In this post, we will explore a common coding interview question for college recruitment that tests your understanding of C++ fundamentals, as demonstrated in the following code snippet that reverses a string.
#include <iostream>
#include <string>
#include <algorithm>
int main() {
std::string str = "Hello, World!";
std::reverse(str.begin(), str.end());
std::cout << str << std::endl; // Output: !dlroW ,olleH
return 0;
}
Understanding Coding Interview Questions
Types of Coding Questions
Coding interviews often assess a candidate's problem-solving skills and their understanding of fundamental concepts. There are three main types of coding questions you can expect:
- Algorithmic problems: These questions typically require you to devise algorithms to solve a given problem effectively.
- Data structures questions: Often focused on your understanding of how various data structures operate—like arrays, linked lists, trees, etc.
- System design questions: Mostly for more experienced candidates, even beginners can benefit from a basic understanding of how to design systems effectively.
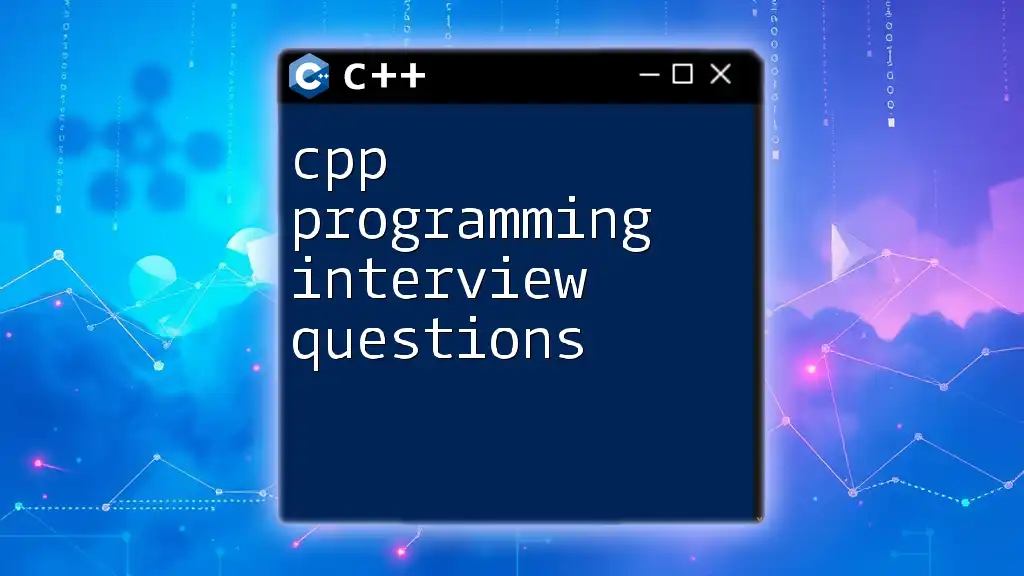
Essential C++ Concepts to Review
Basic Syntax and Structure
A solid foundation in C++ syntax is crucial for successfully navigating coding interview questions. Understanding how to structure a C++ program lays the groundwork for everything else.
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Data Types and Variables
C++ offers various data types, each suited for specific purposes:
- int: For integers.
- float: For floating-point numbers.
- char: For characters.
- string: For strings.
Knowing when to use each type can significantly affect both memory allocation and processing time, which can be crucial during an interview. Here’s an example of declaring and initializing variables:
int age = 25;
float salary = 50000.50;
char grade = 'A';
string name = "John Doe";
Control Structures
Control structures dictate the flow of execution in programming. Here’s a brief overview:
- If-else statements: Useful for conditional execution.
if (age >= 18) {
cout << "Eligible to vote" << endl;
} else {
cout << "Not eligible to vote" << endl;
}
- Switch-case statements: Ideal for handling multiple conditions efficiently.
switch (grade) {
case 'A':
cout << "Excellent" << endl;
break;
case 'B':
cout << "Good" << endl;
break;
default:
cout << "Needs Improvement" << endl;
}
- Looping mechanisms (for, while, do-while): These constructs allow you to execute a block of code multiple times.
