In this post, we will explore essential C++ interview questions that can help you demonstrate your understanding of the language, including key concepts and code snippets. Here’s a basic example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ Basics
What is C++?
C++ is a powerful programming language that was developed as an enhancement to the C language in the early 1980s by Bjarne Stroustrup. It is known for its flexibility and performance, which has made it highly popular for system/software development, game programming, and even in large-scale enterprise applications.
The language supports various programming paradigms, primarily object-oriented programming (OOP), but also includes features of generic programming and functional programming. Some of the notable characteristics of C++ include its ability to manipulate hardware and fine-tune resource management.
Basic C++ Syntax
At its core, C++ programs are comprised of functions and classes. Here's a simplified structure of a C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this code snippet:
- `#include <iostream>` imports the input-output stream library, enabling the use of `cout`.
- `main()` is the entry point of any C++ program, representing the initial function that gets executed.
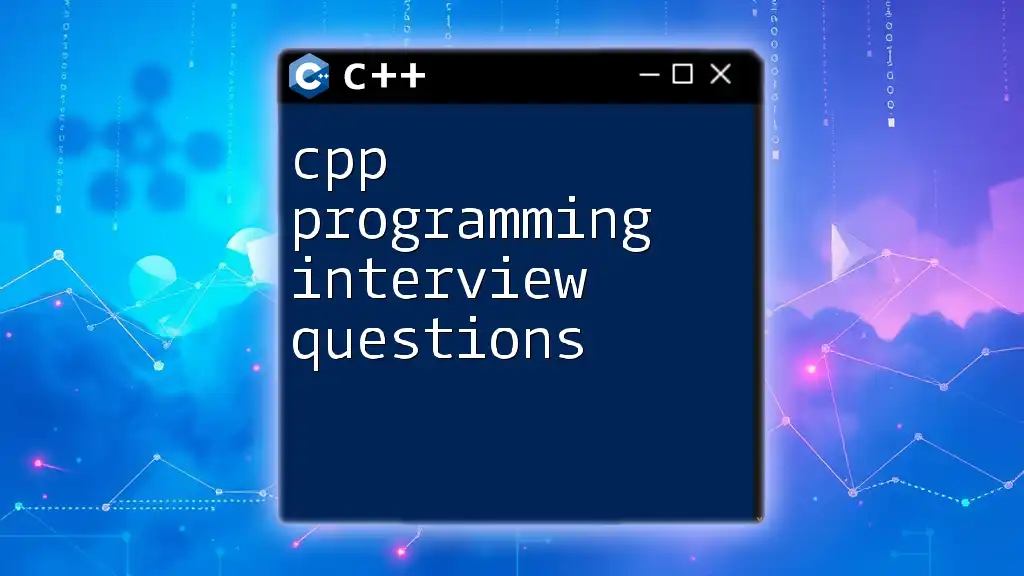
Core C++ Concepts
Object-Oriented Programming (OOP)
C++ is particularly esteemed for its support for OOP, which enhances code organization and reuse. The four fundamental principles of OOP in C++ are:
- Encapsulation: Bundling data and methods that operate on the data, restricting outside access and modifying certain behaviors.
- Inheritance: Deriving new classes from existing ones, allowing code reuse and hierarchical relationships.
- Polymorphism: Allowing functions or methods to operate in different forms. This can be achieved via function overloading or overriding.
- Abstraction: Hiding complex realities while exposing only the necessary parts.
Here’s an example demonstrating these concepts in C++:
class Animal {
public:
virtual void sound() = 0; // Pure virtual function
};
class Dog : public Animal {
public:
void sound() override { cout << "Bark" << endl; }
};
In this example, `Animal` is a base class with a pure virtual function, promoting abstraction, and `Dog` is a derived class demonstrating inheritance and polymorphism.
Memory Management
Pointers and References
Memory management is a critical aspect of C++, primarily handled through the use of pointers and references. Pointers store memory addresses, while references refer directly to a variable.
Here’s a quick demonstration:
int a = 10;
int* ptr = &a; // Pointer to 'a'
cout << *ptr; // Output: 10
In the example above, `ptr` points to the address of `a`, allowing direct access to its value through dereferencing.
Dynamic Memory Allocation
Dynamic memory allocation in C++ facilitates efficient use of memory, enabling the creation of objects dynamically during runtime using the `new` keyword and releasing it with `delete`.
Example:
int* arr = new int[5]; // Dynamic array allocation
// Use the array
delete[] arr; // Clean up
In this snippet, we allocate an array of integers dynamically and ensure proper memory management by using `delete` at the end.
