C++ is a powerful, high-performance programming language widely used for system/software development, game programming, and applications requiring real-time processing.
Here's a simple example demonstrating the use of a loop in C++:
#include <iostream>
int main() {
for (int i = 0; i < 5; ++i) {
std::cout << "Hello, World!" << std::endl;
}
return 0;
}
What is C++?
C++ is a general-purpose programming language that was created by Bjarne Stroustrup starting in 1979 at Bell Labs as an extension of the C programming language. This powerful language features low-level memory manipulation capabilities and is designed to facilitate efficient software development.
C++ has evolved significantly, bringing forward many enhancements that support diverse programming paradigms, including procedural, object-oriented, and generic programming. As one of the most widely-used programming languages today, its influence stretches across sectors such as systems software, game development, real-time simulations, and high-performance applications.
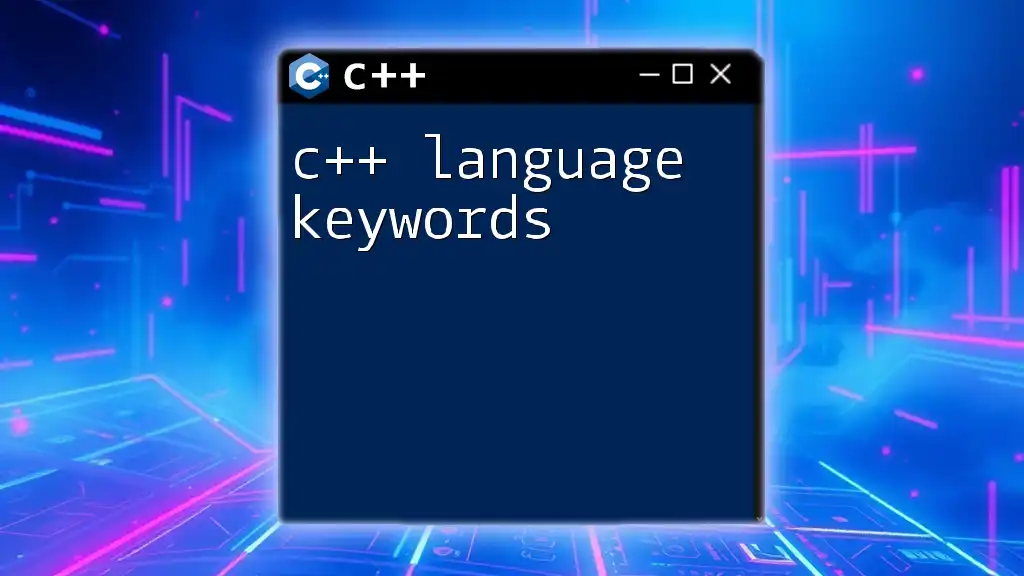
Importance of Learning C++
Understanding the C++ language is essential for aspiring software developers. The language’s versatility allows programmers to develop everything from simple command-line applications to complex systems software. Moreover, C++ plays a significant role in various industries—be it financial software, game engines, or machine learning frameworks.
By learning C++, you will gain a deep appreciation for how software interacts with hardware, allowing you to write highly optimized code that can greatly improve performance.