for (int i = 0; i < 5; i++) {
cout << i << endl;
}
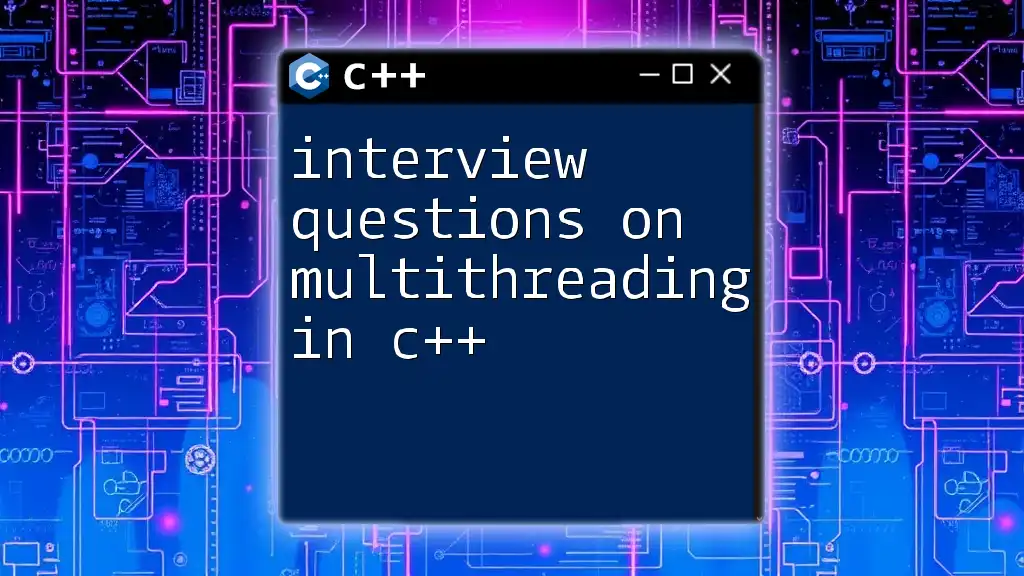
Common C++ Coding Interview Questions
String Manipulation
String manipulation is a common area for interviews. Here are two example questions:
- Example Question: "Reverse a string."
string reverseString(string s) {
reverse(s.begin(), s.end());
return s;
}
This function utilizes the `reverse` from the `<algorithm>` library to reverse the string efficiently.
- Example Question: "Check if a string is a palindrome."
bool isPalindrome(string s) {
string reversed = reverseString(s);
return s == reversed;
}
This leverages the earlier function and checks equivalency, demonstrating both string manipulation and the use of functions.
Array and Vector Problems
Arrays and vectors encompass an extensive range of problems in coding interviews.
- Example Question: "Find the maximum element in an array."
int findMax(int arr[], int size) {
int maxValue = arr[0];
for (int i = 1; i < size; i++) {
if (arr[i] > maxValue) {
maxValue = arr[i];
}
}
return maxValue;
}
This simple loop traverses the array to find the maximum value, showcasing basic iterative logic.
- Example Question: "Remove duplicates from a sorted array."
int removeDuplicates(int arr[], int size) {
if (size == 0) return 0;
int uniqueIndex = 1;
for (int i = 1; i < size; i++) {
if (arr[i] != arr[i - 1]) {
arr[uniqueIndex++] = arr[i];
}
}
return uniqueIndex;
}
This code demonstrates an efficient way to filter through a sorted array while maintaining a linear complexity.
Linked Lists
Linked lists often appear for more advanced coding questions.
- Example Question: "Implement a function to reverse a linked list."
Understanding how linked lists operate is fundamental.
struct Node {
int data;
Node* next;
};
Node* reverseLinkedList(Node* head) {
Node* prev = nullptr;
Node* current = head;
Node* next = nullptr;
while (current) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
return prev;
}
This function effectively reverses the linked list by adjusting the pointers within the nodes.
- Example Question: "Detect a cycle in a linked list."
bool hasCycle(Node* head) {
Node* slow = head;
Node* fast = head;
while (fast != nullptr && fast->next != nullptr) {
slow = slow->next;
fast = fast->next->next;
if (slow == fast) {
return true; // Cycle found
}
}
return false; // No cycle
}
Using the slow and fast pointer technique demonstrates efficient cycle detection.
