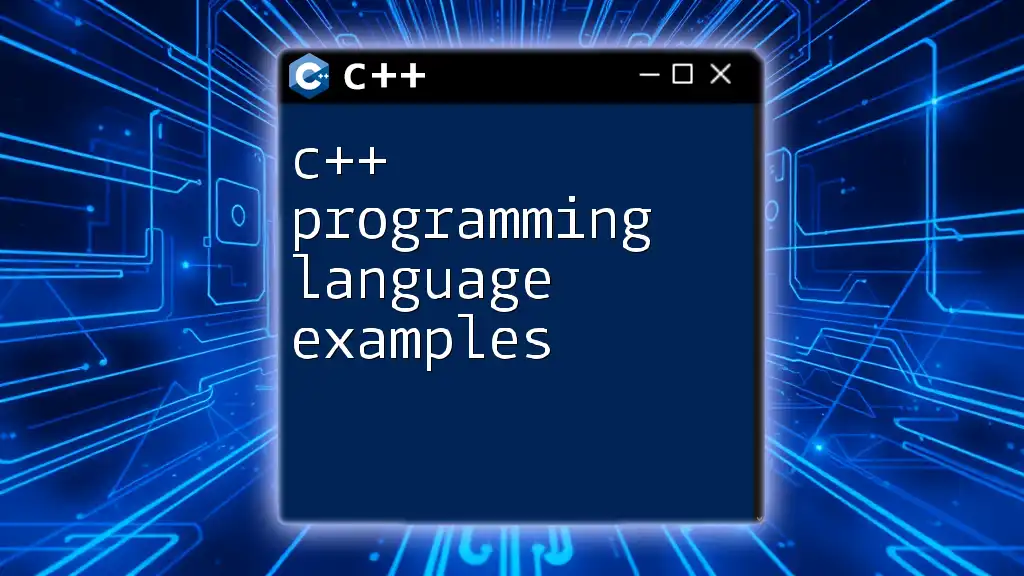
Advantages of Using C++ for Scripting
Performance Benefits
One primary advantage of employing C++ for scripting lies in its performance capabilities. C++ is renowned for its execution speed, particularly in computationally intensive tasks. In scenarios where raw processing power is crucial, such as simulations or gaming, C++ outshines traditional scripting languages, making it an invaluable tool.
Flexibility and Control
By providing low-level control over system resources, C++ allows developers to manipulate memory and hardware directly. This flexibility is advantageous in scenarios where fine-tuned performance is critical, such as in embedded systems or real-time applications.
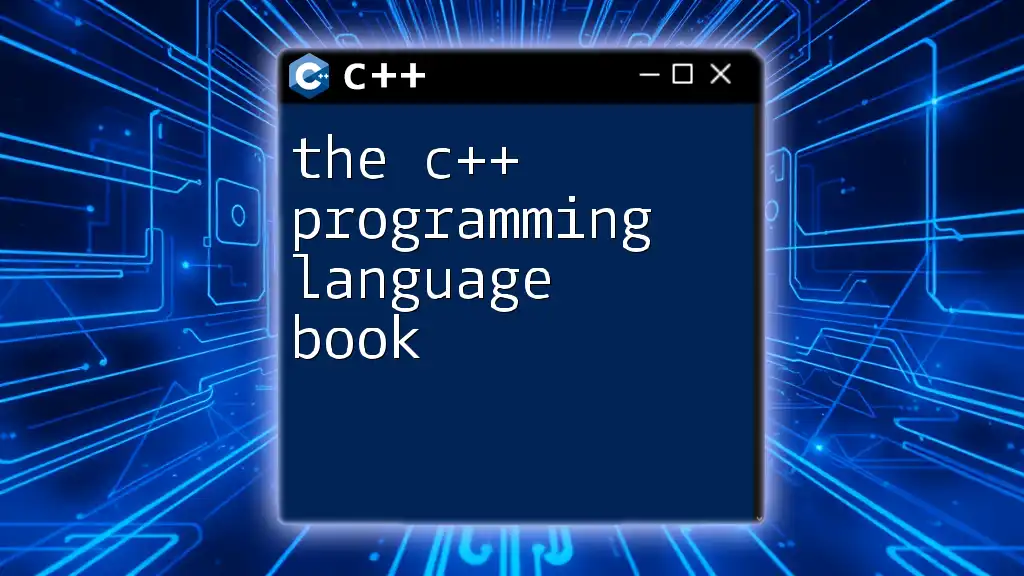
Limitations of C++ as a Scripting Language
Learning Curve
Despite its advantages, C++ poses a steeper learning curve compared to most scripting languages due to its complex syntax and concepts such as pointers and memory management. For new programmers, this complexity can be intimidating, making languages like Python or JavaScript more appealing for quick scripting tasks.