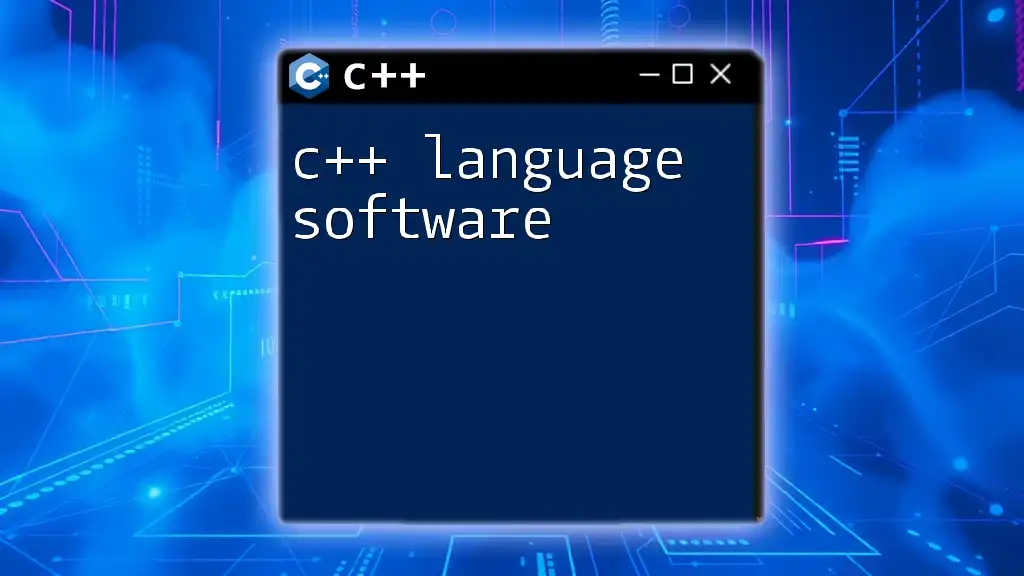
Key Features of C++
Object-Oriented Programming
C++ stands out due to its support for object-oriented programming (OOP), which facilitates code organization and reusability. The four main principles of OOP are:
- Encapsulation: Wrapping data and functions together within classes allows for data hiding and abstraction.
- Inheritance: Classes can inherit properties and behaviors from other classes, enabling code reuse and the creation of hierarchical relationships.
- Polymorphism: C++ allows for method overriding, offering flexibility in how objects are utilized within code.
Standard Template Library (STL)
The Standard Template Library (STL) is another critical feature of C++. This robust library includes a set of templates that streamline common programming tasks, such as data storage and algorithm implementation. STL provides:
- Containers: Such as vectors, lists, and sets, which allow efficient data management.
- Algorithms: Predefined algorithms for sorting, searching, and manipulation that save development time and promote code consistency.
Memory Management
C++ offers fine-grained control over system memory through pointers and dynamic memory allocation, making it a robust choice for performance-sensitive applications. Understanding how to manage memory effectively is critical to prevent memory leaks and optimize resource usage.
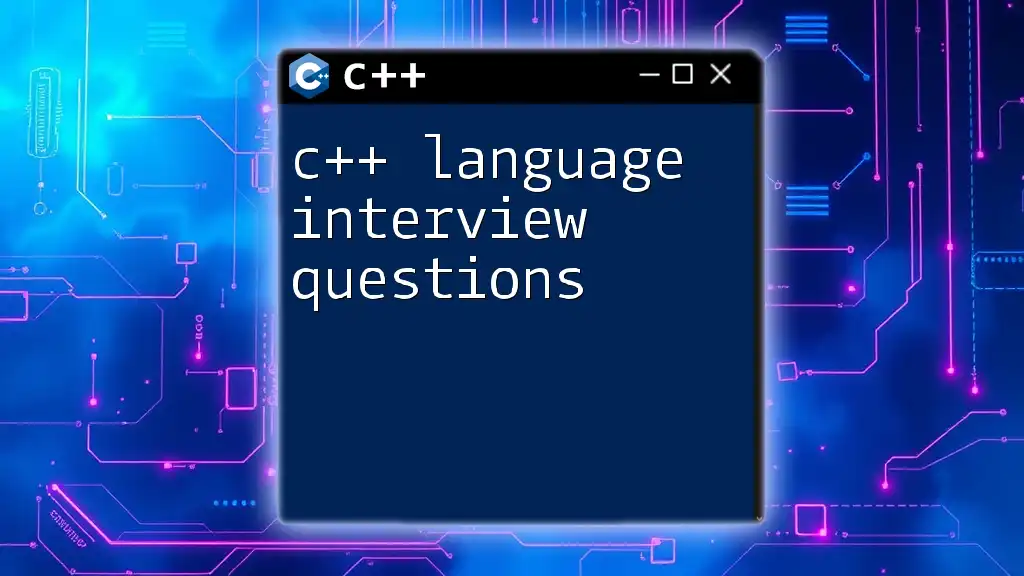
Setting Up the Environment
Installing a C++ Compiler
To begin coding in C++, you'll need to install a compiler. Here are the steps for some popular compilers:
- GCC: Use your system's package manager (like `apt-get` on Debian-based systems) or download from the official website.