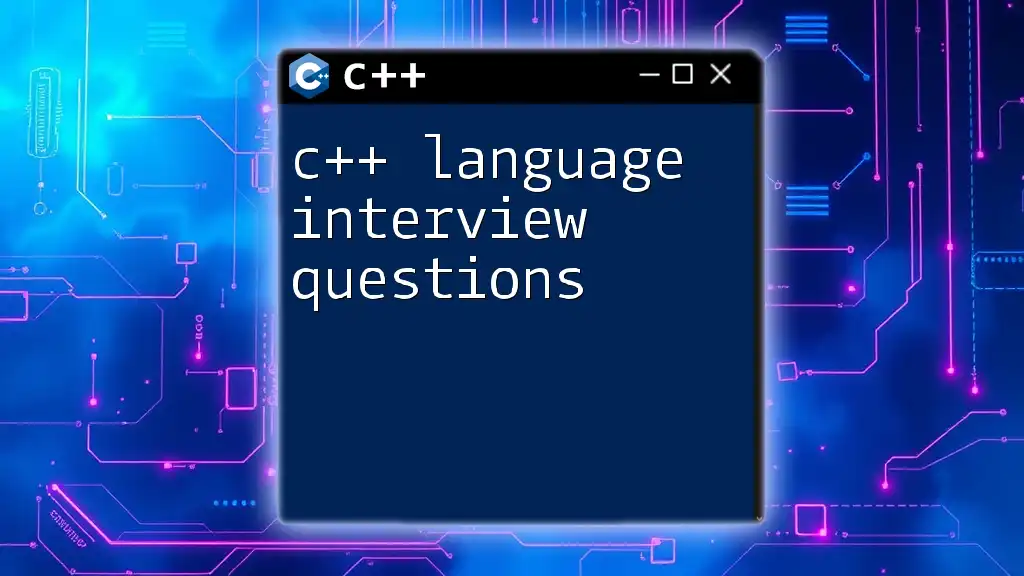
Working with Classes and Objects
Object-Oriented Programming in C++
Object-oriented programming is a key concept in C++. It not only organizes code better but also encourages reuse.
- Example Question: "Design a simple bank account class."
class BankAccount {
private:
double balance;
public:
BankAccount() : balance(0.0) {}
void deposit(double amount) {
balance += amount;
}
void withdraw(double amount) {
if (amount <= balance) {
balance -= amount;
}
}
double getBalance() {
return balance;
}
};
This class encapsulates the essential operations for a bank account, exemplifying principles of encapsulation.
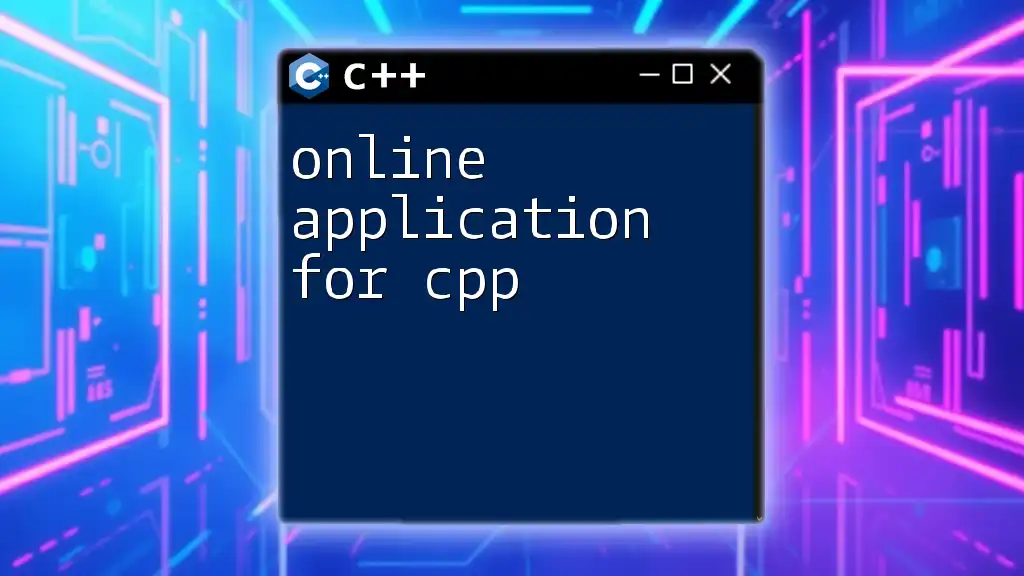
Advanced C++ Topics
Templates and STL (Standard Template Library)
Understanding templates can vastly improve your flexibility in coding.
- Example Question: "Write a function that swaps two numbers using templates."
template <typename T>
void swap(T& a, T& b) {
T temp = a;
a = b;
b = temp;
}
Using templates allows this function to handle input of any data type, showcasing versatility.
Memory Management
Effective memory management is crucial in languages like C++.
Dynamic memory allocation and management can be the difference between efficient usage and memory leaks.
-
Example Short Question: “Explain the difference between new/delete and malloc/free.”
new/delete operates in C++ with constructors and destructors and automatically calls these functions. Conversely, malloc/free from C is more rudimentary, focusing solely on memory allocation. Understanding these nuances can significantly enhance your performance in interviews.