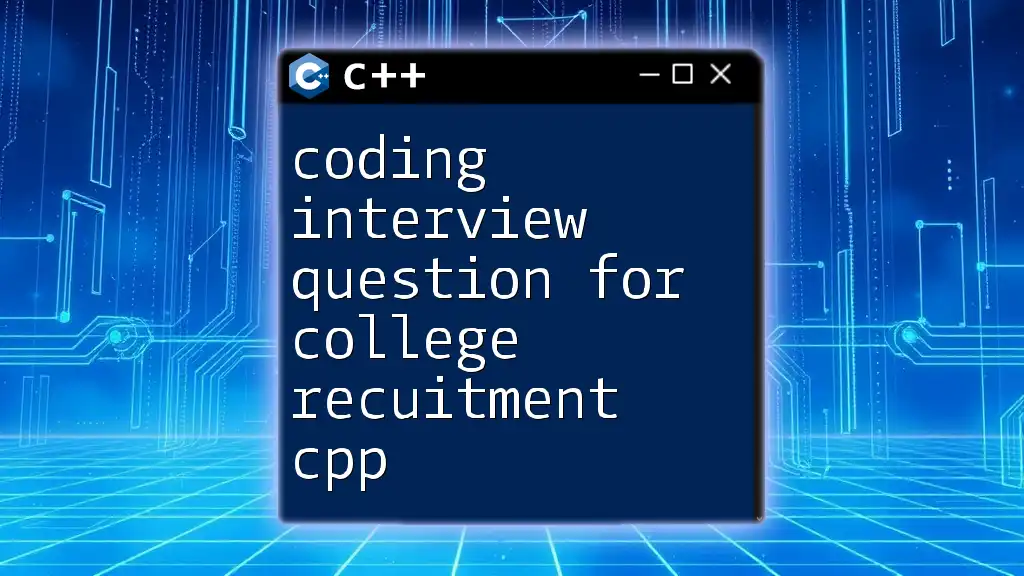
Common C++ Interview Questions
Syntax and Semantics
What is the difference between `struct` and `class` in C++?
While both `struct` and `class` in C++ are used to create user-defined types, the key distinction lies in their default access modifiers. Specifically, members of a `struct` are public by default, whereas members of a `class` are private by default. This distinction affects how data encapsulation is managed in your code.
Here’s a brief example:
struct Point {
int x; // Public by default
};
class Circle {
private:
int radius; // Private by default
public:
void setRadius(int r) { radius = r; }
};
Can you explain the concept of RAII (Resource Acquisition Is Initialization)?
RAII is a programming idiom used to manage resource allocation and deallocation automatically. It ties resource management to object lifetime, ensuring that resources such as memory or file handles are released when the object goes out of scope. Smart pointers (like `std::unique_ptr` and `std::shared_ptr`) in modern C++ exemplify RAII principles:
#include <memory>
std::shared_ptr<int> ptr = std::make_shared<int>(10); // Automatically managed memory
This not only increases safety by preventing memory leaks but also enhances code readability and maintainability.
Advanced C++ Topics
What are templates and how do they work?
Templates in C++ enable writing generic and reusable code. They allow you to create functions and classes that operate independently of the specific types being manipulated. This promotes versatility and reduces code duplication.
Example of a function template:
template <typename T>
T add(T a, T b) {
return a + b;
}
In this snippet, `add` is defined as a template function that can operate on any data type, enhancing flexibility in your programs.
Understanding the Standard Template Library (STL)
The Standard Template Library (STL) is a powerful feature of C++ that provides a rich set of template classes to manage collections of data. It consists of containers (like `vector`, `list`, `map`), algorithms (like `sort`, `find`), and iterators which facilitate data traversal.
A simple demonstration of using a vector:
#include <vector>
std::vector<int> v = {1, 2, 3, 4};
for(auto i : v) {
std::cout << i << " "; // Output: 1 2 3 4
}
In this example, the `vector` container dynamically handles storage and provides a simple way to iterate through the elements.