- Clang: Available through similar channels as GCC or can be installed via Homebrew on macOS.
- MSVC: Obtain it as part of Visual Studio, directly from Microsoft's website.
Choosing an IDE
An Integrated Development Environment (IDE) can streamline the coding process. Some well-regarded IDEs for C++ include:
- Visual Studio: A powerful IDE for Windows, providing robust debugging and code navigation tools.
- Code::Blocks: A lightweight and versatile option suitable for multiple platforms.
- CLion: A cross-platform IDE from JetBrains, offering smart code assistance and deep integration with CMake.
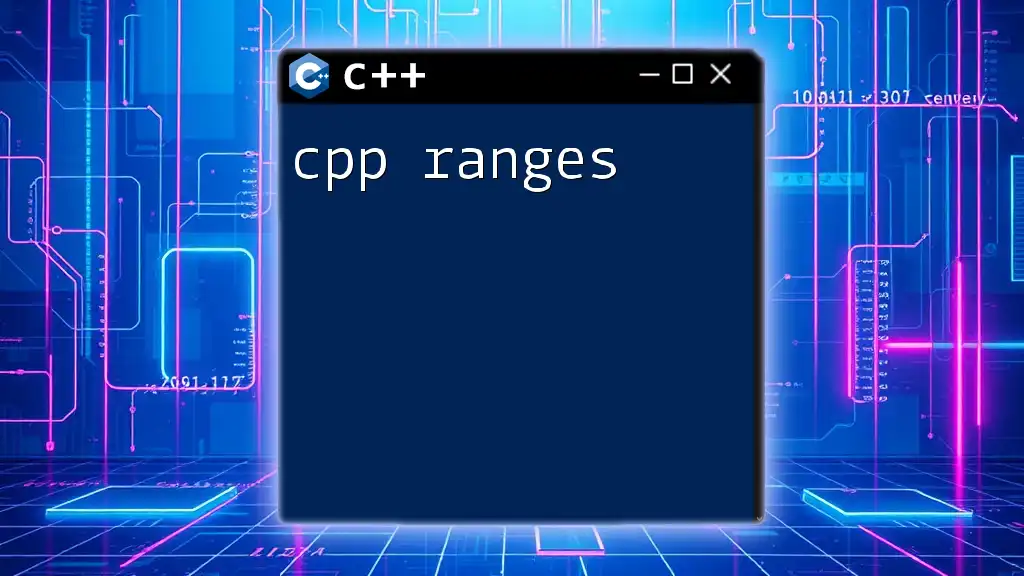
Writing Your First C++ Program
Let's dive into writing your first C++ program, often referred to as the "Hello World" example. Here’s how you can do it:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation of Each Part
- `#include <iostream>`: This includes the input-output stream library needed for print operations.
- `int main() {...}`: This defines the main function, the entry point of the program.
- `std::cout`: Used to output text to the console. `std::endl` produces a newline.
- `return 0;`: Indicates that the program finished successfully.
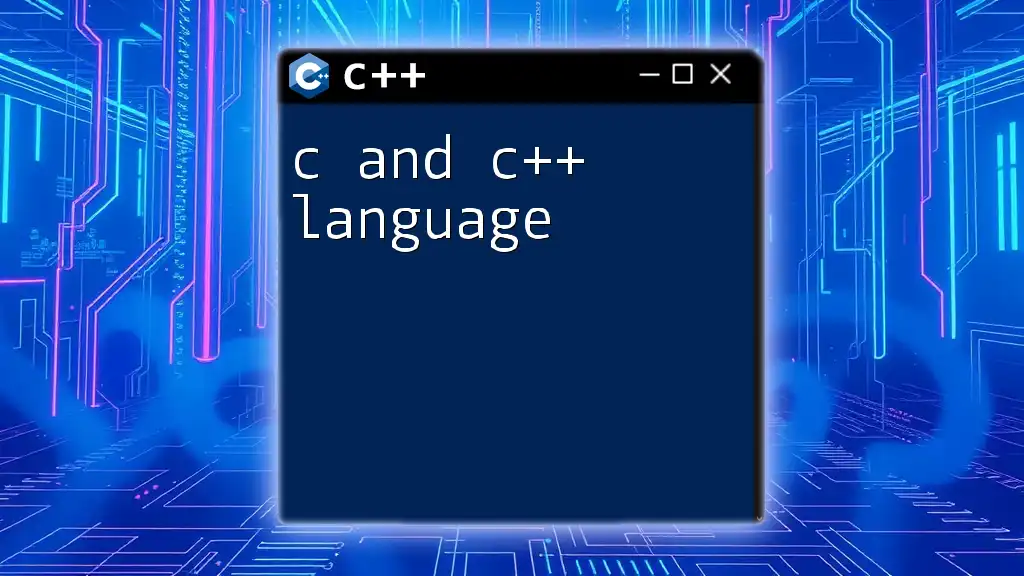
Data Types and Variables
Understanding data types is crucial in the C++ language as it defines what kind of data can be stored and manipulated. C++ offers both primitive and user-defined data types.