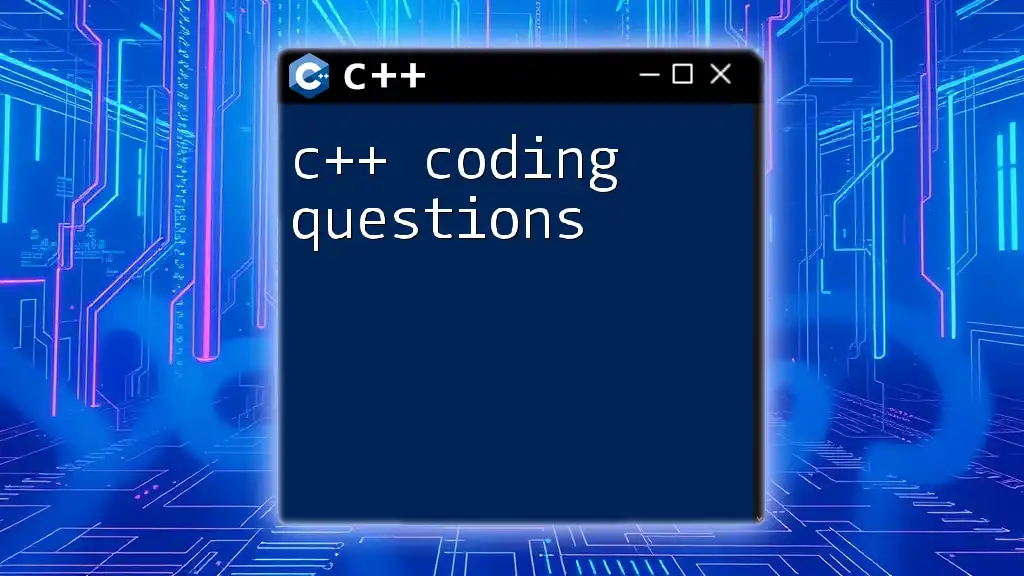
Problem-Solving Strategies
Best Practices for Coding Interviews
The key to solving coding interview questions effectively lies in a few best practices:
- Understanding the problem requirements: Ensure you grasp what is being asked before diving into the solution.
- Breaking down complex problems: Simplifying can lead to better logical flow and easier coding.
- Testing your code: Place emphasis on edge cases to ensure robustness.
Common Mistakes to Avoid
Awareness of common pitfalls can set you apart:
- Overcomplicating solutions: Aim for clarity as well as functionality.
- Neglecting edge cases: Always consider potential anomalies to avoid bugs.
- Focusing solely on syntax: Logical flow is just as vital as writing correct C++ syntax.
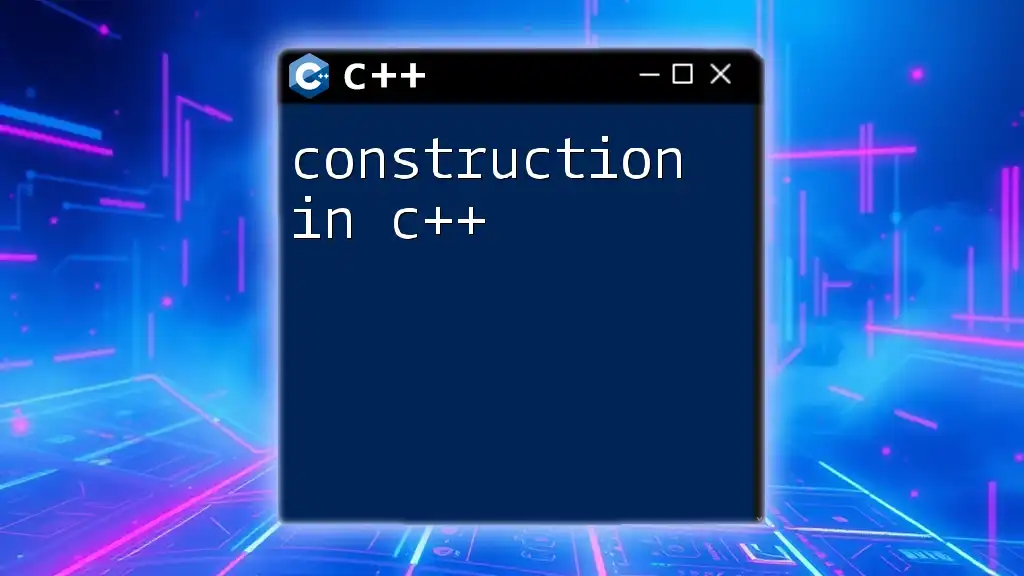
Practice Resources
Online Coding Platforms
Make use of various online coding platforms like LeetCode, HackerRank, or Codecademy. These platforms provide ample coding problems with varying difficulty levels, perfect for preparing for interviews.