Environment Dependency
C++ requires a compilation step, which can slow down development, particularly in rapid prototyping or iterative testing environments. This dependency on a compile cycle limits C++'s effectiveness in situations where immediate feedback is essential.
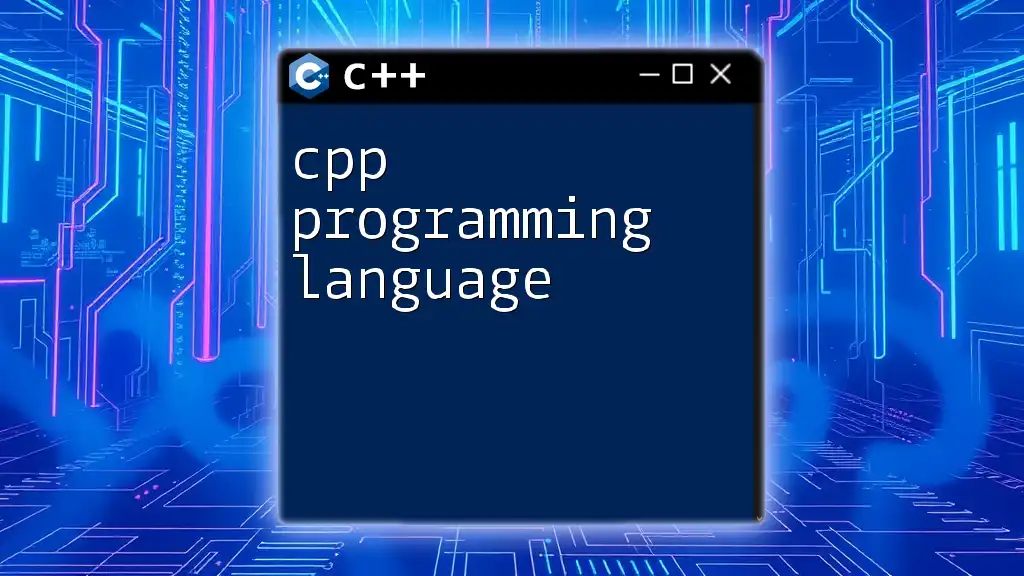
C++ vs. Popular Scripting Languages
Comparison with Python
Python’s syntax is often considered more straightforward and intuitive. For beginners, its readability and dynamic nature make it an excellent choice for scripting. Whereas C++ excels in performance and systems-level programming, Python offers rapid prototyping and ease of use, making them suitable for different tasks.
Comparison with JavaScript
JavaScript is prevalent in web development, allowing developers to build dynamic web pages efficiently. While C++ can be employed to implement performance-sensitive elements within a web environment (through WebAssembly, for example), JavaScript excels in event-driven programming contexts where interactivity is paramount.