Primitive Data Types
C++ supports several primitive types, including:
- int: Represents integers (whole numbers).
- float: Used for floating-point numbers (decimals).
- char: Represents a single character.
- boolean: Holds true/false values.
User-defined Data Types
In addition to primitive types, C++ enables the creation of user-defined types:
- Structs: Used to group related variables.
- Enums: Define a variable that can hold a set of predefined constants.
Variable Declaration and Scope
When you declare variables, they have specific scopes, which define their visibility throughout your code. Understanding this helps avoid name conflicts and improves code organization.
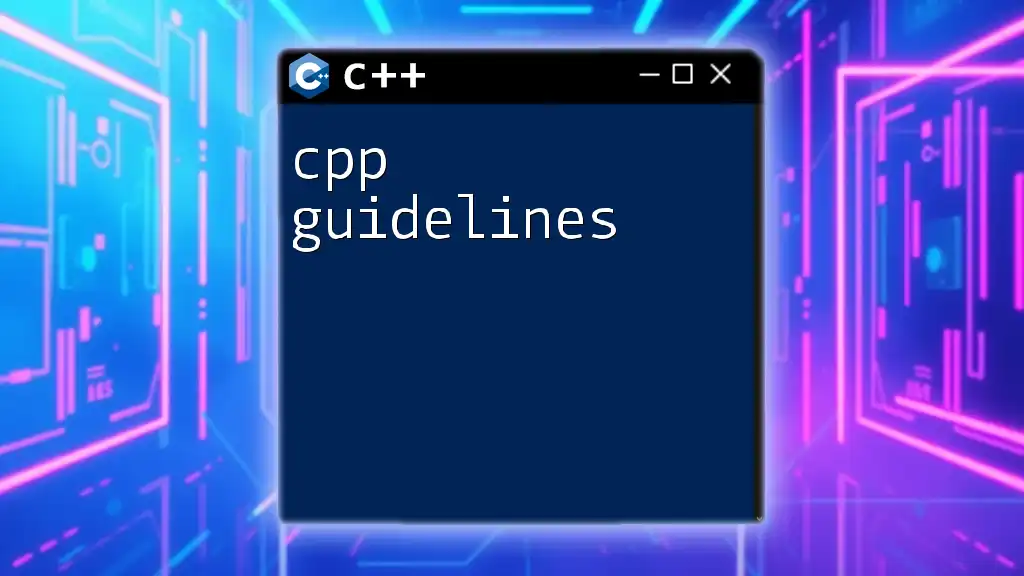
Control Structures
Conditional Statements
C++ provides conditional statements that allow programs to make decisions based on specific conditions.
If-Else Statements Example:
if (condition) {
// code executed if true
} else {
// code executed if false
}
Looping Mechanisms
Loops help you execute a block of code multiple times. The two most common iterative constructs in C++ are `for` and `while` loops.
