Insertion sort is a simple and efficient sorting algorithm that builds the final sorted array one item at a time, as demonstrated in the following C++ code snippet:
#include <iostream>
using namespace std;
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
int main() {
int arr[] = {12, 11, 13, 5, 6};
int n = sizeof(arr) / sizeof(arr[0]);
insertionSort(arr, n);
for (int i = 0; i < n; i++)
cout << arr[i] << " ";
return 0;
}
How Insertion Sort Works
Algorithm Explanation
Insertion Sort is a straightforward sorting algorithm that builds a sorted array one element at a time. It works by taking an element from the unsorted part of the array and finding its correct position by shifting other elements to make space. The algorithm can be visualized as sorting a hand of playing cards, where you pick a card and insert it into the correct position among the already sorted cards.
A typical step in the Insertion Sort can be broken down as follows:
- Initialization: Start from the second element (index 1) of the array, treating the first element as a sorted subarray.
- Finding Position: Compare the current element (the key) with the elements in the sorted subarray.
- Shifting Elements: If the elements in the sorted subarray are greater than the key, shift them one position to the right.
- Insertion: Insert the key at its correct location.
This algorithm is particularly effective for small datasets or partially sorted data, as its simplicity leads to good performance in these scenarios.
Time Complexity
Insertion Sort has varying time complexities based on the nature of the input dataset:
- Best Case: O(n). This occurs when the array is already sorted. Each element only needs to be compared once.
- Average Case: O(n²). This is typical for unsorted data, where each element is compared to every other element in the worst scenario.
- Worst Case: O(n²). This happens when the array is sorted in reverse order, requiring maximum comparisons and shifts.
In terms of space complexity, Insertion Sort is O(1) because it only requires a constant amount of additional space for the key variable, making it in-place.
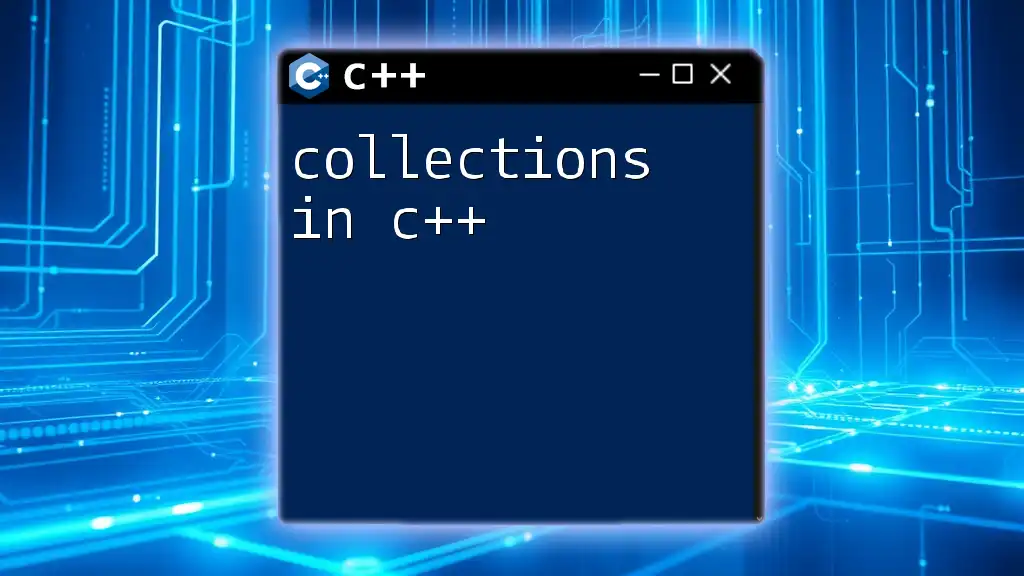
Setting up a C++ Environment
Before coding, you need a C++ development environment. Here’s how to set it up:
- Software Requirements: Install a C++ compiler. Popular choices include GCC, Clang, or Microsoft Visual Studio (MSVC).
- IDE: You can choose an Integrated Development Environment (IDE) like Code::Blocks, Eclipse, or Visual Studio for coding convenience.
- Running Your First C++ Program: After the installation, you can create a simple "Hello World" program to ensure everything is working correctly.