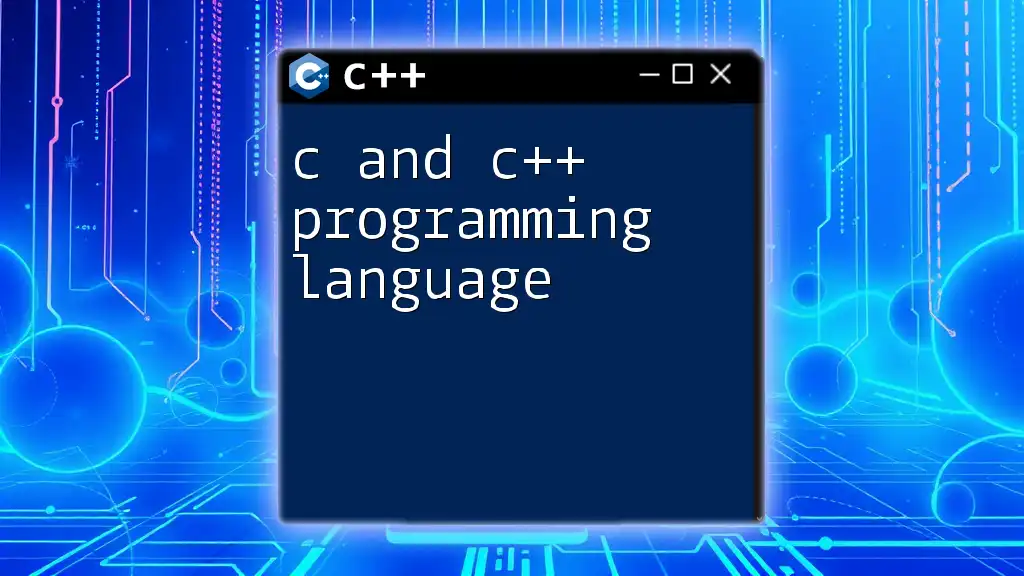
Conclusion
In summary, the question of whether C++ is a scripting language invites nuanced exploration. While C++ is primarily a systems programming language, it certainly has capabilities that align with scripting practices, particularly in contexts where performance is critical. However, its complexities and compilation requirements differentiate it from traditional scripting languages.
Understanding C++ within this spectrum allows developers to appreciate its value while recognizing situations where more straightforward scripting languages might be preferable.
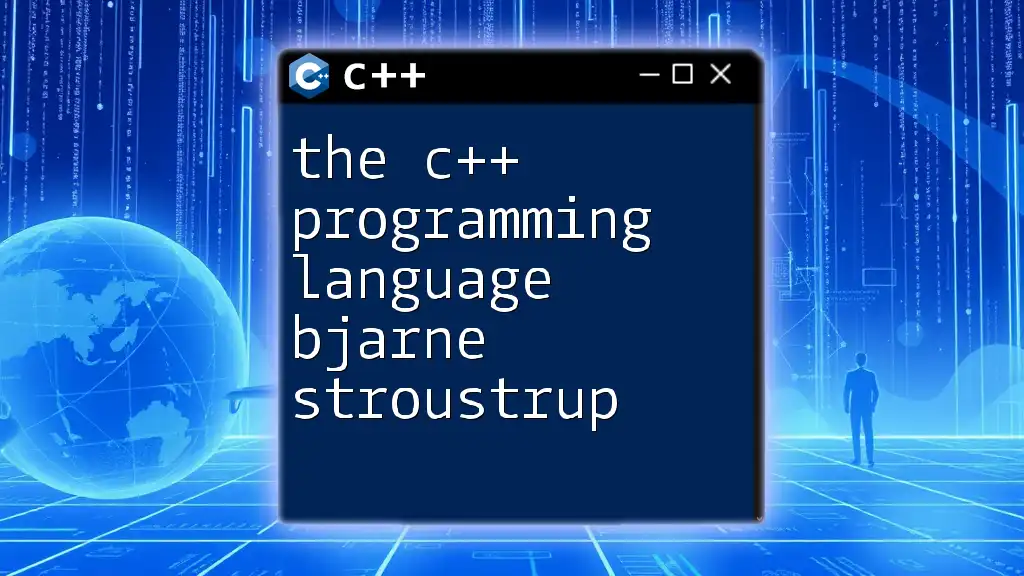
Call to Action
We invite you to share your experiences with C++ and scripting languages. Have you used C++ in a scripting context? Join us for more resources and discussions as you explore the dynamic world of programming languages!