For Loop Example:
for (int i = 0; i < n; i++) {
// code executed n times
}
While Loop Example:
while (condition) {
// code executed while condition is true
}
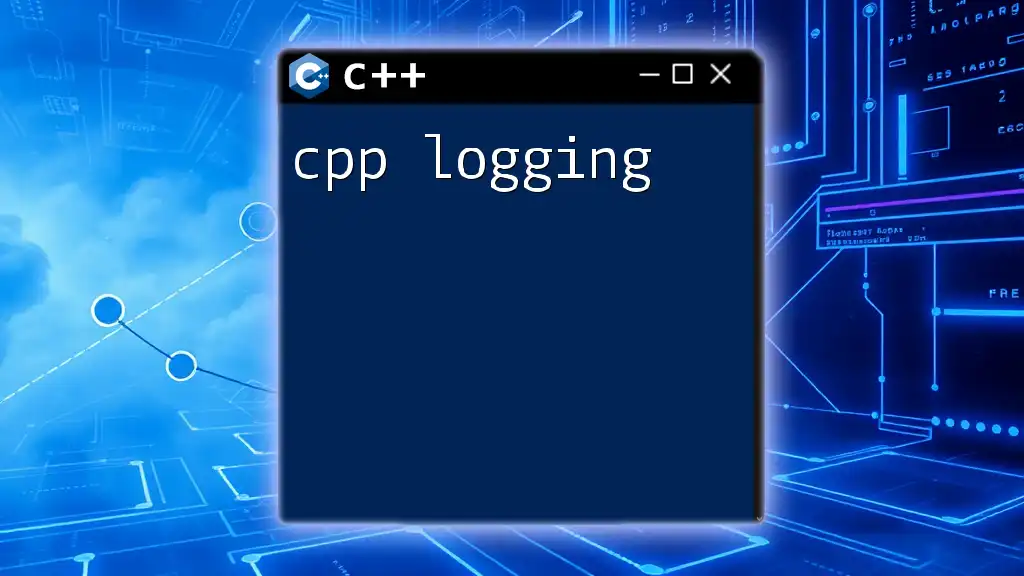
Functions and Modularity
Understanding Functions
Functions allow you to modularize code, promoting code reuse and maintainability. This encapsulation enables you to break your program into smaller, manageable parts.
Function Declaration and Definition
The declaration specifies the function name, return type, and parameters:
int add(int a, int b);
The definition provides the implementation:
int add(int a, int b) {
return a + b;
}
Here, `add` takes two integers and returns their sum.
Return Values and Parameters
C++ functions can return values and accept parameters, which can significantly alter the behavior or output of your code. Proper parameter handling is essential, especially when dealing with multiple inputs.
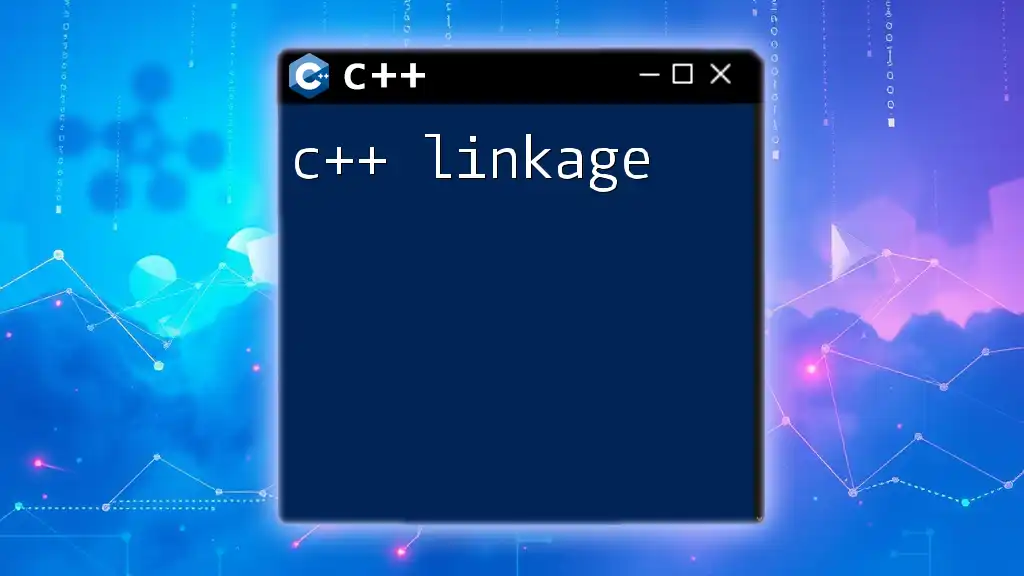
Object-Oriented Programming in C++
Classes and Objects
In C++, a class is a blueprint for creating objects (instances). Below is how you define a simple class:
class Car {
public:
void drive() {
std::cout << "Driving..." << std::endl;
}
};
In this example, `Car` is the class, and `drive` is a member function that outputs a message to the console.