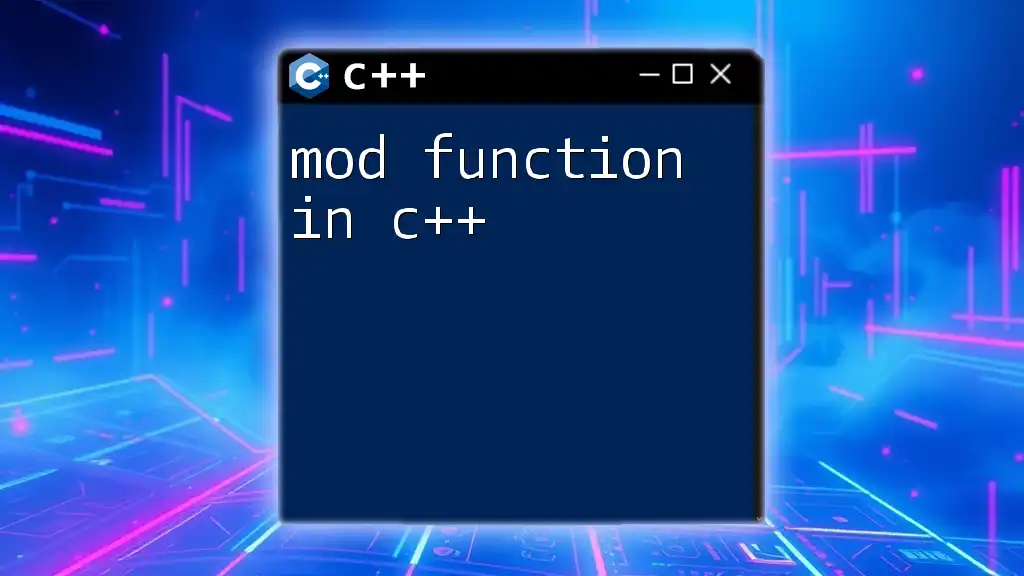
Implementation of Insertion Sort in C++
Basic Implementation
Now that we understand the algorithm, let’s dive into its implementation in C++.
void insertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j--;
}
arr[j + 1] = key;
}
}
In this code snippet, we declare a function `insertionSort` that takes an array and its size as parameters. The outer `for` loop iterates through each element starting from the second one, while the inner `while` loop is used to shift elements. The key is inserted into its appropriate position after all necessary elements are shifted.
Example: If we run the above implementation on an array like `{5, 2, 9, 1, 5, 6}`, it will gradually shift elements to yield the sorted output `{1, 2, 5, 5, 6, 9}`.
Optimized Implementation
To enhance efficiency, we can implement a more optimized version of Insertion Sort that reduces the number of shifts. This version uses a binary search approach to find the insertion point.
void optimizedInsertionSort(int arr[], int n) {
for (int i = 1; i < n; i++) {
int key = arr[i];
int left = 0, right = i - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] <= key)
left = mid + 1;
else
right = mid - 1;
}
for (int j = i; j > left; j--) {
arr[j] = arr[j - 1];
}
arr[left] = key;
}
}
In this enhanced version, a binary search is employed to find the proper index for the key, resulting in fewer comparisons. This reduces the number of shifts needed when inserting the key, thereby optimizing the overall efficiency.
Example: Running this optimized code on a larger array would demonstrate performance improvements, especially in cases with many elements.