Books and Online Courses
Consider reading books such as "Cracking the Coding Interview" or online courses tailored to C++ programming. These resources not only delve into C++ specifics but also general preparation for coding interviews.
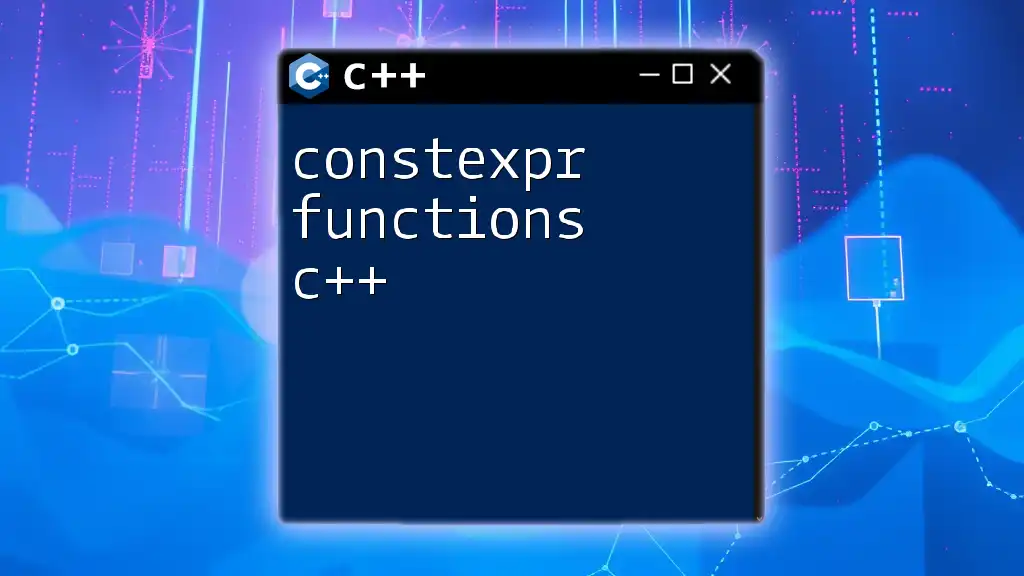
Conclusion
In summary, brushing up on C++ fundamentals and practicing problems related to coding interviews is key to performing well in college recruitment interviews. Understanding core concepts and diligently practicing will bolster your confidence and skill set.
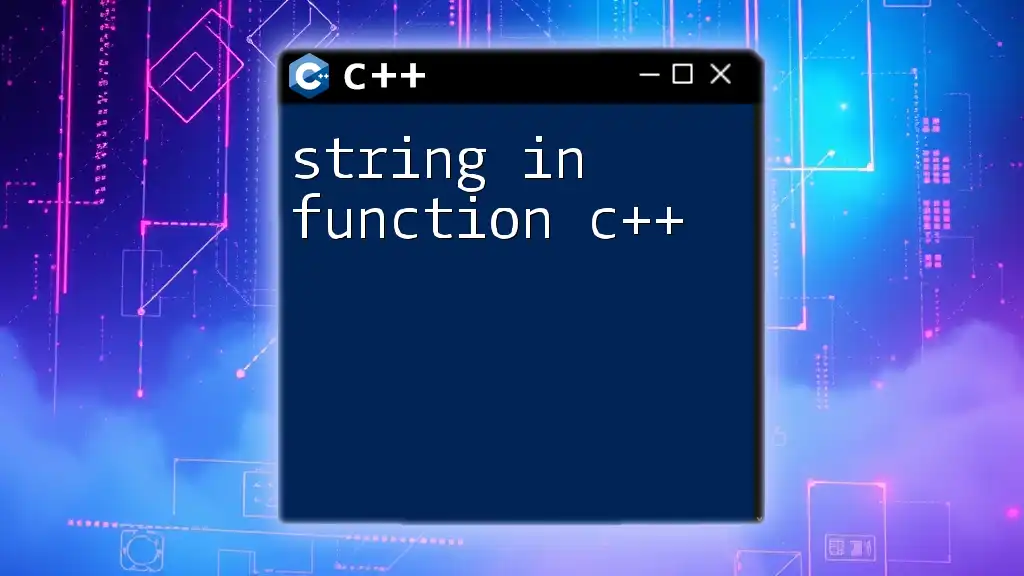
Call to Action
Join our C++ learning community today! Engage with us for workshops and tutorials designed specifically to help you master C++ and excel in your coding interviews. Your journey to acing those coding interview questions for college recruitment in C++ begins now!