Inheritance and Polymorphism
Inheritance allows a class to inherit properties and behavior from another class, promoting code reuse. Polymorphism allows derived classes to be treated as instances of their base class.
For example:
class Vehicle {
public:
virtual void start() {
std::cout << "Vehicle is starting..." << std::endl;
}
};
class Car : public Vehicle {
public:
void start() override {
std::cout << "Car is starting..." << std::endl;
}
};
Here, `Car` inherits from `Vehicle` and overrides the `start()` method.
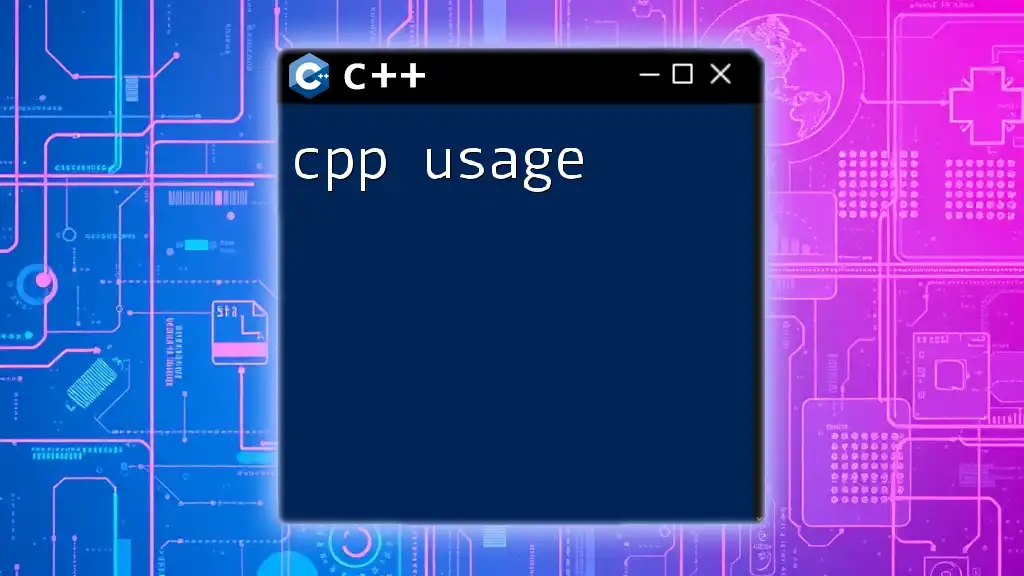
Advanced Features
Templates and Generic Programming
Templates allow you to write generic functions or classes that can operate on different data types. This flexibility is a cornerstone of C++ and enhances code reusability.
Here’s a simple template for a function:
template <typename T>
T add(T a, T b) {
return a + b;
}
This `add` function can perform addition on various data types, from integers to floats.
Exception Handling
Robust applications need to gracefully handle unexpected situations. C++ employs exception handling using `try`, `catch`, and `throw` keywords.
Here’s a basic example:
try {
// Code that may throw an exception
} catch (const std::exception& e) {
std::cerr << "Exception: " << e.what() << std::endl;
}
Importance of Exception Handling
Exception handling promotes robustness by allowing the program to continue running or exit gracefully in response to errors, ensuring that critical operations can recover from unforeseen circumstances.