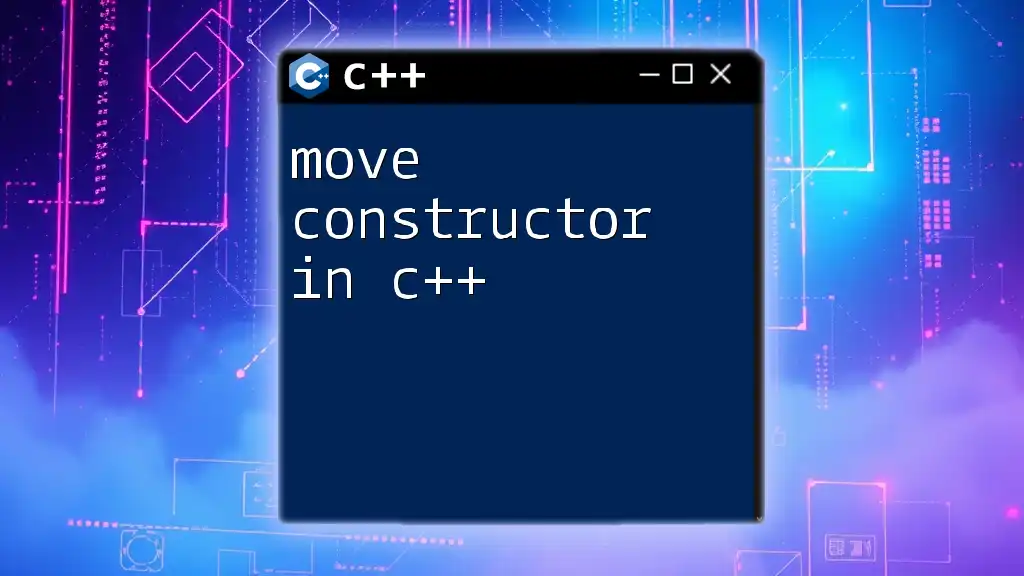
Visual Representation of Insertion Sort
Step-by-Step Illustration
Although visuals cannot be presented here, think of the sorting process as a metaphorical hand of cards. Each time an element is inserted into the sorted section, you can imagine the current element being placed in a specific spot, pushing other cards to the side.
Debugging Tips
Common issues when implementing Insertion Sort can include:
- Off-by-one errors in index management. Double-check loop boundaries and indexing.
- Not correctly handling edge cases, such as empty arrays or arrays with a single element.
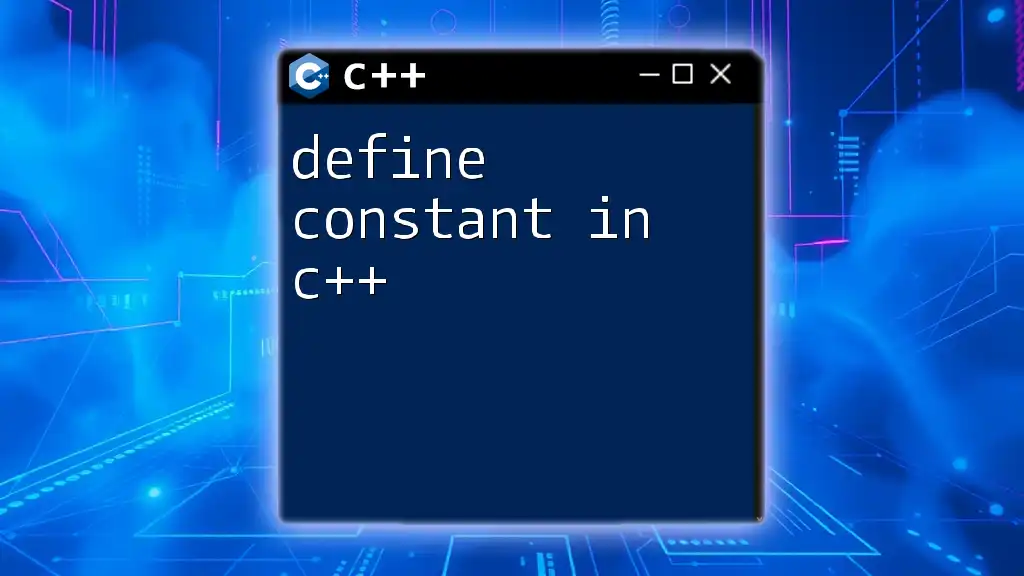
Real-World Applications of Insertion Sort
Insertion Sort is efficient for small or nearly sorted datasets. In real-world applications, you might find it in:
- Algorithm libraries: Often as a component of more complex sorting algorithms when sorting small chunks.
- Real-time systems: Where speed is more crucial than memory usage, such as in certain embedded systems.
By understanding the intrinsic nature of Insertion Sort, developers can better utilize it in appropriate contexts, enhancing both performance and efficiency.