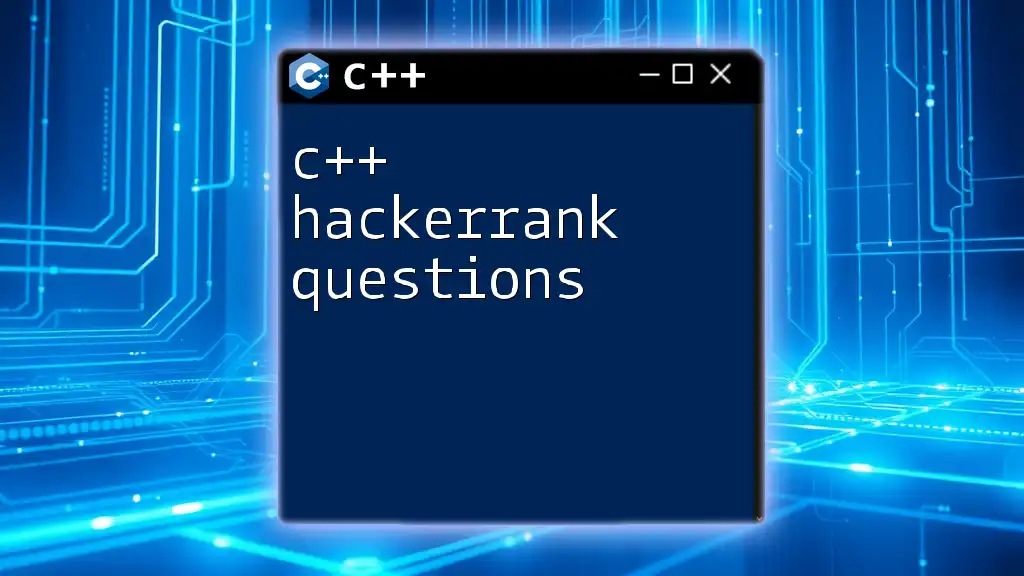
Practical Coding Problems
Coding Challenges
Reverse a String
A common question in C++ interviews is to reverse a string. The challenge tests your grasp on string manipulation.
Problem Statement: Write a function that reverses a string.
Solution:
std::string reverse(const std::string& str) {
return std::string(str.rbegin(), str.rend());
}
In this code, `rbegin` and `rend` create reverse iterators, allowing easy reversal without complex looping methods.
Find the Largest Element in an Array
Another standard interview question involves locating the largest number in an array.
Problem Statement: Write a function that finds the largest element in an integer array.
Solution:
int largestElement(int arr[], int size) {
int max = arr[0];
for(int i = 1; i < size; i++) {
if(arr[i] > max) max = arr[i];
}
return max;
}
This function initializes the maximum to the first element and iteratively compares to find the largest value.
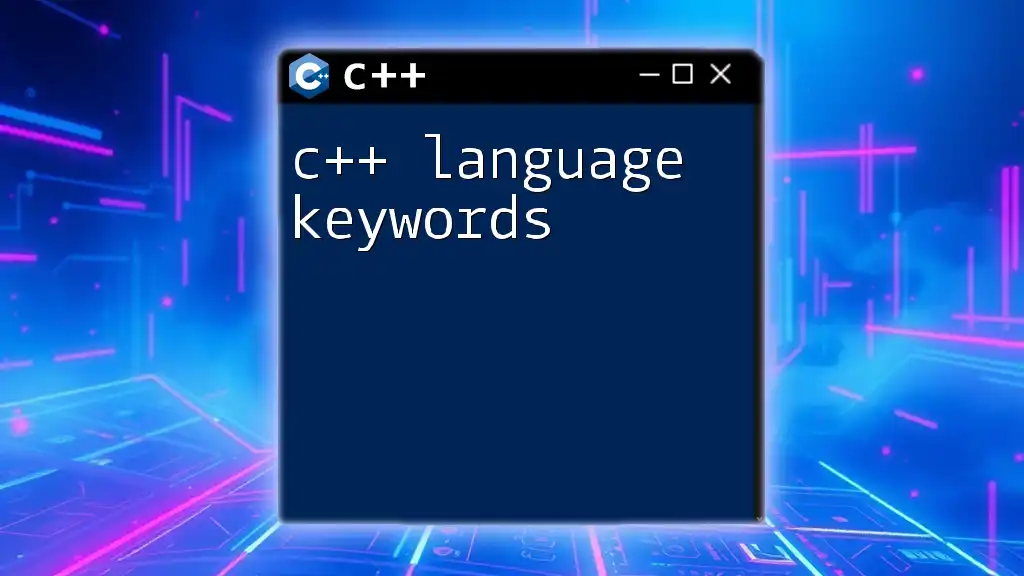
Tips for Acing C++ Interviews
Preparation Strategies
A strategic approach to preparing for C++ language interview questions includes a thorough study of core concepts and consistent practice with coding problems. Books like "The C++ Programming Language" by Bjarne Stroustrup prove invaluable, along with reputable online platforms such as LeetCode and HackerRank for hands-on coding practice.