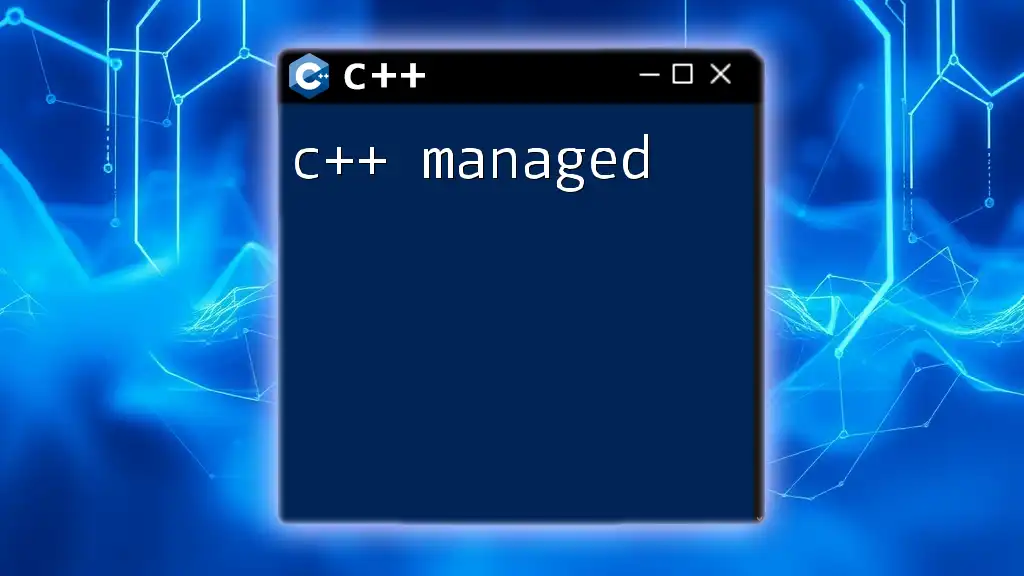
Best Practices for C++ Programming
Code Readability
Maintaining readability is essential in C++ programming. Use descriptive variable names, consistent indentation, and comment your code to convey the purpose of complex sections clearly.
Memory Management Best Practices
Effective memory management is vital to prevent memory leaks and optimize resource use. C++ encourages the use of RAII (Resource Acquisition Is Initialization), where resources are tied to object lifetimes, ensuring they are properly released when no longer needed.
Moreover, using smart pointers—such as `unique_ptr` and `shared_ptr`—can help manage dynamic memory automatically without manual intervention.
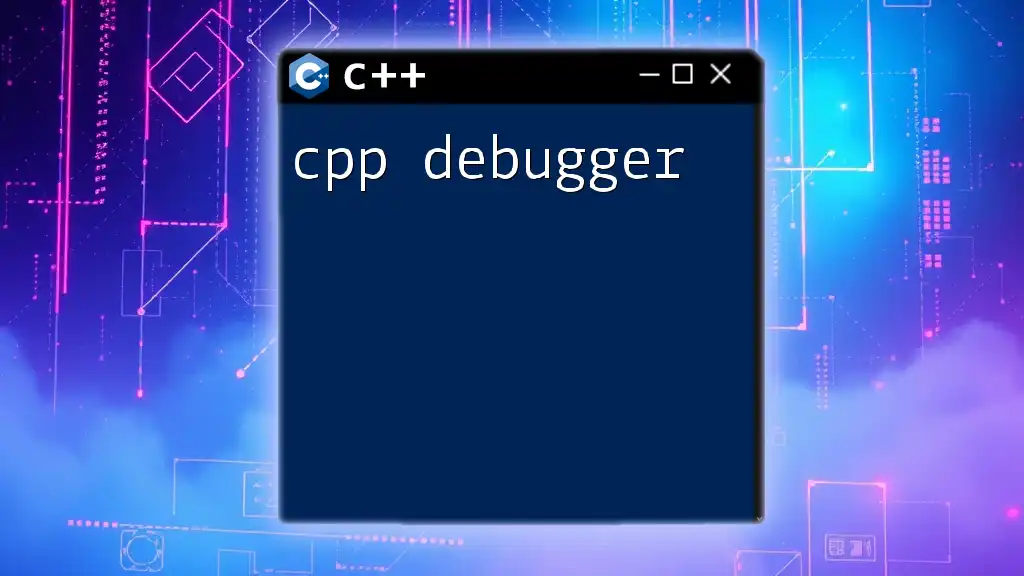
Conclusion
The C++ language remains one of the pillars of modern programming, and its features provide immense flexibility and power to developers. As it continues to evolve, keeping abreast of the latest standards and best practices is essential.
Continuous learning from books, online courses, and community forums will further enhance your skills and keep you updated on trends in C++. Dive into C++ programming today and explore its capabilities!