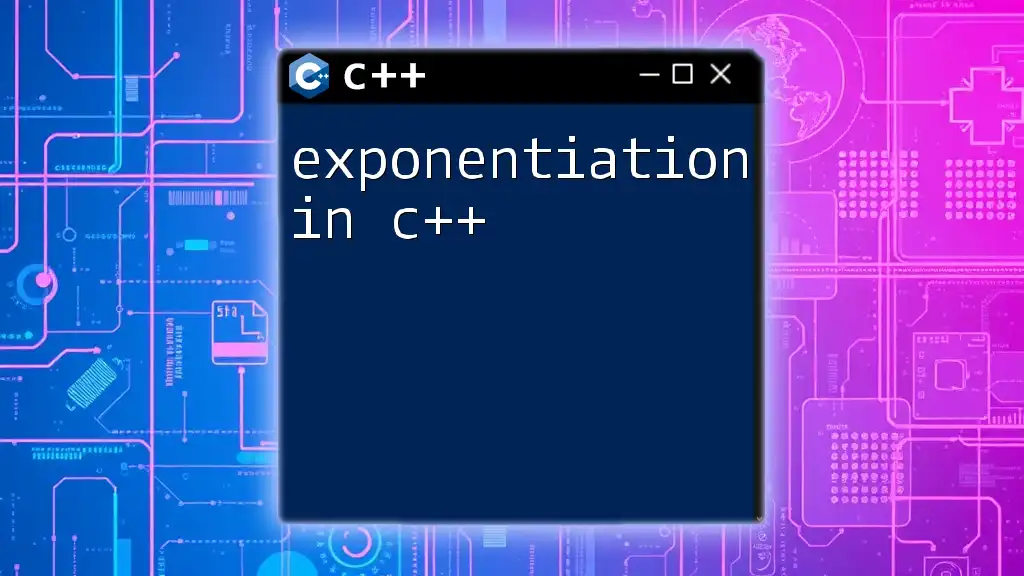
Conclusion
In this article, we explored the code for insertion sort in C++, covering its principles, implementation strategies, time complexities, real-world applications, and debugging approaches. By contrast with other sorting algorithms, Insertion Sort is particularly valuable for its simplicity and efficiency in certain conditions. As you practice and experiment with the code, you'll gain a deeper understanding of sorting principles in C++. Keep exploring and refining your coding skills!
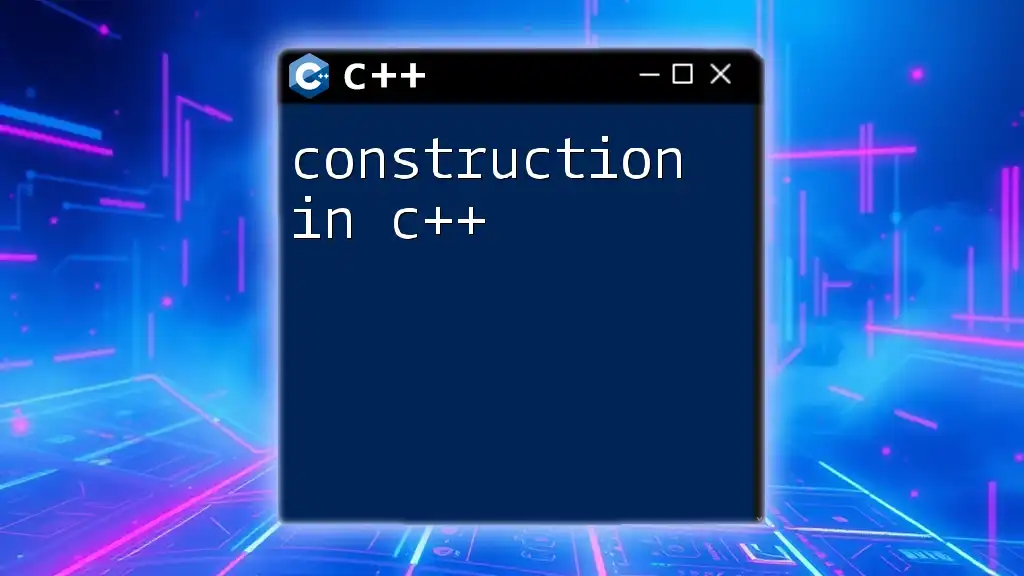
Additional Resources
For further guidance and to enhance your knowledge, refer to:
- Recommended textbooks on algorithms and data structures.
- Online platforms like Codecademy or Coursera for C++ courses.
- Community forums like Stack Overflow for peer support and sharing insights.