Mock Interviews
Conducting mock interviews is essential in simulating the actual interview environment. Choose a peer or utilize online platforms to engage in mock sessions focusing on both coding speed and accuracy. Always be ready to explain your thought process and the rationale behind your coding decisions.
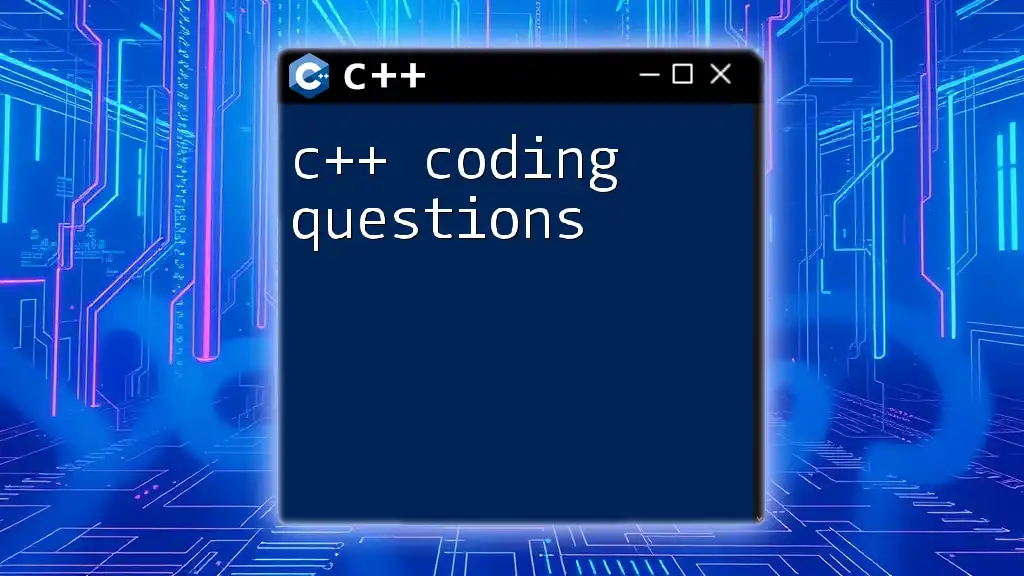
Conclusion
In conclusion, preparing for C++ language interview questions not only hones your coding skills but also deepens your understanding of the language's intricate functionalities. Mastering these concepts and practicing with real-world scenarios will significantly boost your confidence and performance in interviews.
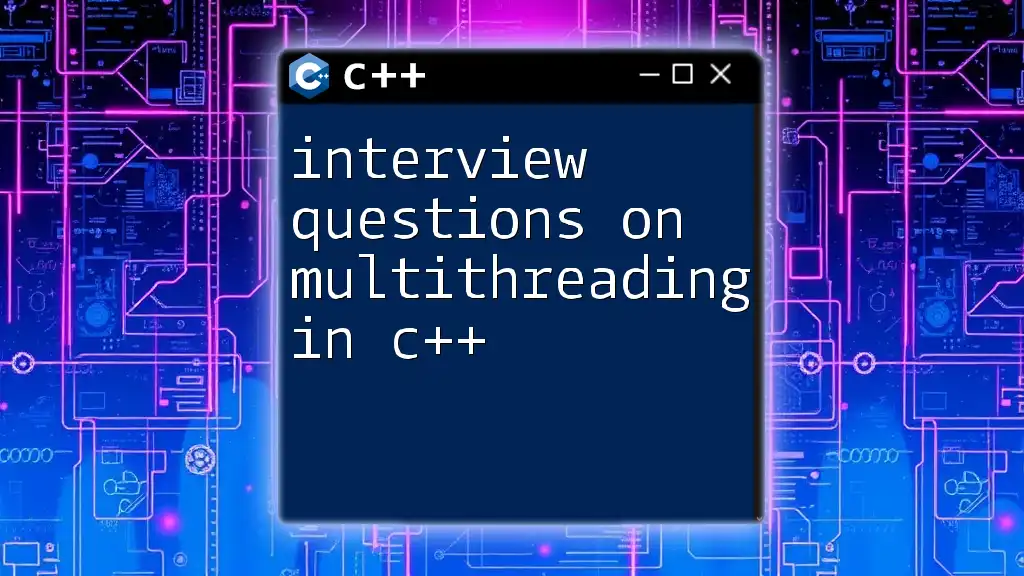
Additional Resources
To further enhance your C++ knowledge, explore online forums, tutorial websites, and community discussions. Engage with resources like C++ reference guides, textbooks, and video tutorials to stay updated and connected with other programming enthusiasts.